Considerations for API testing
This is a book about how to use Postman to make better APIs. Part of making a better API is testing it. There is a lot to API testing, and I will walk you through many aspects of it as we go through this book, but now that you know some of the basics of how an API request works, what are some of the things to consider when you test an API?
Beginning with exploration
I can still clearly remember the first time I saw a modern web API in action. The company I was working at was building a centralized reporting platform for all the automated tests and I was assigned to help test the reporting platform. One of the key aspects of this platform was the ability to read and manipulate data through a web API. As I started testing this system, I quickly realized how powerful this paradigm was.
Another part of my job at that time was to work with a custom-built test automation system. This system was quite different from the more standard test automation framework that many others in the company were using. However, the fact that the new reporting platform had an API meant that my custom test automation system could put data into this reporting platform, even though it worked very differently to the other test automation systems. The test reporting application did not need to know anything about how my system, or any other one, worked. This was a major paradigm shift for me and was probably instrumental in leading me down the path to where I am writing this book. However, something else I noticed as I tested this was that there were flaws and shortcomings in the API.
It can be tempting to think that all API testing needs to be done programmatically, but I would argue that the place to start is with exploration. When I tested the API for that test reporting platform, I barely knew how to use an API, let alone how to automate tests for it, and yet I found many issues that we were able to correct. If you want to improve the quality of an API, you need to understand what it does and how it works. You need to explore it.
But how do you do that?
Thankfully, Postman is one of the best tools out there for exploring APIs. With Postman, you can easily try out many different endpoints and queries and you can get instant feedback on what is going on in the API. Postman makes it easy to play around with an API and to go from one place to another. Exploring involves following the clues that are right in front of you. As you get results back from a request, you want to think of questions that those results bring to mind and try to answer them with further calls. This is all straightforward with Postman. To Illustrate, I will walk you through a case study of a short exploratory session using Postman.
For this session, I will use the https://swapi.dev/ API. This is a fun little API that exposes data about the Star Wars movies. Don't worry if you aren't into Star Wars. No specialized knowledge is needed!
Exploratory testing case study
Let's try this out. First of all, create a new collection called Star Wars API and add a request to it called Get People. Put https://swapi.dev/api/people/1/ into the URL field and send that request. You should get back a response with some data for the character Luke Skywalker:
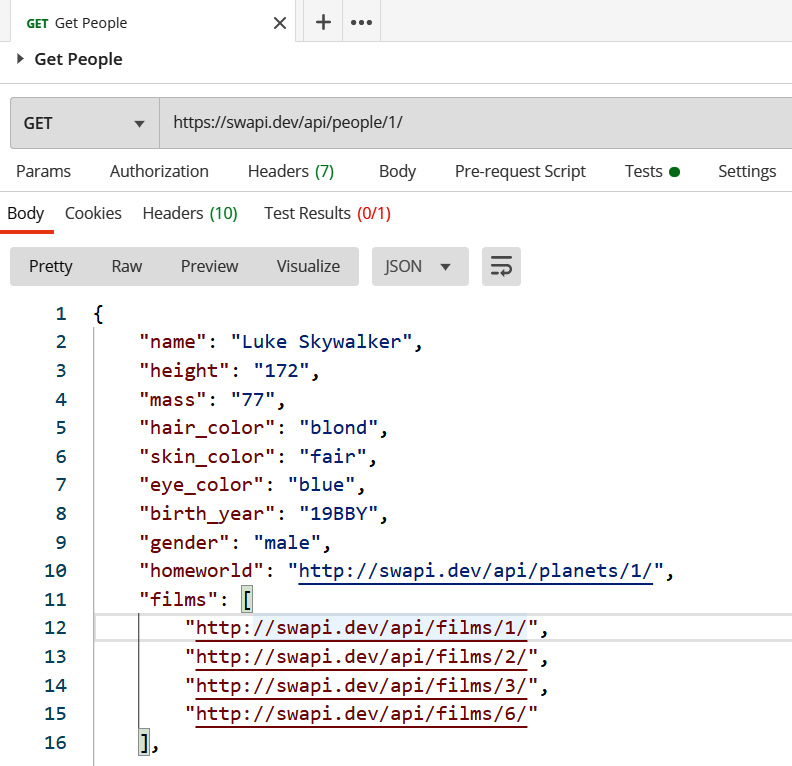
Figure 1.7 – Response for the Star Wars API request
In the response, there are links to other parts of the API. A response that includes links to other relevant resources is known as a Hypermedia API. The first link in the films list will give us information about the film with ID 1. Since we are exploring, let's go ahead and look at that link and see what it does. You can just click on it and Postman will open a new requests tab with it already loaded. At this point, you know what to do: just click on Send and Postman will give you data about that first film. Under the characters in that film, you can see that there are several different characters, including, of course, a link to /people/1
.
Seeing this triggers a thought that there might be more things to check in the /people
API, so let's go back and explore that part of the API a bit more. Click on the Get People tab to go back to that request and change the URL to remove the /1 from the end of it. You can now click Send to send a request to the endpoint, https://swapi.dev/api/people. This response gives back a list of the different people in the database. You can see at the top of the response that it says there is a count of 82.
We are in exploration mode, so we ask the question, "I wonder what would happen if I tried to request person number 83?" This API response seems to indicate that there are only 82 people, so perhaps something will go wrong if we try to get a person that is past the end of this dataset. In order to check this, add /83
to the end of the URL and click Send again. Interestingly (if the API hasn't changed since I wrote this), we get back data for a Star Wars character. It seems like either the count is wrong, or perhaps a character has been removed somewhere along the way. Probably we have just stumbled across a bug!
Whatever the case may be, this illustrates just how powerful a little bit of exploration can be. We will get to some powerful ways to use Postman for test automation later in this book, but don't rush right past the obvious. API testing is testing. When we are testing, we are trying to find out new information or problems that we might have missed. If we rush straight to test automation, we might miss some important things. Take the time to explore and understand APIs early in the process.
Exploration is a key part of any testing, but it takes more than just trying things out in an application. Good testing also requires the ability to connect the work you are doing to business value.
Looking for business problems
When considering API testing and design, it is important to consider the business problem that the API is solving. An API does not exist in a vacuum. It is there to help the company meet some kind of business need. Understanding what that need is will help to guide the testing approaches and strategies that you take. For example, if an API is only going to be used by internal consumers, the kinds of things that it needs to do and support are very different to those needed if it is going to be a public API that external clients can access.
When testing an API, look for business problems. If you can find problems that prevent the API from doing what the business needs it to do, you will be finding valuable problems and enhancing the quality of the application. Not all bugs are created equal.
Trying weird things
Not every user of an API is going to use it in the way that those who wrote the API thought they would. We are all limited by our own perspectives on life and it is hard to get into someone else's mind and see things through their perspective. We can't know every possible thing that users of our system will do, but there are strategies that can help you get better at seeing things in a different light. Try doing some things that are just weird or strange. Try different inputs and see what happens. Mess around with things that seem like they shouldn't be messed with. Do things that seem weird to you. Often, when you do this, nothing will happen, but occasionally it will trigger something interesting that you might never have thought of otherwise.
Testing does not need to be mindless repetition of the same test cases. Use your imagination. Try strange and interesting things. See what you can learn. The whole point of this book is for you to learn how to use a new tool. The fact that you have picked up this book shows that you are interested in learning. Take that learning attitude into your testing. Try something weird and see what happens.
There is obviously a lot more to testing than just these few considerations that I have gone over here. However, these are some important foundational principles for testing. I will cover a lot of different ways to use Postman for testing in this book, but most of the things that I talk about will be examples of how to put these strategies into practice. Before moving on to more details on using Postman though, I want to give you a brief survey of some of the different types of APIs that you might encounter.