The evolution of code bases
While learning about frameworks, it is fascinating to reflect on how building for the web has changed over time. This exploration helps us understand why we build web applications the way we do today and helps us learn from historical shifts. It also allows us to be more mindful concerning framework usability and development decisions when we take on large projects. As technology pushes forward, the requirements and expectations around how websites and web applications are built drastically change. Depending on how long someone has been involved in web development, they either experienced many rapidly evolving shifts to how the code bases are structured or were lucky enough to avoid the times when the tooling and the process were highly tedious.
Initially, the code bases comprised isolated frontend components stitched together, consisting of code repetition and mixes of coding patterns. Code organization, usage of software development patterns, and performance optimizations were not a primary focus for developers. The web application deployment process used to be rudimentary as well. In many cases, the websites were manually updated and did not use source control or version tracking. Testing was also highly manual and would only exist in a few projects that were large enough to enable it. This was before deployment pipelines with continuous integration, deployment automation, and advanced testing infrastructure, rigorously verified every change. There used to be a time when developers had to optimize their CSS selectors for performance reasons.
Luckily, productivity and workflows rapidly started to improve once the industry started focusing more on building complex applications. Today we have source control, we have a myriad of testing and deployment tools to choose from, and we have established software paradigms that considerably improve our lives as developers and vastly improve the quality of the projects we build. Improvements to JavaScript engines unlocked new pathways for frameworks, and enhancements to web browsers fixed slow DOM interactivity with techniques such as the virtual DOM, Shadow DOM, and Web Components. These days, frontend frameworks have a better client platform to target as well, and the more established and improved web standards make it possible to perform much more complex operations. For example, with the help of WebAssembly (webassembly.org) standards, we can now run low-level code with improved performance, all within the browser.
As part of all these developments and growth in popularity, the web application development workflow got a lot more complex in many ways. Almost at every point of interaction with a web application project, there is a tooling set designed to improve our workflow. Some examples of this would be Git source control, various pre- and post-processors of our files, code editors with plugins, browser extensions, and many more. Here we have an example that illustrates the key components of a modern web application code base structure, in this case, generated by SvelteKit:
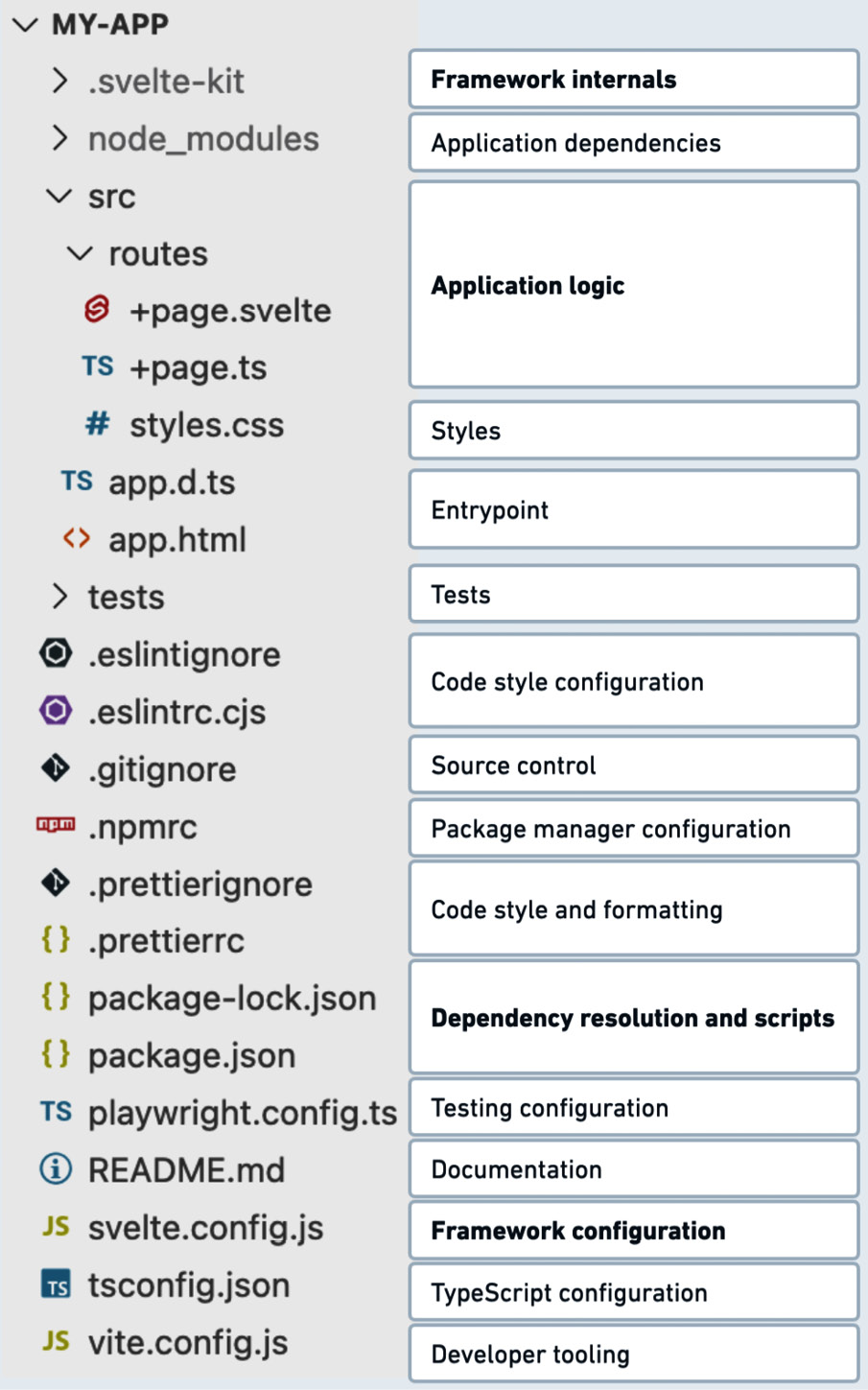
Figure 1.2: SvelteKit code base structure
We will go over SvelteKit later in the Frameworks that use React section of this chapter, and even if you have never used Svelte, this project file tree will look very familiar if you work with other frameworks. This dynamic structure of tools enables flexibility when it comes to switching out certain functionality. For example, Prettier can be substituted for another code formatting tool if need be, while the rest of the project structure remains the same and functions as it was.
With the establishment of the first frameworks in JavaScript, we experienced the introduction of a build step into our projects, which meant that either external or bundled tooling would help run or build the application. Today, this build step, popularized by Webpack or esbuild, is almost impossible to avoid. As part of this build step, we fetch application dependencies using package managers, process CSS, create code bundles, and run various optimization steps to make our app run fast and consume the least bandwidth. The ecosystem also introduced JavaScript transpilers, which are a type of source-to-source code compiler. They are used to take one particular syntax, which could consist of more modern features or include additional features, and convert them to be compatible with broadly accepted JavaScript syntax. Transpilers, such as Babel, began to see everyday use, integrated with the build step in many projects; this pattern generally motivated the use of the latest language features while also supporting old browser engines. These days, transpilation and build steps apply to files beyond JavaScript, as well as files such as CSS and specific templating formats.
Integrating with the build step are the package managers, such as npm
or yarn
, which play an essential role in resolving project dependencies. If you want to bootstrap a workflow with a framework, you will likely rely on the package manager to initialize the framework structure and its dependencies. For new projects, it is almost impossible to have a sensible framework workflow without using a package manager or some form of dependency resolution. As the project grows, the package manager facilitates the organization of newer dependencies while keeping track of updates to modules that are already in use. These days text editors, such as Visual Studio Code and IntelliJ WebStorm, adapt to our code bases and provide excellent tooling to enable source control of our code. The editors rely on built-in features and external plugins that encourage better formatting, easier debugging, and framework-specific improvements.
The code bases will keep changing as technology develops further, and the tools will keep improving to enable us to develop applications more quickly. Regarding the framework organization, we can expect higher levels of abstractions that simplify the way we do web development. Many programming languages, such as Java and Swift, have pre-defined development workflows encapsulating all aspects of development. JavaScript code bases so far have been an exception to these rules and allowed for high levels of flexibility. This trend is going to continue for many more years as the rapid pace of tooling and innovation in web development is not slowing down at all.
Now that we understand how the JavaScript ecosystem has evolved and how codebases have changed over time, let us explore what JavaScript frameworks offer in terms of frontend, backend, testing, and beyond.