Preparing your system
To be able to use gRPC on ASP.NET Core, you will need an integrated development environment (IDE) or a code editor that has full .NET support. You will also need the latest software development kit (SDK) version of .NET, which, at the time of writing, is .NET 5.
Other than these components, you don't need anything else to start developing a gRPC application for .NET. It's already included in the framework. And whenever you need an add-on library, you will be instructed on how to obtain it.
Because .NET is an OS-independent framework, you can write applications for it on either Windows, Mac, or Linux. However, your setup steps will be slightly different, so please follow the section that is relevant to your system.
Setting up your environment on Windows
On Windows, you have three main options regarding an IDE for .NET. They are listed here in order of preference, based on how many features they have and how easy they are to use:
- JetBrains Rider
- Microsoft Visual Studio
- Visual Studio Code
Rider is a fully functioning IDE. Compared to the other options, it has many additional tools. It's also easier to optimize and configure.
The downside of Rider is that it's only available as a paid-for premium, although a 30-day free trial is available for new users.
To download Rider, navigate to https://www.jetbrains.com/rider/download/ and follow the setup instructions provided:
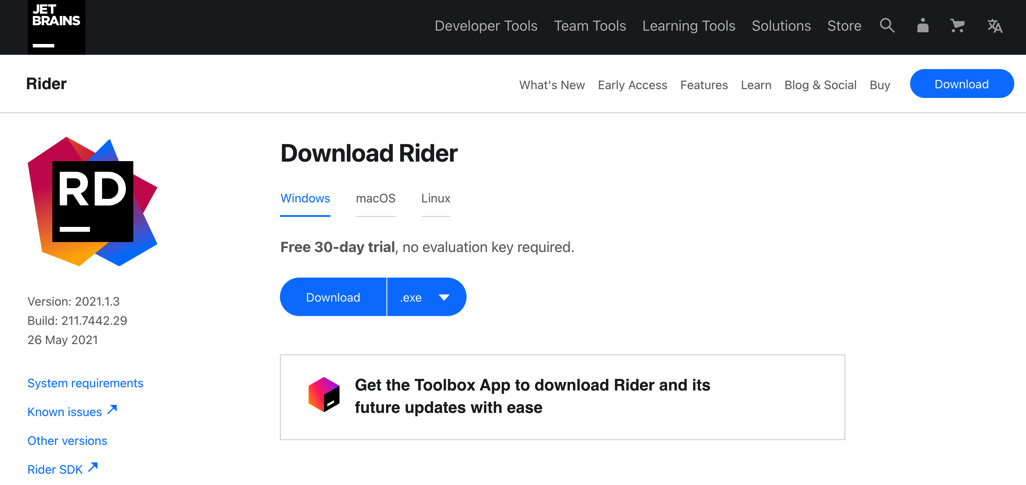
Figure 1.1 – JetBrains Rider download page
Alternatively, you can download Visual Studio. It's the official IDE for .NET from Microsoft. And, unlike Rider, it has a free tier version known as Community Edition.
To download the latest version of Visual Studio (Visual Studio 2019, at the time of writing), go to https://visualstudio.microsoft.com/downloads/:
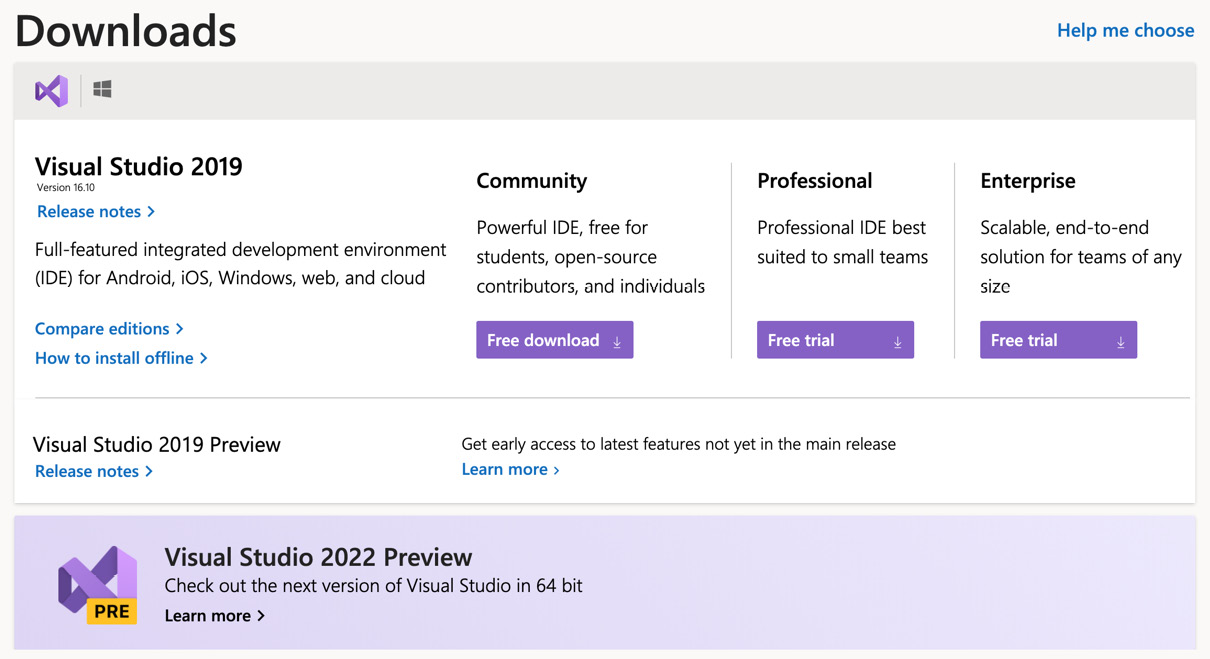
Figure 1.2 – Microsoft Visual Studio download page
Lastly, there is Visual Studio Code, which, despite sounding similar to Visual Studio, is a completely different product. While Visual Studio is a fully-fledged IDE, Visual Studio Code is merely a code editor.
However, despite being just a code editor, it's still a powerful tool that you can develop your code in. And it's highly configurable, so you will be able to use it to write code in many different languages, not just the ones that are specific to .NET.
The advantage of Visual Studio Code over either Visual Studio or Rider is that it's lightning-fast. Because it's just an editor that lacks many tools that IDEs have, it has far fewer things to load and run in the background.
The disadvantage of using Visual Studio Code over either Visual Studio or Rider is that, as a code editor, it lacks some basic features that are typically embedded into an IDE. For example, you will not be able to compile your project without integrating the editor with some add-on tool or using the CLI.
Visual Studio Code can be obtained via https://code.visualstudio.com/download:
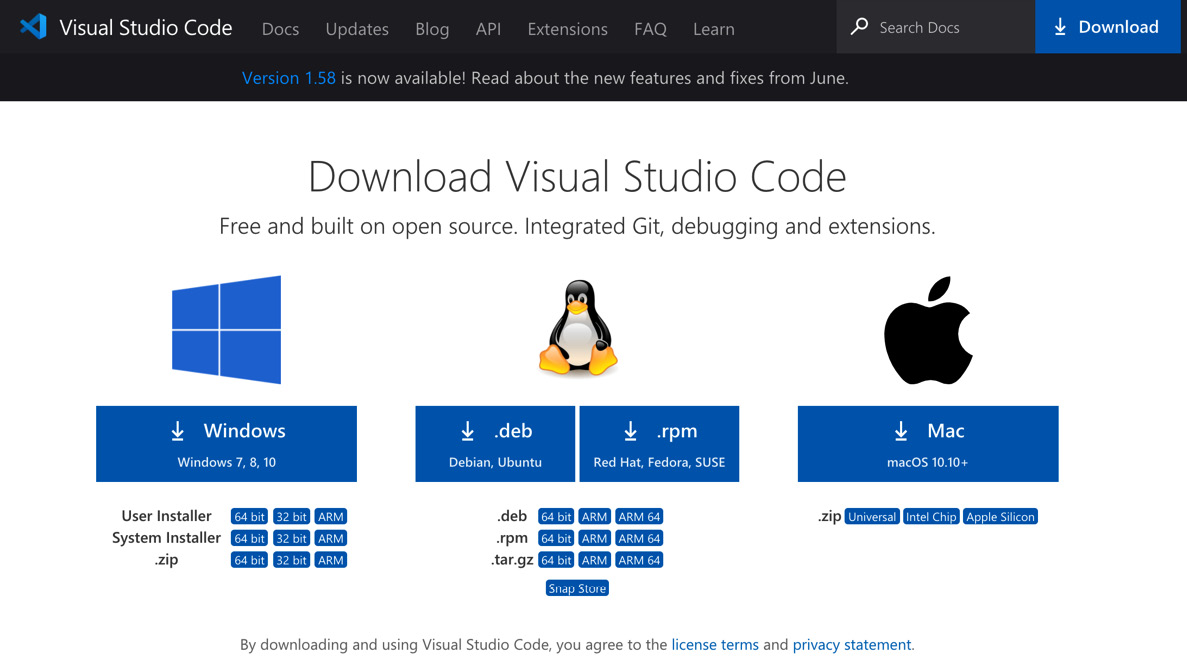
Figure 1.3 – Visual Studio Code download page
Once you have downloaded your preferred IDE, you can proceed with its installation.
Setting up your environment on Mac
On Mac, you have three main options regarding an IDE for .NET. They are listed here in their order of preference, based on how many features they have and how easy they are to use:
- JetBrains Rider
- Microsoft Visual Studio for Mac
- Visual Studio Code
Rider is a fully functioning IDE. Compared to the alternative options, it has many additional tools. It's also easier to optimize and configure.
The downside of Rider is that it's only available as a paid-for premium, although a 30-day free trial is available for new users.
To download Rider, navigate to https://www.jetbrains.com/rider/download/ and follow the setup instructions provided.
Alternatively, you can download Visual Studio for Mac. It's the official IDE for .NET from Microsoft. And, unlike Rider, it can be downloaded for free.
To download the latest version of Visual Studio for Mac, go to https://visualstudio.microsoft.com/vs/mac.
Lastly, there is Visual Studio Code, which, despite sounding similar to Visual Studio for Mac, is a completely different product. While Visual Studio for Mac is a fully-fledged IDE, Visual Studio Code is merely a code editor.
However, despite being just a code editor, it's still a powerful tool for developing your code. And it's highly configurable, so you will be able to use it to write code in many different languages, not just the ones that are specific to .NET.
The advantage of Visual Studio Code over either Visual Studio for Mac or Rider is that it's lightning-fast. Because it's just an editor that lacks many tools that IDEs have, it has far fewer things to load and run in the background.
The disadvantage of using Visual Studio Code over either Visual Studio for Mac or Rider is that, as a code editor, it lacks some basic features that are embedded into an IDE. For example, you will not be able to compile your project without integrating the editor with some add-on tool or using the CLI.
Visual Studio Code can be obtained via https://code.visualstudio.com/download.
Setting up your environment on Linux
On Linux, you have two main options regarding an IDE for .NET. They are listed here in their order of preference, based on how many features they have and how easy they are to use:
- JetBrains Rider
- Visual Studio Code
Rider is a fully functioning IDE. Compared to the alternative options, it has many additional tools. It's also easier to optimize and configure.
The downside of Rider is that it's only available as a paid-for premium, although a 30-day free trial is available for new users.
To download Rider, navigate to https://www.jetbrains.com/rider/download/ and follow the setup instructions provided.
Lastly, there is Visual Studio Code, which is a highly configurable code editor, so you will be able to use it to write code in many different languages, not just the ones that are specific to .NET.
The advantage of Visual Studio Code over Rider is that it's lightning-fast. Because it's just an editor that lacks many tools that IDEs have, it has far fewer things to load and run in the background.
The disadvantage of using Visual Studio Code over Rider is that, as a code editor, it lacks some basic features that are embedded into an IDE. For example, you will not be able to compile your project without integrating the editor without some add-on tool or using the CLI.
Visual Studio Code can be obtained via https://code.visualstudio.com/download.
With this, our IDE setup is complete.
Downloading the .NET SDK (all operating systems)
Lastly, to write .NET applications, you will need to download the .NET platform.
There are two versions of it: runtime and SDK. As a developer, you will need the SDK. The runtime is only suitable for running .NET applications that have already been compiled; it cannot be used to write application code and compile applications.
The .NET SDK can be obtained via the following link. Please use the latest full release version. Further instructions are available at https://dotnet.microsoft.com/download/dotnet.
Now that your environment has been set up, you can start building an ASP.NET Core application with basic gRPC capabilities.