Mozilla is a company known for its mission to develop tools for and drive the open standards web, most notably through its flagship browser Firefox. Every browser today, including Firefox, is written in C++, some 1,29,00,992 lines of code for Firefox, and 44,90,488 lines of code for Chrome. This makes them fast, but it is inherently unsafe because the memory manipulations allowed by C and C++ are not checked for validity. If the code is written without the utmost programming discipline on the part of the developers, program crashes, memory leaks, segmentation faults, buffer overflows, and null pointers can occur at program execution. Some of these can result in serious security vulnerabilities, all too familiar in existing browsers. Rust is designed from the ground up to avoid those kind of problems.
Compared to C or C++, on the other side of the programming language spectrum we have Haskell, which is widely known to be a very safe and reliable language, but with very little or no control at the level of memory allocation and other hardware resources. We can plot different languages along this control that is safety axis, and it seems that when a language is safer, like Java compared to C++, it loses low-level control. The inverse is also true; a language that gives more control over resources like C++ provides much less safety.
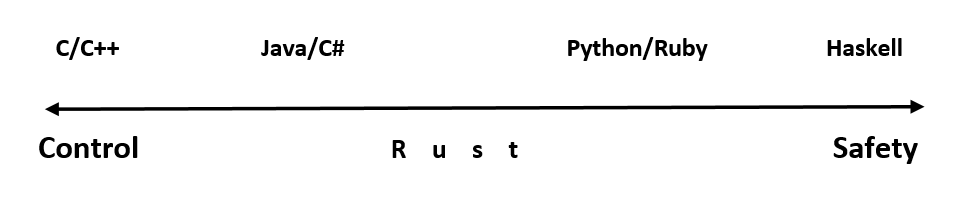
Rust is made to overcome this dilemma by providing:
- High safety through its strong type system and smart compiler
- Deep but safe control over low-level resources (as much as C or C++), so it runs close to the hardware
Its main website, http://www.rust-lang.org/en-US/, contains links to installation instructions, docs and the Rust community.
Rust lets you specify exactly how your values are laid out in memory and how that memory is managed; that's why it works well at both ends of the control and safety line. This is the unique selling point of Rust, it breaks the safety-control dichotomy that, before Rust, existed among programming languages. With Rust they can be achieved together without losing performance.
Rust can accomplish both these goals without a garbage collector, in contrast to most modern languages like Java, C#, Python, Ruby, Go, and the like. In fact Rust doesn't even have a garbage collector yet (though an optional garbage collector is being designed).
Rust is a compiled language: the strict safety rules are enforced by the compiler, so they do not cause runtime overhead. As a consequence, Rust can work with a very small runtime, so it can be used for real-time or embedded projects and it can easily integrate with other languages or projects.
Rust is meant for developers and projects where performance and low-level optimizations are important, but also where a safe and stable execution environment is needed. The robustness of the language is specifically suited for projects where that is important, leading to less pressure in the maintenance cycle. Moreover, Rust adds a lot of high-level functional programming techniques to the language, so that it feels at the same time like a low-level and a high-level language.