Introduction
In the previous chapter, you learned the basics of the Python programming language and essential elements such as string
and int
, as well as how to use conditionals and loops to control the flow of a Python program. By utilizing these elements, you should now be familiar with writing basic programs in Python.
In this chapter, you are going to learn how to use data structures to store more complex types of data that help model actual data and represent it in the real world.
In programming languages, data structures refer to objects that can hold some data together, which means they are used to store a collection of related data.
For instance, you can use a list to store our to-do items for the day. The following is an example that shows how lists are coded:
todo = ["pick up laundry", "buy Groceries", "pay electric
bills"]
We can also use a dictionary object to store more complex information such as subscribers’ details from our mailing list. Here is an example code snippet, but don’t worry, we will cover this later in this chapter:
User = {
"first_name": "Jack",
"last_name":"White",
"age": 41,
"email": "[email protected]"
}
Here is a tuple of a point in the x-y plane, another data type that will be covered later:
point = (1,2)
And here is a set of points, whose details will come at the end of this chapter:
my_set = {3, 5, 11, 17, 31}
There are four types of data structures in Python: list
, tuple
, dictionary
, and set
:
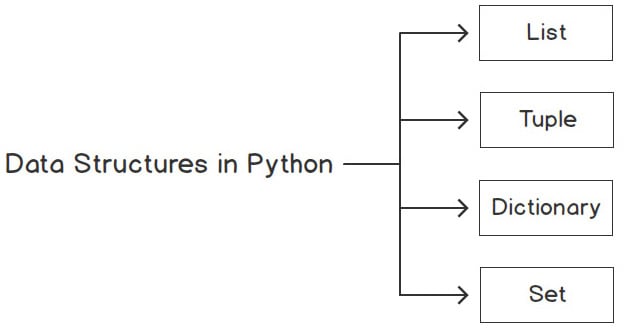
Figure 2.1 – The different data structures in Python
These data structures define the relationship between data and the operations that can be performed on data. They are a way of organizing and storing data that can be accessed efficiently under different circumstances.
In this chapter, we will cover the following topics:
- The power of lists
- List methods
- Matrix operations
- Dictionary keys and values
- Dictionary methods
- Tuples
- A survey of sets
- Choosing types
Let’s start!