Create an object called
shape
that has atype
property and agetType()
method.Define a
Triangle()
constructor function whose prototype isshape
. Objects created with()
should have three own properties—a
,b
,c
representing the sides of a triangle.Add a new method to the prototype called
getPerimeter()
.Test your implementation with this code:
>>> var t = new Triangle(1, 2, 3); >>> t.constructor
Triangle(a, b, c)
>>> shape.isPrototypeOf(t)
true
>>> t.getPerimeter()
6
>>> t.getType()
"triangle"
Loop over
t
showing only own properties and methods (none of the prototype's).Make this code work:
>>> [1,2,3,4,5,6,7,8,9].shuffle()
[2, 4, 1, 8, 9, 6, 5, 3, 7]
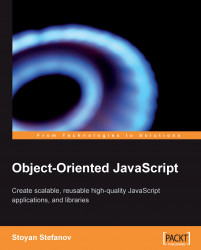
Object-Oriented JavaScript
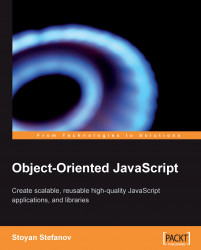
Object-Oriented JavaScript
Overview of this book
Table of Contents (18 chapters)
Object-Oriented JavaScript
Credits
About the Author
About the Reviewers
Preface
Introduction
Primitive Data Types, Arrays, Loops, and Conditions
Functions
Inheritance
The Browser Environment
Coding and Design Patterns
Reserved Words
Built-in Functions
Built-in Objects
Regular Expressions
Index
Customer Reviews