The index of a DataFrame provides a label for each of the rows. If no index is explicitly provided upon DataFrame creation, then by default, a RangeIndex is created with labels as integers from 0 to n-1, where n is the number of rows.
Making the index meaningful
Getting ready
This recipe replaces the meaningless default row index of the movie dataset with the movie title, which is much more meaningful.
How to do it...
- Read in the movie dataset, and use the set_index method to set the title of each movie as the new index:
>>> movie = pd.read_csv('data/movie.csv')
>>> movie2 = movie.set_index('movie_title')
>>> movie2
- Alternatively, it is possible to choose a column as the index upon initial read with the index_col parameter of the read_csv function:
>>> movie = pd.read_csv('data/movie.csv', index_col='movie_title')
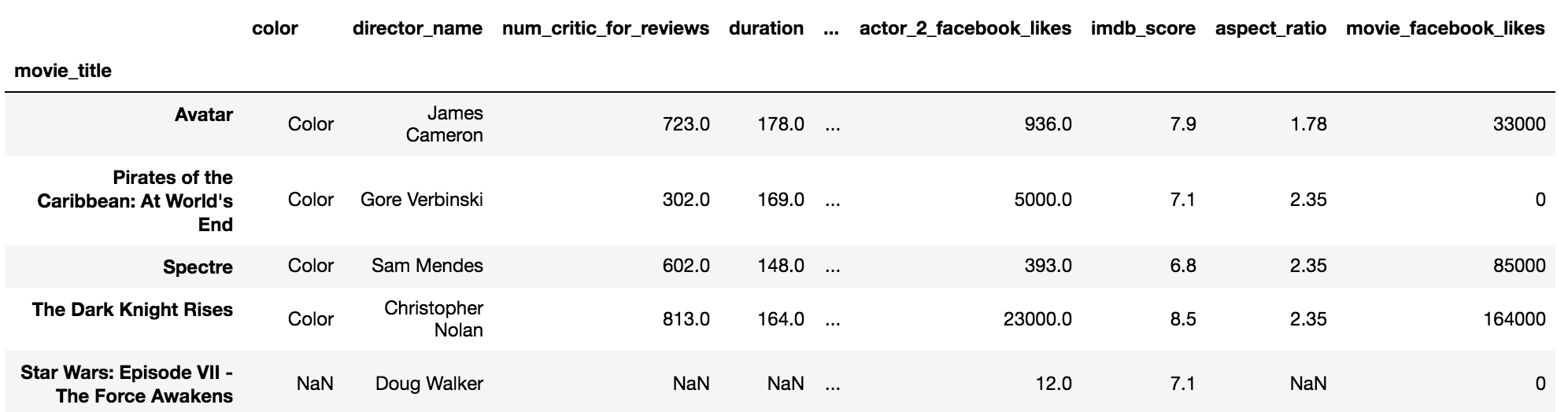
How it works...
A meaningful index is one that clearly identifies each row. The default RangeIndex is not very helpful. Since each row identifies data for exactly one movie, it makes sense to use the movie title as the label. If you know ahead of time which column will make a good index, you can specify this upon import with the index_col parameter of the read_csv function.
By default, both set_index and read_csv drop the column used as the index from the DataFrame. With set_index, it is possible to keep the column in the DataFrame by setting the drop parameter to False.
There's more...
Conversely, it is possible to turn the index into a column with the reset_index method. This will make movie_title a column again and revert the index back to a RangeIndex. reset_index always puts the column as the very first one in the DataFrame, so the columns may not be in their original order:
>>> movie2.reset_index()
See also
- Pandas official documentation on RangeIndex (http://bit.ly/2hs6DNL)