Applications in the digital domain share a common thread of architecture across multiple industrial sector domains. Obviously, they share and rely on the Java EE 7 standard platform and JVM in order to piggy-bank the development, various nonfunctional attributes, and enterprise application infrastructure. There are subtle differences in the building of the applications.
The standard Java EE web architecture is derived from the client-server models of the 1990s. The application server is the key component as it is responsible for three containers in the full Java EE 7 specification: Servlet, CDI, and EJB. Generally, we map these containers to the layer tier architecture for a monolithic web application. The idea of this architecture is to enhance the best practice from the point of view of solid software engineering. We want to maintain a separation of the concerns in the layers in order to avoid rigid coupling between layers and have a strong cohesion in the layers. The three layers are as follows:
Presentation layer is strongly associated with code that depends and requires the Servlet container. FacesServlet is supplied as a part of the JSF. Alternative web application frameworks also have the idea of Front Controller that dispatches a request to the separate controllers. The presentation tier also contains the controllers and view templates.
Domain layer is associated with code that holds the business logic and projections of the persistence objects. It contains the application rules, business logic, and business process management. Domain objects may or may not be a part of a dependency injection. In most of the modern web applications, almost all the domain layer components and objects are a part of a dependency injection container. Therefore, domain layer is associated with the CDI container.
Integration layer is associated with the transportation of data from the application to the durable persistence or service areas of the system. This layer maps to a POJO that is a part of the EJB container. Usually, these objects handle the service calls from the application. As EJBs, they are transactional and not necessarily contextual, which is why they are not a part of the CDI container. These objects communicate with an external system like database through JPA and/or JDBC asynchronously to the other systems through a message over JMS or they can also synchronously (or asynchronously) invoke the remote web service endpoints through the REST or SOAP calls.
The following diagram illustrates the architecture:
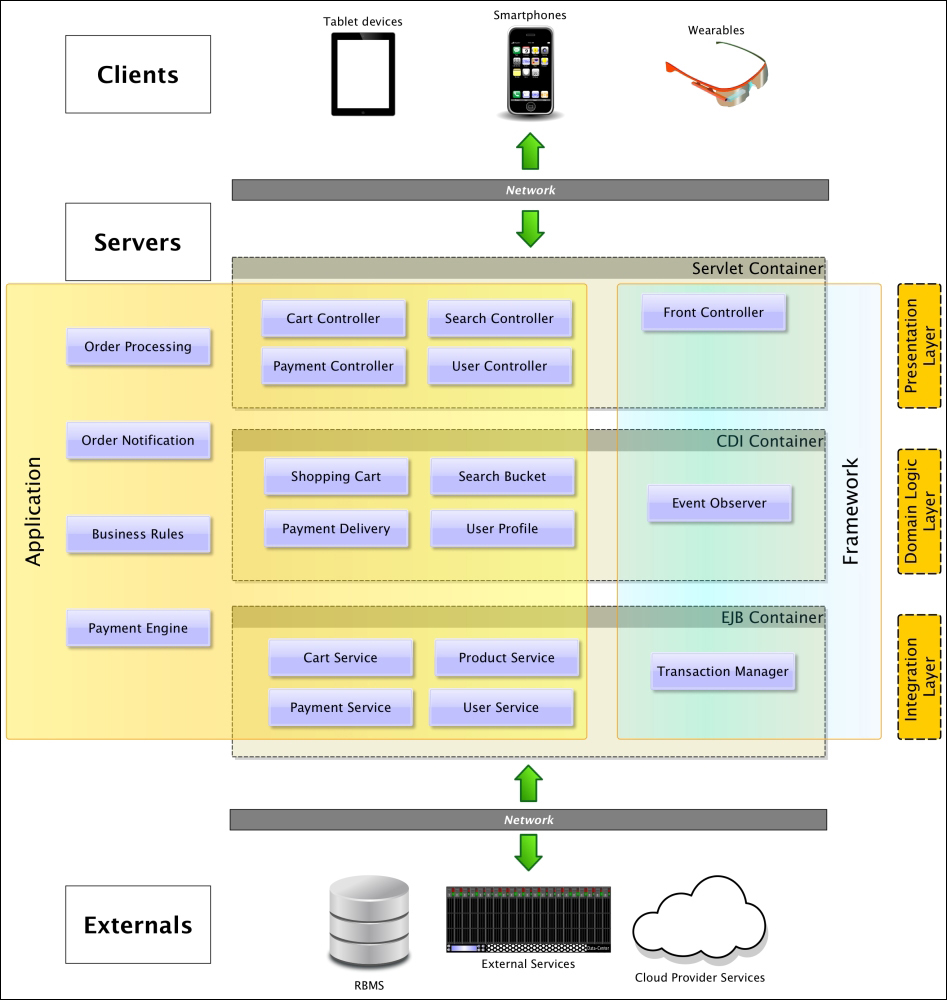
The standard Java EE web architecture
From the standard Java web architecture, there are several adaptations. Organizations may choose to optimize their architecture in order to suit a particular trade-off. A trade-off might be performance versus scalability, which may mean restructuring the integration layer in order to use a NoSQL database over a particular RDMS solution.
In this section, we will examine availability versus performance for a particular customer. Availability is the degree of a system to be accessible. If the system is down, then it is not available. Performance is the ability of the system to carry out the required functionality in a target timeframe. So, this customer is truly interested in the uptime of the system because the downtime will cost money, but they also want a fixed quantum of throughput.
Which items can degrade the performance? If the application has too much sanity checking in the code (check pointing), then it will degrade the performance. If the performance is degraded, then it may also reduce availability. However, if you do not perform a sanity check for enough parameters, then a system will be culpable to errors. If a hacker finds a weakness in your security or your caseworkers enter the wrong data too often, then your availability is compromised because your business will suffer downtime to fix issues.
One solution is to split the architecture in two computation bunkers. We can take advantage of the modern power in smartphones, tablets, and desktop computers to render the content on the client side. Therefore, this architecture, suits rich clients and other suitable devices with enough GPU power.
The code that has been sanity-checked and rendered is removed from the server side to a large degree. Depending on the architecture, we can use the JavaScript framework technologies that rely on Model View ViewModel (MVVM), which is supported by AngularJS. Based on the technology choice, we can use an alternative to JSF such as the upcoming Java EE 8 MVC or directly the JAX-RS endpoints. We must ensure that correct validation takes places on both the client and server sides. We must also design the REST API or other remote invocation between client and server to be secure, safe, and idempotent. Note that we still have the features such as CDI, EJB, JMS, and JPA available to us on the server side.
There are benefits in this extended Java EE 7 architecture that help in the performance versus scalability trade-off. If we introduce a caching layer of data, we will gain the ability to return the data that is mostly derived from static references or changes very infrequently or is requested most often. The key benefit of caching requests from the smart clients means that response times are minimized.
Just after Java EE 7 was released in 2013, JCache temporary caching 1.0 final JSR 107 (https://jcp.org/en/jsr/detail?id=107) was released, which is now supported by brands such as HazelCast and Terracota.
This architecture suits a hybrid form that extends Java EE and goes beyond and outside the box of specifications. The Java EE 7 umbrella specification does not specify the orchestration of the servers, monitoring of servers or systems, deep authorization, and cloud provisioning. Let's take a look at the following figure:
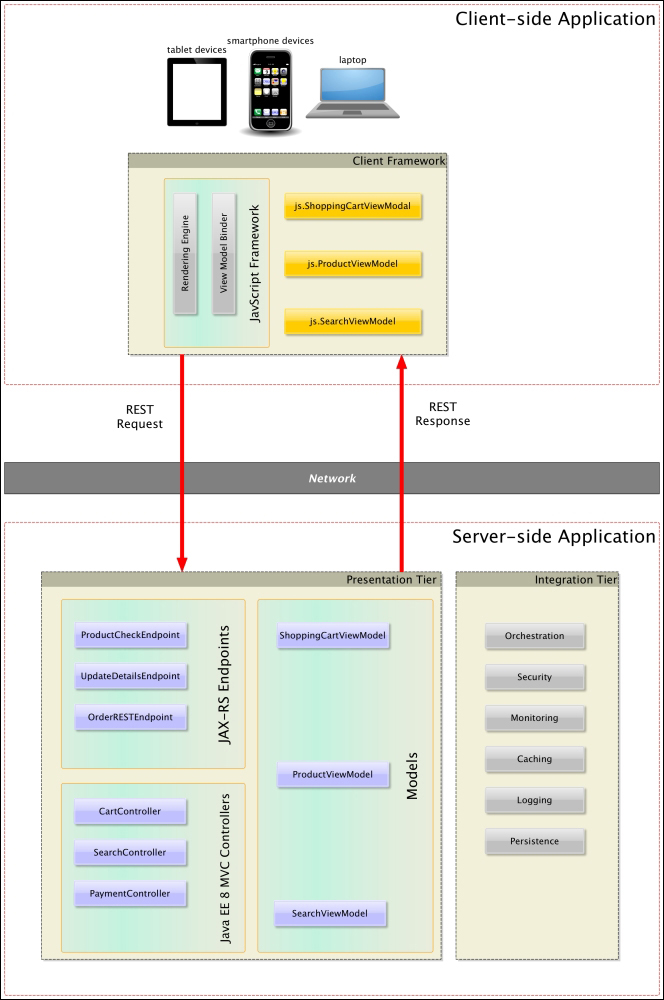
An extended hybrid Java EE architecture that remedies performance versus availability
There is another architecture that is popular with the leading edge businesses that favor continuous deployment and guerilla engineering. This is called containerless application, which is actually a misnomer. If we think about it, every entity system is contained in some abstract component from the highest level container and down to the hardware CPU. The operating system is constrained by the CPU, the JVM process is constrained by the operating system, and the application server contains the deployed Java EE application.
Technical architects make trade-offs all the time. They may consider agility like time-to-market versus cost, innovation versus affordability, and politically, the integration of legacy systems with new technology, such as a containerless system. The proper name for the containerless application ought to be embedded application controlled server, which accurately describes the architecture. There are several Java EE application server providers that allow a standalone Java application to spin a fully embedded server from the static void main()
entry point. Vendors such as JBoss's WildFly, Tomitribe's Tom EE, and of course, Oracle's GlassFish have nonstandard APIs for embedded execution. Some of the proprietary vendor solutions even allow the modular selection of the Java EE abilities in the runtime of the embedded server. An architect may thus pick and choose the provisioning of the JAX-RS, JSF, CDI, EJB, and JMS modules. A well-balanced and proficient developed team is able to circumvent the change management. The team operates under the radar by writing a Containerless solution instead of being told for the 100th time that they are not permitted to upgrade the IBM WebSphere 7 application server.
Therefore, embedded servers are popular for the beginning of building the microservices architecture across several concerns. Allowing the application to control the start up and halting of the embedded servers means that these archetypes play well with the modern DevOps movement (Developers/Operations team) and the concepts around the automatic configuration management control.
The clear disadvantage of the containerless solution is that the building of the development tools for the traditional WAR deployment does not understand this mode. So, a digital engineer may lose the interactive and fast turnaround of increment engineering using a Java EE solution. However, it might be a short-term issue as the Java IDE makers are fairly good at picking up trends in engineering. We can hope that the Java EE technical leaders, architects, and the wider community voice their support for the specification to include a containerless API.
With an embedded server, engineering teams have the responsibility to ensure that the infrastructure is correctly set up. The technical architects gain the benefit of precisely controlling the external integration points and this is useful for the security, authorization and authentication, monitoring and logging, and persistent access. However, going from request to the response is exactly the same as in the standard Java web architecture. Let's have a look at the following screenshot:
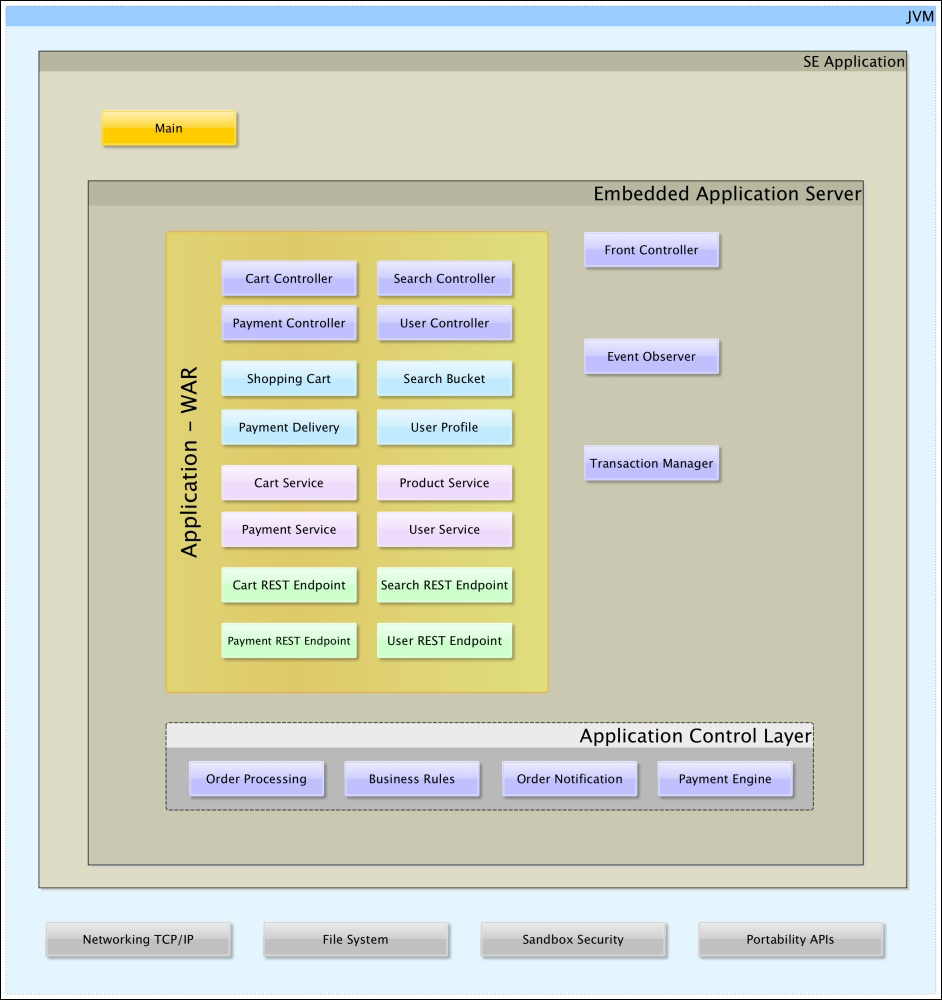
Containerless Java EE architecture diagram
Although there is no standardization on Java EE 8 for the embedded server application architecture, other developers have at least precipitated some innovations in order to move the community in this direction. There is the Apache Delta Spike CDI Container Control (https://deltaspike.apache.org/documentation/container-control.html) that currently provides a cross server library to start and stop the CDI container in a standalone Java SE environment. Delta Spike is an award-winning open source project that has specialist modules for Bean Validation, CDI, Data, Security, and Servlets as well as container management. It is worth keeping an eye on this project because some of their innovations have made it to the Java EE 8 standard. The @javax.transactional.Transational
annotation that allows CDI managed beans take part in Container Managed Transactions (Java EE 7 and JTA 1.2) was first proposed and developed in project Delta Spike.
The embedded application controlled server is a gateway to the Java-based microservices architecture. These microservices are a style of designing an enterprise architecture where each component solves and operates one requirement of the overall system. These key drivers are the nonfunctional requirements and can be any combination of Availability, Flexibility, Maintainability, Networkability, Performance, Robustness and Scalability. Of these, the original requirements for businesses to choose this style over the traditional monolithic architecture were Availability and Scalability.
Architects see certain advantages in microservices: these components follow the UNIX architectural principle of do one thing and do it well and the ability to chop and change the component at will. The microservices style enables the language agnostic communications; therefore, the implementer has the freedom to write a component in Java, Groovy, or Scala or even in a non-JVM language such as C++. This architecture strongly favors JSON or XML over REST; however, there is nothing to stop a software shop from using SOAP and XML. Technically, microservices are some of the most exciting digital projects on the planet today; because of these investments, some businesses have clear distinct commercial advantages.
The cost of microservices is the networking complexity that includes payload sizes, monitoring (heart-beating), logging, and fault tolerance with redundancy management and service routing. There are ancillary costs that business managers and stakeholders should be aware of, namely time-to-marketing, training, information silos, and of course, change culture.
As with many movements, there is also a spectrum of effort, ability, and feasibility. A business may not necessarily have to give up on the monolith architecture completely, especially if scalability and high availability are not a high priority. There is certainly a route for the componentized services architecture for the majority of the digital business, which takes the best of the monolith—transactions, persistence, and configuration—and radically employs as much of the styles of the microservices as possible. Therefore, an embedded server dedicated for order management should not have code that is relevant to the payment processing in it. Rather, the order management component should invoke the payment processing component externally. Let's have a look at the following figure:
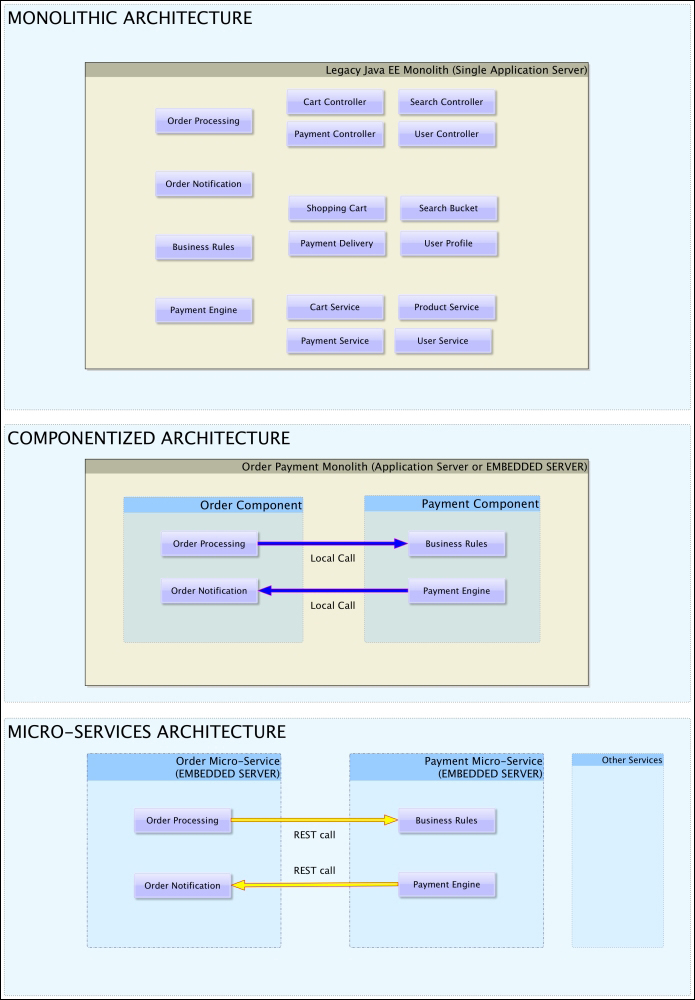
An evolution of a Java EE monolith to a microservices architecture
The preceding illustration shows a decomposition of the standard Java EE web architecture into a hybrid component architecture and then an evolution to a full microservice.
There are many ways to go from the request and then back to the response. In addition to where do we go as a team in order to get there? We must ask ourselves the key questions: how do we get there? Why do we want to get there?