Hosting models
As we mentioned earlier, Razor components are the building blocks of Blazor applications. Where those Razor components are hosted varies depending on the hosting model.
Blazor has three different hosting models:
- Blazor Server
- Blazor Hybrid
- Blazor WebAssembly
The first hosting model that Microsoft released was Blazor Server. In this hosting model, the Razor components are executed on the server. The second hosting model that Microsoft released, and the topic of this book, is Blazor WebAssembly. In this hosting model, the Razor components are executed on the browser using WebAssembly. The newest hosting model is Blazor Hybrid. Blazor Hybrid allows you to build native client apps by hosting the Razor components in an embedded Web View control.
Each hosting model has its own advantages and disadvantages. However, they all rely upon the same underlying architecture. Therefore, it is possible to write and test your code independently of the hosting model.
The major differences between the hosting models concern where the code executes, latency, security, payload size, and offline support. The one thing that all the hosting models have in common is the ability to execute at near native speed.
Blazor Server
As we just mentioned, the Blazor Server hosting model was the first hosting model released by Microsoft. It was released as part of the .NET Core 3 release in September 2019.
The following diagram illustrates the Blazor Server hosting model:
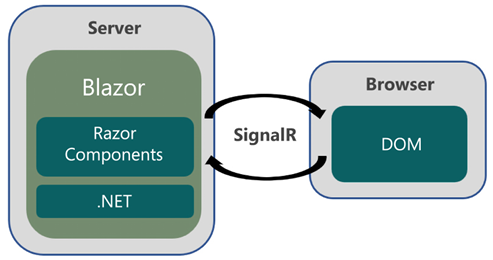
Figure 1.1: Blazor Server
In this hosting model, the web app is executed on the server and only updates to the UI are sent to the client’s browser. The browser is treated as a thin client and all the processing occurs on the server. Therefore, this model requires a constant connection to the server. When using Blazor Server, UI updates, event handling, and JavaScript calls are all handled over an ASP.NET Core SignalR connection.
IMPORTANT NOTE
SignalR is a software library that allows the web server to push real-time notifications to the browser. Blazor Server uses it to send UI updates to the browser.
Advantages of Blazor Server
There are a few advantages of using Blazor Server versus using Blazor WebAssembly. However, the key advantage is that everything happens on the server. Since the web app runs on the server, it has access to everything on the server. As a result, security and data access are simplified. Also, since everything happens on the server, the assemblies (DLLs) that contain the web app’s code remain on the server.
Another advantage of using Blazor Server is that it can run on thin clients and older browsers, such as Internet Explorer, that do not support WebAssembly.
Finally, the initial load time for the first use of a web app that is using Blazor Server can be much less than that of a web app that is using Blazor WebAssembly because there are much fewer files to download.
Disadvantages of Blazor Server
The Blazor Server hosting model has several disadvantages versus Blazor WebAssembly. The biggest disadvantage is that the browser must maintain a constant connection to the server. Since there is no offline support, every single user interaction requires a network roundtrip. As a result of all these roundtrips, Blazor Server web apps have higher latency than Blazor WebAssembly web apps and can feel sluggish. Also, network interruptions may cause a client to unexpectedly disconnect.
Another disadvantage of using Blazor Server is that it relies on SignalR for every single UI update. Microsoft’s support for SignalR has been improving, but it can be challenging to scale. When too many concurrent connections to the server are open, connection exhaustion can prevent other clients from establishing new connections.
Finally, a Blazor Server web app must be served from an ASP.NET Core server.
Blazor Hybrid
The Blazor Hybrid hosting model is the most recent hosting model released by Microsoft. It was released as part of the .NET 6 release in November 2021.
The following diagram illustrates the Blazor Hybrid hosting model:
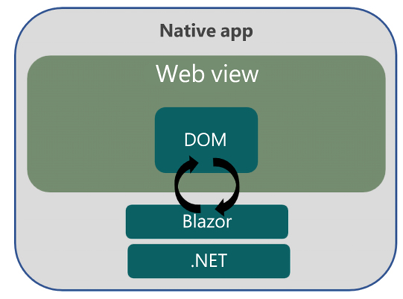
Figure 1.2: Blazor Hybrid
In this model, the Razor components run in an embedded Web View control. Blazor Hybrid apps include Windows Forms, WPF, and .NET MAUI apps. By using the Blazor Hybrid hosting model, your apps have full access to the native capabilities of the devices that you choose to target.
Advantages of Blazor Hybrid
The advantage of using this model versus Blazor WebAssembly is that it does not require WebAssembly. Also, since the component’s C# code is executed in the host process, the Blazor Hybrid apps have access to the native capabilities of the device.
Disadvantages of Blazor Hybrid
The major disadvantage of using Blazor Hybrid is that they are hosted in a Web View component in the native app. So, the developer must know how to develop each type of native client app that they want to target. Another disadvantage is that they usually require a server to deliver the app. In contrast, a Blazor WebAssembly app can be downloaded as a set of static files.
Blazor WebAssembly
The Blazor WebAssembly hosting model is the topic of this book.
Blazor WebAssembly 3.2.0 was released in May 2020. Blazor WebAssembly in .NET 5 was released as part of the .NET 5.0 release in November 2020. ASP.NET Core Blazor was released as part of the .NET 6.0 release in November 2021, and it is a long-term support (LTS) release. The most recent release of Blazor WebAssembly was released as part of the .NET 7 release in November 2022 This book will be using Blazor WebAssembly in .NET 7 for all the projects.
TIP
LTS releases are supported by Microsoft for at least 3 years after their initial release. Blazor WebAssembly in .NET 7 is a current release rather than an LTS release. Current releases get free support and patches for 18 months. We recommend that if you are starting a new project with Blazor WebAssembly, you should use the most recent release.
The following diagram illustrates the Blazor WebAssembly hosting model:
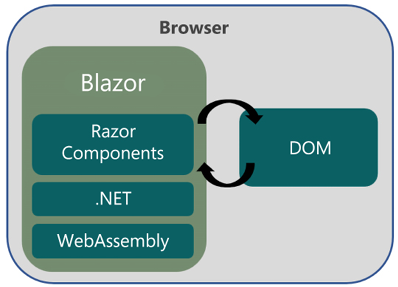
Figure 1.3: Blazor WebAssembly
In this hosting model, the web app is executed on the browser. For both the web app and the .NET runtime to run on the browser, the browser must support WebAssembly. WebAssembly is a web standard supported by all modern browsers, including mobile browsers. While Blazor WebAssembly itself does not require a server, the web app may require one for data access and authentication.
In the past, the only way to run C# code on the browser was to use a plugin, such as Silverlight. Silverlight was a free browser plugin provided by Microsoft. It was very popular until Apple decided to forbid the use of any browser plugins on iOS. As a result of Apple’s decision, Silverlight was abandoned by Microsoft.
IMPORTANT NOTE
Blazor does not rely on plugins or recompiling the code into other languages. Instead, it is based on open web standards and is supported by all modern browsers, including mobile browsers.
Advantages of Blazor WebAssembly
Blazor WebAssembly has many advantages. First, since it runs on the browser, it relies on client resources instead of server resources. Therefore, the processing is offloaded to the client. Also, unlike Blazor Server, there is no latency due to each UI interaction requiring a roundtrip to the server.
Blazor WebAssembly can be used to create a Progressive Web App (PWA). A PWA is a web app that looks and feels like a native application. They provide offline functionality, background activity, native API layers, and push notifications. They can even be listed in the various app stores. By configuring your Blazor WebAssembly app as a PWA, your app can reach anyone, anywhere, on any device with a single code base. For more information on creating a PWA, refer to Chapter 6, Building a Weather App as a Progressive Web App (PWA).
Finally, a Blazor WebAssembly web app does not rely on an ASP.NET Core server. In fact, it is possible to deploy a Blazor WebAssembly web app via a Content Delivery Network (CDN).
Disadvantages of Blazor WebAssembly
To be fair, there are some disadvantages when using Blazor WebAssembly that should be considered. For starters, when using Blazor WebAssembly, the .NET runtime, the dotnet.wasm
file, and your assemblies need to be downloaded to the browser for your web app to work. Therefore, the first time you run a Blazor WebAssembly application it usually takes longer to initially load than an identical Blazor Server application. However, there are strategies that you can use to speed up the initial load time, such as deferring the loading of some of the assemblies until they are needed. Also, this is only an issue during the initial load since subsequent runs of the application will access the files from a local cache.
Another disadvantage of Blazor WebAssembly web apps is that they are only as powerful as the browser that they run on. Therefore, thin clients are not supported. Blazor WebAssembly can only run on a browser that supports WebAssembly. Luckily, due to a significant amount of coordination between the World Wide Web Consortium (W3C) and engineers from Apple, Google, Microsoft, and Mozilla, all modern browsers support WebAssembly.
Hosting model differences
The following table indicates the differences between the three models:
Blazor WebAssembly |
Blazor Hybrid |
Blazor Server |
|
Native execution speed |
X |
X |
X |
Executes on client |
X |
X |
|
Executes on server |
X |
||
Low latency after initial load time |
X |
X |
|
Fast initial load time |
X |
||
Offline support |
X |
X |
|
Does not require a server |
X |
||
Requires constant connection to a server |
X |
||
Can build PWAs |
X |
||
Assemblies sent to client |
X |
X |
|
Assembles remain on server |
X |
||
Can access native client features |
X |
||
Requires WebAssembly |
X |
||
Requires SignalR |
X |
||
Can run on thin clients |
X |
Table 1.1: Hosting model differences
The Blazor framework provides three different hosting models, Blazor Server, Blazor Hybrid, and Blazor WebAssembly. A Blazor Server web app runs on the server and uses SignalR to serve the HTML to the browser. A Blazor Hybrid web app runs in a Web View control in the native app. A Blazor WebAssembly web app runs directly in the browser using WebAssembly. They each have their advantages and disadvantages. However, if you want to create interactive, highly responsive, native-like web apps that can work offline, we recommend Blazor WebAssembly. Let’s learn more about WebAssembly in the next section.