Leading a code review
When leading code reviews, it is important to have the right people present. The people who will attend the peer code review will be agreed upon with the project manager. The programmer(s) responsible for submitting the code for review will be present at the code review unless they work remotely. In the case of remote working, the reviewer will review the code and either accept the pull request, decline the pull request, or send the developer some questions to be answered before taking any further action.
A suitable lead for a code review should possess the following skills and knowledge:
- Be a technical authority: The person leading the code review should be a technical authority who understands the company’s coding guidelines and software development methodologies. It is also important that they have a good overall understanding of the software under review. The person doing the code review should also be a master in the technology in which the code is written.
- Have good soft skills: As the leader of the code review, the person must be a warm and encouraging individual who can provide constructive feedback and not be overly critical. The person reviewing the programmer’s code must have good soft skills so that there is no conflict between the reviewer and the person whose code is being reviewed.
In my experience, peer code reviews are always carried out on pull requests in the source control tool being used by the team. A programmer will submit the code to source control and then issue a pull request. The peer code reviewer will then review the code in the pull request. Constructive feedback will be provided in the form of comments that will be attached to the pull request. If there are problems with the pull request, then the reviewer will reject the change request and comment on specific issues that need to be addressed by the programmer. If the code review is successful, then the reviewer may add a comment providing positive feedback, merge the pull request, and close it.
Programmers will need to note any comments made by the reviewer and take them on board. If the code needs to be resubmitted, then the programmer will need to ensure that all the reviewer’s comments have been addressed before resubmitting.
It is a good idea to keep code reviews short and not review too many lines at any one time.
Since a code review normally starts with a pull request, we will look at issuing a pull request, followed by responding to a pull request.
Issuing a pull request
In source control, a pull request is a mechanism for submitting proposed changes to the code base to the main branch or repository. It is a request to merge changes made in one branch of a repository into another branch, usually the main branch.
The process typically involves a developer creating a new branch from the main branch, making changes to the code in the new branch, and then submitting a pull request to merge the changes into the main branch. The pull request includes information about the changes made, the reasons for making them, and any related issues or tickets.
Once the pull request has been submitted, other developers can review the proposed changes, suggest modifications, or approve the changes for merging into the main branch. The code changes are typically reviewed for quality, compatibility, and compliance with any coding standards or best practices before they are merged into the main branch.
To issue a pull request, all you must do (once you’ve checked your code in or pushed it) is click on the Pull requests tab of your source control. There will then be a button you can click on – New pull request. This will add your pull request to a queue, where it will be picked up by the relevant reviewers.
Information
We will be focusing on using GitHub for version control. If you have never used GitHub before or are new to version control with GitHub, you can learn more about GitHub at GitHub Skills: https://skills.github.com/.
GitHub is a web-based platform that provides a collaborative environment for developers to store, manage, and share their code repositories. It is a cloud-based source control system that enables teams to work together on projects and manage the changes that are made to the code base over time.
GitHub allows developers to create and manage their own Git repositories, which can be either public or private. Developers can then use Git commands to push code changes to their repositories and track the history of changes made to the code base over time. Other developers can then clone or fork the repository to access the code and contribute to the project.
GitHub provides a range of features and tools to support collaboration, including issue tracking, pull requests, code reviews, and project management tools. These features enable teams to work together more efficiently, resolve issues quickly, and maintain high standards of code quality.
In addition to its core functionality as a version control system, GitHub has become a hub for open-source software development, with millions of open-source projects hosted on the platform. It also provides a marketplace for third-party integrations and tools, making it a valuable resource for developers looking to streamline their development workflows.
The following screenshots show the process of requesting and fulfilling a pull request via GitHub.
On your GitHub project page, click on the Pull requests tab:
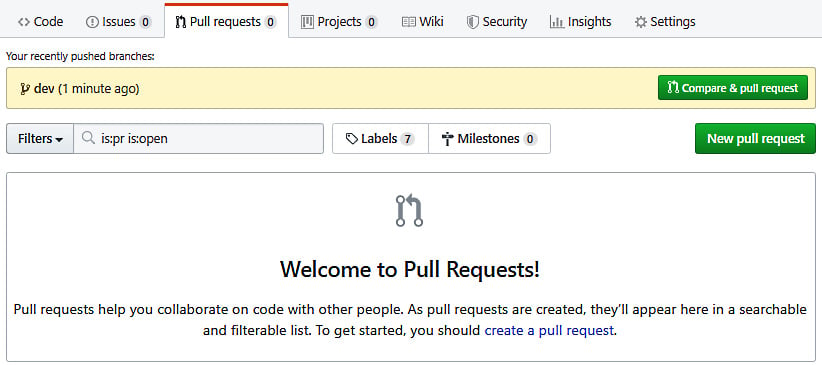
Figure 2.2: The Pull requests tab
Then, click on the New pull request button. This will display the Comparing changes page:
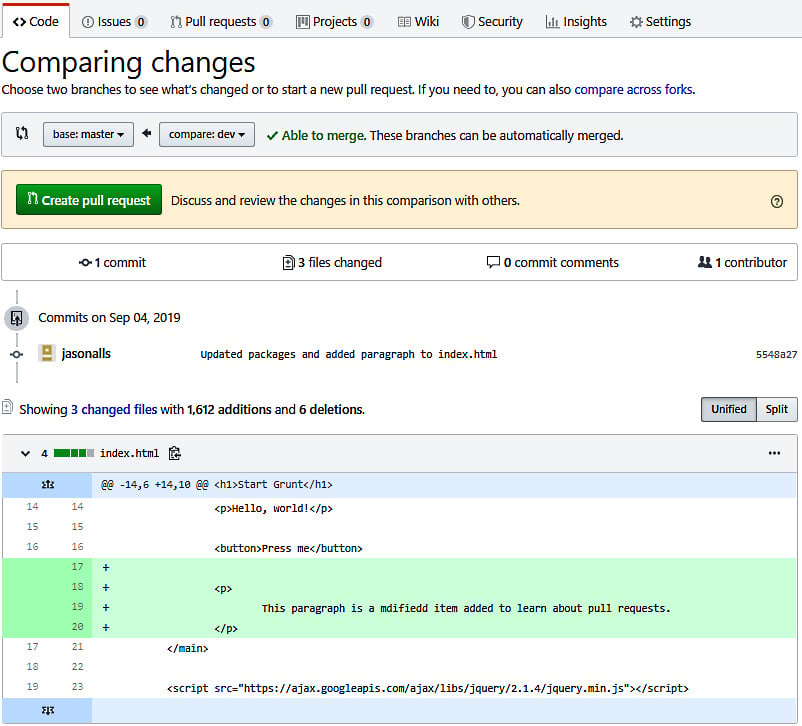
Figure 2.3: The Comparing changes page
If you are happy, then click on the Create pull request button to start the pull request. You will be presented with the Open a pull request screen:
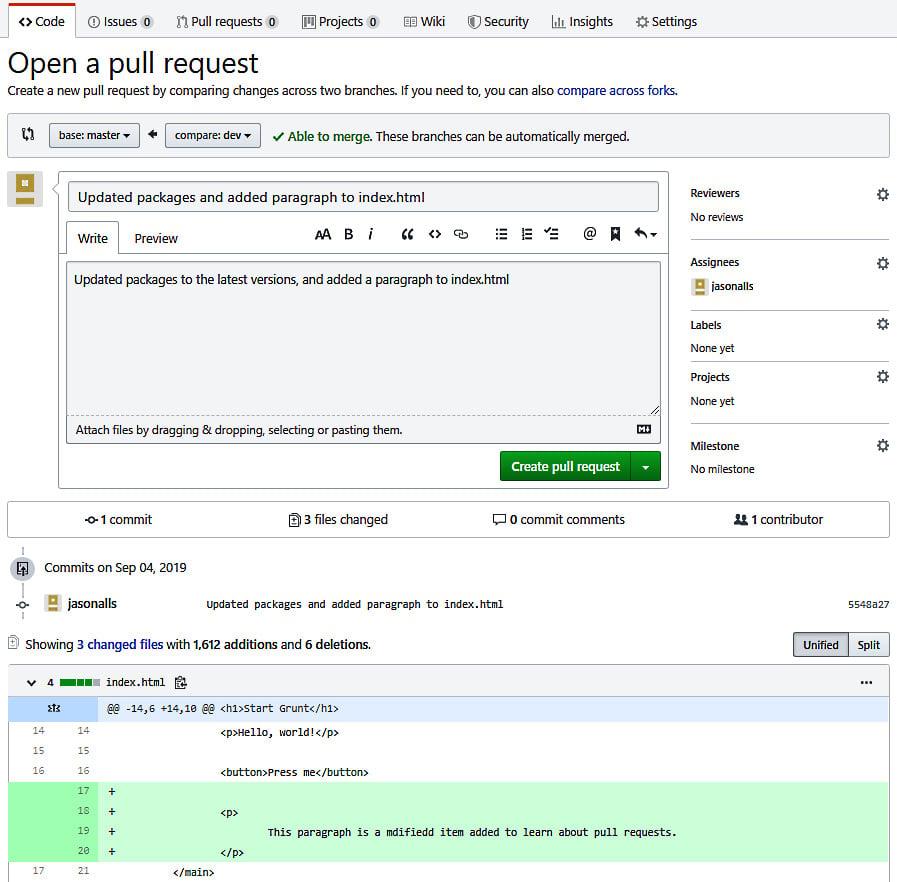
Figure 2.4: The Open a pull request page
Write your comment regarding the pull request. Provide all the necessary information for the code reviewer, but keep it brief and to the point. Useful comments include those that identify what changes have been made. Modify the Reviewers, Assignees, Labels, Projects, and Milestones fields as necessary. Then, once you are happy with the pull request details, click on the Create pull request button to create the pull request. Your code will now be ready to be reviewed by your peers.
Code conflict
In GitHub, code conflict resolution refers to the process of resolving conflicts that arise when two or more developers make changes to the same code file or lines of code in a Git repository. Code conflicts can occur when two or more developers modify the same piece of code in different ways, or when one developer modifies a code file while another deletes it.
When a code conflict occurs, GitHub will highlight the conflicting lines of code in the code file and notify the developers who made the conflicting changes. The developers can then use the GitHub web interface or a Git client to review the changes and resolve the conflict.
There are several ways to resolve code conflicts in GitHub:
- Merge: Developers can merge their changes if they have made non-conflicting changes to different parts of the code. This involves reviewing the changes and manually merging the code changes.
- Rebase: Developers can use the
git rebase
command to apply their changes on top of the changes made by another developer. This involves rebasing their changes on top of the changes made by the other developer and resolving any conflicts that arise. - Manual resolution: If the changes made by the developers conflict with each other, developers may need to manually resolve the conflicts by reviewing the changes and deciding which changes to keep and which to discard.
GitHub provides a range of tools to help developers resolve code conflicts, including visual diff tools, merge tools, and conflict resolution workflows. Code conflict resolution in GitHub aims to ensure that the code base is kept up to date, that conflicts are resolved efficiently, and that code quality is maintained.
Responding to a pull request
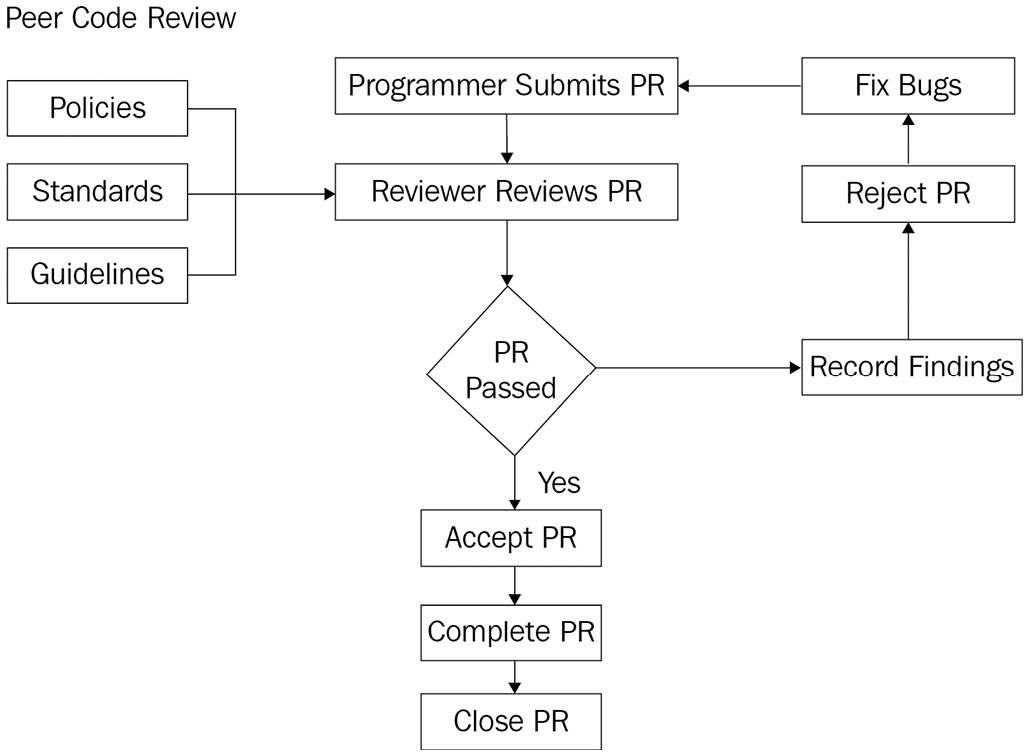
Figure 2.5: Responding to a pull request
Since the reviewer is responsible for reviewing pull requests before merging branches, we would do well to look at responding to pull requests:
- Start by cloning a copy of the code under review.
- Review the comments and changes in the pull request.
- Check that there are no conflicts with the base branch. If there are, then you will have to reject the pull request with the necessary comments.
Otherwise, you can review the changes, make sure the code builds without errors, and make sure there are no compilation warnings. At this stage, you will also look out for code smells and any potential bugs. Code smell is a term that’s used in software development to describe common signs of poor code design or implementation that can lead to code that is difficult to understand, maintain, or extend.
They are not necessarily errors, but rather indicators that the code could be improved. You will also check that the tests build, run, are correct, and provide good test coverage of the feature to be merged. Make any comments necessary and reject the pull request unless you are satisfied. When satisfied, you can add your comments and merge the pull request by clicking on the Merge pull request button, as shown here:
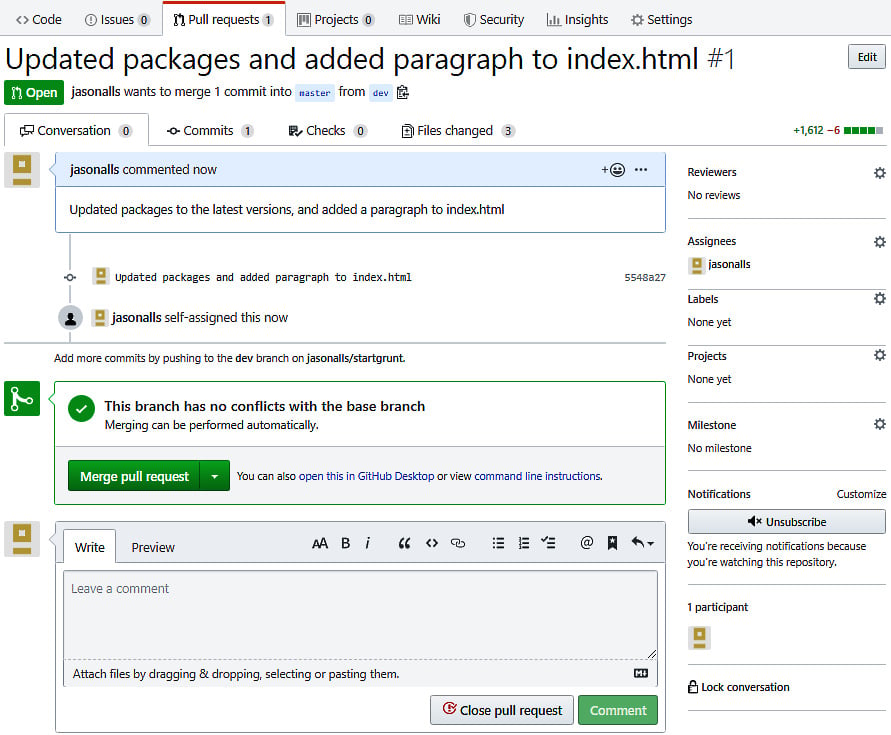
Figure 2.6: Merging pull requests
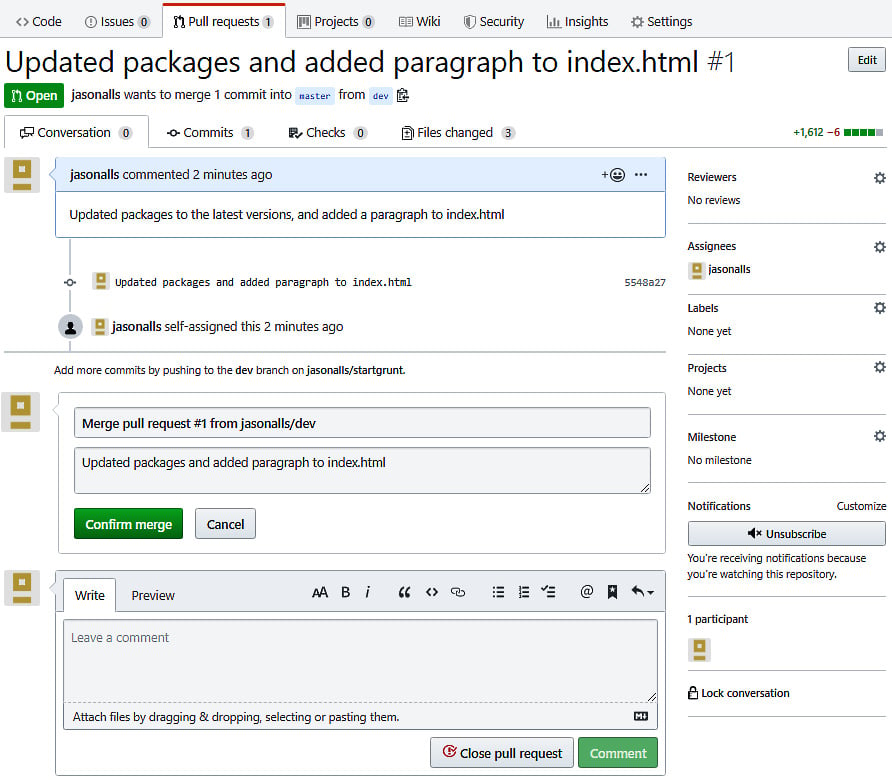
Figure 2.7: Confirming the merge
- Once the pull request has been merged and the pull request has been closed, the branch can be deleted by clicking on the Delete branch button, as can be seen in the following screenshot:
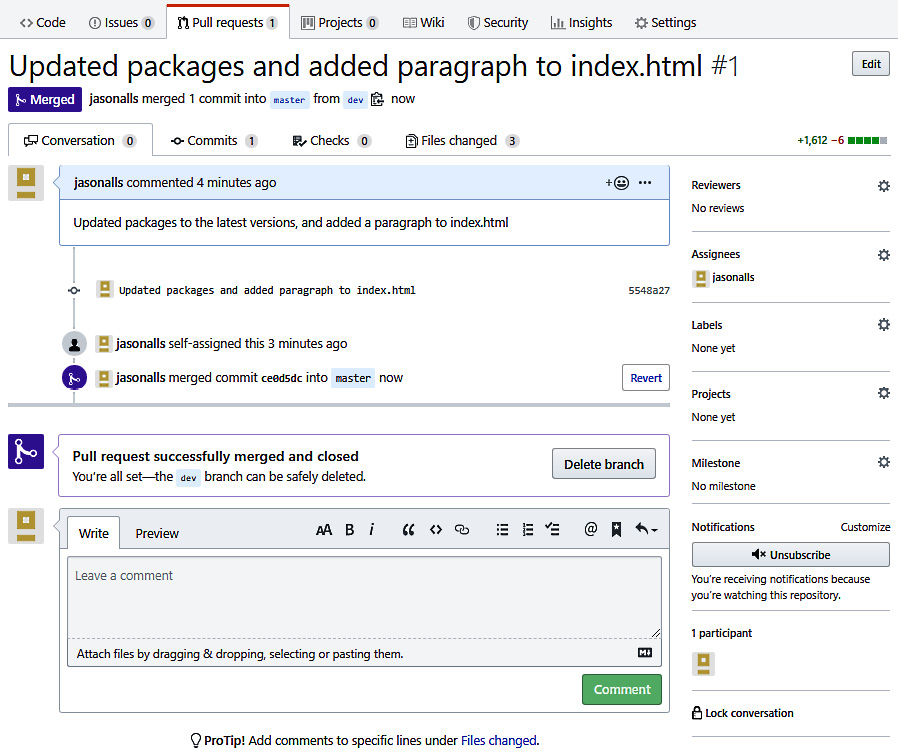
Figure 2.8: The Delete branch button
In the previous section, you saw how the reviewee raises a pull request to have their code peer-reviewed before it is merged. In this section, you saw how to review a pull request and complete it as part of a code review. Now, we will look at the negative and positive feedback that affects reviewees.
Effects of feedback on reviewees
When performing a code review of your peer’s code, you must also consider the fact that feedback can be positive or negative. Negative feedback does not provide specific details about the problem. The reviewer focuses on the reviewee and not on the problem. Suggestions for improving the code are not offered to the reviewee by the reviewer, and the reviewer’s feedback is aimed at hurting the reviewee.
Such negative feedback received by the reviewee offends them. This has a negative impact and can cause them to start doubting themselves. A lack of motivation then develops within the reviewee, and this can negatively impact the team, as work is not done on time or to the required level. The bad feelings between the reviewer and the reviewee will also be felt by the team, and an oppressive atmosphere that negatively impacts everyone on the team can ensue. This can lead to other colleagues becoming demotivated, and the overall project can end up suffering as a result.
In the end, it gets to the point where the reviewee has had enough and leaves for a new position somewhere else to get away from it all. The project then suffers time-wise and even financially, as time and money will need to be spent on finding a replacement. Whoever is found to fill the position must be trained in the system and the working procedures and guidelines. The following diagram shows negative feedback from the reviewer toward the reviewee:
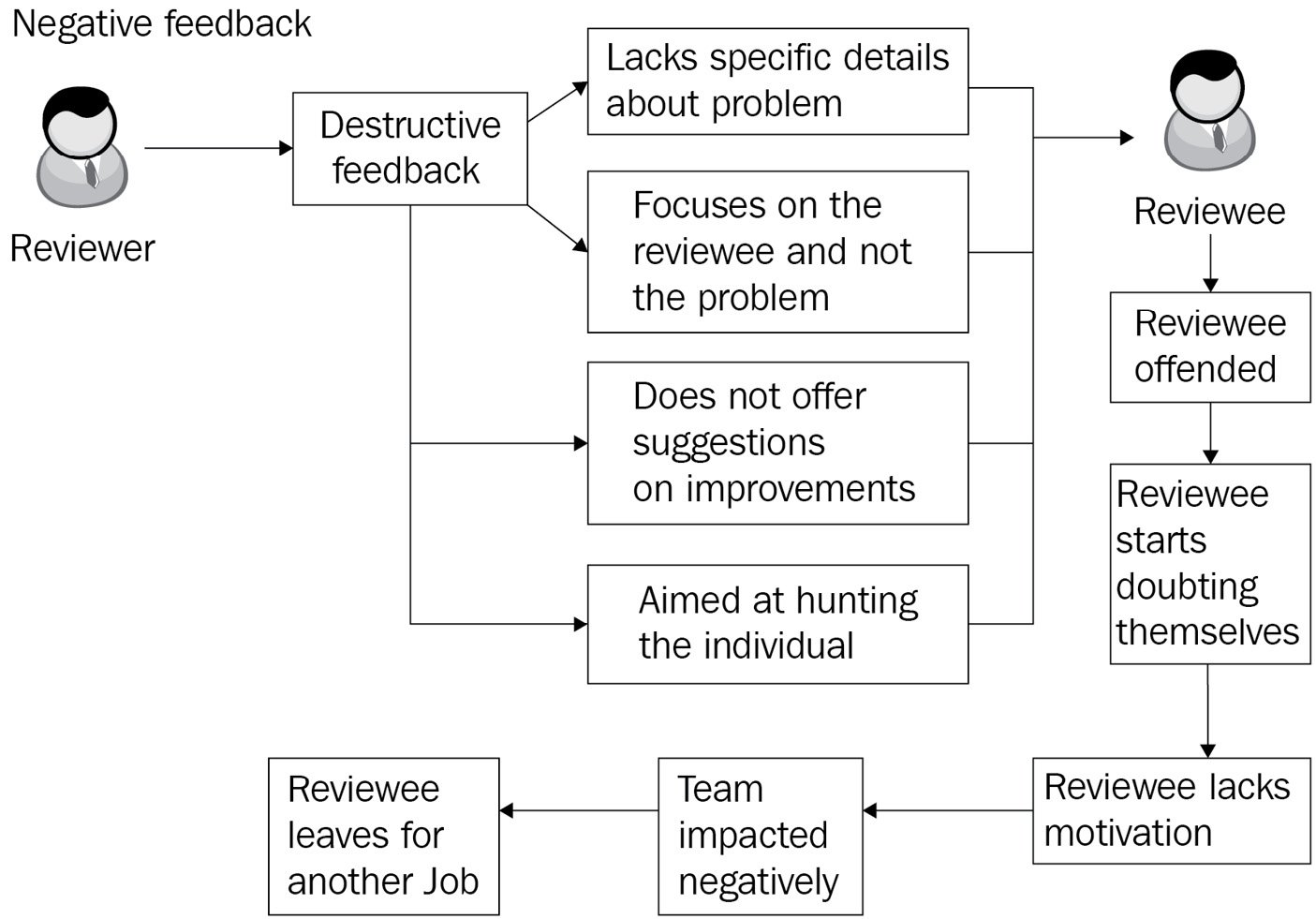
Figure 2.9: The negative feedback process
Conversely, positive feedback from the reviewer to the reviewee has the opposite effect. When the reviewer provides positive feedback to the reviewee, they focus on the problem and not on the person. They explain why the code they’ve submitted is not good, along with the problems it can cause. The reviewer will then suggest to the reviewee ways in which the code can be improved. The feedback provided by the reviewer is only given to improve the quality of the code submitted by the reviewee.
When the reviewee receives the positive (constructive) feedback, they respond positively. They take on board the reviewer’s comments and respond appropriately by answering any questions and asking any relevant questions themselves. After this, the code is then updated based on the reviewer’s feedback. The amended code is then resubmitted for review and acceptance. This has a positive impact on the team as the atmosphere remains a positive one, and work is done on time and to the required quality. The following diagram shows the results of positive feedback on the reviewee from the reviewer:
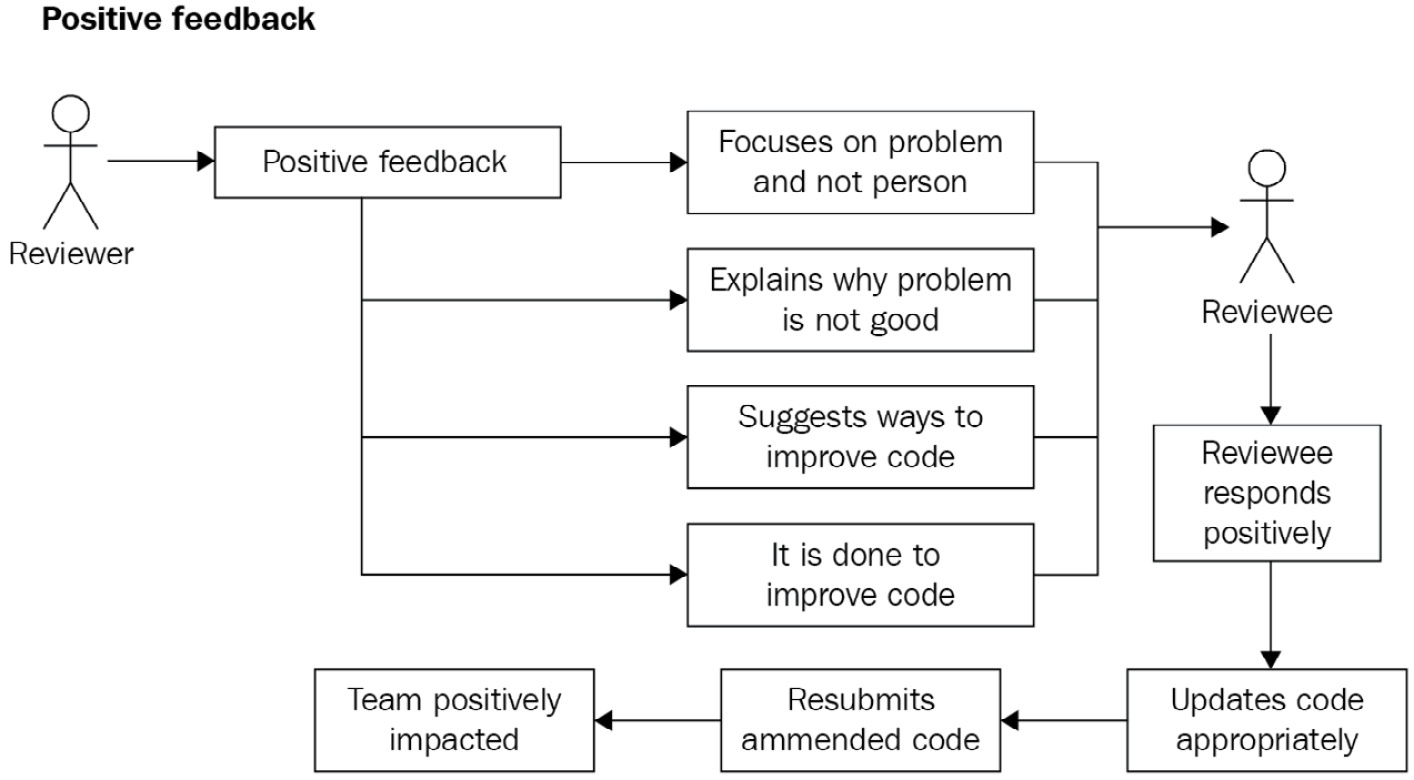
Figure 2.10: Positive feedback process
The point to remember is that your feedback can be constructive or destructive. Your aim as a reviewer is to be constructive and not destructive. A happy team is a productive team. A demoralized team is not productive and is damaging to the project. So, always strive to maintain a happy team through positive feedback.
A technique for positive criticism is the feedback sandwich technique. You start with praise on the good points, then you provide constructive criticism, and then you finish with further praise. This technique can be very useful if you have members on the team who don’t react well to any form of criticism. Your soft skills in dealing with people are just as important as your software skills in delivering quality code. Don’t forget that!
We will now move on and look at what we should review.