Installing sqlc
We have defined the database structure so now it’s time to talk a bit more about the tool that we are going to be using called sqlc. sqlc is an open source tool that generates type-safe code from SQL; this allows developers to focus on writing SQL and leave the Go code to sqlc. This reduces the development time, as sqlc takes care of the mundane coding of queries and types.
The tool is available at https://github.com/kyleconroy/sqlc. The tool helps developers focus on writing the SQL code that is needed for the application and it will generate all the relevant code needed for the application. This way, developers will be using pure Go code for database operations. The separation is clean and easily trackable.
The following diagram shows the flow that developers normally adopt when using the tool at a high level.
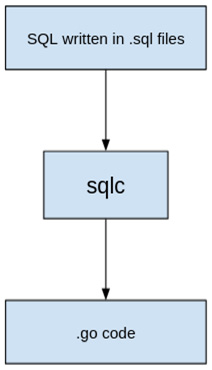
Figure 1.3 – Flow to use sqlc to generate Go code
All SQL code will be written in .sql
files, which will be read and converted by the sqlc tool into the different Go code.
Download and install SQL binary by using the following command:
go install github.com/kyleconroy/sqlc/cmd/sqlc@latest
Make sure your path includes the GOPATH/bin
directory – for example, in our case, our path looks like the following:
…:/snap/bin:/home/nanik/goroot/go1.16.15/go/bin:/home/nanik/go/bin
If you don’t have GOPATH
as part of the PATH
environment variable, then you can use the following command to run sqlc:
$GOPATH/bin/sqlc Usage: sqlc [command] Available Commands: compile Statically check SQL for syntax and type errors completion Generate the autocompletion script for the specified shell generate Generate Go code from SQL help Help about any command init Create an empty sqlc.yaml settings file upload Upload the schema, queries, and configuration for this project version Print the sqlc version number Flags: -x, --experimental enable experimental features (default: false) -f, --file string specify an alternate config file (default: sqlc.yaml) -h, --help help for sqlc
Use "sqlc [command] --help"
for more information about a command.
At the time of writing, the latest version of sqlc is v1.13.0.
Now that we have installed the tool and understand the development workflow that we will be following when using the tool, we will look at how to use the tool for our application.