Programming can be thought of as code manipulating data. In Java, code is organized around the following:
Packages
Classes
Methods
Packages are collections of classes with similar functionality. Classes are composed of methods that support the functionality of the class. This organization provides structure to applications. Classes will always be in a package and methods will always be in a class.
Note
If the package statement is not included in a class definition, the class becomes part of a default package which consists of all of the classes in the same directory that doesn't have a package statement.
A class is the fundamental building block of object-oriented programs. It generally represents a real-world object. A class definition in Java consists of member variable declarations and method declarations. It begins with the class
keyword. The body of the class is enclosed with brackets and contains all instance variables and methods:
class classname { // define class level variables // define methods }
Note
A pair of open and close curly braces constitutes a block statement. This is used in many other parts of Java.
A class is a pattern or template for creating multiple objects with similar features. It defines the variables and methods of the class. It declares the capabilities of the class. However, before these capabilities can be used, an object must be created. An object is an instantiation of a class. That is, an object is composed of the memory allocated for the member variables of the class. Each object has its own set of member variables.
Note
The following occurs when a new object is created:
The new keyword is used to create an instance of a class
Memory is physically allocated for the new instance of the class
Any static initializers are executed (as detailed in the Java initialization sequence section in Chapter 6, Classes, Constructors, and Methods)
A constructor is called to do initialization
A reference to the object is returned
The state of an object is typically hidden from the users of the object and is reflected in the value of its instance variables. The behavior of an object is determined by the methods it possesses. This is an example of data encapsulation.
Note
An object is the instantiation of a class. Each instance of a class has its own unique set of instance variables.
Objects in Java are always allocated on the heap. The heap is an area of memory that is used for dynamically allocated memory, such as objects. In Java, objects are allocated in a program and then released by the JVM. This release of memory is called garbage collection and performed automatically by the JVM. An application has little control over this process. The primary benefit of this technique is the minimization of memory leaks.
Note
A memory leak occurs when memory is dynamically allocated but is never released. This has been a common problem with languages such as C and C++, where it is the responsibility of the programmer to manage the heap.
A memory leak can still occur in Java if an object is allocated but the reference to the object is never released when the object is no longer needed.
Constructors are used to initialize an object. Whenever an object is created, a constructor executes. A default constructor is the one that has no arguments and is provided automatically for all classes. This constructor will initialize all instance variables to default values.
However, if the developer provides a constructor, the compiler's default constructor is no longer added. The developer will need to explicitly add a default constructor. It is a good practice to always have a default, no-argument constructor.
An interface is similar to an abstract class. It is declared using the interface
keyword and consists of only abstract methods and final variables. An abstract class normally has one or more abstract methods. An abstract method is the one that does not have an implementation. It is intended to support polymorphic behavior, as discussed in Chapter 7, Inheritance and Polymorphism. The following code defines an interface used to designate a class as capable of being drawn:
interface Drawable { final int unit = 1; public void draw(); }
All executable code executes either within an initializer list or a method. Here, we will examine the definition and use of methods. The initializer list is discussed in Chapter 6, Classes, Constructors, and Methods. Methods will always be contained within a class. The visibility of a method is controlled by its access modifiers as detailed in the Access modifiers section. Methods may be either static or instance. Here, we will consider instance methods. As we will see in Chapter 6, Classes, Constructors, and Methods, static methods typically access static variables that are shared between objects of a class.
Regardless of the type of method, there is only a single copy of a method. That is, while a class may have zero, one, or more methods, each instance of the class (an object) uses the same definition of the method.
An option modifier
A return type
The method name
A parameter list enclosed in parentheses
An optional throws clause
A block statement containing the method's statements
The following setName
method illustrates these parts of a method:
public void setName(String name) throws Exception { if(name == null) { throw new Exception("Names must not be null"); } else { this.name = name; } }
While the else clause in this example is technically not required, it is a good practice to always use else clauses as it represents a possible execution sequence. In this example, if the if statement's logical expression evaluates to true, then the exception will be thrown and the rest of the method is skipped. Exception handling is covered in detail in Chapter 8, Handling Exceptions in an Application.
Methods frequently manipulate instance variables to define the new state of an object. In a well designed class, the instance variables can typically only be changed by the class' methods. They are private to the class. Thus, data encapsulation is achieved.
Methods are normally visible and allow the user of the object to manipulate that object. There are two ways to classify methods:
In the Customer
class, setter and getter methods were provided for all of the instance variables, except for the locale variable. We could have easily included a get and set method for this variable but did not, to conserve space.
Note
A variable that has a get method but not an otherwise visible set method is referred to as a read-only member variable . The designer of the class decided to restrict direct access to the variable.
A variable that has a set method but not an otherwise visible get method is referred to as a write-only member variable . While you may encounter such a variable, they are rare.
The signature of a method consists of:
The name of the method
The number of arguments
The types of the arguments
The order of the arguments
The signature is an important concept to remember and is used in overloading/overriding methods and constructors as discussed in Chapter 7, Inheritance and Polymorphism. A constructor will also have a signature. Notice that the definition of a signature does not include the return type.
The examples used in the book will be console program applications. These programs typically read from the keyboard and display the output on the console. When a console application is executed by the operating system, the main
method is executed first. It may then execute other methods.
The main
method can be used to pass information from the command line. This information is passed to the arguments of the main
method. It consists of an array of strings representing the program's parameters. We will see this in action in Chapter 4, Using Arrays and Collections.
There is only one form of the main
method in Java, shown as follows:
public static void main(String[] args) { // Body of method }
The following table shows elements of the main method:
Elements |
Meaning |
---|---|
| |
|
The method can be invoked without creating an object of the class type. |
| |
|
The main
method returns void
, meaning that it is not possible to return a value back to the operating system as part of the normal method invocation sequence. However, it is sometimes useful to return a value to indicate whether the program terminated successfully or not. This is useful when the program is used in a batch type operation where multiple programs are being executed. If one program fails in this execution sequence, then the sequence may be altered. Information can be returned from an application using the System.exit
method. The following use of the methods will terminate the application and return a zero to the operating system:
System.exit(0);
Variables and methods can be declared as one of four types, shown in the following table:
Access type |
Keyword |
Meaning |
---|---|---|
|
Access is provided to users outside the class. | |
|
Restricts access to members of the class. | |
|
Access is provided to classes that inherit the class or are members of the same package. | |
none |
Access is provided to members of the same package. |
Most of the time, a member variable is declared as private and a method is declared as public. However, the existence of the other access types implies other potential ways of controlling the visibility of a member. These usages will be examined in Chapter 7, Inheritance and Polymorphism.
In the Customer
class, all of the class variables were declared as private and all of the methods were made public. In the CustomerDriver
class, we saw the use of the setBalance
and toString
methods:
customer.setBalance(12506.45f); System.out.println(customer.toString());
As these methods were declared as public, they can be used with the Customer
object. It is not possible to directly access the balance instance variable. The following statement attempts this:
customer.balance = new BigDecimal(12506.45f);
The compiler will issue a compile-time error similar to the following:
balance has private access in com.company.customer.Customer
The documentation of a program is an important part of the software development process. It explains the code to other developers and provides reminders to the developers of what and why they did what they did.
Documentation is achieved through several techniques. Here, we will address three common techniques:
Comment: This is the documentation embedded in the application
Naming conventions: Following the standard Java naming conventions makes an application more readable
Javadoc: This is a tool used to generate documentation about an application in the form of HTML files
Comments are used to document a program. They are not executable and are ignored by a compiler. Good comments can go a long way to make a program more readable and maintainable. Comments can be grouped into three types—C style, C++ style, and Java style, as summarized in the following table:
Type of Comment |
Description | |
---|---|---|
Example | ||
C style |
The C style comment uses a two character sequence at the beginning and the end of a comment. This type of comment can extend across multiple lines. The beginning character sequence is a | |
/* A multi-line comment … */ /* A single line comment */
| ||
C++ style |
The C++ style comment begins with two forward slashes and the comment continues until the end of the line. Essentially everything from the | |
// The entire line is a comment int total; // Comment used to clarify variable area = height*width; // This computes the area of a rectangle
| ||
Java Style |
The Java style is identical in syntax to the C style comment, except that it starts with | |
/** * This method computes the area of a rectangle * * @param height The height of the rectangle * @param width The width of the rectangle * @return The method returns the area of a rectangle * */ public int computeArea(int height, int width) { return height * width; }
|
Java uses a series of naming conventions to make the programs more readable. It is recommended that you always follow this naming convention. By doing so:
You make your code more readable
It supports the use of JavaBeans
Note
More detail on naming conventions can be found at http://www.oracle.com/technetwork/java/codeconvtoc-136057.html.
The Java naming conventions' rules and examples are shown in the following table:
Element |
Convention |
Example |
---|---|---|
Package |
All letters in lowercase. |
|
Class |
First letter of each word is capitalized. |
|
Interface |
First letter of each word is capitalized. |
|
Variable |
First word is not capitalized but the subsequent words are capitalized |
|
Method |
First word is not capitalized but subsequent words are capitalized. Methods should be verbs. |
|
Constant |
Every letter is uppercase. |
|
The Javadoc tool produces a series of HTML files based on the source code and Javadoc tags embedded in the source code. This tool is also distributed with the JDK. While the following example is not an attempt to provide a complete treatment of Javadocs, it should give you a good idea of what Javadocs can do for you:
public class SuperMath { /** * Compute PI - Returns a value for PI. * Able to compute pi to an infinite number of decimal * places in a single machine cycle. * @return A double number representing PI */ public static double computePI() { // } }
The javadoc
command, when used with this class, results in the generation of several HTML files. A part of the index.html
file is shown in the following screenshot:
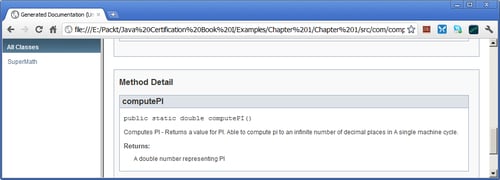
Note
More information on the use and creation of Javadoc files can be found at http://www.oracle.com/technetwork/java/javase/documentation/index-137868.html.