Chapter 3. When Objects Are Alike
In the programming world, duplicate code is considered evil. We should not have multiple copies of the same, or similar, code in different places.
There are many ways to merge pieces of code or objects that have a similar functionality. In this chapter, we'll be covering the most famous object-oriented principle: inheritance. As discussed in Chapter 1, Object-oriented Design, inheritance allows us to create is a relationships between two or more classes, abstracting common logic into superclasses and managing specific details in the subclass. In particular, we'll be covering the Python syntax and principles for:
- Basic inheritance
- Inheriting from built-ins
- Multiple inheritance
- Polymorphism and duck typing
Basic inheritance
Technically, every class we create uses inheritance. All Python classes are subclasses of the special class named object
. This class provides very little in terms of data and behaviors (the behaviors it does provide are all double-underscore methods intended for internal use only), but it does allow Python to treat all objects in the same way.
If we don't explicitly inherit from a different class, our classes will automatically inherit from object
. However, we can openly state that our class derives from object
using the following syntax:
class MySubClass(object): pass
This is inheritance! This example is, technically, no different from our very first example in Chapter 2, Objects in Python, since Python 3 automatically inherits from object
if we don't explicitly provide a different superclass. A superclass, or parent class, is a class that is being inherited from. A subclass is a class that is inheriting from a superclass. In this case, the superclass is object
, and MySubClass
is the subclass. A subclass is also said to be derived from its parent class or that the subclass extends the parent.
As you've probably figured out from the example, inheritance requires a minimal amount of extra syntax over a basic class definition. Simply include the name of the parent class inside parentheses after the class name but before the colon terminating the class definition. This is all we have to do to tell Python that the new class should be derived from the given superclass.
How do we apply inheritance in practice? The simplest and most obvious use of inheritance is to add functionality to an existing class. Let's start with a simple contact manager that tracks the name and e-mail address of several people. The contact class is responsible for maintaining a list of all contacts in a class variable, and for initializing the name and address for an individual contact:
class Contact: all_contacts = [] def __init__(self, name, email): self.name = name self.email = email Contact.all_contacts.append(self)
This example introduces us to class variables. The all_contacts
list, because it is part of the class definition, is shared by all instances of this class. This means that there is only one Contact.all_contacts
list, which we can access as Contact.all_contacts
. Less obviously, we can also access it as self.all_contacts
on any object instantiated from Contact
. If the field can't be found on the object, then it will be found on the class and thus refer to the same single list.
Tip
Be careful with this syntax, for if you ever set the variable using self.all_contacts
, you will actually be creating a new instance variable associated only with that object. The class variable will still be unchanged and accessible as Contact.all_contacts
.
This is a simple class that allows us to track a couple pieces of data about each contact. But what if some of our contacts are also suppliers that we need to order supplies from? We could add an order
method to the Contact
class, but that would allow people to accidentally order things from contacts who are customers or family friends. Instead, let's create a new Supplier
class that acts like our Contact
class, but has an additional order
method:
class Supplier(Contact): def order(self, order): print("If this were a real system we would send " "'{}' order to '{}'".format(order, self.name))
Now, if we test this class in our trusty interpreter, we see that all contacts, including suppliers, accept a name and e-mail address in their __init__
, but only suppliers have a functional order method:
>>> c = Contact("Some Body", "[email protected]") >>> s = Supplier("Sup Plier", "[email protected]") >>> print(c.name, c.email, s.name, s.email) Some Body [email protected] Sup Plier [email protected] >>> c.all_contacts [<__main__.Contact object at 0xb7375ecc>, <__main__.Supplier object at 0xb7375f8c>] >>> c.order("I need pliers") Traceback (most recent call last): File "<stdin>", line 1, in <module> AttributeError: 'Contact' object has no attribute 'order' >>> s.order("I need pliers") If this were a real system we would send 'I need pliers' order to 'Sup Plier '
So, now our Supplier
class can do everything a contact can do (including adding itself to the list of all_contacts
) and all the special things it needs to handle as a supplier. This is the beauty of inheritance.
Extending built-ins
One interesting use of this kind of inheritance is adding functionality to built-in classes. In the Contact
class seen earlier, we are adding contacts to a list of all contacts. What if we also wanted to search that list by name? Well, we could add a method on the Contact
class to search it, but it feels like this method actually belongs to the list itself. We can do this using inheritance:
class ContactList(list):
def search(self, name):
'''Return all contacts that contain the search value
in their name.'''
matching_contacts = []
for contact in self:
if name in contact.name:
matching_contacts.append(contact)
return matching_contacts
class Contact:
all_contacts = ContactList()
def __init__(self, name, email):
self.name = name
self.email = email
self.all_contacts.append(self)
Instead of instantiating a normal list as our class variable, we create a new ContactList
class that extends the built-in list
. Then, we instantiate this subclass as our all_contacts
list. We can test the new search functionality as follows:
>>> c1 = Contact("John A", "[email protected]") >>> c2 = Contact("John B", "[email protected]") >>> c3 = Contact("Jenna C", "[email protected]") >>> [c.name for c in Contact.all_contacts.search('John')] ['John A', 'John B']
Are you wondering how we changed the built-in syntax []
into something we can inherit from? Creating an empty list with []
is actually a shorthand for creating an empty list using list()
; the two syntaxes behave identically:
>>> [] == list() True
In reality, the []
syntax is actually so-called syntax sugar that calls the list()
constructor under the hood. The list
data type is a class that we can extend. In fact, the list itself extends the object
class:
>>> isinstance([], object) True
As a second example, we can extend the dict
class, which is, similar to the list, the class that is constructed when using the {}
syntax shorthand:
class LongNameDict(dict): def longest_key(self): longest = None for key in self: if not longest or len(key) > len(longest): longest = key return longest
This is easy to test in the interactive interpreter:
>>> longkeys = LongNameDict() >>> longkeys['hello'] = 1 >>> longkeys['longest yet'] = 5 >>> longkeys['hello2'] = 'world' >>> longkeys.longest_key() 'longest yet'
Most built-in types can be similarly extended. Commonly extended built-ins are object
, list
, set
, dict
, file
, and str
. Numerical types such as int
and float
are also occasionally inherited from.
Overriding and super
So, inheritance is great for adding new behavior to existing classes, but what about changing behavior? Our contact
class allows only a name and an e-mail address. This may be sufficient for most contacts, but what if we want to add a phone number for our close friends?
As we saw in Chapter 2, Objects in Python, we can do this easily by just setting a phone
attribute on the contact after it is constructed. But if we want to make this third variable available on initialization, we have to override __init__
. Overriding means altering or replacing a method of the superclass with a new method (with the same name) in the subclass. No special syntax is needed to do this; the subclass's newly created method is automatically called instead of the superclass's method. For example:
class Friend(Contact): def __init__(self, name, email, phone): self.name = name self.email = email self.phone = phone
Any method can be overridden, not just __init__
. Before we go on, however, we need to address some problems in this example. Our Contact
and Friend
classes have duplicate code to set up the name
and email
properties; this can make code maintenance complicated as we have to update the code in two or more places. More alarmingly, our Friend
class is neglecting to add itself to the all_contacts
list we have created on the Contact
class.
What we really need is a way to execute the original __init__
method on the Contact
class. This is what the super
function does; it returns the object as an instance of the parent class, allowing us to call the parent method directly:
class Friend(Contact):
def __init__(self, name, email, phone):
super().__init__(name, email)
self.phone = phone
This example first gets the instance of the parent object using super
, and calls __init__
on that object, passing in the expected arguments. It then does its own initialization, namely, setting the phone
attribute.
Note
Note that the super()
syntax does not work in older versions of Python. Like the [] and {} syntaxes for lists and dictionaries, it is a shorthand for a more complicated construct. We'll learn more about this shortly when we discuss multiple inheritance, but know for now that in Python 2, you would have to call super(EmailContact, self).__init__()
. Specifically notice that the first argument is the name of the child class, not the name as the parent class you want to call, as some might expect. Also, remember the class comes before the object. I always forget the order, so the new syntax in Python 3 has saved me hours of having to look it up.
A super()
call can be made inside any method, not just __init__
. This means all methods can be modified via overriding and calls to super
. The call to super
can also be made at any point in the method; we don't have to make the call as the first line in the method. For example, we may need to manipulate or validate incoming parameters before forwarding them to the superclass.
Multiple inheritance
Multiple inheritance is a touchy subject. In principle, it's very simple: a subclass that inherits from more than one parent class is able to access functionality from both of them. In practice, this is less useful than it sounds and many expert programmers recommend against using it.
Tip
As a rule of thumb, if you think you need multiple inheritance, you're probably wrong, but if you know you need it, you're probably right.
The simplest and most useful form of multiple inheritance is called a mixin. A mixin is generally a superclass that is not meant to exist on its own, but is meant to be inherited by some other class to provide extra functionality. For example, let's say we wanted to add functionality to our Contact
class that allows sending an e-mail to self.email
. Sending e-mail is a common task that we might want to use on many other classes. So, we can write a simple mixin class to do the e-mailing for us:
class MailSender: def send_mail(self, message): print("Sending mail to " + self.email) # Add e-mail logic here
For brevity, we won't include the actual e-mail logic here; if you're interested in studying how it's done, see the smtplib
module in the Python standard library.
This class doesn't do anything special (in fact, it can barely function as a standalone class), but it does allow us to define a new class that describes both a Contact
and a MailSender
, using multiple inheritance:
class EmailableContact(Contact, MailSender): pass
The syntax for multiple inheritance looks like a parameter list in the class definition. Instead of including one base class inside the parentheses, we include two (or more), separated by a comma. We can test this new hybrid to see the mixin at work:
>>> e = EmailableContact("John Smith", "[email protected]") >>> Contact.all_contacts [<__main__.EmailableContact object at 0xb7205fac>] >>> e.send_mail("Hello, test e-mail here") Sending mail to [email protected]
The Contact
initializer is still adding the new contact to the all_contacts
list, and the mixin is able to send mail to self.email
so we know everything is working.
This wasn't so hard, and you're probably wondering what the dire warnings about multiple inheritance are. We'll get into the complexities in a minute, but let's consider some other options we had, rather than using a mixin here:
- We could have used single inheritance and added the
send_mail
function to the subclass. The disadvantage here is that the e-mail functionality then has to be duplicated for any other classes that need e-mail. - We can create a standalone Python function for sending an e-mail, and just call that function with the correct e-mail address supplied as a parameter when the e-mail needs to be sent.
- We could have explored a few ways of using composition instead of inheritance. For example,
EmailableContact
could have aMailSender
object instead of inheriting from it. - We could monkey-patch (we'll briefly cover monkey-patching in Chapter 7, Python Object-oriented Shortcuts) the
Contact
class to have asend_mail
method after the class has been created. This is done by defining a function that accepts theself
argument, and setting it as an attribute on an existing class.
Multiple inheritance works all right when mixing methods from different classes, but it gets very messy when we have to call methods on the superclass. There are multiple superclasses. How do we know which one to call? How do we know what order to call them in?
Let's explore these questions by adding a home address to our Friend
class. There are a few approaches we might take. An address is a collection of strings representing the street, city, country, and other related details of the contact. We could pass each of these strings as a parameter into the Friend
class's __init__
method. We could also store these strings in a tuple or dictionary and pass them into __init__
as a single argument. This is probably the best course of action if there are no methods that need to be added to the address.
Another option would be to create a new Address
class to hold those strings together, and then pass an instance of this class into the __init__
method of our Friend
class. The advantage of this solution is that we can add behavior (say, a method to give directions or to print a map) to the data instead of just storing it statically. This is an example of composition, as we discussed in Chapter 1, Object-oriented Design. The "has a" relationship of composition is a perfectly viable solution to this problem and allows us to reuse Address
classes in other entities such as buildings, businesses, or organizations.
However, inheritance is also a viable solution, and that's what we want to explore. Let's add a new class that holds an address. We'll call this new class "AddressHolder" instead of "Address" because inheritance defines an is a relationship. It is not correct to say a "Friend" is an "Address" , but since a friend can have an "Address" , we can argue that a "Friend" is an "AddressHolder". Later, we could create other entities (companies, buildings) that also hold addresses. Here's our AddressHolder
class:
class AddressHolder: def __init__(self, street, city, state, code): self.street = street self.city = city self.state = state self.code = code
Very simple; we just take all the data and toss it into instance variables upon initialization.
The diamond problem
We can use multiple inheritance to add this new class as a parent of our existing Friend
class. The tricky part is that we now have two parent __init__
methods both of which need to be initialized. And they need to be initialized with different arguments. How do we do this? Well, we could start with a naive approach:
class Friend(Contact, AddressHolder): def __init__( self, name, email, phone,street, city, state, code): Contact.__init__(self, name, email) AddressHolder.__init__(self, street, city, state, code) self.phone = phone
In this example, we directly call the __init__
function on each of the superclasses and explicitly pass the self
argument. This example technically works; we can access the different variables directly on the class. But there are a few problems.
First, it is possible for a superclass to go uninitialized if we neglect to explicitly call the initializer. That wouldn't break this example, but it could cause hard-to-debug program crashes in common scenarios. Imagine trying to insert data into a database that has not been connected to, for example.
Second, and more sinister, is the possibility of a superclass being called multiple times because of the organization of the class hierarchy. Look at this inheritance diagram:
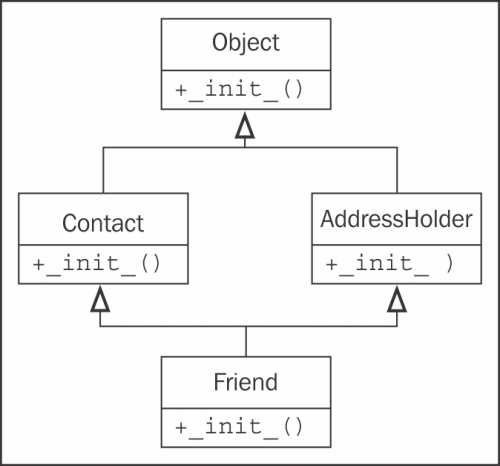
The __init__
method from the Friend
class first calls __init__
on Contact
, which implicitly initializes the object
superclass (remember, all classes derive from object
). Friend
then calls __init__
on AddressHolder
, which implicitly initializes the object
superclass again. This means the parent class has been set up twice. With the object
class, that's relatively harmless, but in some situations, it could spell disaster. Imagine trying to connect to a database twice for every request!
The base class should only be called once. Once, yes, but when? Do we call Friend
, then Contact
, then Object
, then AddressHolder
? Or Friend
, then Contact
, then AddressHolder
, then Object
?
Tip
The order in which methods can be called can be adapted on the fly by modifying the __mro__
(Method Resolution Order) attribute on the class. This is beyond the scope of this book. If you think you need to understand it, I recommend Expert Python Programming, Tarek Ziadé, Packt Publishing, or read the original documentation on the topic at http://www.python.org/download/releases/2.3/mro/.
Let's look at a second contrived example that illustrates this problem more clearly. Here we have a base class that has a method named call_me
. Two subclasses override that method, and then another subclass extends both of these using multiple inheritance. This is called diamond inheritance because of the diamond shape of the class diagram:
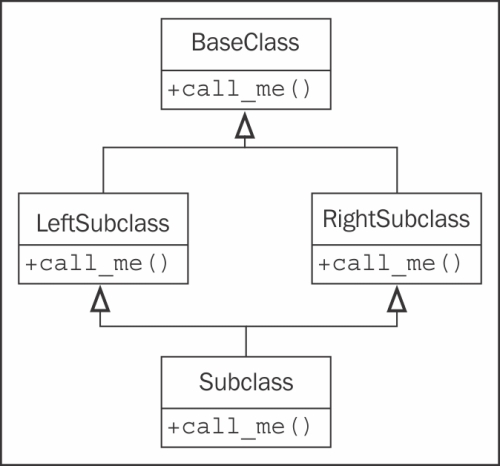
Let's convert this diagram to code; this example shows when the methods are called:
class BaseClass: num_base_calls = 0 def call_me(self): print("Calling method on Base Class") self.num_base_calls += 1 class LeftSubclass(BaseClass): num_left_calls = 0 def call_me(self): BaseClass.call_me(self) print("Calling method on Left Subclass") self.num_left_calls += 1 class RightSubclass(BaseClass): num_right_calls = 0 def call_me(self): BaseClass.call_me(self) print("Calling method on Right Subclass") self.num_right_calls += 1 class Subclass(LeftSubclass, RightSubclass): num_sub_calls = 0 def call_me(self): LeftSubclass.call_me(self) RightSubclass.call_me(self) print("Calling method on Subclass") self.num_sub_calls += 1
This example simply ensures that each overridden call_me
method directly calls the parent method with the same name. It lets us know each time a method is called by printing the information to the screen. It also updates a static variable on the class to show how many times it has been called. If we instantiate one Subclass
object and call the method on it once, we get this output:
>>> s = Subclass() >>> s.call_me() Calling method on Base Class Calling method on Left Subclass Calling method on Base Class Calling method on Right Subclass Calling method on Subclass >>> print( ... s.num_sub_calls, ... s.num_left_calls, ... s.num_right_calls, ... s.num_base_calls) 1 1 1 2
Thus we can clearly see the base class's call_me
method being called twice. This could lead to some insidious bugs if that method is doing actual work—like depositing into a bank account—twice.
The thing to keep in mind with multiple inheritance is that we only want to call the "next" method in the class hierarchy, not the "parent" method. In fact, that next method may not be on a parent or ancestor of the current class. The super
keyword comes to our rescue once again. Indeed, super
was originally developed to make complicated forms of multiple inheritance possible. Here is the same code written using super
:
class BaseClass: num_base_calls = 0 def call_me(self): print("Calling method on Base Class") self.num_base_calls += 1 class LeftSubclass(BaseClass): num_left_calls = 0 def call_me(self): super().call_me() print("Calling method on Left Subclass") self.num_left_calls += 1 class RightSubclass(BaseClass): num_right_calls = 0 def call_me(self): super().call_me() print("Calling method on Right Subclass") self.num_right_calls += 1 class Subclass(LeftSubclass, RightSubclass): num_sub_calls = 0 def call_me(self): super().call_me() print("Calling method on Subclass") self.num_sub_calls += 1
The change is pretty minor; we simply replaced the naive direct calls with calls to super()
, although the bottom subclass only calls super
once rather than having to make the calls for both the left and right. The change is simple enough, but look at the difference when we execute it:
>>> s = Subclass() >>> s.call_me() Calling method on Base Class Calling method on Right Subclass Calling method on Left Subclass Calling method on Subclass >>> print(s.num_sub_calls, s.num_left_calls, s.num_right_calls, s.num_base_calls) 1 1 1 1
Looks good, our base method is only being called once. But what is super()
actually doing here? Since the print
statements are executed after the super
calls, the printed output is in the order each method is actually executed. Let's look at the output from back to front to see who is calling what.
First, call_me
of Subclass
calls super().call_me()
, which happens to refer to LeftSubclass.call_me()
. The LeftSubclass.call_me()
method then calls super().call_me()
, but in this case, super()
is referring to RightSubclass.call_me()
.
Pay particular attention to this: the super
call is not calling the method on the superclass of LeftSubclass
(which is BaseClass
). Rather, it is calling RightSubclass
, even though it is not a direct parent of LeftSubclass
! This is the next method, not the parent method. RightSubclass
then calls BaseClass
and the super
calls have ensured each method in the class hierarchy is executed once.
Different sets of arguments
This is going to make things complicated as we return to our Friend
multiple inheritance example. In the __init__
method for Friend
, we were originally calling __init__
for both parent classes, with different sets of arguments:
Contact.__init__(self, name, email) AddressHolder.__init__(self, street, city, state, code)
How can we manage different sets of arguments when using super
? We don't necessarily know which class super
is going to try to initialize first. Even if we did, we need a way to pass the "extra" arguments so that subsequent calls to super
, on other subclasses, receive the right arguments.
Specifically, if the first call to super
passes the name
and email
arguments to Contact.__init__
, and Contact.__init__
then calls super
, it needs to be able to pass the address-related arguments to the "next" method, which is AddressHolder.__init__
.
This is a problem whenever we want to call superclass methods with the same name, but with different sets of arguments. Most often, the only time you would want to call a superclass with a completely different set of arguments is in __init__
, as we're doing here. Even with regular methods, though, we may want to add optional parameters that only make sense to one subclass or set of subclasses.
Sadly, the only way to solve this problem is to plan for it from the beginning. We have to design our base class parameter lists to accept keyword arguments for any parameters that are not required by every subclass implementation. Finally, we must ensure the method freely accepts unexpected arguments and passes them on to its super
call, in case they are necessary to later methods in the inheritance order.
Python's function parameter syntax provides all the tools we need to do this, but it makes the overall code look cumbersome. Have a look at the proper version of the Friend
multiple inheritance code:
class Contact: all_contacts = [] def __init__(self, name='', email='', **kwargs): super().__init__(**kwargs) self.name = name self.email = email self.all_contacts.append(self) class AddressHolder: def __init__(self, street='', city='', state='', code='', **kwargs): super().__init__(**kwargs) self.street = street self.city = city self.state = state self.code = code class Friend(Contact, AddressHolder): def __init__(self, phone='', **kwargs): super().__init__(**kwargs) self.phone = phone
We've changed all arguments to keyword arguments by giving them an empty string as a default value. We've also ensured that a **kwargs
parameter is included to capture any additional parameters that our particular method doesn't know what to do with. It passes these parameters up to the next class with the super
call.
Tip
If you aren't familiar with the **kwargs
syntax, it basically collects any keyword arguments passed into the method that were not explicitly listed in the parameter list. These arguments are stored in a dictionary named kwargs
(we can call the variable whatever we like, but convention suggests kw
, or kwargs
). When we call a different method (for example, super().__init__
) with a **kwargs
syntax, it unpacks the dictionary and passes the results to the method as normal keyword arguments. We'll cover this in detail in Chapter 7, Python Object-oriented Shortcuts.
The previous example does what it is supposed to do. But it's starting to look messy, and it has become difficult to answer the question, What arguments do we need to pass into
Friend.__init__
? This is the foremost question for anyone planning to use the class, so a docstring should be added to the method to explain what is happening.
Further, even this implementation is insufficient if we want to reuse variables in parent classes. When we pass the **kwargs
variable to super
, the dictionary does not include any of the variables that were included as explicit keyword arguments. For example, in Friend.__init__
, the call to super
does not have phone
in the kwargs
dictionary. If any of the other classes need the phone
parameter, we need to ensure it is in the dictionary that is passed. Worse, if we forget to do this, it will be tough to debug because the superclass will not complain, but will simply assign the default value (in this case, an empty string) to the variable.
There are a few ways to ensure that the variable is passed upwards. Assume the Contact
class does, for some reason, need to be initialized with a phone
parameter, and the Friend
class will also need access to it. We can do any of the following:
- Don't include
phone
as an explicit keyword argument. Instead, leave it in thekwargs
dictionary.Friend
can look it up using the syntaxkwargs['phone']
. When it passes**kwargs
to thesuper
call,phone
will still be in the dictionary. - Make
phone
an explicit keyword argument but update thekwargs
dictionary before passing it tosuper
, using the standard dictionary syntaxkwargs['phone'] = phone
. - Make
phone
an explicit keyword argument, but update thekwargs
dictionary using thekwargs.update
method. This is useful if you have several arguments to update. You can create the dictionary passed intoupdate
using either thedict(phone=phone)
constructor, or the dictionary syntax{'phone': phone}
. - Make
phone
an explicit keyword argument, but pass it to the super call explicitly with the syntaxsuper().__init__(phone=phone, **kwargs)
.
We have covered many of the caveats involved with multiple inheritance in Python. When we need to account for all the possible situations, we have to plan for them and our code will get messy. Basic multiple inheritance can be handy but, in many cases, we may want to choose a more transparent way of combining two disparate classes, usually using composition or one of the design patterns we'll be covering in Chapter 10, Python Design Patterns I and Chapter 11, Python Design Patterns II.
The diamond problem
We can use multiple inheritance to add this new class as a parent of our existing Friend
class. The tricky part is that we now have two parent __init__
methods both of which need to be initialized. And they need to be initialized with different arguments. How do we do this? Well, we could start with a naive approach:
class Friend(Contact, AddressHolder): def __init__( self, name, email, phone,street, city, state, code): Contact.__init__(self, name, email) AddressHolder.__init__(self, street, city, state, code) self.phone = phone
In this example, we directly call the __init__
function on each of the superclasses and explicitly pass the self
argument. This example technically works; we can access the different variables directly on the class. But there are a few problems.
First, it is possible for a superclass to go uninitialized if we neglect to explicitly call the initializer. That wouldn't break this example, but it could cause hard-to-debug program crashes in common scenarios. Imagine trying to insert data into a database that has not been connected to, for example.
Second, and more sinister, is the possibility of a superclass being called multiple times because of the organization of the class hierarchy. Look at this inheritance diagram:
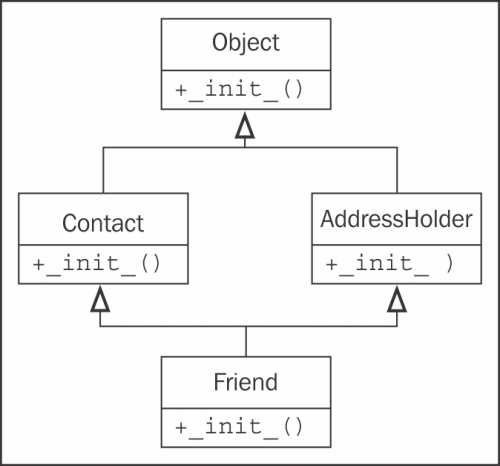
The __init__
method from the Friend
class first calls __init__
on Contact
, which implicitly initializes the object
superclass (remember, all classes derive from object
). Friend
then calls __init__
on AddressHolder
, which implicitly initializes the object
superclass again. This means the parent class has been set up twice. With the object
class, that's relatively harmless, but in some situations, it could spell disaster. Imagine trying to connect to a database twice for every request!
The base class should only be called once. Once, yes, but when? Do we call Friend
, then Contact
, then Object
, then AddressHolder
? Or Friend
, then Contact
, then AddressHolder
, then Object
?
Tip
The order in which methods can be called can be adapted on the fly by modifying the __mro__
(Method Resolution Order) attribute on the class. This is beyond the scope of this book. If you think you need to understand it, I recommend Expert Python Programming, Tarek Ziadé, Packt Publishing, or read the original documentation on the topic at http://www.python.org/download/releases/2.3/mro/.
Let's look at a second contrived example that illustrates this problem more clearly. Here we have a base class that has a method named call_me
. Two subclasses override that method, and then another subclass extends both of these using multiple inheritance. This is called diamond inheritance because of the diamond shape of the class diagram:
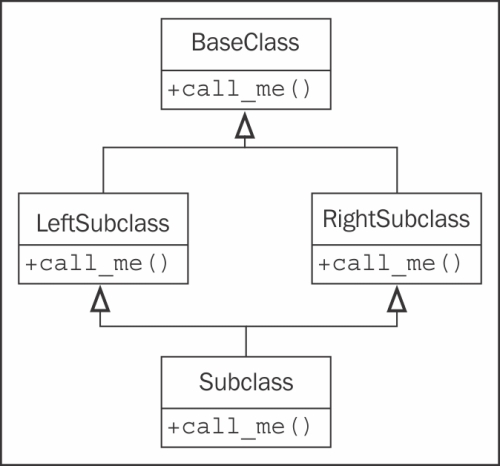
Let's convert this diagram to code; this example shows when the methods are called:
class BaseClass: num_base_calls = 0 def call_me(self): print("Calling method on Base Class") self.num_base_calls += 1 class LeftSubclass(BaseClass): num_left_calls = 0 def call_me(self): BaseClass.call_me(self) print("Calling method on Left Subclass") self.num_left_calls += 1 class RightSubclass(BaseClass): num_right_calls = 0 def call_me(self): BaseClass.call_me(self) print("Calling method on Right Subclass") self.num_right_calls += 1 class Subclass(LeftSubclass, RightSubclass): num_sub_calls = 0 def call_me(self): LeftSubclass.call_me(self) RightSubclass.call_me(self) print("Calling method on Subclass") self.num_sub_calls += 1
This example simply ensures that each overridden call_me
method directly calls the parent method with the same name. It lets us know each time a method is called by printing the information to the screen. It also updates a static variable on the class to show how many times it has been called. If we instantiate one Subclass
object and call the method on it once, we get this output:
>>> s = Subclass() >>> s.call_me() Calling method on Base Class Calling method on Left Subclass Calling method on Base Class Calling method on Right Subclass Calling method on Subclass >>> print( ... s.num_sub_calls, ... s.num_left_calls, ... s.num_right_calls, ... s.num_base_calls) 1 1 1 2
Thus we can clearly see the base class's call_me
method being called twice. This could lead to some insidious bugs if that method is doing actual work—like depositing into a bank account—twice.
The thing to keep in mind with multiple inheritance is that we only want to call the "next" method in the class hierarchy, not the "parent" method. In fact, that next method may not be on a parent or ancestor of the current class. The super
keyword comes to our rescue once again. Indeed, super
was originally developed to make complicated forms of multiple inheritance possible. Here is the same code written using super
:
class BaseClass: num_base_calls = 0 def call_me(self): print("Calling method on Base Class") self.num_base_calls += 1 class LeftSubclass(BaseClass): num_left_calls = 0 def call_me(self): super().call_me() print("Calling method on Left Subclass") self.num_left_calls += 1 class RightSubclass(BaseClass): num_right_calls = 0 def call_me(self): super().call_me() print("Calling method on Right Subclass") self.num_right_calls += 1 class Subclass(LeftSubclass, RightSubclass): num_sub_calls = 0 def call_me(self): super().call_me() print("Calling method on Subclass") self.num_sub_calls += 1
The change is pretty minor; we simply replaced the naive direct calls with calls to super()
, although the bottom subclass only calls super
once rather than having to make the calls for both the left and right. The change is simple enough, but look at the difference when we execute it:
>>> s = Subclass() >>> s.call_me() Calling method on Base Class Calling method on Right Subclass Calling method on Left Subclass Calling method on Subclass >>> print(s.num_sub_calls, s.num_left_calls, s.num_right_calls, s.num_base_calls) 1 1 1 1
Looks good, our base method is only being called once. But what is super()
actually doing here? Since the print
statements are executed after the super
calls, the printed output is in the order each method is actually executed. Let's look at the output from back to front to see who is calling what.
First, call_me
of Subclass
calls super().call_me()
, which happens to refer to LeftSubclass.call_me()
. The LeftSubclass.call_me()
method then calls super().call_me()
, but in this case, super()
is referring to RightSubclass.call_me()
.
Pay particular attention to this: the super
call is not calling the method on the superclass of LeftSubclass
(which is BaseClass
). Rather, it is calling RightSubclass
, even though it is not a direct parent of LeftSubclass
! This is the next method, not the parent method. RightSubclass
then calls BaseClass
and the super
calls have ensured each method in the class hierarchy is executed once.
Different sets of arguments
This is going to make things complicated as we return to our Friend
multiple inheritance example. In the __init__
method for Friend
, we were originally calling __init__
for both parent classes, with different sets of arguments:
Contact.__init__(self, name, email) AddressHolder.__init__(self, street, city, state, code)
How can we manage different sets of arguments when using super
? We don't necessarily know which class super
is going to try to initialize first. Even if we did, we need a way to pass the "extra" arguments so that subsequent calls to super
, on other subclasses, receive the right arguments.
Specifically, if the first call to super
passes the name
and email
arguments to Contact.__init__
, and Contact.__init__
then calls super
, it needs to be able to pass the address-related arguments to the "next" method, which is AddressHolder.__init__
.
This is a problem whenever we want to call superclass methods with the same name, but with different sets of arguments. Most often, the only time you would want to call a superclass with a completely different set of arguments is in __init__
, as we're doing here. Even with regular methods, though, we may want to add optional parameters that only make sense to one subclass or set of subclasses.
Sadly, the only way to solve this problem is to plan for it from the beginning. We have to design our base class parameter lists to accept keyword arguments for any parameters that are not required by every subclass implementation. Finally, we must ensure the method freely accepts unexpected arguments and passes them on to its super
call, in case they are necessary to later methods in the inheritance order.
Python's function parameter syntax provides all the tools we need to do this, but it makes the overall code look cumbersome. Have a look at the proper version of the Friend
multiple inheritance code:
class Contact: all_contacts = [] def __init__(self, name='', email='', **kwargs): super().__init__(**kwargs) self.name = name self.email = email self.all_contacts.append(self) class AddressHolder: def __init__(self, street='', city='', state='', code='', **kwargs): super().__init__(**kwargs) self.street = street self.city = city self.state = state self.code = code class Friend(Contact, AddressHolder): def __init__(self, phone='', **kwargs): super().__init__(**kwargs) self.phone = phone
We've changed all arguments to keyword arguments by giving them an empty string as a default value. We've also ensured that a **kwargs
parameter is included to capture any additional parameters that our particular method doesn't know what to do with. It passes these parameters up to the next class with the super
call.
Tip
If you aren't familiar with the **kwargs
syntax, it basically collects any keyword arguments passed into the method that were not explicitly listed in the parameter list. These arguments are stored in a dictionary named kwargs
(we can call the variable whatever we like, but convention suggests kw
, or kwargs
). When we call a different method (for example, super().__init__
) with a **kwargs
syntax, it unpacks the dictionary and passes the results to the method as normal keyword arguments. We'll cover this in detail in Chapter 7, Python Object-oriented Shortcuts.
The previous example does what it is supposed to do. But it's starting to look messy, and it has become difficult to answer the question, What arguments do we need to pass into
Friend.__init__
? This is the foremost question for anyone planning to use the class, so a docstring should be added to the method to explain what is happening.
Further, even this implementation is insufficient if we want to reuse variables in parent classes. When we pass the **kwargs
variable to super
, the dictionary does not include any of the variables that were included as explicit keyword arguments. For example, in Friend.__init__
, the call to super
does not have phone
in the kwargs
dictionary. If any of the other classes need the phone
parameter, we need to ensure it is in the dictionary that is passed. Worse, if we forget to do this, it will be tough to debug because the superclass will not complain, but will simply assign the default value (in this case, an empty string) to the variable.
There are a few ways to ensure that the variable is passed upwards. Assume the Contact
class does, for some reason, need to be initialized with a phone
parameter, and the Friend
class will also need access to it. We can do any of the following:
- Don't include
phone
as an explicit keyword argument. Instead, leave it in thekwargs
dictionary.Friend
can look it up using the syntaxkwargs['phone']
. When it passes**kwargs
to thesuper
call,phone
will still be in the dictionary. - Make
phone
an explicit keyword argument but update thekwargs
dictionary before passing it tosuper
, using the standard dictionary syntaxkwargs['phone'] = phone
. - Make
phone
an explicit keyword argument, but update thekwargs
dictionary using thekwargs.update
method. This is useful if you have several arguments to update. You can create the dictionary passed intoupdate
using either thedict(phone=phone)
constructor, or the dictionary syntax{'phone': phone}
. - Make
phone
an explicit keyword argument, but pass it to the super call explicitly with the syntaxsuper().__init__(phone=phone, **kwargs)
.
We have covered many of the caveats involved with multiple inheritance in Python. When we need to account for all the possible situations, we have to plan for them and our code will get messy. Basic multiple inheritance can be handy but, in many cases, we may want to choose a more transparent way of combining two disparate classes, usually using composition or one of the design patterns we'll be covering in Chapter 10, Python Design Patterns I and Chapter 11, Python Design Patterns II.
Different sets of arguments
This is going to make things complicated as we return to our Friend
multiple inheritance example. In the __init__
method for Friend
, we were originally calling __init__
for both parent classes, with different sets of arguments:
Contact.__init__(self, name, email) AddressHolder.__init__(self, street, city, state, code)
How can we manage different sets of arguments when using super
? We don't necessarily know which class super
is going to try to initialize first. Even if we did, we need a way to pass the "extra" arguments so that subsequent calls to super
, on other subclasses, receive the right arguments.
Specifically, if the first call to super
passes the name
and email
arguments to Contact.__init__
, and Contact.__init__
then calls super
, it needs to be able to pass the address-related arguments to the "next" method, which is AddressHolder.__init__
.
This is a problem whenever we want to call superclass methods with the same name, but with different sets of arguments. Most often, the only time you would want to call a superclass with a completely different set of arguments is in __init__
, as we're doing here. Even with regular methods, though, we may want to add optional parameters that only make sense to one subclass or set of subclasses.
Sadly, the only way to solve this problem is to plan for it from the beginning. We have to design our base class parameter lists to accept keyword arguments for any parameters that are not required by every subclass implementation. Finally, we must ensure the method freely accepts unexpected arguments and passes them on to its super
call, in case they are necessary to later methods in the inheritance order.
Python's function parameter syntax provides all the tools we need to do this, but it makes the overall code look cumbersome. Have a look at the proper version of the Friend
multiple inheritance code:
class Contact: all_contacts = [] def __init__(self, name='', email='', **kwargs): super().__init__(**kwargs) self.name = name self.email = email self.all_contacts.append(self) class AddressHolder: def __init__(self, street='', city='', state='', code='', **kwargs): super().__init__(**kwargs) self.street = street self.city = city self.state = state self.code = code class Friend(Contact, AddressHolder): def __init__(self, phone='', **kwargs): super().__init__(**kwargs) self.phone = phone
We've changed all arguments to keyword arguments by giving them an empty string as a default value. We've also ensured that a **kwargs
parameter is included to capture any additional parameters that our particular method doesn't know what to do with. It passes these parameters up to the next class with the super
call.
Tip
If you aren't familiar with the **kwargs
syntax, it basically collects any keyword arguments passed into the method that were not explicitly listed in the parameter list. These arguments are stored in a dictionary named kwargs
(we can call the variable whatever we like, but convention suggests kw
, or kwargs
). When we call a different method (for example, super().__init__
) with a **kwargs
syntax, it unpacks the dictionary and passes the results to the method as normal keyword arguments. We'll cover this in detail in Chapter 7, Python Object-oriented Shortcuts.
The previous example does what it is supposed to do. But it's starting to look messy, and it has become difficult to answer the question, What arguments do we need to pass into
Friend.__init__
? This is the foremost question for anyone planning to use the class, so a docstring should be added to the method to explain what is happening.
Further, even this implementation is insufficient if we want to reuse variables in parent classes. When we pass the **kwargs
variable to super
, the dictionary does not include any of the variables that were included as explicit keyword arguments. For example, in Friend.__init__
, the call to super
does not have phone
in the kwargs
dictionary. If any of the other classes need the phone
parameter, we need to ensure it is in the dictionary that is passed. Worse, if we forget to do this, it will be tough to debug because the superclass will not complain, but will simply assign the default value (in this case, an empty string) to the variable.
There are a few ways to ensure that the variable is passed upwards. Assume the Contact
class does, for some reason, need to be initialized with a phone
parameter, and the Friend
class will also need access to it. We can do any of the following:
- Don't include
phone
as an explicit keyword argument. Instead, leave it in thekwargs
dictionary.Friend
can look it up using the syntaxkwargs['phone']
. When it passes**kwargs
to thesuper
call,phone
will still be in the dictionary. - Make
phone
an explicit keyword argument but update thekwargs
dictionary before passing it tosuper
, using the standard dictionary syntaxkwargs['phone'] = phone
. - Make
phone
an explicit keyword argument, but update thekwargs
dictionary using thekwargs.update
method. This is useful if you have several arguments to update. You can create the dictionary passed intoupdate
using either thedict(phone=phone)
constructor, or the dictionary syntax{'phone': phone}
. - Make
phone
an explicit keyword argument, but pass it to the super call explicitly with the syntaxsuper().__init__(phone=phone, **kwargs)
.
We have covered many of the caveats involved with multiple inheritance in Python. When we need to account for all the possible situations, we have to plan for them and our code will get messy. Basic multiple inheritance can be handy but, in many cases, we may want to choose a more transparent way of combining two disparate classes, usually using composition or one of the design patterns we'll be covering in Chapter 10, Python Design Patterns I and Chapter 11, Python Design Patterns II.
Polymorphism
We were introduced to polymorphism in Chapter 1, Object-oriented Design. It is a fancy name describing a simple concept: different behaviors happen depending on which subclass is being used, without having to explicitly know what the subclass actually is. As an example, imagine a program that plays audio files. A media player might need to load an AudioFile
object and then play
it. We'd put a play()
method on the object, which is responsible for decompressing or extracting the audio and routing it to the sound card and speakers. The act of playing an AudioFile
could feasibly be as simple as:
audio_file.play()
However, the process of decompressing and extracting an audio file is very different for different types of files. The .wav
files are stored uncompressed, while .mp3
, .wma
, and .ogg
files all have totally different compression algorithms.
We can use inheritance with polymorphism to simplify the design. Each type of file can be represented by a different subclass of AudioFile
, for example, WavFile
, MP3File
. Each of these would have a play()
method, but that method would be implemented differently for each file to ensure the correct extraction procedure is followed. The media player object would never need to know which subclass of AudioFile
it is referring to; it just calls play()
and polymorphically lets the object take care of the actual details of playing. Let's look at a quick skeleton showing how this might look:
class AudioFile: def __init__(self, filename): if not filename.endswith(self.ext): raise Exception("Invalid file format") self.filename = filename class MP3File(AudioFile): ext = "mp3" def play(self): print("playing {} as mp3".format(self.filename)) class WavFile(AudioFile): ext = "wav" def play(self): print("playing {} as wav".format(self.filename)) class OggFile(AudioFile): ext = "ogg" def play(self): print("playing {} as ogg".format(self.filename))
All audio files check to ensure that a valid extension was given upon initialization. But did you notice how the __init__
method in the parent class is able to access the ext
class variable from different subclasses? That's polymorphism at work. If the filename doesn't end with the correct name, it raises an exception (exceptions will be covered in detail in the next chapter). The fact that AudioFile
doesn't actually store a reference to the ext
variable doesn't stop it from being able to access it on the subclass.
In addition, each subclass of AudioFile
implements play()
in a different way (this example doesn't actually play the music; audio compression algorithms really deserve a separate book!). This is also polymorphism in action. The media player can use the exact same code to play a file, no matter what type it is; it doesn't care what subclass of AudioFile
it is looking at. The details of decompressing the audio file are encapsulated. If we test this example, it works as we would hope:
>>> ogg = OggFile("myfile.ogg") >>> ogg.play() playing myfile.ogg as ogg >>> mp3 = MP3File("myfile.mp3") >>> mp3.play() playing myfile.mp3 as mp3 >>> not_an_mp3 = MP3File("myfile.ogg") Traceback (most recent call last): File "<stdin>", line 1, in <module> File "polymorphic_audio.py", line 4, in __init__ raise Exception("Invalid file format") Exception: Invalid file format
See how AudioFile.__init__
is able to check the file type without actually knowing what subclass it is referring to?
Polymorphism is actually one of the coolest things about object-oriented programming, and it makes some programming designs obvious that weren't possible in earlier paradigms. However, Python makes polymorphism less cool because of duck typing. Duck typing in Python allows us to use any object that provides the required behavior without forcing it to be a subclass. The dynamic nature of Python makes this trivial. The following example does not extend AudioFile
, but it can be interacted with in Python using the exact same interface:
class FlacFile: def __init__(self, filename): if not filename.endswith(".flac"): raise Exception("Invalid file format") self.filename = filename def play(self): print("playing {} as flac".format(self.filename))
Our media player can play this object just as easily as one that extends AudioFile
.
Polymorphism is one of the most important reasons to use inheritance in many object-oriented contexts. Because any objects that supply the correct interface can be used interchangeably in Python, it reduces the need for polymorphic common superclasses. Inheritance can still be useful for sharing code but, if all that is being shared is the public interface, duck typing is all that is required. This reduced need for inheritance also reduces the need for multiple inheritance; often, when multiple inheritance appears to be a valid solution, we can just use duck typing to mimic one of the multiple superclasses.
Of course, just because an object satisfies a particular interface (by providing required methods or attributes) does not mean it will simply work in all situations. It has to fulfill that interface in a way that makes sense in the overall system. Just because an object provides a play()
method does not mean it will automatically work with a media player. For example, our chess AI object from Chapter 1, Object-oriented Design, may have a play()
method that moves a chess piece. Even though it satisfies the interface, this class would likely break in spectacular ways if we tried to plug it into a media player!
Another useful feature of duck typing is that the duck-typed object only needs to provide those methods and attributes that are actually being accessed. For example, if we needed to create a fake file object to read data from, we can create a new object that has a read()
method; we don't have to override the write
method if the code that is going to interact with the object will only be reading from the file. More succinctly, duck typing doesn't need to provide the entire interface of an object that is available, it only needs to fulfill the interface that is actually accessed.
Abstract base classes
While duck typing is useful, it is not always easy to tell in advance if a class is going to fulfill the protocol you require. Therefore, Python introduced the idea of abstract base classes. Abstract base classes, or ABCs, define a set of methods and properties that a class must implement in order to be considered a duck-type instance of that class. The class can extend the abstract base class itself in order to be used as an instance of that class, but it must supply all the appropriate methods.
In practice, it's rarely necessary to create new abstract base classes, but we may find occasions to implement instances of existing ABCs. We'll cover implementing ABCs first, and then briefly see how to create your own if you should ever need to.
Using an abstract base class
Most of the abstract base classes that exist in the Python Standard Library live in the collections
module. One of the simplest ones is the Container
class. Let's inspect it in the Python interpreter to see what methods this class requires:
>>> from collections import Container >>> Container.__abstractmethods__ frozenset(['__contains__'])
So, the Container
class has exactly one abstract method that needs to be implemented, __contains__
. You can issue help(Container.__contains__)
to see what the function signature should look like:
Help on method __contains__ in module _abcoll:__contains__(self, x) unbound _abcoll.Container method
So, we see that __contains__
needs to take a single argument. Unfortunately, the help file doesn't tell us much about what that argument should be, but it's pretty obvious from the name of the ABC and the single method it implements that this argument is the value the user is checking to see if the container holds.
This method is implemented by list
, str
, and dict
to indicate whether or not a given value is in that data structure. However, we can also define a silly container that tells us whether a given value is in the set of odd integers:
class OddContainer: def __contains__(self, x): if not isinstance(x, int) or not x % 2: return False return True
Now, we can instantiate an OddContainer
object and determine that, even though we did not extend Container
, the class is a Container
object:
>>> from collections import Container >>> odd_container = OddContainer() >>> isinstance(odd_container, Container) True >>> issubclass(OddContainer, Container) True
And that is why duck typing is way more awesome than classical polymorphism. We can create is a relationships without the overhead of using inheritance (or worse, multiple inheritance).
The interesting thing about the Container
ABC is that any class that implements it gets to use the in
keyword for free. In fact, in
is just syntax sugar that delegates to the __contains__
method. Any class that has a __contains__
method is a Container
and can therefore be queried by the in
keyword, for example:
>>> 1 in odd_container True >>> 2 in odd_container False >>> 3 in odd_container True >>> "a string" in odd_container False
Creating an abstract base class
As we saw earlier, it's not necessary to have an abstract base class to enable duck typing. However, imagine we were creating a media player with third-party plugins. It is advisable to create an abstract base class in this case to document what API the third-party plugins should provide. The abc
module provides the tools you need to do this, but I'll warn you in advance, this requires some of Python's most arcane concepts:
import abc class MediaLoader(metaclass=abc.ABCMeta): @abc.abstractmethod def play(self): pass @abc.abstractproperty def ext(self): pass @classmethod def __subclasshook__(cls, C): if cls is MediaLoader: attrs = set(dir(C)) if set(cls.__abstractmethods__) <= attrs: return True return NotImplemented
This is a complicated example that includes several Python features that won't be explained until later in this book. It is included here for completeness, but you don't need to understand all of it to get the gist of how to create your own ABC.
The first weird thing is the metaclass
keyword argument that is passed into the class where you would normally see the list of parent classes. This is a rarely used construct from the mystic art of metaclass programming. We won't be covering metaclasses in this book, so all you need to know is that by assigning the ABCMeta
metaclass, you are giving your class superpower (or at least superclass) abilities.
Next, we see the @abc.abstractmethod
and @abc.abstractproperty
constructs. These are Python decorators. We'll discuss those in Chapter 5, When to Use Object-oriented Programming. For now, just know that by marking a method or property as being abstract, you are stating that any subclass of this class must implement that method or supply that property in order to be considered a proper member of the class.
See what happens if you implement subclasses that do or don't supply those properties:
>>> class Wav(MediaLoader): ... pass ... >>> x = Wav() Traceback (most recent call last): File "<stdin>", line 1, in <module> TypeError: Can't instantiate abstract class Wav with abstract methods ext, play >>> class Ogg(MediaLoader): ... ext = '.ogg' ... def play(self): ... pass ... >>> o = Ogg()
Since the Wav
class fails to implement the abstract attributes, it is not possible to instantiate that class. The class is still a legal abstract class, but you'd have to subclass it to actually do anything. The Ogg
class supplies both attributes, so it instantiates cleanly.
Going back to the MediaLoader
ABC, let's dissect that __subclasshook__
method. It is basically saying that any class that supplies concrete implementations of all the abstract attributes of this ABC should be considered a subclass of MediaLoader
, even if it doesn't actually inherit from the MediaLoader
class.
More common object-oriented languages have a clear separation between the interface and the implementation of a class. For example, some languages provide an explicit interface
keyword that allows us to define the methods that a class must have without any implementation. In such an environment, an abstract class is one that provides both an interface and a concrete implementation of some but not all methods. Any class can explicitly state that it implements a given interface.
Python's ABCs help to supply the functionality of interfaces without compromising on the benefits of duck typing.
Demystifying the magic
You can copy and paste the subclass code without understanding it if you want to make abstract classes that fulfill this particular contract. We'll cover most of the unusual syntaxes throughout the book, but let's go over it line by line to get an overview.
@classmethod
This decorator marks the method as a class method. It essentially says that the method can be called on a class instead of an instantiated object:
def __subclasshook__(cls, C):
This defines the __subclasshook__
class method. This special method is called by the Python interpreter to answer the question, Is the class
C
a subclass of this class?
if cls is MediaLoader:
We check to see if the method was called specifically on this class, rather than, say a subclass of this class. This prevents, for example, the Wav
class from being thought of as a parent class of the Ogg
class:
attrs = set(dir(C))
All this line does is get the set of methods and properties that the class has, including any parent classes in its class hierarchy:
if set(cls.__abstractmethods__) <= attrs:
This line uses set notation to see whether the set of abstract methods in this class have been supplied in the candidate class. Note that it doesn't check to see whether the methods have been implemented, just if they are there. Thus, it's possible for a class to be a subclass and yet still be an abstract class itself.
return True
If all the abstract methods have been supplied, then the candidate class is a subclass of this class and we return True
. The method can legally return one of the three values: True
, False
, or NotImplemented
. True
and False
indicate that the class is or is not definitively a subclass of this class:
return NotImplemented
If any of the conditionals have not been met (that is, the class is not MediaLoader
or not all abstract methods have been supplied), then return NotImplemented
. This tells the Python machinery to use the default mechanism (does the candidate class explicitly extend this class?) for subclass detection.
In short, we can now define the Ogg
class as a subclass of the MediaLoader
class without actually extending the MediaLoader
class:
>>> class Ogg(): ... ext = '.ogg' ... def play(self): ... print("this will play an ogg file") ... >>> issubclass(Ogg, MediaLoader) True >>> isinstance(Ogg(), MediaLoader) True
Using an abstract base class
Most of the abstract base classes that exist in the Python Standard Library live in the collections
module. One of the simplest ones is the Container
class. Let's inspect it in the Python interpreter to see what methods this class requires:
>>> from collections import Container >>> Container.__abstractmethods__ frozenset(['__contains__'])
So, the Container
class has exactly one abstract method that needs to be implemented, __contains__
. You can issue help(Container.__contains__)
to see what the function signature should look like:
Help on method __contains__ in module _abcoll:__contains__(self, x) unbound _abcoll.Container method
So, we see that __contains__
needs to take a single argument. Unfortunately, the help file doesn't tell us much about what that argument should be, but it's pretty obvious from the name of the ABC and the single method it implements that this argument is the value the user is checking to see if the container holds.
This method is implemented by list
, str
, and dict
to indicate whether or not a given value is in that data structure. However, we can also define a silly container that tells us whether a given value is in the set of odd integers:
class OddContainer: def __contains__(self, x): if not isinstance(x, int) or not x % 2: return False return True
Now, we can instantiate an OddContainer
object and determine that, even though we did not extend Container
, the class is a Container
object:
>>> from collections import Container >>> odd_container = OddContainer() >>> isinstance(odd_container, Container) True >>> issubclass(OddContainer, Container) True
And that is why duck typing is way more awesome than classical polymorphism. We can create is a relationships without the overhead of using inheritance (or worse, multiple inheritance).
The interesting thing about the Container
ABC is that any class that implements it gets to use the in
keyword for free. In fact, in
is just syntax sugar that delegates to the __contains__
method. Any class that has a __contains__
method is a Container
and can therefore be queried by the in
keyword, for example:
>>> 1 in odd_container True >>> 2 in odd_container False >>> 3 in odd_container True >>> "a string" in odd_container False
Creating an abstract base class
As we saw earlier, it's not necessary to have an abstract base class to enable duck typing. However, imagine we were creating a media player with third-party plugins. It is advisable to create an abstract base class in this case to document what API the third-party plugins should provide. The abc
module provides the tools you need to do this, but I'll warn you in advance, this requires some of Python's most arcane concepts:
import abc class MediaLoader(metaclass=abc.ABCMeta): @abc.abstractmethod def play(self): pass @abc.abstractproperty def ext(self): pass @classmethod def __subclasshook__(cls, C): if cls is MediaLoader: attrs = set(dir(C)) if set(cls.__abstractmethods__) <= attrs: return True return NotImplemented
This is a complicated example that includes several Python features that won't be explained until later in this book. It is included here for completeness, but you don't need to understand all of it to get the gist of how to create your own ABC.
The first weird thing is the metaclass
keyword argument that is passed into the class where you would normally see the list of parent classes. This is a rarely used construct from the mystic art of metaclass programming. We won't be covering metaclasses in this book, so all you need to know is that by assigning the ABCMeta
metaclass, you are giving your class superpower (or at least superclass) abilities.
Next, we see the @abc.abstractmethod
and @abc.abstractproperty
constructs. These are Python decorators. We'll discuss those in Chapter 5, When to Use Object-oriented Programming. For now, just know that by marking a method or property as being abstract, you are stating that any subclass of this class must implement that method or supply that property in order to be considered a proper member of the class.
See what happens if you implement subclasses that do or don't supply those properties:
>>> class Wav(MediaLoader): ... pass ... >>> x = Wav() Traceback (most recent call last): File "<stdin>", line 1, in <module> TypeError: Can't instantiate abstract class Wav with abstract methods ext, play >>> class Ogg(MediaLoader): ... ext = '.ogg' ... def play(self): ... pass ... >>> o = Ogg()
Since the Wav
class fails to implement the abstract attributes, it is not possible to instantiate that class. The class is still a legal abstract class, but you'd have to subclass it to actually do anything. The Ogg
class supplies both attributes, so it instantiates cleanly.
Going back to the MediaLoader
ABC, let's dissect that __subclasshook__
method. It is basically saying that any class that supplies concrete implementations of all the abstract attributes of this ABC should be considered a subclass of MediaLoader
, even if it doesn't actually inherit from the MediaLoader
class.
More common object-oriented languages have a clear separation between the interface and the implementation of a class. For example, some languages provide an explicit interface
keyword that allows us to define the methods that a class must have without any implementation. In such an environment, an abstract class is one that provides both an interface and a concrete implementation of some but not all methods. Any class can explicitly state that it implements a given interface.
Python's ABCs help to supply the functionality of interfaces without compromising on the benefits of duck typing.
Demystifying the magic
You can copy and paste the subclass code without understanding it if you want to make abstract classes that fulfill this particular contract. We'll cover most of the unusual syntaxes throughout the book, but let's go over it line by line to get an overview.
@classmethod
This decorator marks the method as a class method. It essentially says that the method can be called on a class instead of an instantiated object:
def __subclasshook__(cls, C):
This defines the __subclasshook__
class method. This special method is called by the Python interpreter to answer the question, Is the class
C
a subclass of this class?
if cls is MediaLoader:
We check to see if the method was called specifically on this class, rather than, say a subclass of this class. This prevents, for example, the Wav
class from being thought of as a parent class of the Ogg
class:
attrs = set(dir(C))
All this line does is get the set of methods and properties that the class has, including any parent classes in its class hierarchy:
if set(cls.__abstractmethods__) <= attrs:
This line uses set notation to see whether the set of abstract methods in this class have been supplied in the candidate class. Note that it doesn't check to see whether the methods have been implemented, just if they are there. Thus, it's possible for a class to be a subclass and yet still be an abstract class itself.
return True
If all the abstract methods have been supplied, then the candidate class is a subclass of this class and we return True
. The method can legally return one of the three values: True
, False
, or NotImplemented
. True
and False
indicate that the class is or is not definitively a subclass of this class:
return NotImplemented
If any of the conditionals have not been met (that is, the class is not MediaLoader
or not all abstract methods have been supplied), then return NotImplemented
. This tells the Python machinery to use the default mechanism (does the candidate class explicitly extend this class?) for subclass detection.
In short, we can now define the Ogg
class as a subclass of the MediaLoader
class without actually extending the MediaLoader
class:
>>> class Ogg(): ... ext = '.ogg' ... def play(self): ... print("this will play an ogg file") ... >>> issubclass(Ogg, MediaLoader) True >>> isinstance(Ogg(), MediaLoader) True
Creating an abstract base class
As we saw earlier, it's not necessary to have an abstract base class to enable duck typing. However, imagine we were creating a media player with third-party plugins. It is advisable to create an abstract base class in this case to document what API the third-party plugins should provide. The abc
module provides the tools you need to do this, but I'll warn you in advance, this requires some of Python's most arcane concepts:
import abc class MediaLoader(metaclass=abc.ABCMeta): @abc.abstractmethod def play(self): pass @abc.abstractproperty def ext(self): pass @classmethod def __subclasshook__(cls, C): if cls is MediaLoader: attrs = set(dir(C)) if set(cls.__abstractmethods__) <= attrs: return True return NotImplemented
This is a complicated example that includes several Python features that won't be explained until later in this book. It is included here for completeness, but you don't need to understand all of it to get the gist of how to create your own ABC.
The first weird thing is the metaclass
keyword argument that is passed into the class where you would normally see the list of parent classes. This is a rarely used construct from the mystic art of metaclass programming. We won't be covering metaclasses in this book, so all you need to know is that by assigning the ABCMeta
metaclass, you are giving your class superpower (or at least superclass) abilities.
Next, we see the @abc.abstractmethod
and @abc.abstractproperty
constructs. These are Python decorators. We'll discuss those in Chapter 5, When to Use Object-oriented Programming. For now, just know that by marking a method or property as being abstract, you are stating that any subclass of this class must implement that method or supply that property in order to be considered a proper member of the class.
See what happens if you implement subclasses that do or don't supply those properties:
>>> class Wav(MediaLoader): ... pass ... >>> x = Wav() Traceback (most recent call last): File "<stdin>", line 1, in <module> TypeError: Can't instantiate abstract class Wav with abstract methods ext, play >>> class Ogg(MediaLoader): ... ext = '.ogg' ... def play(self): ... pass ... >>> o = Ogg()
Since the Wav
class fails to implement the abstract attributes, it is not possible to instantiate that class. The class is still a legal abstract class, but you'd have to subclass it to actually do anything. The Ogg
class supplies both attributes, so it instantiates cleanly.
Going back to the MediaLoader
ABC, let's dissect that __subclasshook__
method. It is basically saying that any class that supplies concrete implementations of all the abstract attributes of this ABC should be considered a subclass of MediaLoader
, even if it doesn't actually inherit from the MediaLoader
class.
More common object-oriented languages have a clear separation between the interface and the implementation of a class. For example, some languages provide an explicit interface
keyword that allows us to define the methods that a class must have without any implementation. In such an environment, an abstract class is one that provides both an interface and a concrete implementation of some but not all methods. Any class can explicitly state that it implements a given interface.
Python's ABCs help to supply the functionality of interfaces without compromising on the benefits of duck typing.
Demystifying the magic
You can copy and paste the subclass code without understanding it if you want to make abstract classes that fulfill this particular contract. We'll cover most of the unusual syntaxes throughout the book, but let's go over it line by line to get an overview.
@classmethod
This decorator marks the method as a class method. It essentially says that the method can be called on a class instead of an instantiated object:
def __subclasshook__(cls, C):
This defines the __subclasshook__
class method. This special method is called by the Python interpreter to answer the question, Is the class
C
a subclass of this class?
if cls is MediaLoader:
We check to see if the method was called specifically on this class, rather than, say a subclass of this class. This prevents, for example, the Wav
class from being thought of as a parent class of the Ogg
class:
attrs = set(dir(C))
All this line does is get the set of methods and properties that the class has, including any parent classes in its class hierarchy:
if set(cls.__abstractmethods__) <= attrs:
This line uses set notation to see whether the set of abstract methods in this class have been supplied in the candidate class. Note that it doesn't check to see whether the methods have been implemented, just if they are there. Thus, it's possible for a class to be a subclass and yet still be an abstract class itself.
return True
If all the abstract methods have been supplied, then the candidate class is a subclass of this class and we return True
. The method can legally return one of the three values: True
, False
, or NotImplemented
. True
and False
indicate that the class is or is not definitively a subclass of this class:
return NotImplemented
If any of the conditionals have not been met (that is, the class is not MediaLoader
or not all abstract methods have been supplied), then return NotImplemented
. This tells the Python machinery to use the default mechanism (does the candidate class explicitly extend this class?) for subclass detection.
In short, we can now define the Ogg
class as a subclass of the MediaLoader
class without actually extending the MediaLoader
class:
>>> class Ogg(): ... ext = '.ogg' ... def play(self): ... print("this will play an ogg file") ... >>> issubclass(Ogg, MediaLoader) True >>> isinstance(Ogg(), MediaLoader) True
Demystifying the magic
You can copy and paste the subclass code without understanding it if you want to make abstract classes that fulfill this particular contract. We'll cover most of the unusual syntaxes throughout the book, but let's go over it line by line to get an overview.
@classmethod
This decorator marks the method as a class method. It essentially says that the method can be called on a class instead of an instantiated object:
def __subclasshook__(cls, C):
This defines the __subclasshook__
class method. This special method is called by the Python interpreter to answer the question, Is the class
C
a subclass of this class?
if cls is MediaLoader:
We check to see if the method was called specifically on this class, rather than, say a subclass of this class. This prevents, for example, the Wav
class from being thought of as a parent class of the Ogg
class:
attrs = set(dir(C))
All this line does is get the set of methods and properties that the class has, including any parent classes in its class hierarchy:
if set(cls.__abstractmethods__) <= attrs:
This line uses set notation to see whether the set of abstract methods in this class have been supplied in the candidate class. Note that it doesn't check to see whether the methods have been implemented, just if they are there. Thus, it's possible for a class to be a subclass and yet still be an abstract class itself.
return True
If all the abstract methods have been supplied, then the candidate class is a subclass of this class and we return True
. The method can legally return one of the three values: True
, False
, or NotImplemented
. True
and False
indicate that the class is or is not definitively a subclass of this class:
return NotImplemented
If any of the conditionals have not been met (that is, the class is not MediaLoader
or not all abstract methods have been supplied), then return NotImplemented
. This tells the Python machinery to use the default mechanism (does the candidate class explicitly extend this class?) for subclass detection.
In short, we can now define the Ogg
class as a subclass of the MediaLoader
class without actually extending the MediaLoader
class:
>>> class Ogg(): ... ext = '.ogg' ... def play(self): ... print("this will play an ogg file") ... >>> issubclass(Ogg, MediaLoader) True >>> isinstance(Ogg(), MediaLoader) True
Case study
Let's try to tie everything we've learned together with a larger example. We'll be designing a simple real estate application that allows an agent to manage properties available for purchase or rent. There will be two types of properties: apartments and houses. The agent needs to be able to enter a few relevant details about new properties, list all currently available properties, and mark a property as sold or rented. For brevity, we won't worry about editing property details or reactivating a property after it is sold.
The project will allow the agent to interact with the objects using the Python interpreter prompt. In this world of graphical user interfaces and web applications, you might be wondering why we're creating such old-fashioned looking programs. Simply put, both windowed programs and web applications require a lot of overhead knowledge and boilerplate code to make them do what is required. If we were developing software using either of these paradigms, we'd get so lost in GUI programming or web programming that we'd lose sight of the object-oriented principles we're trying to master.
Luckily, most GUI and web frameworks utilize an object-oriented approach, and the principles we're studying now will help in understanding those systems in the future. We'll discuss them both briefly in Chapter 13, Concurrency, but complete details are far beyond the scope of a single book.
Looking at our requirements, it seems like there are quite a few nouns that might represent classes of objects in our system. Clearly, we'll need to represent a property. Houses and apartments may need separate classes. Rentals and purchases also seem to require separate representation. Since we're focusing on inheritance right now, we'll be looking at ways to share behavior using inheritance or multiple inheritance.
House
and Apartment
are both types of properties, so Property
can be a superclass of those two classes. Rental
and Purchase
will need some extra thought; if we use inheritance, we'll need to have separate classes, for example, for HouseRental
and HousePurchase
, and use multiple inheritance to combine them. This feels a little clunky compared to a composition or association-based design, but let's run with it and see what we come up with.
Now then, what attributes might be associated with a Property
class? Regardless of whether it is an apartment or a house, most people will want to know the square footage, number of bedrooms, and number of bathrooms. (There are numerous other attributes that might be modeled, but we'll keep it simple for our prototype.)
If the property is a house, it will want to advertise the number of stories, whether it has a garage (attached, detached, or none), and whether the yard is fenced. An apartment will want to indicate if it has a balcony, and if the laundry is ensuite, coin, or off-site.
Both property types will require a method to display the characteristics of that property. At the moment, no other behaviors are apparent.
Rental properties will need to store the rent per month, whether the property is furnished, and whether utilities are included, and if not, what they are estimated to be. Properties for purchase will need to store the purchase price and estimated annual property taxes. For our application, we'll only need to display this data, so we can get away with just adding a display()
method similar to that used in the other classes.
Finally, we'll need an Agent
object that holds a list of all properties, displays those properties, and allows us to create new ones. Creating properties will entail prompting the user for the relevant details for each property type. This could be done in the Agent
object, but then Agent
would need to know a lot of information about the types of properties. This is not taking advantage of polymorphism. Another alternative would be to put the prompts in the initializer or even a constructor for each class, but this would not allow the classes to be applied in a GUI or web application in the future. A better idea is to create a static method that does the prompting and returns a dictionary of the prompted parameters. Then, all the Agent
has to do is prompt the user for the type of property and payment method, and ask the correct class to instantiate itself.
That's a lot of designing! The following class diagram may communicate our design decisions a little more clearly:
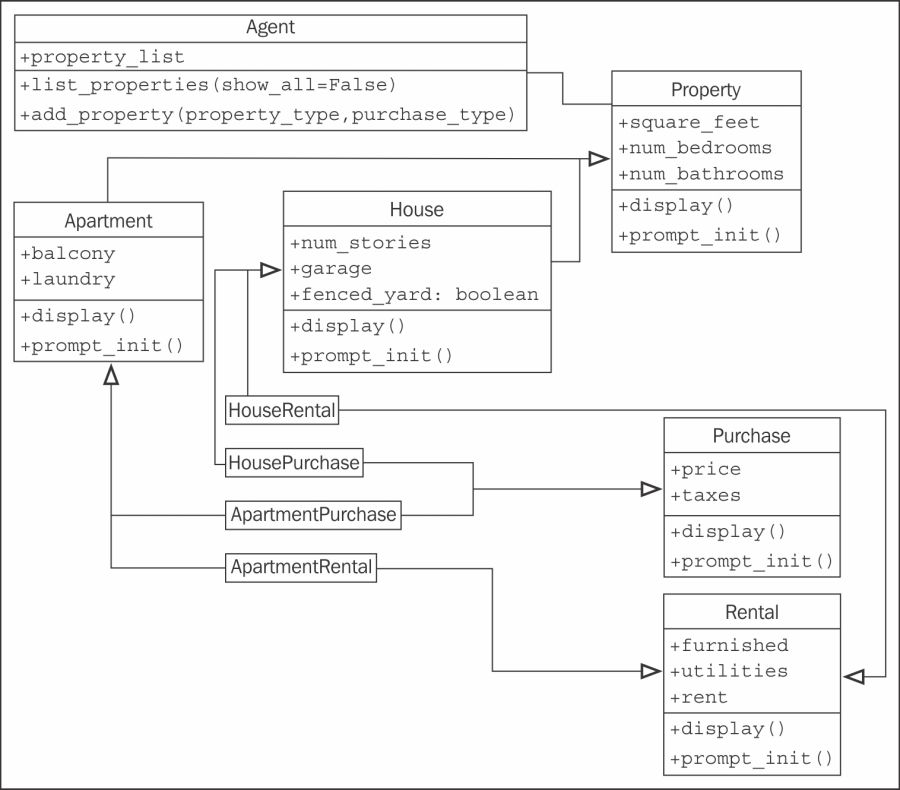
Wow, that's a lot of inheritance arrows! I don't think it would be possible to add another level of inheritance without crossing arrows. Multiple inheritance is a messy business, even at the design stage.
The trickiest aspects of these classes is going to be ensuring superclass methods get called in the inheritance hierarchy. Let's start with the Property
implementation:
class Property: def __init__(self, square_feet='', beds='', baths='', **kwargs): super().__init__(**kwargs) self.square_feet = square_feet self.num_bedrooms = beds self.num_baths = baths def display(self): print("PROPERTY DETAILS") print("================") print("square footage: {}".format(self.square_feet)) print("bedrooms: {}".format(self.num_bedrooms)) print("bathrooms: {}".format(self.num_baths)) print() def prompt_init(): return dict(square_feet=input("Enter the square feet: "), beds=input("Enter number of bedrooms: "), baths=input("Enter number of baths: ")) prompt_init = staticmethod(prompt_init)
This class is pretty straightforward. We've already added the extra **kwargs
parameter to __init__
because we know it's going to be used in a multiple inheritance situation. We've also included a call to super().__init__
in case we are not the last call in the multiple inheritance chain. In this case, we're consuming the keyword arguments because we know they won't be needed at other levels of the inheritance hierarchy.
We see something new in the prompt_init
method. This method is made into a static method immediately after it is initially created. Static methods are associated only with a class (something like class variables), rather than a specific object instance. Hence, they have no self
argument. Because of this, the super
keyword won't work (there is no parent object, only a parent class), so we simply call the static method on the parent class directly. This method uses the Python dict
constructor to create a dictionary of values that can be passed into __init__
. The value for each key is prompted with a call to input
.
The Apartment
class extends Property
, and is similar in structure:
class Apartment(Property): valid_laundries = ("coin", "ensuite", "none") valid_balconies = ("yes", "no", "solarium") def __init__(self, balcony='', laundry='', **kwargs): super().__init__(**kwargs) self.balcony = balcony self.laundry = laundry def display(self): super().display() print("APARTMENT DETAILS") print("laundry: %s" % self.laundry) print("has balcony: %s" % self.balcony) def prompt_init(): parent_init = Property.prompt_init() laundry = '' while laundry.lower() not in \ Apartment.valid_laundries: laundry = input("What laundry facilities does " "the property have? ({})".format( ", ".join(Apartment.valid_laundries))) balcony = '' while balcony.lower() not in \ Apartment.valid_balconies: balcony = input( "Does the property have a balcony? " "({})".format( ", ".join(Apartment.valid_balconies))) parent_init.update({ "laundry": laundry, "balcony": balcony }) return parent_init prompt_init = staticmethod(prompt_init)
The display()
and __init__()
methods call their respective parent class methods using super()
to ensure the Property
class is properly initialized.
The prompt_init
static method is now getting dictionary values from the parent class, and then adding some additional values of its own. It calls the dict.update
method to merge the new dictionary values into the first one. However, that prompt_init
method is looking pretty ugly; it loops twice until the user enters a valid input using structurally similar code but different variables. It would be nice to extract this validation logic so we can maintain it in only one location; it will likely also be useful to later classes.
With all the talk on inheritance, we might think this is a good place to use a mixin. Instead, we have a chance to study a situation where inheritance is not the best solution. The method we want to create will be used in a static method. If we were to inherit from a class that provided validation functionality, the functionality would also have to be provided as a static method that did not access any instance variables on the class. If it doesn't access any instance variables, what's the point of making it a class at all? Why don't we just make this validation functionality a module-level function that accepts an input string and a list of valid answers, and leave it at that?
Let's explore what this validation function would look like:
def get_valid_input(input_string, valid_options): input_string += " ({}) ".format(", ".join(valid_options)) response = input(input_string) while response.lower() not in valid_options: response = input(input_string) return response
We can test this function in the interpreter, independent of all the other classes we've been working on. This is a good sign, it means different pieces of our design are not tightly coupled to each other and can later be improved independently, without affecting other pieces of code.
>>> get_valid_input("what laundry?", ("coin", "ensuite", "none")) what laundry? (coin, ensuite, none) hi what laundry? (coin, ensuite, none) COIN 'COIN'
Now, let's quickly update our Apartment.prompt_init
method to use this new function for validation:
def prompt_init(): parent_init = Property.prompt_init() laundry = get_valid_input( "What laundry facilities does " "the property have? ", Apartment.valid_laundries) balcony = get_valid_input( "Does the property have a balcony? ", Apartment.valid_balconies) parent_init.update({ "laundry": laundry, "balcony": balcony }) return parent_init prompt_init = staticmethod(prompt_init)
That's much easier to read (and maintain!) than our original version. Now we're ready to build the House
class. This class has a parallel structure to Apartment
, but refers to different prompts and variables:
class House(Property): valid_garage = ("attached", "detached", "none") valid_fenced = ("yes", "no") def __init__(self, num_stories='', garage='', fenced='', **kwargs): super().__init__(**kwargs) self.garage = garage self.fenced = fenced self.num_stories = num_stories def display(self): super().display() print("HOUSE DETAILS") print("# of stories: {}".format(self.num_stories)) print("garage: {}".format(self.garage)) print("fenced yard: {}".format(self.fenced)) def prompt_init(): parent_init = Property.prompt_init() fenced = get_valid_input("Is the yard fenced? ", House.valid_fenced) garage = get_valid_input("Is there a garage? ", House.valid_garage) num_stories = input("How many stories? ") parent_init.update({ "fenced": fenced, "garage": garage, "num_stories": num_stories }) return parent_init prompt_init = staticmethod(prompt_init)
There's nothing new to explore here, so let's move on to the Purchase
and Rental
classes. In spite of having apparently different purposes, they are also similar in design to the ones we just discussed:
class Purchase: def __init__(self, price='', taxes='', **kwargs): super().__init__(**kwargs) self.price = price self.taxes = taxes def display(self): super().display() print("PURCHASE DETAILS") print("selling price: {}".format(self.price)) print("estimated taxes: {}".format(self.taxes)) def prompt_init(): return dict( price=input("What is the selling price? "), taxes=input("What are the estimated taxes? ")) prompt_init = staticmethod(prompt_init) class Rental: def __init__(self, furnished='', utilities='', rent='', **kwargs): super().__init__(**kwargs) self.furnished = furnished self.rent = rent self.utilities = utilities def display(self): super().display() print("RENTAL DETAILS") print("rent: {}".format(self.rent)) print("estimated utilities: {}".format( self.utilities)) print("furnished: {}".format(self.furnished)) def prompt_init(): return dict( rent=input("What is the monthly rent? "), utilities=input( "What are the estimated utilities? "), furnished = get_valid_input( "Is the property furnished? ", ("yes", "no"))) prompt_init = staticmethod(prompt_init)
These two classes don't have a superclass (other than object
), but we still call super().__init__
because they are going to be combined with the other classes, and we don't know what order the super
calls will be made in. The interface is similar to that used for House
and Apartment
, which is very useful when we combine the functionality of these four classes in separate subclasses. For example:
class HouseRental(Rental, House): def prompt_init(): init = House.prompt_init() init.update(Rental.prompt_init()) return init prompt_init = staticmethod(prompt_init)
This is slightly surprising, as the class on its own has neither an __init__
nor display
method! Because both parent classes appropriately call super
in these methods, we only have to extend those classes and the classes will behave in the correct order. This is not the case with prompt_init
, of course, since it is a static method that does not call super
, so we implement this one explicitly. We should test this class to make sure it is behaving properly before we write the other three combinations:
>>> init = HouseRental.prompt_init() Enter the square feet: 1 Enter number of bedrooms: 2 Enter number of baths: 3 Is the yard fenced? (yes, no) no Is there a garage? (attached, detached, none) none How many stories? 4 What is the monthly rent? 5 What are the estimated utilities? 6 Is the property furnished? (yes, no) no >>> house = HouseRental(**init) >>> house.display() PROPERTY DETAILS ================ square footage: 1 bedrooms: 2 bathrooms: 3 HOUSE DETAILS # of stories: 4 garage: none fenced yard: no RENTAL DETAILS rent: 5 estimated utilities: 6 furnished: no
It looks like it is working fine. The prompt_init
method is prompting for initializers to all the super classes, and display()
is also cooperatively calling all three superclasses.
Note
The order of the inherited classes in the preceding example is important. If we had written class HouseRental(House, Rental)
instead of class HouseRental(Rental, House)
, display()
would not have called Rental.display()
! When display
is called on our version of HouseRental
, it refers to the Rental
version of the method, which calls super.display()
to get the House
version, which again calls super.display()
to get the property version. If we reversed it, display
would refer to the House
class's display()
. When super is called, it calls the method on the Property
parent class. But Property
does not have a call to super
in its display
method. This means Rental
class's display
method would not be called! By placing the inheritance list in the order we did, we ensure that Rental
calls super
, which then takes care of the House
side of the hierarchy. You might think we could have added a super
call to Property.display()
, but that will fail because the next superclass of Property
is object
, and object
does not have a display
method. Another way to fix this is to allow Rental
and Purchase
to extend the Property
class instead of deriving directly from object
. (Or we could modify the method resolution order dynamically, but that is beyond the scope of this book.)
Now that we have tested it, we are prepared to create the rest of our combined subclasses:
class ApartmentRental(Rental, Apartment): def prompt_init(): init = Apartment.prompt_init() init.update(Rental.prompt_init()) return init prompt_init = staticmethod(prompt_init) class ApartmentPurchase(Purchase, Apartment): def prompt_init(): init = Apartment.prompt_init() init.update(Purchase.prompt_init()) return init prompt_init = staticmethod(prompt_init) class HousePurchase(Purchase, House): def prompt_init(): init = House.prompt_init() init.update(Purchase.prompt_init()) return init prompt_init = staticmethod(prompt_init)
That should be the most intense designing out of our way! Now all we have to do is create the Agent
class, which is responsible for creating new listings and displaying existing ones. Let's start with the simpler storing and listing of properties:
class Agent: def __init__(self): self.property_list = [] def display_properties(self): for property in self.property_list: property.display()
Adding a property will require first querying the type of property and whether property is for purchase or rental. We can do this by displaying a simple menu. Once this has been determined, we can extract the correct subclass and prompt for all the details using the prompt_init
hierarchy we've already developed. Sounds simple? It is. Let's start by adding a dictionary class variable to the Agent
class:
type_map = { ("house", "rental"): HouseRental, ("house", "purchase"): HousePurchase, ("apartment", "rental"): ApartmentRental, ("apartment", "purchase"): ApartmentPurchase }
That's some pretty funny looking code. This is a dictionary, where the keys are tuples of two distinct strings, and the values are class objects. Class objects? Yes, classes can be passed around, renamed, and stored in containers just like normal objects or primitive data types. With this simple dictionary, we can simply hijack our earlier get_valid_input
method to ensure we get the correct dictionary keys and look up the appropriate class, like this:
def add_property(self): property_type = get_valid_input( "What type of property? ", ("house", "apartment")).lower() payment_type = get_valid_input( "What payment type? ", ("purchase", "rental")).lower() PropertyClass = self.type_map[ (property_type, payment_type)] init_args = PropertyClass.prompt_init() self.property_list.append(PropertyClass(**init_args))
This may look a bit funny too! We look up the class in the dictionary and store it in a variable named PropertyClass
. We don't know exactly which class is available, but the class knows itself, so we can polymorphically call prompt_init
to get a dictionary of values appropriate to pass into the constructor. Then we use the keyword argument syntax to convert the dictionary into arguments and construct the new object to load the correct data.
Now our user can use this Agent
class to add and view lists of properties. It wouldn't take much work to add features to mark a property as available or unavailable or to edit and remove properties. Our prototype is now in a good enough state to take to a real estate agent
and demonstrate its functionality. Here's how a demo session might work:
>>> agent = Agent() >>> agent.add_property() What type of property? (house, apartment) house What payment type? (purchase, rental) rental Enter the square feet: 900 Enter number of bedrooms: 2 Enter number of baths: one and a half Is the yard fenced? (yes, no) yes Is there a garage? (attached, detached, none) detached How many stories? 1 What is the monthly rent? 1200 What are the estimated utilities? included Is the property furnished? (yes, no) no >>> agent.add_property() What type of property? (house, apartment) apartment What payment type? (purchase, rental) purchase Enter the square feet: 800 Enter number of bedrooms: 3 Enter number of baths: 2 What laundry facilities does the property have? (coin, ensuite, one) ensuite Does the property have a balcony? (yes, no, solarium) yes What is the selling price? $200,000 What are the estimated taxes? 1500 >>> agent.display_properties() PROPERTY DETAILS ================ square footage: 900 bedrooms: 2 bathrooms: one and a half HOUSE DETAILS # of stories: 1 garage: detached fenced yard: yes RENTAL DETAILS rent: 1200 estimated utilities: included furnished: no PROPERTY DETAILS ================ square footage: 800 bedrooms: 3 bathrooms: 2 APARTMENT DETAILS laundry: ensuite has balcony: yes PURCHASE DETAILS selling price: $200,000 estimated taxes: 1500
Exercises
Look around you at some of the physical objects in your workspace and see if you can describe them in an inheritance hierarchy. Humans have been dividing the world into taxonomies like this for centuries, so it shouldn't be difficult. Are there any non-obvious inheritance relationships between classes of objects? If you were to model these objects in a computer application, what properties and methods would they share? Which ones would have to be polymorphically overridden? What properties would be completely different between them?
Now, write some code. No, not for the physical hierarchy; that's boring. Physical items have more properties than methods. Just think about a pet programming project you've wanted to tackle in the past year, but never got around to. For whatever problem you want to solve, try to think of some basic inheritance relationships. Then implement them. Make sure that you also pay attention to the sorts of relationships that you actually don't need to use inheritance for. Are there any places where you might want to use multiple inheritance? Are you sure? Can you see any place you would want to use a mixin? Try to knock together a quick prototype. It doesn't have to be useful or even partially working. You've seen how you can test code using python -i
already; just write some code and test it in the interactive interpreter. If it works, write some more. If it doesn't, fix it!
Now, take a look at the real estate example. This turned out to be quite an effective use of multiple inheritance. I have to admit though, I had my doubts when I started the design. Have a look at the original problem and see if you can come up with another design to solve it that uses only single inheritance. How would you do it with abstract base classes? What about a design that doesn't use inheritance at all? Which do you think is the most elegant solution? Elegance is a primary goal in Python development, but each programmer has a different opinion as to what is the most elegant solution. Some people tend to think and understand problems most clearly using composition, while others find multiple inheritance to be the most useful model.
Finally, try adding some new features to the three designs. Whatever features strike your fancy are fine. I'd like to see a way to differentiate between available and unavailable properties, for starters. It's not of much use to me if it's already rented!
Which design is easiest to extend? Which is hardest? If somebody asked you why you thought this, would you be able to explain yourself?
Summary
We've gone from simple inheritance, one of the most useful tools in the object-oriented programmer's toolbox, all the way through to multiple inheritance, one of the most complicated. Inheritance can be used to add functionality to existing classes and built-ins using inheritance. Abstracting similar code into a parent class can help increase maintainability. Methods on parent classes can be called using super
and argument lists must be formatted safely for these calls to work when using multiple inheritance.
In the next chapter, we'll cover the subtle art of handling exceptional circumstances.