Q1) What about a visual improvement for our game screen, perhaps a nice, light green grassy background instead of just black?
A) You can use most graphics programs such as Gimp or Photoshop to get the RGB value of a nice, light green grassy color. Alternatively, you can use an online color picker such as http://www.colorpicker.com/. Then look at this line in our drawGame
method:
canvas.drawColor(Color.BLACK);//the background
Change it to the following line:
canvas.drawColor(Color.argb(255,186,230,177));//the background
Q2) How about adding some nice flowers to the background?
A) Here is the way to do this. Create a flower bitmap (or use mine), load it, and scale it in the usual way, in the configureDisplay
method. Decide how many flowers to draw. Choose and store locations on the board in the SnakeView
constructor (or write and call a special method, perhaps plantFlowers
).
Draw them before the snake and the apple in the drawGame
method. This will ensure that they can never hide an apple or a part of the snake. You can see my specific implementation in the methods mentioned and a copy of the flower bitmap in the EnhancedSnakeGame
project in the Chapter8
folder.
Q3) If you're feeling brave, make the flowers sway. Think of sprite sheets. The theory is exactly the same as that of the animated snake head. We just need a few lines of code to control the frame rate separately from the game frame rate.
A) Take a look at the new code in the controlFPS
method. We simply set up a new counter for flower animations to switch flower frames once every six game frames. You can also copy the sprite sheet from the EnhancedSnakeGame
project in the Chapter8
folder.
Q4) We could set up another counter and use our snake head animation, but it wouldn't be that useful because the subtle tongue movements would be barely visible due to the smaller size. Nevertheless, we could quite easily swish the tail segment.
A) There is a two-frame tail bitmap in the EnhancedSnakeGame
project in the Chapter8
folder. As this is also two frames, we could use the same frame timer as that used for the flower. Take a look at the implementation in the EnhancedSnakeGame
project in the Chapter8
folder. The only required changes are in configureDisplay
and drawGame
.
Q5) Here is a slightly trickier enhancement. You can't help notice that when the snake sprites are headed in three out of the four possible directions, they don't look right. Can you fix this?
A) We need to rotate them depending upon the way they are heading. Android has a Matrix
class, which allows us to easily rotate Bitmaps, and the Bitmap
class has an overloaded version of the createBitmap
method that takes a Matrix
object as an argument.
So we can create a matrix for each angle we need to handle, like this:
Matrix matrix90 = new Matrix(); matrix90.postRotate(90);
Then we can rotate a bitmap using the following code:
rotatedBitmap = Bitmap.createBitmap(regularBitmap , 0, 0, regularBitmap .getWidth(), regularBitmap .getHeight(), matrix90, true);
Another problem is that as the snake twists and turns, how do we keep track of the individual orientation of each segment? We already have a direction finding scheme: 0 is up, 1 is right, and so on. So we can just create another array for the orientation of each segment that corresponds to a body segment in the snakeX
and snakeY
arrays. Then all we need to do is to ensure that the head has the correct direction, and update from the back on each frame just as we do for the snake's coordinates. You can see this implemented in the EnhancedSnakeGame
project in the Chapter8
folder.
The finished project with a few more enhancements is in the EnhancedSnakeGame
project in the Chapter8
folder. This is the version we will be using as a starting point in the next and final chapter. You can also download the game from Google Play at https://play.google.com/store/apps/details?id=com.packtpub.enhancedsnakegame.enhancedsnakegame.
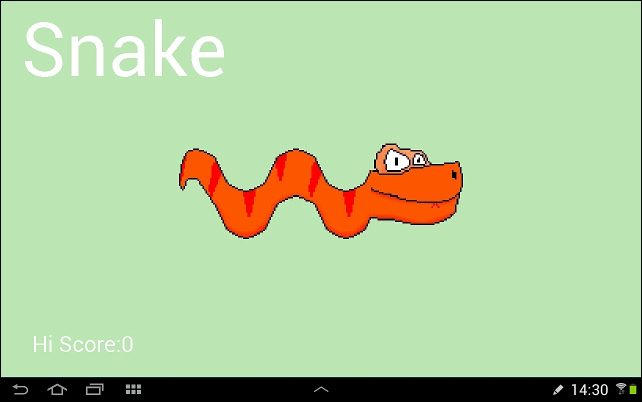
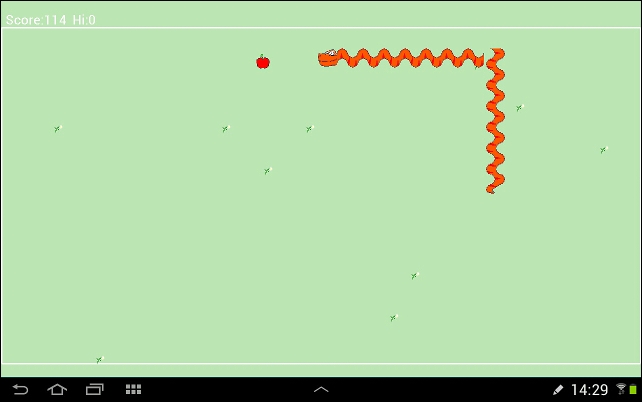