The emergence of JavaScript frameworks
As JavaScript progressed and evolved, the innovators who were heavily involved in the language, both companies and individuals, started writing software libraries to help solve the everyday burdens of web application architectures. The initial focus of the most basic JavaScript libraries was to provide singular features, interactivity, and add-ons, which progressively enhanced the web page. At that time, JavaScript gave life to a static page with its interactive components – simple examples that always come to mind are tiny scripts, which enabled creative button effects and mouse cursor effects. In many cases, these scripts were separate from the site’s core functionality and were not essential to allow users to interact with the content. Since the inception of small libraries, these have paved the way for the complex framework systems that we have today. The frontend technology quickly evolved, and now, developers are much more accustomed to megabytes of scripts powering the frontend code.
JavaScript libraries were the next step in the web development evolution, assisting with cross-browser quirks, complex visual effects, network requests, and web page layout. With the use of these libraries, developers were able to have the cross-browser development challenges under control. CSS started to catch up with its focus on layout features and cross-browser standards, improving the web’s styling features. Developers finally began introducing structure and well-crafted systems into web development.
The time has come to finally focus on building scalable and opinionated software on the web, and this is where we began to see glimpses of complex software paradigms introduced into large websites and web applications. Companies and larger enterprises started treating the web as a serious application platform, which led to a few prominent projects written in JavaScript and compiled to JavaScript from languages such as Java. Tracing back to late 2009, we see the first iterations of Model-View-Controller (MVC) frameworks built entirely with HTML, CSS, and JavaScript. This MVC model allows more extensive projects to stay organized, enriches the development workflow, and opens up the world of frontend development to developers who expect a much more structured approach when writing software. The MVC model fit web applications well enough to spawn a renaissance in framework development.
Many development hours were invested into connecting the mechanisms between the JavaScript engines and the browser web APIs. In Figure 1.1, we see a simplified view of how this interaction happens:
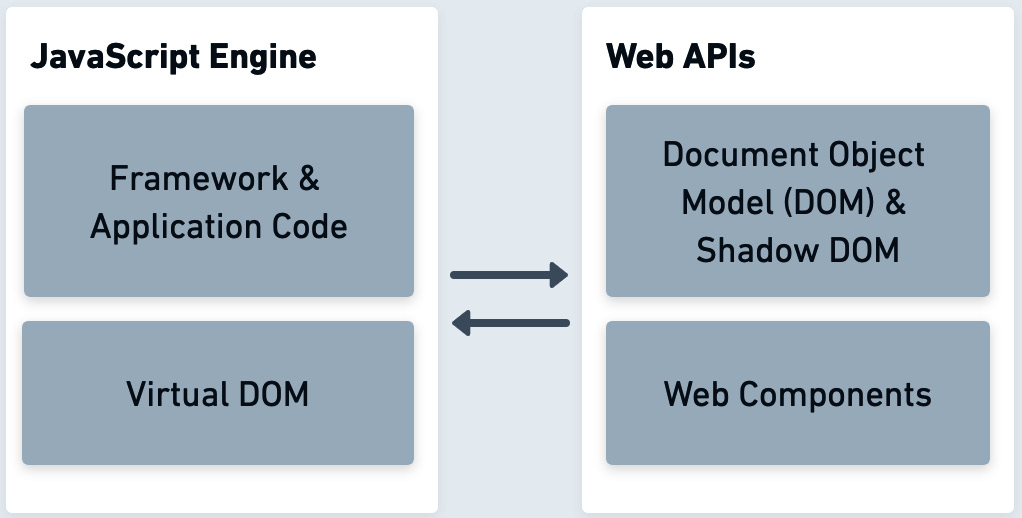
Figure 1.1: Interaction between the JavaScript engine and web APIs
The framework code and its internal technologies, such as the virtual DOM, use the DOM and its components to make the web application experience possible. The web has its own approach to the MVC architecture, with DOM and web API events interacting with Controllers defined in JavaScript. The Controllers interface with Views written in HTML or templated HTML. Furthermore, in this paradigm, the components of the applications utilize a Model to model the data within them. Using this approach, we can later communicate with backend services to retrieve the data in a particular manner.
Each new JavaScript MVC framework tried to perfect its implementation or approach in various ways. About five years after the first MVC frameworks appeared and gained popularity, several new paradigms that focused on the observer software design pattern started gaining traction in the JavaScript community. This observer approach is a software design pattern in which an object maintains a list of its dependants, called observers. The object notifies the observers automatically of the state changes within it. At that time, Flux came about, which is an application architecture that concentrates on simplifying the hurdles faced within MVC. The burdens included dealing with views constantly needing to interact with the models, hard-to-debug, deeply nested logic, and the need for adequate testing solutions of complex applications.
In the observer pattern, we define subjects that include a collection of observers informing about state changes. The Flux architecture expanded this existing pattern to fit better with applications built on the web. In the case of the Flux pattern, it consists of stores interacting with the state of a component. These stores get notified by a dispatcher based on data coming from actions that a user in the view took. Many JavaScript frameworks started adapting this pattern, ultimately simplifying how engineers structured their applications while still enforcing a set of applicable rules to keep the separation of concerns intact. The software patterns in all of these frameworks provide a clear separation of concerns between the interface, data model, and the application logic that integrates them. The Flux-based JavaScript frameworks introduced new concepts that emerged from the known MVC patterns. Still, both the MVC and Flux approaches focused on the principle of the separation of concerns in application development.
Furthermore, while simplifying ideas brought to light by Flux, a library called Redux inspired the next generation of frameworks to switch their approach to application state management. Instead of the Flux dispatchers, Redux frameworks rely on a single store with pure reducer functions, taking the current state and returning an updated state. Even today, frontend patterns are still maturing, and building for the web platform is becoming increasingly easier.
While there’s a lot to mention with regard to frontend technologies, JavaScript has also made a great impact in places outside of web browsers. We cover those areas in the next section.