Java source code is compiled to intermediate bytecode. The bytecode is then interpreted at runtime on any platform that has a Java Virtual Machine (JVM). However, this statement is somewhat misleading as Java technology will often compile bytecode directly to machine code. There have been numerous Just-In-Time compiler improvements that speed up the execution of Java applications that often will run nearly as fast as, or sometimes even faster than, natively-compiled C or C++ applications.
Java source code is found in files that end with a .java
extension. The Java compiler will compile the source code to a bytecode representation and store these bytecodes in a file with a .class
extension.
There are several Integrated Development Environments (IDE) used to support the development of Java applications. A Java application can also be developed from the command line using basic tools from the Java Development Kit (JDK).
A production Java application is normally developed on one platform and then deployed to another. The target platform needs to have a Java Runtime Environment (JRE) installed on it in order for the Java application to execute. There are several tools that assist in this deployment process. Typically, a Java application is compressed in a Java Archive (JAR) file and then deployed. A JAR file is simply a ZIP file with a manifest document embedded within the JAR file. The manifest document often details the content and the type of JAR file being created.
The general steps used to develop a Java application include:
Create the application using an editor
Compile it using the Java compiler (
javac
)Execute it using the Java interpreter (
java
)Optionally debug the application as required using a Java debugger
This process is summarized in the following diagram:
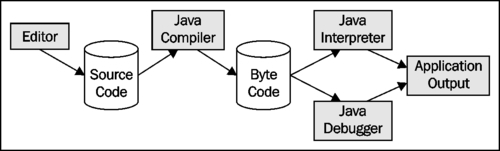
Java source code files are compiled to bytecode files. These bytecode files have a .class extension. When a Java package is distributed, the source code files are not normally stored in the same location as the .class
files.
The Java Software Development Kit (SDK) can be downloaded and used to create and execute many types of Java applications. The Java Enterprise Edition (JEE) is a different SDK and is used to develop enterprise applications typified by web-based applications. The SDK also known as the Java 2 Enterprise Edition (J2EE) and you may see it referenced as J2EE. Here, we will only deal with the Java SDK.
While the actual structure of the SDK distribution will vary by release, the typical SDK consists of a series of directories, listed as follows:
The SDK may include the actual source code for the core classes. This is usually found in the src.zip
file located under the JAVA_HOME
root directory.
Each IDE has a preferred way of organizing the files that make up an application. These organization schemes are not always hard and fast but those presented here are the common ways of arranging the files.
For example, a simple application in the Eclipse IDE consists of two project files and three sub-directories. These files and directories are listed as follows:
.classpath
: This is an XML file containing the classpath-related information.settings
: This is a directory containing theorg.eclipse.jdt.core.prefs
file which specifies compiler preferencesbin
: This is used to contain the package file structure and the application's class filessrc
: This is used to contain the package file structure and the application's source files
This organization scheme is used by the development tools. The tools often include editors, compilers, linkers, debuggers, and others. These languages frequently use a Make tool to determine which files need to be compiled or otherwise manipulated.
In this section we will demonstrate how to compile and execute a Java application on a Windows platform using Java 7. The approach is very similar to that used for other operating systems.
Before we can compile and execute the sample program we need to:
Install the JDK
Create the appropriate file structure for the application
Create the files to hold our classes
The latest version of the JDK can be found at http://www.oracle.com/technetwork/java/javase/downloads/index.html. Download and install the version that meets your needs. Note the location of the installation, as we will use this information shortly.
As explained earlier, the Java classes must be located in a specific file structure as mirrored in its package name. Create a file structure somewhere in your filesystem that has a top-level directory called com
under which is a directory called company
and then under the company
directory, a directory called customer
.
In the customer
directory create two files called Customer.java
and CustomerDriver.java
. Use the corresponding classes as found earlier in the A simple Java application section.
The JDK tools are found in the JDK directory. When the JDK is installed, the environmental variables are normally set up to allow the successful execution of the JDK tools. However, it is necessary to specify the location of these tools. This is accomplished using the set
command. In the following command, we set the path environmental variable to reference C:\Program Files\Java\jdk1.7.0_02\bin directory
, which was the most recent release at the time this chapter was written:
set path= C:\Program Files\Java\jdk1.7.0_02\bin;%path%
This command prefixes the path to the bin
directory in front of any previously assigned paths. The path
environmental variable is used by the operating system to look for the command that is executed at the command prompt. Without this information, the operating system is not aware of the location of the JDK commands.
To compile
the program using the JDK, navigate to the directory above the com
directory. As the classes used as part of this application are part of the com.company.customer
package we need to:
Specify the path in the
javac
commandExecute the command from the directory above the
com
directory
As there are two files that make up this application we need to compile both of them. This can be done using two separate commands as follows:
javac com.company.customer.Customer.java javac com.company.customer.CustomerDriver.java
Or, it can be done using a single command and the asterisk wild card character as follows:
javac com.company.customer.*.java
The output of the compiler is a bytecode file with the name CustomerDriver.class
. To execute the program, invoke the Java interpreter with your class file, as shown in the following command. The class extension is not included and its inclusion as part of the filename will result in an error:
java com.company.customer.CustomerDriver
The output of your program should be as follows:
Name: Default Customer Account number: 12345 Balance: € 12.506,45
The Java environment is the operating system and file structure used to develop and execute Java applications. Earlier, we examined the structure of the JDK which are part of the Java environment. Associated with this environment are a series of environmental variables that are used from time-to-time to facilitate various operations. Here, we will examine a few of them in more detail:
CLASSPATH
PATH
JAVA_VERSION
JAVA_HOME
OS_NAME
OS_VERSION
OS_ARCH
These variables are summarized in the following table:
Name |
Purpose |
Example |
---|---|---|
|
Specifies the root directory for classes. |
|
The location of the commands. | ||
The version of Java to use. |
| |
The location of the Java directory. |
| |
The name of the operating system. |
Windows 7 | |
The version of the operating system |
6.1 | |
The operating system architecture |
AMD64 |
The CLASSPATH
environmental variable is used to identify the root directory of the packages. It is set as follows:
c:>set CLASSPATH=d:\development\increment1;%CLASSPATH%
The CLASSPATH
variable only needs to be set for non-standard packages. The Java compiler will always implicitly append the system's class directories to CLASSPATH
. The default CLASSPATH
is the current directory and the system's class directories.
There are many other environmental variables associated with an application. The following code sequence can be used to display a list of these variables:
java.util.Properties properties = System.getProperties(); properties.list(System.out);
A partial display of the output of this code sequence is as follows:
-- listing properties -- java.runtime.name=Java(TM) SE Runtime Environment sun.boot.library.path=C:\Program Files\Java\jre7\bin java.vm.version=22.0-b10 java.vm.vendor=Oracle Corporation java.vendor.url=http://java.oracle.com/ path.separator=; java.vm.name=Java HotSpot(TM) 64-Bit Server VM …
Annotations provide information about a program. This information does not reside in the program and does not affect its execution. Annotations are used to support tools such as the compiler and during the execution of the program. For example, the @Override
annotation informs the compiler that a method is overriding a base class method. If the method does not actually override the base class method because it is misspelled, the compiler will generate an error.
Annotations are applied to elements of the application such as a class, method, or field. It begins with the at sign, @
, is followed by the name of the annotation, and optionally a list of values enclosed in a set of parentheses.
Common compiler annotations are detailed in the following table:
Annotation |
Usage |
---|---|
Used by the compiler to indicate that the element should not be used | |
|
The method overrides the base class method |
Used to suppress specific compiler warnings |
Annotations can be added to an application and used by the third-party tools for specific purposes. It is also possible to write your own annotations when needed.
Java includes a number of libraries of classes that support the development of applications. These include the following, among others:
java.lang
java.io
java.net
java.util
java.awt
These libraries are organized in packages. Each package holds a set of classes. The structure of a package is reflected in its underlying file system. The CLASSPATH
environmental variable holds the location of packages.
There are a core set of packages that are part of the JDK. These packages provide a crucial element in the success of Java by providing easy access to a standard set of capabilities that were otherwise not readily available with other languages.
The following table shows a list of some of the more commonly used packages: