The project that will start now will be a fully server-side application. We will not use any interface framework such as AngularJS, Ember.js, and others; let's just concentrate on the express framework.
The purpose of this application is to use all the express resources and middleware to create an application following the MVC design pattern.
Middleware is basically functions that are activated by the routing layer of express. The name refers to when a route is activated until its return (from start to end). Middleware is in the middle as the name suggests. It is important to remember that the functions are executed in the order in which they were added.
In the code examples, we will be using middleware including cookie-parser
, body-parser
, and many others.
Note
You can download the code used in this book directly from the book page present at Packt Publishing Website and you can also download this chapter and all others directly from GitHub at:
Each application is given the name of the relevant chapter, so let's dive into our code now.
First off, create a new folder called chapter-01
on your machine. From now on, we will call this folder the root project folder. Before we move on and execute the command to start our project, we will see a few lines about the flags that we use with the express
command.
The command we use is express --ejs --css sass -git
, where:
express
is the default command used to create an application--ejs
means to use the embedded JavaScript template engine, instead of Jade (default)--css sass
means use SASS instead of plain CSS (default)--git
: means to add a.gitignore
file to the project
As I'm using git for version control, it will be useful to use the express option to add a .gitignore
file to my application. But I'll skip all git commands in the book.
To check all the options available from the express
framework, you can type this into your terminal/shell:
express -h
And the framework gives us all the commands available to start a project:
Usage: express [options] [dir] Options: -h, --help output usage information -V, --version output the version number -e, --ejs add ejs engine support (defaults to jade) --hbs add handlebars engine support -H, --hogan add hogan.js engine support -c, --css <engine> add stylesheet <engine> support (less|stylus|compass|sass) (defaults to plain css) --git add .gitignore -f, --force force on non-empty directory
Now, open your terminal/shell and type the following command:
express --ejs --css sass -git
The output in the terminal/shell will be as follows:
create : create : ./package.json create : ./app.js create : ./.gitignore create : ./public create : ./public/javascripts create : ./public/images create : ./public/stylesheets create : ./public/stylesheets/style.sass create : ./routes create : ./routes/index.js create : ./routes/users.js create : ./views create : ./views/index.ejs create : ./views/error.ejs create : ./bin create : ./bin/www install dependencies: $ cd . && npm install run the app: $ DEBUG=chapter-01:* npm start
As you can see in the following screenshot, the generator is very flexible and only creates the minimum necessary structure to start a project:
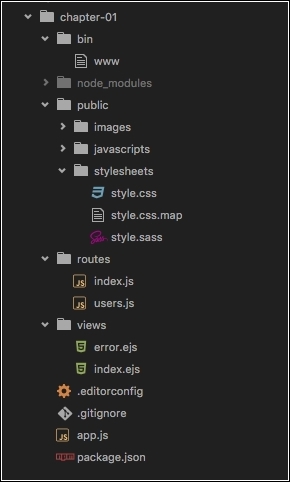
However, we will make some changes before we proceed.
Open package.json
in the root project folder and add the following highlighted lines of code:
{
"name": "chapter-01",
"description": "Build a Twitter Like app using the MVC design pattern",
"license": "MIT",
"author": {
"name": "Fernando Monteiro",
"url": "https://github.com/newaeonweb/node-6-blueprints"
},
"repository": {
"type": "git",
"url": "https://github.com/newaeonweb/node-6-blueprints.git"
},
"keywords": [
"MVC",
"Express Application",
"Expressjs"
],
"version": "0.0.1",
"private": true,
"scripts": {
"start": "node ./bin/www"
},
"dependencies": {
"body-parser": "~1.13.2",
"cookie-parser": "~1.3.5",
"debug": "~2.2.0",
"ejs": "~2.3.3",
"express": "~4.13.1",
"morgan": "~1.6.1",
"node-sass-middleware": "0.8.0",
"serve-favicon": "~2.3.0"
}
}
Even though it is not a high-priority alteration, it is considered a good practice to add this information to your project.
Now we are ready to run the project; let's install the necessary dependencies that are already listed in the package.json
file.
On the terminal/shell, type the following command:
npm install
At the end, we are ready to go!
To run the project and see the application in the browser, type the following command in your terminal/shell:
DEBUG=chapter-01:* npm start
The output in your terminal/shell will be as follows:
chapter-01:server Listening on port 3000 +0ms
You can run just npm start
, but you won't see the previous output with the port name; later in this chapter, we will fix it.
Now, just check out http://localhost:3000
. You'll see the welcome message from express.