In this section, we will take a look at Knockout.js, including a brief introduction and the best features. If you already have some experience with the library, then you can skip this chapter and go to Chapter 2, Creating a Simple Knockout.js Application, to read about the advanced features.
Knockout.js is an open source standalone JavaScript library developed by Steve Sanderson in 2010. The main concept of the library is implementation of the Model-View-ViewModel design pattern for web applications on the client side. The library has powerful tools to make your JavaScript code and HTML/CSS markup easier and more readable with the help of so-called observables objects and declarative bindings.
Thus Knockout.js can help you create rich HTML pages with a clean underlying data model and dynamically self-updatable UI even for very big applications with complex logic and interfaces.
Knockout.js has a lot of features that distinguish it from other similar JavaScript frameworks. There are different solutions to create the common logic of your application and interaction between data and user interface. When you select the main library to build an application, you should understand its benefits.
The following are the best features of Knockout.js:
Nice dependency tracking based on MVVM: Once data is changed, HTML will be automatically updated. You shouldn't think about updating DOM when writing logic code. We will discuss the MVVM pattern in more detail later.
Two-way declarative bindings: This is a very simple way to link DOM elements to your data model, as shown in the following line of code:
<button data-bind="enable: items().count < 7">Add</button><input data-bind="value: username" />
Simple and extensible: You can write your own type of declarative bindings for any purpose. Each new binding is defined by two simple functions for the
init
andupdate
events. For example, you can define a binding for the duration of a slide animation, as follows:<div data-bind="slideDuration: 600">Content</div>
Absence of dependency: You don't need any third-party libraries. However, you may need the jQuery library for some advanced features support or performance issues, but most applications already use this library.
Pure JavaScript: Knockout.js doesn't use any JavaScript superstructure, such as TypeScript or CoffeeScript. The source code of the library is represented by the pure and readable JavaScript code; it works with any server- or client-side technology.
Compatibility with mainstream browsers: Knockout.js supports Mozilla Firefox 2+, Google Chrome 5+, Opera 10+, Safari, and even Internet Explorer 6+. It also works excellently on mobile (phone and tablet) devices.
Small size: The size of Knockout.js is around 46 KB after gzipping for version 3.1.0. The debug versions (without compression) have a size of around 214 KB but you don't need it in the production case.
Templating: The powerful template system will allow you to create reusable HTML chunks, which you can use for parts of a web page. It also has a nice syntax, as shown in the following code:
<script type="text/html" id="person-template"> <h3 data-bind="text: name"></h3> <p>Credits: <span data-bind="text: credits"></span></p> </script>
The Model-View-ViewModel is a design pattern with a clear separation of the user interface from business logic. The main components of MVVM are as follows:
Model: This represents the state and operations of your business objects and is independent of UI. When using Knockout.js, you will usually make Ajax calls to some server-side code to read and write this stored model data.
View: This is a visible UI representing your business objects.
ViewModel: This is an intermediary between the View and Model, and is responsible for handling the UI logic.
You can see the scheme of MVVM in the following diagram:
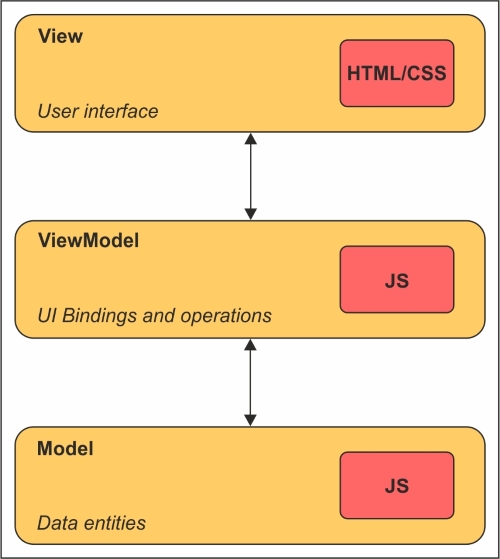
The MVVM pattern looks like the classic Model-View-Controller (MVC) pattern. The Model is also your stored data, the View is also a graphical representation of your data for the user, but instead of a controller, MVVM uses a ViewModel for interaction between the Model and View. The MVVM has a number of advantages over the MVC, such as the following:
Low coupling: It's a property of the MVVM by design, because of which we use a clearer separation between the data, interface, and behavior. MVVM has a clearer separation between the data, interface, and behavior for most application architectures.
Testable: Low coupling makes the application more comfortable for unit-testing; now you can separately test each layer of your application. Testing UI logic by testing only ViewModel is an easier way than testing a complete HTML page.
Сode extensibility: Separation by layers makes it easier to extend each specific part of the code without affecting the others.
Two-way data-binding: This avoids having to write a lot of boilerplate code.
Let's consider MVVM in examples. In parentheses, you can see the representation format of each layer, but note that these are just examples; different applications use different formats:
Knockout.js: This is the target case for this book. Most often, a Knockout.js application will use the following schemes:
Model: This includes some data on the server side; you can read and write it via Ajax calls (JSON).
View: This is the web page (HTML/CSS).
ViewModel: This is the representation of data and operations (JavaScript).
WPF: Many .NET developers are familiar with MVVM on technologies such as WPF, Silverlight, and WinRT. Originally, the MVVM pattern was conceived to support WPF and Silverlight. For example, you can use the following scheme in your WPF application:
Model: This includes some data in the client database (binary)
View: This is the user interface of the WPF application (XAML)
ViewModel: This is the special data context classes (C#)
In this book, we will not explain MVVM in detail because the easiest way to understand MVVM is a careful study of the examples in this book. The examples will work only with View and ViewModel because communication with a real data model (commonly, some data on the server, which can use SQL, RSS, XML, binary, and so on) is another story. Within these examples, you can consider the ViewModel as the Model as well because it actually holds all your data. In a real application, you should select a way to transfer this data to the server.