In the same way that Python modules allow you to organize your functions and classes into separate Python source files, Python packages allow you to group multiple modules together.
A Python package is a directory with certain characteristics. For example, consider the following directory of Python source files:
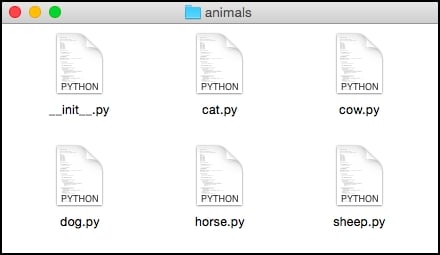
This Python package, called animals
, contains five Python modules: cat
, cow
, dog
, horse
, and sheep
. There is also a special file with the rather unusual name __init__.py
. This file is called a
package initialization file; the presence of this file tells the Python system that this directory contains a package. The package initialization file can also be used to initialize the package (hence the name) and can also be used to make importing the package easier.
Note
Starting with Python version 3.3, packages don't always need to include an initialization file. However, packages without an initialization file (called namespace packages) are still quite uncommon and are only used in very specific circumstances. To keep things simple, we will be using regular packages (with the __init__.py
file) throughout this book.
Just like we used the module name when calling a function within a module, we use the package name when referring to a module within a package. For example, consider the following code:
import animals.cow animals.cow.speak()
In this example, the speak()
function is defined within the cow.py
module, which itself is part of the animals
package.
Packages are a great way of organizing more complicated Python programs. You can use them to group related modules together, and you can even define packages inside packages (called nested packages) to keep your program super-organized.
Note that the import
statement (and the related from...import
statement) can be used in a variety of ways to load packages and modules into your program. We have only scratched the surface here, showing you what modules and packages look like in Python so that you can recognize them when you see them in a program. We will be looking at the way modules and packages can be defined and imported in much more depth in Chapter 3, Using Modules and Packages.
Tip
Downloading the example code
The code bundle for the book is also hosted on GitHub at https://github.com/PacktPublishing/Modular-Programming-with-Python. We also have other code bundles from our rich catalog of books and videos available at https://github.com/PacktPublishing/. Check them out!