Understanding .NET Core
In order to understand the origins of, and motivation for, .NET Core, let's start with a quote:
.NET Core dates back as early as 2001 when Shared Source Common Language Infrastructure (SSCLI) was shared sourced (not for commercial use) under the code name Rotor. This was the ECMA 335, that is, the Common Language Infrastructure (CLI) standard implementation. Rotor could be built on FreeBSD (version 4.7 or newer) and macOS X 10.2. It was designed in such a way that a thin Platform Abstraction Layer (PAL) was the only thing that was needed to port the CLI to a different platform. This release constitutes the initial steps to migrate .NET to a cross-platform infrastructure.
2001 was also the year the Mono project was born as an open source project that ports parts of .NET to the Linux platform as a development platform infrastructure. In 2004, the initial version of Mono was released for Linux, which would lead to ports on other platforms such as iOS (MonoTouch) and Android (MonoDroid), and would eventually be merged into the .NET ecosystem under the Xamarin name.
One of the driving forces behind this approach was the fact that the .NET framework was designed and distributed as a system-wide monolithic framework. Applications that are dependent on only a small portion of the framework required the complete framework to be installed on the target operating system. It did not support application-only scenarios where different applications can be run on different versions without having to install a system-wide upgrade. However, more importantly, applications that were developed with .NET were implicitly bound to Windows because of the tight coupling between the .NET Framework and Windows API components. .NET Core was born out of these incentives and opened up the doors of various platforms for .NET developers.
Finally, in 2020, .NET Core replaced classic .NET. The unified .NET platform now provides developers with a single runtime and framework that can be used to create cross-platform applications using a single code base.
Semantically speaking, .NET now describes the complete infrastructure for the whole set of cross-development tools that rely on a common language infrastructure and multiple runtimes, including .NET Core Runtime, .NET, also known as Big CLR, the Mono runtime, and Xamarin:
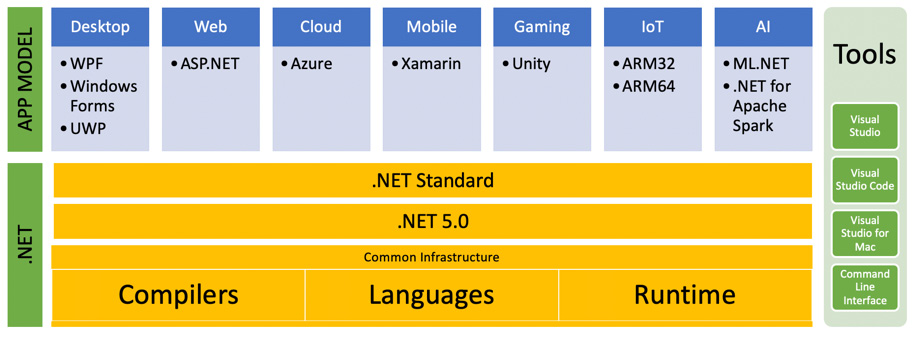
Figure 1.1 – .NET Ecosystem
In this setup, the .NET CLI (Common Language Infrastructure) is made up of the base class library implementation, which defines the standards that need to be provided by the supported runtimes. The base class library is responsible for providing the PAL, which is provided by the hosting runtime under the name of the Adaption Layer. This infrastructure is supported by compiler services such as Roslyn and Mono Compiler (MCS), as well as Just-In-Time (JIT) and Ahead-of-Time (AOT) compilers such as RyuJIT (.NET Core), mTouch, and LLVM (for Xamarin.iOS) in order to produce and execute the application binaries for the target platform.
Overall, .NET Core is a rapidly growing ecosystem with a large number of supported platforms, runtimes, and tools. Most of these components can be found on GitHub as open source projects under the supervision of the .NET Foundation. This open source growth is one of the key factors behind .NET Core reaching its peak today, where the implemented APIs almost fully match those of .NET Framework. This is why the .NET 5.0 release marks the end of the .NET era (as we know it) since the two .NET frameworks are being merged into one in this release, where .NET Core, de facto, replaces .NET itself. Let's now, without further ado, start developing in .NET 5.0.