Wijmo provides a class for accessing data via a datareader
object and an optional proxy parameter. The data could be stored locally via a specific format, and parsed via a datareader
object. The proxy property holds the URL to the service that renders or holds the required data, which is usually in the backend and not stored on the client side.
We refer to this class as the wijdatasource
class. The jquery.wijmo.wijdatasource.js
library is responsible for creating the wijdatasource
widget class and it contains two sample proxy and reader classes.
Assume we want to parse or simply display a list of a few popular cities of the world, and that this data will be created and stored locally in our widget as follows:
var testArr = ["London", "Brussels", "Los Angeles", "Abuja", "Johannesburg", "Paris", "Amsterdam"];
To create our List of major cities
app, we can initialize a wijdatasource
class and utilize our data as follows:
<html> <head> <title>List of major cities app!! </title> <!--jQuery References--> <script src="http://ajax.aspnetcdn.com/ajax/jquery/jquery-1.7.1.min.js" type="text/javascript"></script> <script src="http://ajax.aspnetcdn.com/ajax/jquery.ui/1.8.17/jquery-ui.min.js" type="text/javascript"></script> <!--Wijmo Widgets JavaScript--> <script src="http://cdn.wijmo.com/jquery.wijmo-open.all.2.0.0.min.js" type="text/javascript"></script> <script src="http://cdn.wijmo.com/jquery.wijmo-complete.all.2.0.0.min.js" type="text/javascript"></script> <!--Theme--> <link href="http://cdn.wijmo.com/themes/rocket/jquery-wijmo.css" rel="stylesheet" type="text/css" title="rocket-jqueryui" /> <!--Wijmo Widgets CSS--> <link href="http://cdn.wijmo.com/jquery.wijmo-complete.all.2.0.0.min.css" rel="stylesheet" type="text/css" /> </head> <body> <span><h5>Hi Folks!! </h5></span> <span>This app prints nothing to screen but logs the local data read via datareader to console...</span> <script type="text/javascript"> // array to read {List of popular cities around the world...} var testArr = ["London", "Brussels", "Los Angeles", "Abuja", "Johannesburg", "Paris", "Amsterdam"]; // create datareader of array var myReader = new wijarrayreader([{name: 'label'}, {name: 'value'}, {name: 'selected',defaultValue: false}]); // create datasource var datasource = new wijdatasource({ reader: myReader, data: testArr, loaded: function (data){ // get items by data.items console.log(data.items) } }); // load datasource, loaded event will be fired after loading. datasource.load(); </script> </body> </html>
If the code is successfully run, you will see a message on your browser's screen telling you what the app does. A log of the objects is outputted to the console.
Once again, we add references to the Wijmo widget dependency files from CDN.
Next, we create the list of cities.
Next, we create the
datareader
object that has the format for arranging our list, and we set thedatareader
object to amyReader
handle.Time for the real deal! We initialize the
datasource
object as follows:var datasource = new wijdatasource({ reader: myReader, data: testArr, loaded: function (data){ // get items by data.items console.log(data.items) } });
Then, we load the
datasource
object usingdatasource.load()
. Theloaded
function, which is a property ofwijdatasource
, is called afterdatasource.load()
fires off. The loaded data is referenced as the parameter or argument in that function for which the items within can be referenced bydata.items
.
It is also possible to load data from a prepared datasource
object into a targeted UI element such as a <div>
or <p>
tag. This datasource
object could be prepared via a proxy, which is a mechanism for retrieving data from a remote source, or locally as previously shown.
To show how to work with wijdatasource
and HTML elements, let us look at one more example that utilizes local data. Assume that we have a list of some countries we want to populate in a list. Assume that we also want a selection of those cities visible only after some substring of that country is entered in an input field. We also want this event to trigger only when a button is clicked.
For instance, say we have a list containing the words Computer, Motherboard, Commute, Mother, apple, and people. If the user enters Com
and clicks on the button, the output should be a new list containing Computer
and Commute
. Also, if the user enters ple
and clicks on the button, the output should be a new list containing apple
and people
.
To properly illustrate this, we will make use of a Wijmo widget called wijlist
. A wijlist
object is simply a Wijmo list widget, which can be created by targeting a given element on the page and initiating the widget on it with the following code:
var myArr = [6,7,8,9,0]; $('.myList').wijlist('setItems', myArr);//Render the list in the client browserlist.wijlist('renderList');
The preceding code assumes the existence of a class called myList
, and we initialize or host the wijlist
object on all elements with a myList
class. We create a local array referenced by myArr
. Finally, we render the wijlist
object on our browser.
Having understood what a wijlist
object is and how to deploy one in our widget, we can proceed with our app. Enter the following code in your favorite editor:
<!--jQuery References--> <html> <head> <script src="http://ajax.aspnetcdn.com/ajax/jquery/jquery-1.7.1.min.js" type="text/javascript"></script> <script src="http://ajax.aspnetcdn.com/ajax/jquery.ui/1.8.17/jquery-ui.min.js" type="text/javascript"></script> <!--Wijmo Widgets JavaScript--> <script src="http://cdn.wijmo.com/jquery.wijmo-open.all.2.0.0.min.js" type="text/javascript"></script> <script src="http://cdn.wijmo.com/jquery.wijmo-complete.all.2.0.0.min.js" type="text/javascript"></script> <!--Theme--> <link href="http://cdn.wijmo.com/themes/rocket/jquery-wijmo.css" rel="stylesheet" type="text/css" title="rocket-jqueryui" /> <!--Wijmo Widgets CSS--> <link href="http://cdn.wijmo.com/jquery.wijmo-complete.all.2.0.0.min.css" rel="stylesheet" type="text/css" /> </head> <body> <div class="ui-widget"> <P>Enter either whole or part of any of the following words (representing some countries): "United States of America", "United Arab Emirates", "Australia", "Canada", "Luxembourg", "Granada", "Nigeria" or "Niger", then click the Load Data button. Data that matches the whole or part of the value you entered, will be loaded into the wijlist.</p> <input style="width: 250px" id="testInput" type="textbox" class="ui-widget-content ui-corner-all" /> <input type="button" id="loadData" value="Load Data" /> <div id="list" style="height: 300px; width: 400px;"></div> </div> <script> $(document).ready(function () { $("#list").wijlist({}); var testArray = [{ label: 'United States of America', value: 'United States of America' }, { label: 'United Arab Emirates', value: 'United Arab Emirates' }, { label: 'Australia', value: 'Australia' }, { label: 'Canada', value: 'Canada' }, { label: 'Luxembourg', value: 'Luxembourg' }, { label: 'Granada', value: 'Granada' }, { label: 'Nigeria', value: 'Nigeria' }, { label: 'Niger', value: 'Niger' }]; loadList(testArray); $('input#loadData').click(function(){ var text = $('input#testInput').val().toLowerCase(); if(text == "" || text == null){ alert('No Match'); return ; } var matches = []; for(var i = 0; i < testArray.length; i++){ var newTxt = testArray[i].label.toLowerCase(); if(newTxt.indexOf(text) > -1){ //console.log('found'); matches.push(testArray[i]); } } loadList(matches) return ; //console.log('failed'); }); function loadList(arr){ var list = $("#list"); //set the array testArray to the Wijmo list widget list.wijlist('setItems', arr); //Render the list in the client browser list.wijlist('renderList'); } }); </script> </body> </html>
If the preceding code is successfully run, you will have something like this in your browser:
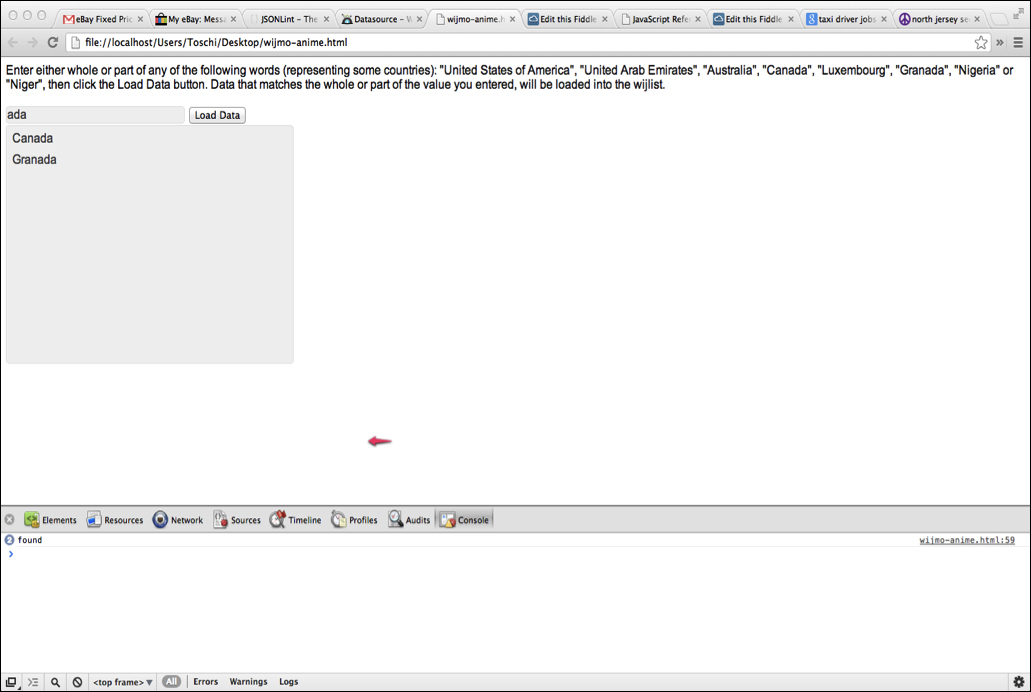
So once again, starting from the document.ready
function, we will go through our code to explain how this widget works:
First of all, we create a
wijlist
object by hosting it on thediv
tag withid = list
.Next, we create a local array,
testArray
, which will be digested by theloadList
function. This will settestArray
as the new content of thewijlist
object.By clicking the Load Data button, we compare the text entered in the input field with
id = "testInput"
, and each of the items in thewijlist
object via afor
loop.During the loop, we append subsequent matches to a new local array,
newList
.At the end of the loop, if
newList
is not empty we setnewList
as the new Wijmo list data, thus overwriting the previous list data and getting a new result in our browser.
In the just-concluded example, it is important to note that we only used a local array without making reference to a wijdatasource
object. Usually, some array is passed as one of the properties in the parameters of a wijdatasource
object, which sets the array as a datasource
object. The app we just created did not use a datasource
object but a wijlist
object.
To use the datasource
and wijlist
objects, copy the following code into your editor and run it:
<!--jQuery References--> <html> <script src="http://ajax.aspnetcdn.com/ajax/jquery/jquery-1.7.1.min.js" type="text/javascript"></script> <script src="http://ajax.aspnetcdn.com/ajax/jquery.ui/1.8.17/jquery-ui.min.js" type="text/javascript"></script> <!--Wijmo Widgets JavaScript--> <script src="http://cdn.wijmo.com/jquery.wijmo-open.all.2.0.0.min.js" type="text/javascript"></script> <script src="http://cdn.wijmo.com/jquery.wijmo-complete.all.2.0.0.min.js" type="text/javascript"></script> <!--Theme--> <link href="http://cdn.wijmo.com/themes/rocket/jquery-wijmo.css" rel="stylesheet" type="text/css" title="rocket-jqueryui" /> <!--Wijmo Widgets CSS--> <link href="http://cdn.wijmo.com/jquery.wijmo-complete.all.2.0.0.min.css" rel="stylesheet" type="text/css" /> <div class="ui-widget"> <P>Enter either whole or part of any of the following words (representing some countries): "United States of America", "United Arab Emirates", "Australia", "Canada", "Luxembourg", "Granada", "Nigeria" or "Niger", then click the Load Data button. Data that matches the whole or part of the value you entered, will be loaded into the wijlist.</p> <input style="width: 250px" id="testInput" type="textbox" class="ui-widget-content ui-corner-all" /> <input type="button" id="loadData" value="Load Data" /> <div id="list" style="height: 300px; width: 400px;"></div> </div> <script> $(document).ready(function () { $("#list").wijlist({}); var testArray = [{ label: 'United States of America', value: 'United States of America' }, { label: 'United Arab Emirates', value: 'United Arab Emirates' }, { label: 'Australia', value: 'Australia' }, { label: 'Canada', value: 'Canada' }, { label: 'Luxembourg', value: 'Luxembourg' }, { label: 'Granada', value: 'Granada' }, { label: 'Nigeria', value: 'Nigeria' }, { label: 'Niger', value: 'Niger' }]; //var datasource = null; var myReader = new wijarrayreader([{name: 'label'}, {name: 'value'}, {name: 'selected',defaultValue: false}]); var datasource = new wijdatasource({ reader: myReader, data: testArray, loaded: function (data){ // get items by data.items loadList(data.items); } }); datasource.load(); function loadList(items){ var list = $("#list"); //set the array testArray to the Wijmo list widget list.wijlist('setItems', items); //Render the list in the client browser list.wijlist('renderList'); } $('#loadData').click(function(){ //process this section when the load Data is clicked... var text = $('input#testInput').val().toLowerCase(); if(text == "" || text == null){ alert('No Match'); return ; } var newList = []; for(var i = 0; i < testArray.length; i++){ var newTxt = testArray[i].label.toLowerCase(); if(newTxt.indexOf(text) > -1){ //console.log('found'); newList.push(testArray[i]); } } if(newList.length > 0){ loadList(newList) return ; } //console.log('failed'); return ; }); }); </script> </html>
In the preceding code, we actually instantiate a datasource
object using the local array or data. These are all done when the page loads.
datasource = new wijdatasource({ reader: myReader, data: testArray, loaded: function (data){ // get items by data.items loadList(data.items); } });
The reader
property is set to myReader
. This is the format for processing testArray
, which is assigned to the data
property of the wijdatasource
object. The loaded
function is called after the wijdatasource
object is created, and it calls loadList()
. This, in turn, renders the wijlist
object in the list
div. The functionality of the app, however, remains the same.
So far, we've demonstrated how to manipulate local data sources. The team at Wijmo was also aware of the possibilities of working with distributed systems that host data in remote locations, and thus provided a mechanism for digesting remote or distributed data sources over the network. This is achievable using the proxy
property of the wijdatasource
object. The proxy is set to the remote location of the data.
The following code was obtained from http://wijmo.com/wiki/index.php/Datasource and modified to function like our wijdatasource
app, as was done previously, but makes use of a proxy now.
<html> <head> <script src="http://ajax.aspnetcdn.com/ajax/jquery/jquery-1.7.1.min.js" type="text/javascript"></script> <script src="http://ajax.aspnetcdn.com/ajax/jquery.ui/1.8.17/jquery-ui.min.js" type="text/javascript"></script> <!--Wijmo Widgets JavaScript--> <script src="http://cdn.wijmo.com/jquery.wijmo-open.all.2.0.0.min.js" type="text/javascript"></script> <script src="http://cdn.wijmo.com/jquery.wijmo-complete.all.2.0.0.min.js" type="text/javascript"></script> <!--Theme--> <link href="http://cdn.wijmo.com/themes/rocket/jquery-wijmo.css" rel="stylesheet" type="text/css" title="rocket-jqueryui" /> <!--Wijmo Widgets CSS--> <link href="http://cdn.wijmo.com/jquery.wijmo-complete.all.2.0.0.min.css" rel="stylesheet" type="text/css" /> <style> input#testInput{width: 250px} </style> </head> <body> <div class="ui-widget"> <P>Enter either whole or part of any of the following words (representing some countries): "United States of America", "United Arab Emirates", "Australia", "Canada", "Luxembourg", "Granada", "Nigeria" or "Niger", then click the Load Data button. Data that matches the whole or part of the value you entered, will be loaded into the wijlist.</p> <input id="testInput" type="textbox" class="ui-widget-content ui-corner-all" /> <input type="button" id="loadData" value="Load Data" /> <div id="list" style="height: 300px; width: 400px;"></div> </div> <script> $(document).ready(function () { $("#list").wijlist({}); var proxy = new wijhttpproxy({ url: "http://ws.geonames.org/searchJSON", dataType: "jsonp", data: { featureClass: "P", style: "full", maxRows: 10, name_startsWith: 'ab' }, key: 'geonames' }); var datasource = null; var thisData = null; var myReader = new wijarrayreader([ { name: 'label', mapping: function (item){ return item.name + (item.adminName1 ? ", " + item.adminName1 : "") + ", " + item.countryName } }, { name: 'value',mapping: 'name' } ]); datasource = new wijdatasource({ reader: myReader, proxy: proxy, loaded: function (data){ // get items by data.items thisData = data.items; loadList(data.items); } }); datasource.load(); function loadList(items){ var list = $("#list"); //set the array testArray to the Wijmo list widget list.wijlist('setItems', items); //Render the list in the client browser list.wijlist('renderList'); } $('#loadData').click(function(){ //process this section when the load Data is clicked... var text = $('input#testInput').val().toLowerCase(); proxy.options.data.name = text; datasource.load(); }); }); </script> </body> </html>
The preceding code pulls data from a proxy instead of local data. The data obtained has been limited to a maximum of 12, starting with ab
. The functionality of our widget remains the same.
For more insight into wijdatasources
, you can visit http://wijmo.com/wiki/index.php/Datasource. There are more configurations and options there that are very useful in customizing your widget.