Initializing and configuring FastAPI
Learning how to create applications using FastAPI is easy and straightforward. A simple application can be created just by creating a main.py
file inside your /ch01
project folder. In our online academic discussion forum, for instance, the application started with this code:
from fastapi import FastAPI app = FastAPI()
This initializes the FastAPI framework. The application needs to instantiate the core FastAPI
class from the fastapi
module and use app
as the reference variable to the object. Then, this object is used later as a Python @app
decorator, which provides our application with some features such as routes, middleware, exception handlers, and path operations.
Important note
You can replace app
with your preferred but valid Python variable name, such as main_app
, forum
, or myapp
.
Now, your application is ready to manage REST APIs that are technically Python functions. But to declare them as REST service methods, we need to decorate them with the appropriate HTTP request method provided by the path operation @app
decorator. This decorator contains the get()
, post()
, delete()
, put()
, head()
, patch()
, trace()
, and options()
path operations, which correspond to the eight HTTP request methods. And these path operations are decorated or annotated on top of the Python functions that we want to handle the request and response.
In our specimen, the first sample that the REST API created was this:
@app.get("/ch01/index") def index(): return {"message": "Welcome FastAPI Nerds"}
The preceding is a GET
API service method that returns a JSON
object. To locally run our application, we need to execute the following command:
uvicorn main:app --reload
This command will load the forum application to the uvicorn live server through the application’s main.py
file with FastAPI object referencing. Live reload is allowed by adding the --reload
option, which enables the restart of the development server whenever there are changes in the code.
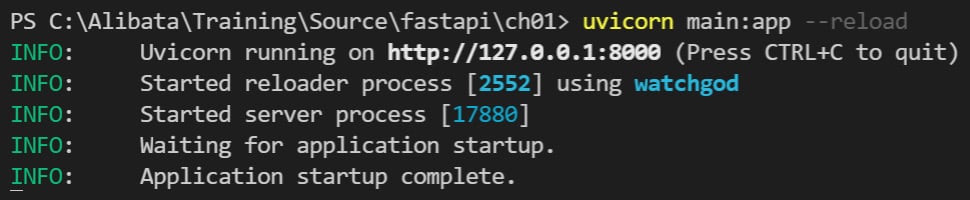
Figure 1.1 – The uvicorn console log
Figure 1.1 shows that uvicorn uses localhost
to run the application with the default port 8000
. We can access our index page through http://localhost:8000/ch01/index
. To stop the server, you just need to press the Ctrl + C keyboard keys.
After running our first endpoint, let us now explore how to implement the other types of HTTP methods, namely POST
, DELETE
, PUT
, and PATCH
.