The code examples used in the recipes throughout this book are all based on the template we will create in this recipe. This template sets up the necessary Moodle environment and displays the standard Moodle header and footer.
This will allow us to look at the generic tools and techniques available to us within Moodle that pertain to JavaScript, allowing you, as the developer, to decide how and where to apply them.
In most instances, it is highly recommended to work with Moodle's rich plugin infrastructure to extend any functionality. This allows you to keep your enhancements completely separate from the core of Moodle, preventing you from introducing bugs into the core code, and making the Moodle upgrade process just a case of ensuring that your particular plugin folders are copied into the upgraded Moodle installation.
Please refer to the Moodle documentation at http://docs.moodle.org/ to decide which plugin framework suits your requirements.
There may be rare cases when it is simply not possible to implement the functionality you desire inside the plugin frameworks, requiring the modification of core code. If this is the case, it is likely that the functionality you desire will be useful to all Moodle users. Therefore, you should look to engage with the Moodle development community to seek advice regarding the feasibility of having your changes included in the next revision of the core code.
If, however, you know for a fact that you definitely need to make changes to core code and that those changes will only be useful to your bespoke use of Moodle, you may consider (as a last resort) modifying the core code files. Be aware that this method is not without issues, incurring significant disadvantages, such as:
Keeping track of each change you make to each file, and merging these changes as you upgrade to future versions of Moodle. Version control systems such as CVS or GIT may assist you here.
Your changes will not be subject to the same standard of testing and debugging as that of the official core code.
Your changes will not be guaranteed to continue to work in future versions; you will need to fully test your changes with each future upgrade.
So, as you can see, there is a trade-off in functionality versus maintainability for each method of extending and enhancing Moodle; so you should choose wisely. If in doubt, use a plugin or theme!
To begin with, we will create a subfolder named cook
within our Moodle installation where we will put all the files we will work with throughout the book.
Next we will create the template PHP file blank.php
, with the following content:
<?php require_once(dirname(__FILE__) . '/../config.php'); $PAGE->set_context(get_context_instance(CONTEXT_SYSTEM)); $PAGE->set_url('/cook/blank.php'); echo $OUTPUT->header(); echo $OUTPUT->footer(); ?>
Note
Downloading the example code
You can download the example code files for all Packt books you have purchased from your account at http://www.PacktPub.com. If you purchased this book elsewhere, you can visit http://www.PacktPub.com/support and register to have the files e-mailed directly to you.
We now have our template saved at /path/to/moodle/cook/blank.php
.
To test this new template, we can load it in a web browser and check if all is well. Assuming we have a web server running with Moodle served at the base URL http://localhost/moodle
, we can load the following URL to test our new template: http://localhost/moodle/cook/blank.php
.
If the template is set up correctly, we will see a basic page with standard header and footer, similar to the following:
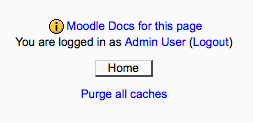
We begin by opening the PHP tags in the standard way, using <?php
.
Note that we have not used the shorthand notation of <?
, as this goes against the Moodle programming guidelines and also against general good practice.
Next, we must include Moodle's global configuration file. The inclusion of this file sets up the requisite Moodle programming environment, including two global variables we will make use of: $PAGE
and $OUTPUT
.
$PAGE
is defined as a central store of information about the current page we are generating in response to the user's request.
The $PAGE
object allows us to start defining the properties of the page, namely the context and the URL. The context of the page is the scope of the page, that is, where in the Moodle system it is being used. We are using the System context; other examples are in the Course or Module context.
Next, we set the URL of the page. This mirrors our directory structure; so in this case we just set it to /cook/blank.php
.
$OUTPUT
is defined as an instance of core_renderer
or one of its subclasses. Use it to generate HTML for output.
The $OUTPUT
object allows us to generate the header
and footer
for our page, based on the current Moodle theme. This is done by calling the header and footer methods of the $OUTPUT
object, and using echo to write them to the page.
After opening the PHP tag, we can begin inserting the PHP code. We use require_once
to include the Moodle configuration file (this ensures that the file is included only once, and will halt execution of the page if the file is unavailable). The path to the configuration file is determined by using the dirname
function to get the directory path to the current file (using the built-in __FILE__
constant), and then traversing up one directory (as we are in our cook
subdirectory) to find the config.php
file.
Next, we set the page context by calling the set_context
method of the $PAGE
object. This takes a context instance. We retrieve the instance for the system context by passing the CONTEXT_SYSTEM
constant to the get_context_instance
function.
The final usage of the $PAGE
object is to set the page URL, which is done using the set_url
method, passing in a web root relative URL path, which is /cook/blank.php
in this case.
Next, we need to use the $OUTPUT
object to generate the header and footer of the page. Both header and footer methods return HTML as a string, so we can use the echo
construct to write these strings to the page.