An array is every developer's best friend and saves the collection of data in an ordered list. In Swift, an array is very easy to use and contains many helpful methods for use. Before exploring it, we have to clarify some important points.
Array by default is mutable so that it accepts adding, changing, or removing items from it, except if we define it as a constant, using let
. In this case, it will be immutable, as it becomes constant.
In Objective-C, you can save any type of object, and you won't have to specify any information about their type. In Swift, arrays are typed; this means that the type of item should be clear, and all the items should be of the same type. The type can be defined explicitly, or it can be inferred.
An array doesn't have to be of a class type. So, you can create an array of Int
, and in such a case, you can't insert any other value than the Int
type.
The following are examples to make these points clear:
let languages = ["Arabic", "English", "French"] //Here type is inferred as String, So this Array is of type String //Also this array is immutable as it defined as let var primes :[Int] = [2, 3, 5, 7, 11] //Type is written explicitly primes.append(13) //mutable array as it defined as var
To initialize and create an array, you can use the previous ways with initial values, or you can either create it empty, or by using repeating values:
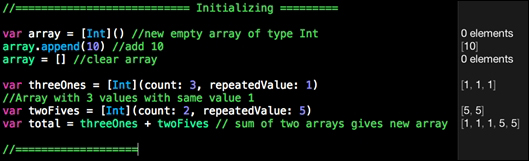
As you see in the example, it's very easy to initialize empty arrays, or arrays with repeating values. Swift also provides a great feature to append two arrays that result in a new array.
The explanation is not complete without mentioning how to iterate over an array. Actually, iterating over an array in Swift is very easy and straightforward. To iterate over values of an array, you will use the for-in
loop like this:
var seasons = ["Winter", "Spring", "Summer", "Autumn"] for season in seasons{ println(season) } /* Winter Spring Summer Autumn */
And if you want the index of the value in each iteration, you can use the enumerate
method. In each iteration, the method returns a tuple that is composed of an index and value of each item in the array. Check the next example to make things clear:
for (index, season) in enumerate(seasons){ println("Season #\(index + 1) is \(season)") } /* Season #1 is Winter Season #2 is Spring Season #3 is Summer Season #4 is Autumn */
For all those who don't know tuples, tuples in Swift are used to create and pass around a group of values. It can be used to return multiple values from a function in a single group.
You can append items easily by using the append
method, which appends an item at the end of an array. To append multiple items at once, append an array of these items.
You can also use the insert
method that inserts items at a specific location.
var nums = [1, 3] // [1, 3] nums.append(4) // [1, 3, 4] nums.insert(5, atIndex: 1) // [1, 5, 3, 4] nums += [10, 11] // [1, 5, 3, 4, 10, 11]
An array has a built-in removeAtIndex
function to remove an item at the index and a removeLast
function to remove the last item. These functions are awesome. While you call them, they return the deleted item at the same time and thus, you don't have to write another line of code to grab the item before deleting it.
nums.removeAtIndex(1) // return 5 nums.removeLast() // return 11 nums // [1, 3, 4, 10] nums[0...2] = [] // array now is [10]
In this code we removed an item at index 1
and the last item, as you see in the first two lines.
Another great feature in Swift is using ranges, which we used for replacing the items in the range from 0-2. This replaces the first three items with an empty array. That means the first three items have been removed. You can also replace it with an array containing data. Now, replace the items in the range with the items in the array. The most important thing is to be careful with the ranges that are used, and make sure that they are an inbound of the array. The out of bound ranges will throw exceptions.