In this section, we're going to take a quick look at the Go programming language. Go is an expressive, concise, and clean language; it has concurrency mechanisms, and this helps programmers to write programs that get the most out of multi-core and networking machines. It also compiles quickly to machine code and has the convenience of garbage collection and the power of runtime reflection. It is a statically typed-in, compiled language, but, for most, it feels like a dynamically typed and interpreted language. All right then! Let's look at the syntax of Go by navigating to https://tour.golang.org/welcome/1; this is a good starting point for those who want to learn Go syntax:
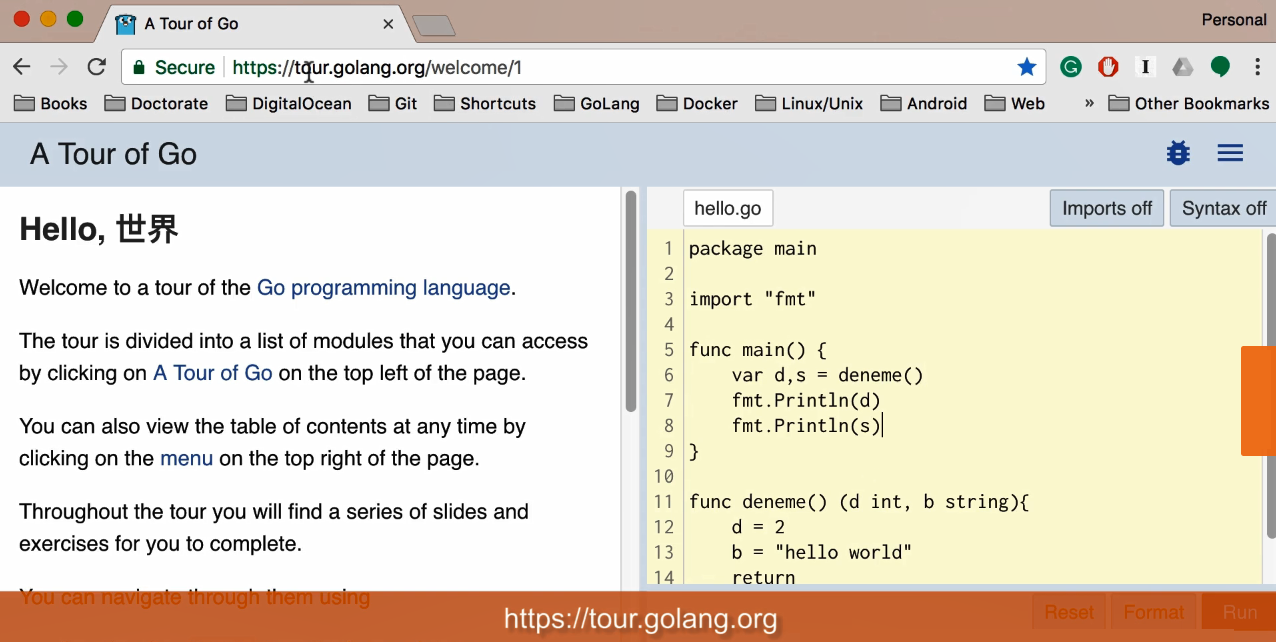
OK, So if you look at the syntax in the screenshot, and if you come from languages such as Java and C#, or C and C++, you may find the syntax a little bit different. For instance, if you look at the return type, instead of defining the type, you actually define the return types at the end of your function. We also have a main function, which is the entry point of our application, similar to many other programming languages, and if you look at the context shown in the following screenshot, you can see that we have packages, variables, and functions, and flow control statements: for
, if...else
, and types such as struct
, slices
, and maps
:
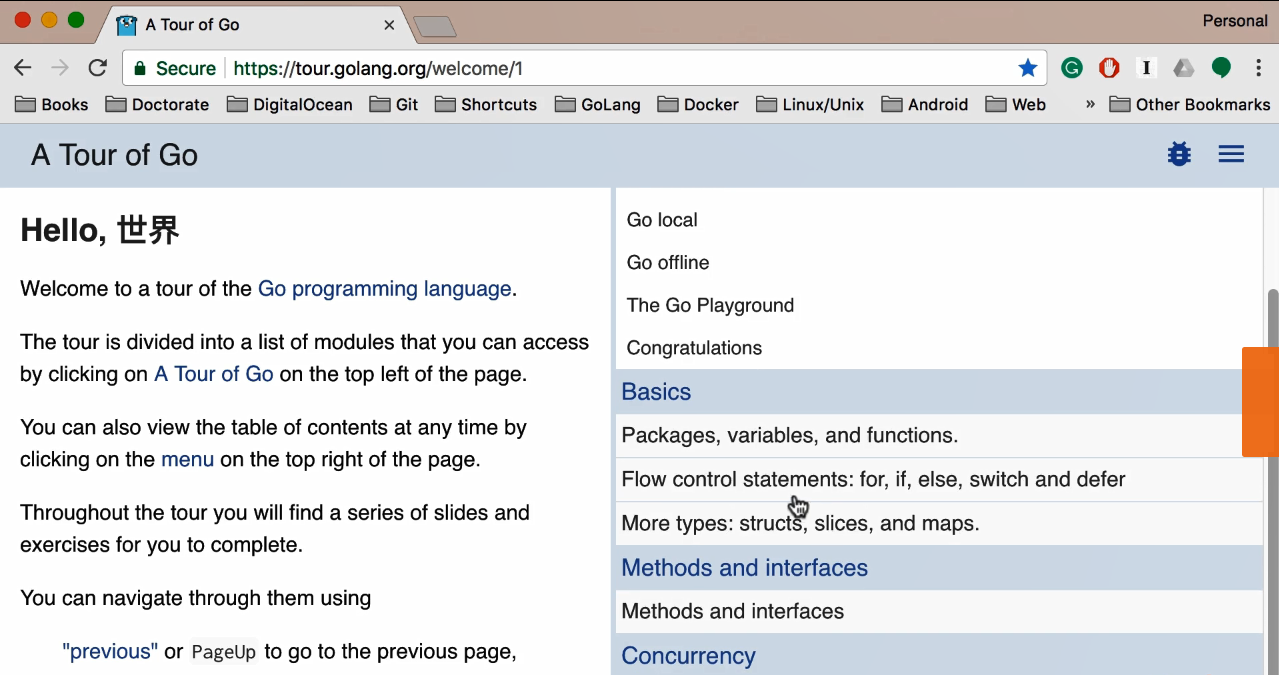
If you want to create a class, such as structure, you can use a struct type and combine it with a pointer. Additionally, it has methods and interfaces and concurrency, but it doesn't have generics.
Having said that, I will also talk about the tools that I'm going to be using throughout this book. There are a couple of tools available in GoLand. GoLand is a relatively new IDE by JetBrains. We will be using GoLand throughout the book. You can easily create new projects and give them a name and choose the SDK, which is Go 1.9. You can also add new files or new packages, and so on.
You can define your configurations, and build your Go, by typing your entry file, as shown in the following screenshot. You can then run main.go
and click OK:
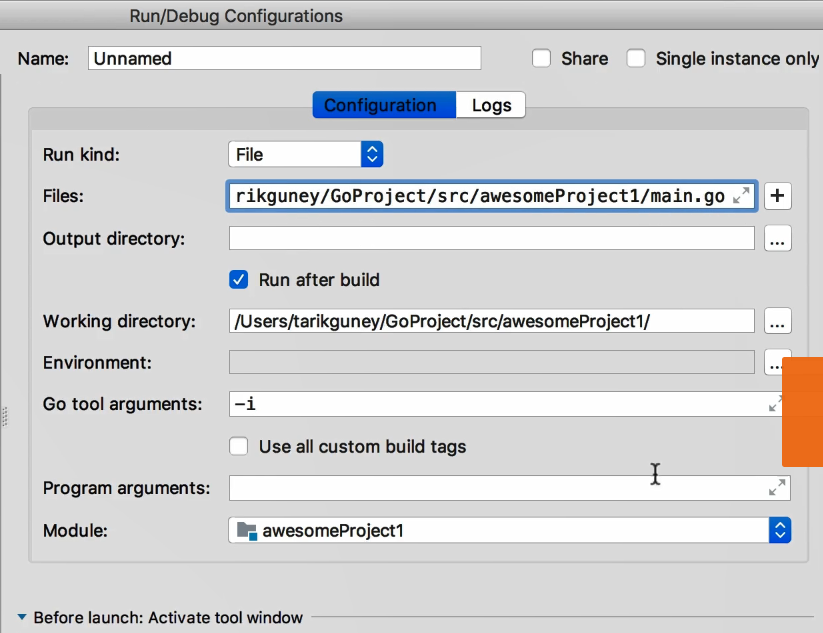
Finally, pressing Ctrl + r will build your project, as can be seen in the following screenshot:
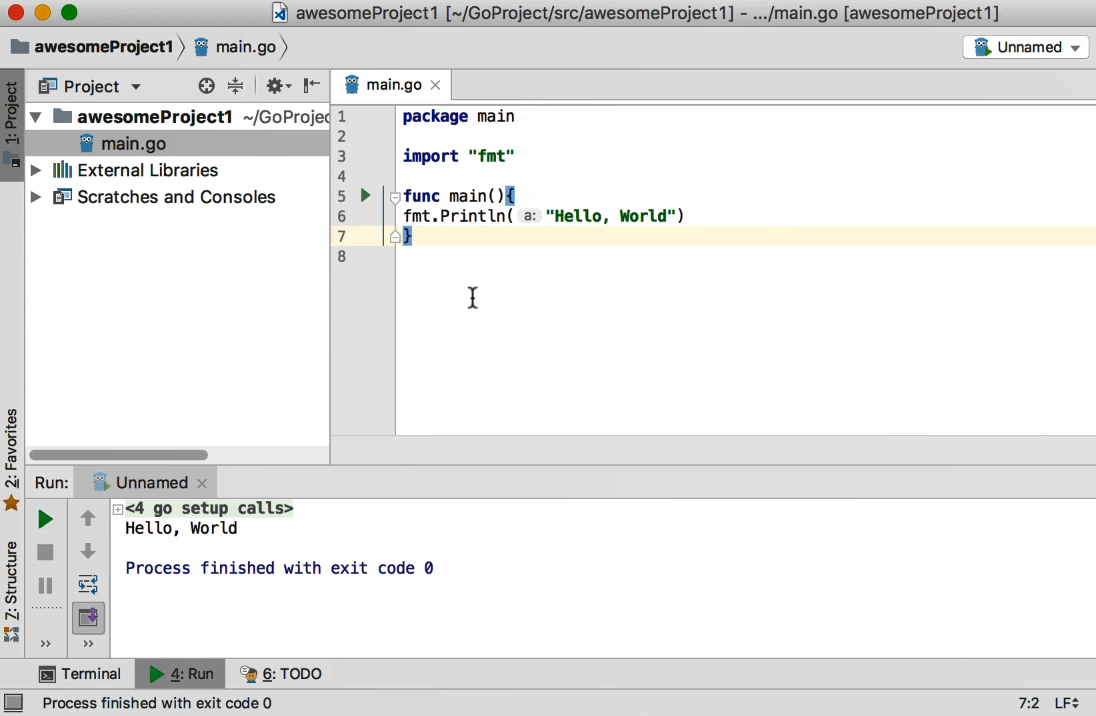
Before I conclude this chapter, let me quickly show you an example that uses just the terminal. I'm going to use the touch
command to create the main.go
file and add the following code:
package main import "fmt" func main(){ fmt.Println(a:"Hello World") }
You can run it by using the go run main.go
command and you will get the following output:
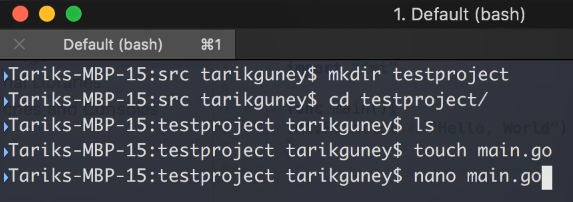
You can save it and then run it. So, this is how you can use the terminal to quickly write Go code and run it.