Choosing your IDE
From a TDD perspective, different IDEs will affect your productivity. TDD implementation can be boosted by IDEs that have rich code refactoring and code generation capabilities, and selecting the right one will reduce repetitive—and potentially boring—tasks.
In the following sections, I have presented three popular IDEs with C# support: Visual Studio (VS), VS Code, and JetBrains Rider.
Microsoft VS
This chapter and the rest of the book will use VS 2022 Community Edition—this should also work with the Professional and Enterprise editions. Individual developers can use VS Community Edition for free to create their own free or paid applications. Organizations can also use it under some restrictions. For the full license and product details, visit https://visualstudio.microsoft.com/vs/community/.
If you have an earlier version of VS and do not want to upgrade, then you can have VS 2022 Community Edition installed side by side with previous versions.
Both the Windows and Mac editions of VS 2022 have the required tools to build our code and run the tests. I have done all the projects, screenshots, and instructions in this book using the Windows edition. You can download VS from https://visualstudio.microsoft.com/downloads/.
When installing VS, you will need at least the ASP.NET and web development box selected to be able to follow along with the book, as illustrated in the following screenshot:
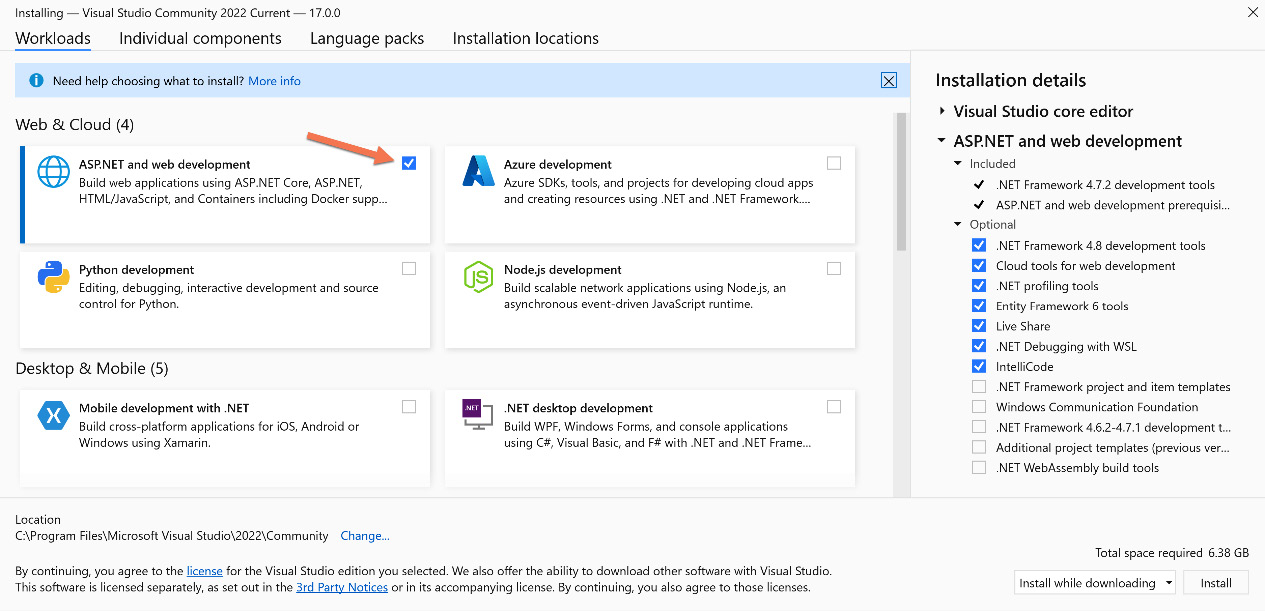
Figure 1.1 – VS installation dialog
If you have VS previously installed, you can check if ASP.NET and web development is already installed by following these steps:
- Go to Windows Settings | Apps | Apps & features.
- Search for
Visual Studio
under App list. - Select the vertical ellipsis (the three vertical dots).
- Select Modify, as shown in the following screenshot:
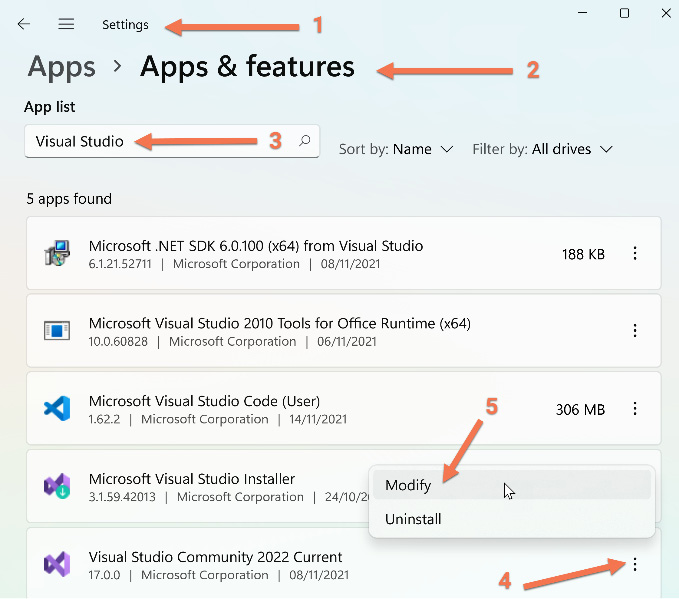
Figure 1.2 – Modifying VS installation
VS is big, as it contains plenty of components to install. Also, after installation, it is the slowest to load, in comparison with Rider and VS Code.
ReSharper
JetBrains ReSharper is a popular commercial plugin for VS. ReSharper adds multiple features to VS; however, from a TDD standpoint, we are interested in the following aspects:
- Refactoring: ReSharper adds many refactoring features that come in handy when you reach the refactoring stage of TDD.
- Code generation: Generating code with ReSharper is particularly useful when creating your unit tests first, then generating code after.
- Unit testing: ReSharper supercharges the unit testing tools in VS and has support for more unit testing frameworks.
ReSharper is a subscription-based product with a 30-day trial. I would recommend you to start first with VS without ReSharper, then add it later when you are familiar with the capabilities of VS so that you recognize the benefits of adding ReSharper.
Note
Each new release of VS adds additional code refactoring and code generation capabilities similar to those of ReSharper. However, as of now, ReSharper has more advanced features.
In this book, the discussion on ReSharper will be limited to this section. You can download ReSharper here: https://www.jetbrains.com/resharper/.
JetBrains Rider
JetBrains, the company behind Rider, is the same company behind the popular ReSharper VS plugin. If you have chosen JetBrains Rider for your .NET development, then you have all the features that are required in this book. Rider has the following:
- A powerful unit test runner that competes with Test Explorer of VS
- Feature-rich code refactoring and code generation capabilities with more advanced features than those of VS 2022
The aforementioned points are crucial for building a system TDD-style; however, I have chosen VS for this book rather than Rider. Although the instructions in this book are meant for VS 2022, they can be applied to Rider, taking into consideration that Rider has a different menu system and shortcuts.
Note
VS .NET (VS release with .NET support) was released in February 2002, while Rider is more recent and was released in August 2017; so, VS is more established between .NET developers. I have nominated VS for this book over Rider for this reason.
You can download Rider here: https://www.jetbrains.com/rider/.
VS Code
If you are a fan of VS Code, you will be pleased to know that Microsoft added native support for visual unit testing (which is essential for TDD) in July 2021, with the version 1.59 release.
VS Code is a lightweight IDE—it has good native refactoring options and a bunch of third-party refactoring plugins. The simplicity and elegance of VS Code attract many TDD practitioners, but the available C# features—especially those used in TDD—are not as advanced as those of VS or Rider.
I will be using VS in this book, but you can adapt the examples to VS Code where relevant. To download VS Code, you can visit https://visualstudio.microsoft.com/downloads/.
.NET and C# versions
VS 2022 comes with .NET 6 and C# 10 support. This is what we will be using for the purposes of this chapter and the rest of the book.
I initiated a small poll to gather some public opinion in my LinkedIn group—you can see the results here:
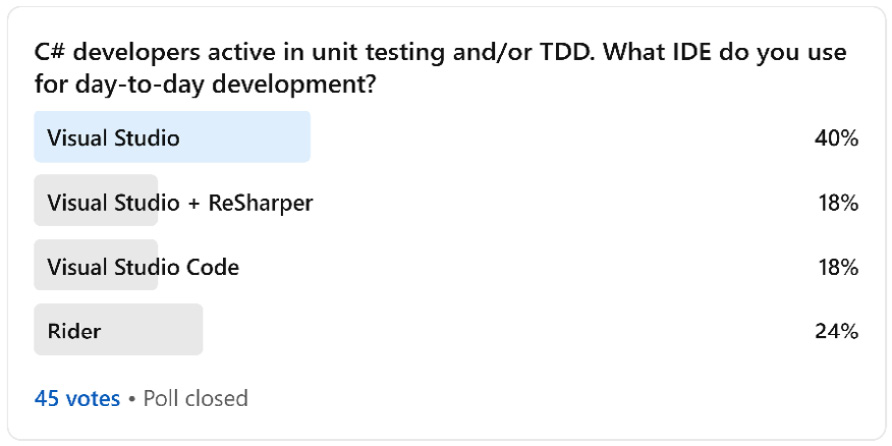
Figure 1.3 – LinkedIn IDE poll results
As you can see, VS has the highest usage of 58%, with 18% who use the ReSharper plugin with VS, then Rider comes second at 24%, then in third place comes VS Code with 18%. However, given that this is only 45 votes, it is meant to give you an indication and would definitely not reflect the market.
Picking the right IDE is a debatable subject between developers. I know that every time I ask a developer practicing TDD about their chosen IDE, they would swear by how good their IDE is! In conclusion, use the IDE that makes you more productive.