Prerequisites for starting with ROS
Before getting started with ROS and trying the code in this book, the following prerequisites should be met:
- Ubuntu 20.04 LTS/Debian 10: ROS is officially supported by the Ubuntu and Debian operating systems. We prefer to stick with the LTS version of Ubuntu; that is, Ubuntu 20.04.
- ROS noetic desktop full installation: Install the full desktop installation of ROS. The version we prefer is ROS Noetic, which is the latest stable version. The following link provides the installation instructions for the latest ROS distribution: http://wiki.ros.org/noetic/Installation/Ubuntu. Choose the
ros-noetic-desktop-full
package from the repository list.
Let's see the different versions of ROS framework.
ROS distributions
ROS updates are released with new ROS distributions. A new distribution of ROS is composed of an updated version of its core software and a set of new/updated ROS packages. ROS follows the same release cycle as the Ubuntu Linux distribution: a new version of ROS is released every 6 months. Typically, for each Ubuntu LTS version, an LTS version of ROS is released. Long Term Support (LTS) and means that the released software will be maintained for a long time (5 years in the case of ROS and Ubuntu):
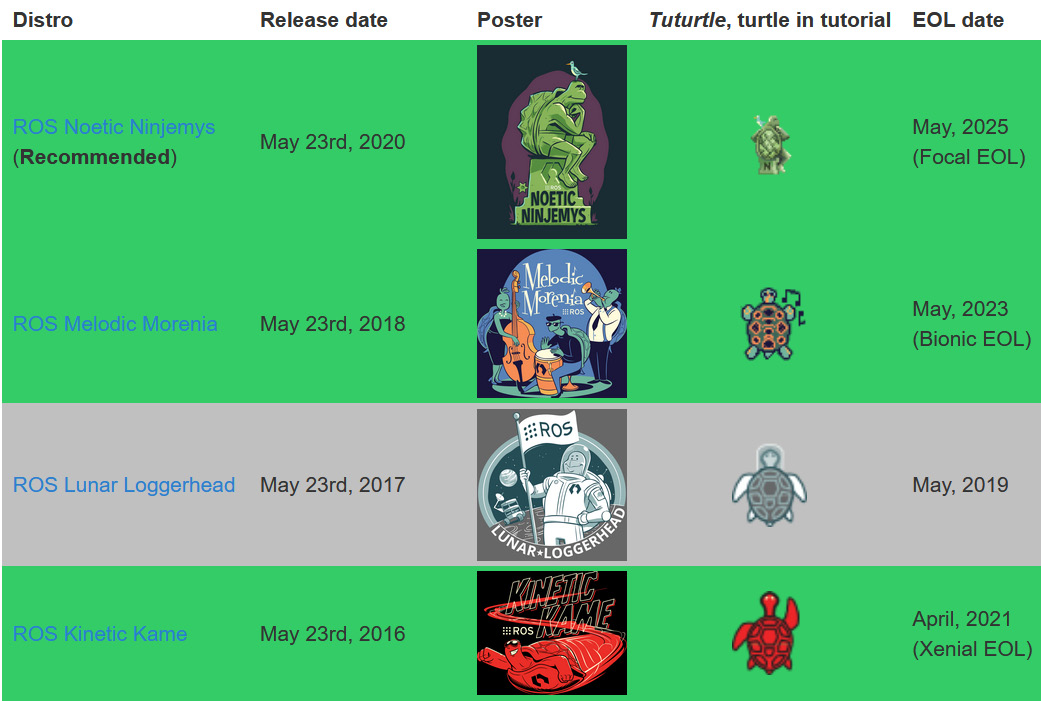
Figure 1.9 – List of recent ROS releases
The tutorials in this book are based on the latest LTS version of ROS, known as ROS Noetic Ninjemys. It represents the thirteenth ROS distribution release. The list of recent ROS distributions is shown in the preceding image.
Running the ROS master and the ROS parameter server
Before running any ROS nodes, we should start the ROS master and the ROS parameter server. We can start the ROS master and the ROS parameter server by using a single command called roscore
, which will start the following programs:
- ROS master
- ROS parameter server
rosout
logging nodes
The rosout
node will collect log messages from other ROS nodes and store them in a log file, and will also re-broadcast the collected log message to another topic. The /rosout
topic is published by ROS nodes using ROS client libraries such as roscpp
and rospy
, and this topic is subscribed by the rosout
node, which rebroadcasts the message in another topic called /rosout_agg
. This topic contains an aggregate stream of log messages. The roscore
command should be run as a prerequisite to running any ROS nodes. The following screenshot shows the messages that are printed when we run the roscore
command in a Terminal.
Use the following command to run roscore
on a Linux Terminal:
roscore
After running this command, we will see the following text in the Linux Terminal:
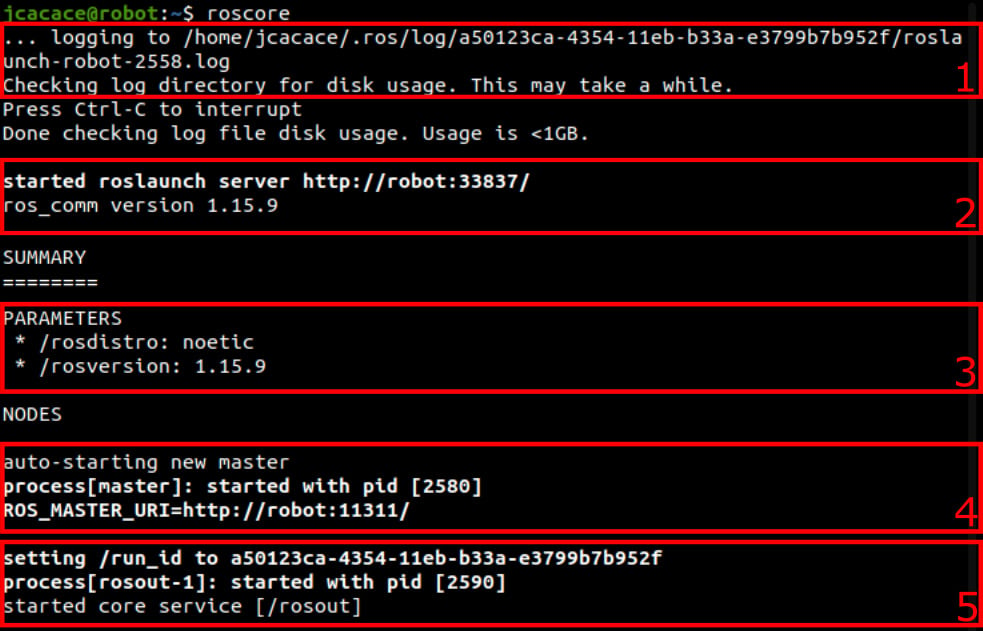
Figure 1.10 – Terminal messages while running the roscore
command
The following are explanations of each section when executing roscore
in a Terminal:
- In section 1, we can see that a log file is created inside the
~/.ros/log
folder for collecting logs from ROS nodes. This file can be used for debugging purposes. - In section 2, the command starts a ROS launch file called
roscore.xml
. When a launch file starts, it automatically startsrosmaster
and the ROS parameter server. Theroslaunch
command is a Python script, which can startrosmaster
and the ROS parameter server whenever it tries to execute a launch file. This section shows the address of the ROS parameter server within the port. - In section 3, we can see parameters such as
rosdistro
androsversion
being displayed in the Terminal. These parameters are displayed when it executesroscore.xml
. We will look atroscore.xml
in more detail in the next section. - In section 4, we can see that the
rosmaster
node is being started withROS_MASTER_URI
, which we defined earlier as an environment variable. - In section 5, we can see that the
rosout
node is being started, which will start subscribing to the/rosout
topic and rebroadcasting it to/rosout_agg
.
The following is the content of roscore.xml
:
<launch> <group ns="/"> <param name="rosversion" command="rosversion roslaunch" /> <param name="rosdistro" command="rosversion -d" /> <node pkg="rosout" type="rosout" name="rosout" respawn="true"/> </group> </launch>
When the roscore
command is executed, initially, the command checks the command-line argument for a new port number for rosmaster
. If it gets the port number, it will start listening to the new port number; otherwise, it will use the default port. This port number and the roscore.xml
launch file will be passed to the roslaunch
system. The roslaunch
system is implemented in a Python module; it will parse the port number and launch the roscore.xml
file.
In the roscore.xml
file, we can see that the ROS parameters and nodes are encapsulated in a group XML tag with a /
namespace. The group XML tag indicates that all the nodes inside this tag have the same settings.
The rosversion
and rosdistro
parameters store the output of the rosversionroslaunch
and rosversion-d
commands using the command
tag, which is a part of the ROS param
tag. The command
tag will execute the command mentioned in it and store the output of the command in these two parameters.
rosmaster
and the parameter server are executed inside roslaunch
modules via the ROS_MASTER_URI
address. This happens inside the roslaunch
Python module. ROS_MASTER_URI
is a combination of the IP address and port that rosmaster
is going to listen to. The port number can be changed according to the given port number in the roscore
command.
Checking the roscore command's output
Let's check out the ROS topics and ROS parameters that are created after running roscore
. The following command will list the active topics in the Terminal:
rostopic list
The list of topics is as follows, as per our discussion of the rosout
node's subscribe /rosout
topic. This contains all the log messages from the ROS nodes. /rosout_agg
will rebroadcast the log messages:
/rosout /rosout_agg
The following command lists the parameters that are available when running roscore
. The following command is used to list the active ROS parameter:
rosparam list
These parameters are mentioned here; they provide the ROS distribution name, version, the address of the roslaunch
server, and run_id
, where run_id
is a unique ID associated with a particular run of roscore
:
/rosdistro /roslaunch/uris/host_robot_virtualbox__51189 /rosversion /run_id
The list of ROS services that's generated when running roscore
can be checked by using the following command:
rosservice list
The list of services that are running is as follows:
/rosout/get_loggers /rosout/set_logger_level
These ROS services are generated for each ROS node, and they are used to set the logging levels.