To get a playable game you just need to wait a second after the player picked the second tile before removing/covering them.
This can be done by adding a timer in the game. Timer
class included in flash.utils
package will let us use timers, and TimerEvent
class included in flash.events
handles timer events.
Let's start importing the classes and declaring a new variable. Change your script until Main
function looks like this:
package { // importing classes import flash.display.Sprite; import flash.events.MouseEvent; import flash.events.TimerEvent; import flash.utils.Timer; // end of importing classes public class Main extends Sprite { private var pickedTiles:Array = new Array(); private const NUMBER_OF_TILES:uint=20; private var pauseGame:Timer; public function Main() { ... } } }
We just imported the two time-related classes in our package and created a new Timer
variable called pauseGame
. It will come into play when the player selects the second tile, so modify the block that checks if we picked two tiles this way:
// checking if we picked 2 tiles if (pickedTiles.length==2) { pauseGame=new Timer(1000,1); pauseGame.start(); if (pickedTiles[0].cardType==pickedTiles[1].cardType) { // tiles match!! trace("tiles match!!!!"); pauseGame.addEventListener(TimerEvent.TIMER_COMPLETE,removeTiles); } else { // tiles do not match trace("tiles do not match"); pauseGame.addEventListener(TimerEvent.TIMER_COMPLETE,resetTiles); } // no more pickedTiles = new Array(); } // end checking if we picked 2 tiles
Once we know the player just picked the second tile, it's time to wait for one second.
pauseGame=new Timer(1000,1);
Let's initialize the timer with the constructor, which is the function that generates it. The first parameter defines the delay between timer events, in milliseconds, while the second one specifies the number of repetitions. In this case, pauseGame
will wait for 1 second only once.
Again, you can use a constant, to store the number of milliseconds. I am not using it because it should be clear how to use variables and constants and I want to focus on new features.
pauseGame.start();
To make the timer start, use start()
method.
When the timer reaches 1 second, it will dispatch a TimerEvent.TIMER_COMPLETE
event. So we have to make pauseGame
listen for such an event.
pauseGame.addEventListener(TimerEvent.TIMER_COMPLETE,removeTiles);
and
pauseGame.addEventListener(TimerEvent.TIMER_COMPLETE,resetTiles);
Will make the program wait for the Timer
object to complete its delay (one second) and then call removeTiles
or resetTiles
function.
These functions will just handle the removing and the resetting of tiles in the same way we did before. Add the functions inside Main
class but outside Main
function, just as you did with onTileClicked
function:
private function removeTiles(e:TimerEvent) { pauseGame.removeEventListener(TimerEvent.TIMER_COMPLETE,removeTiles); pickedTiles[0].removeEventListener(MouseEvent.CLICK,onTileClicked); pickedTiles[1].removeEventListener(MouseEvent.CLICK,onTileClicked); removeChild(pickedTiles[0]); removeChild(pickedTiles[1]); pickedTiles = new Array(); }
As you can see the function just removes the listeners and the tiles, just as before.
private function resetTiles(e:TimerEvent) { pauseGame.removeEventListener(TimerEvent.TIMER_COMPLETE,resetTiles); pickedTiles[0].gotoAndStop(NUMBER_OF_TILES/2+1); pickedTiles[1].gotoAndStop(NUMBER_OF_TILES/2+1); pickedTiles = new Array(); }
and this one just covers the tiles again.
Notice how both functions remove TimerEvent
listener and clear pickedTiles
array by initializing it again. Also, such array is no longer cleared in the block where we checked if we picked 2 tiles block. Why not? Because it would clear the picked tiles array before the script knows which tiles to remove/cover, as it happens after a second.
Run the program: it works! You can see the second tile for 1 second before the script decides what to do. Your Concentration game is finished!
No, it's not.
Try to quickly pick three or four tiles. You can, because nobody told the script to ignore clicks when it's waiting the second necessary to show you the tile you just picked. So you can quickly take a look at more than two cards during a single turn. That's cheating.
We can see more than two tiles if we quickly select a bunch of them.
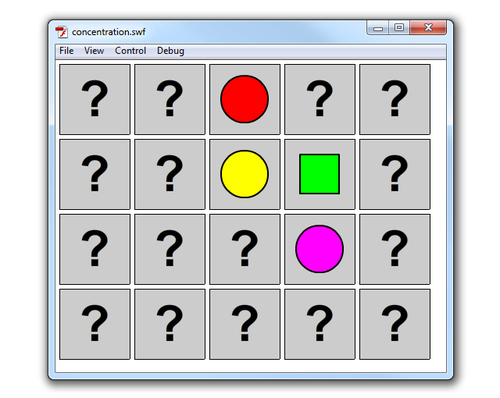
Believe it or not, although the game is not finished yet, you have learned everything you need to create a basic Concentration prototype. You just saw how to:
Execute different blocks of code according to a specific condition
Remove DisplayObject from the stage
Use timers to make the game wait
Let's make life impossible for those die hard cheaters!