To make our app work with methods, we need to create tree methods and move all the edits from the client to the server.
Let's place all the methods in a folder called api
on the root of the client
. The concept of Methods
is very similar to Ajax calls to the server:
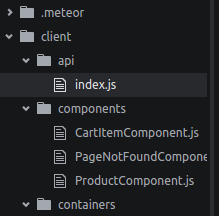
On the server, we can define them when on the startup:
Meteor.startup(() => { ….. Methods definition }); Meteor.methods({ cartInsert: function(product) { CartCollection.insert({ 'title' : product.title, 'price' : product.price, 'inventory' : product.inventory, 'quantity': 1 }); },
In Meteor.methods
, we can define all the methods.
Let's start by adding the product
method in the cart.
The cartInsert
method is the name of the method and, in the body
function, we can just copy exactly the same code that we had on the client.
To call that method on the client, we do it with Meteor.call('cartInsert', product);
.
On the client, in the api/index.js
, we create all the methods and export them...