To keep with the example, we will build a list of products, with their prices and an Add button. Now create a controller in application/controllers
, call it shop.php
, and for the moment, only add the following code:
<?php class Shop extends Controller { function Shop() { parent::Controller(); } function index() { $this->load->view('home'); } }
We are only defining the class
, the constructor, and creating an index
method, which calls the view. This view will be the place where we build our list of products, but for now create the file in application/views/home.php
, and place some simple content in it:
<h1>This is our shop home page</h1>
If we want to check that everything is working fine, we only need to go to http://localhost/codeigniterc/index.php/shop
.
Note
As you can see, this time we have the index.php
part in the URL. This is so that we can see another way of working, not that we like having it, but just to see how fast it is to start working with CI. We have just downloaded it and configured the database connection, we don't need anything else.
Let's continue; we will now create our shop's list of products. For this to be easier, we will make use of the form
helper, so load it in the controller, application/controllers/shop.php
, in the index
function:
function index() { $this->load->helper('form'); $this->load->view('home'); }
As we have loaded the form
helper, we are able to use it in our view; let's add some code to it. Remember it was in application/views/home.php
:
<h1>This is our shop home page</h1> <table border="2"> <tr> <td> <?php //Here we are opening the form echo form_open('shop/add'); //Adding some hidden values echo form_hidden('id', '1'); echo form_hidden('price', 12); echo form_hidden('name', 'Vegetable 1'); //Echoing our product name echo "Vegetable 1"; ?> </td> <td> <?php //Creating an input box so our users can write how many of our //products do they want echo "Quantity ".form_input('qty', 1); ?> </td> <td> 12 $ / u </td> <td> <?php //And then we create the buy button and close the form echo form_submit('buy', 'Buy!!!'); echo form_close(); ?> </td> </tr> <tr> <td> <?php //And start another form for the next product echo form_open('shop/add'); echo form_hidden('id', '2'); echo form_hidden('price', 5); echo form_hidden('name', 'Vegetable 2'); echo "Vegetable 2"; ?> </td> <td> <?php echo "Quantity ".form_input('qty', 1); ?> </td> <td> <?php echo form_submit('buy', 'Buy!!!'); echo form_close(); ?> </td> </tr> </table>
Now, if we go to the URL http://localhost/codeigniterc/index.php/shop
, we will see a table showing our products. It is a very simple table, but see the idea behind it. We have created two forms, one for each product, so when our visitors click on the Buy button, the information contained in the form is sent to the controller.
The field names we have used are not arbitrary, the cart
library expects to receive this data. In order to insert product data in the session, we need:
id
is a unique identifier for the productqty
is the quantity of products of this type added to the shopping cartprice
is the price of the productname
is, of course, the name of the product
Note
However, we can add our own keys to the array if we want to, but remember two things:
There is an options
array, in which we can place additional data in the form of an array. For example:
'options' => array('class' => 'A', 'type' => 'some type')
Also there are two reserved words, rowid
and subtotal
, which are used internally by the library, so it's better not to use them.
The cart
library expects to receive all this data. We can name the form
fields in any way and create variables in the controller with the required name, but that would be a bit confusing.
Now back to the controller; let's see what we can do with this data. Create a new function in application/controllers/shop.php
and call it add
:
function add() { $data = array( 'id' => $_POST['id'], 'qty' => $_POST['qty'], 'price' => $_POST['price'], 'name' => $_POST['name'] ); $this->cart->insert($data); redirect('shop/index/', 'refresh'); }
In this function we create an array with the received data. Remember that the cart
library expects these variables, hence, they are all required, so changing them may cause failure in adding this data to the session.
After the array is prepared, we call the cart->insert
method, passing the array as a parameter, and we are done with inserting the data. Now we can redirect to a view of the cart, but as we don't have it yet, we will return to the index page.
Don't worry if you have not seen the load->library
method or the load->helper
one; we have put them in the class constructor, as we will need them throughout all the methods of our class:
function Shop() { parent::Controller(); $this->load->library('cart'); $this->load->helper('url'); }
We are loading the necessary libraries and helpers, and as we load them in the class constructor, they will be available for each function in our class.
Note
The cart
library loads the Session
class automatically, so we don't need to load the Session
class too.
Now you can go to http://localhost/codeigniterc/index.php/shop/index
and give it a try. It should look something similar to:
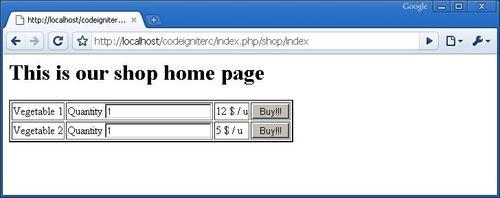
When you click on the Buy button, you will be returned to this page again. Don't worry, if no errors are shown, all has gone well. In the next section we will see how to show the cart.