ScrolledText
widgets are much larger than simple Entry
widgets and span multiple lines. They are widgets like Notepad and wrap lines, automatically enabling vertical scrollbars when the text gets larger than the height of the ScrolledText
widget.
This recipe extends the previous recipe, Using radio button widgets. You can download the code for each chapter of this book from https://github.com/PacktPublishing/Python-GUI-Programming-Cookbook-Second-Edition/.
By adding the following lines of code, we create a ScrolledText
widget:
GUI_scrolledtext_widget.py
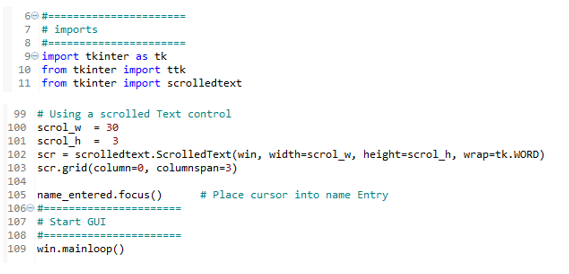
We can actually type into our widget, and if we type enough words, the lines will automatically wrap around:
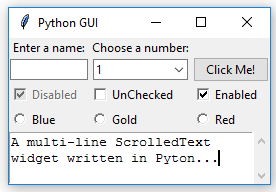
Once we type in more words than the height the widget can display, the vertical scrollbar becomes enabled. This all works out-of-the-box without us needing to write any more code to achieve this:
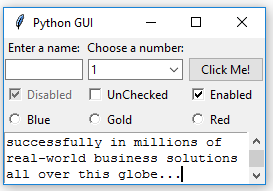
In line 11, we import the module that contains the ScrolledText
widget class. Add this to the top of the module, just below the other two import
statements.
Lines 100 and 101 define the width and height of the ScrolledText
widget we are about to create. These are hardcoded values we are passing into the ScrolledText
widget constructor in line 102.
These values are magic numbers found by experimentation to work well. You might experiment by changing scol_w
from 30 to 50 and observe the effect!
In line 102, we are also setting a property on the widget by passing in wrap=tk.WORD
.
By setting the wrap
property to tk.WORD
we are telling the ScrolledText
widget to break lines by words so that we do not wrap around within a word. The default option is tk.CHAR
, which wraps any character regardless of whether we are in the middle of a word.
The second screenshot shows that the vertical scrollbar moved down because we are reading a longer text that does not entirely fit into the x, y dimensions of the SrolledText
control we created.
Setting the columnspan
property of the grid widget to 3
for the SrolledText
widget makes this widget span all the three columns. If we do not set this property, our SrolledText
widget would only reside in column one, which is not what we want.