Pagination is the act of collating large amounts of data and presenting it to the user in small, easy-to-read sections or pages.
With a combination of jQuery, JavaScript functions, and event handlers, we are able to easily collate and present data to the user in pages.
To create a paginated set of data, we first need some data to paginate and then a location to place the paginated data. Use the following code to create an HTML page:
<!DOCTYPE html> <html> <head> <title>Chapter 1 :: DOM Manipulation</title> <script src="jquery.min.js"></script> <script> var animals = [ { id: 1, name: 'Dog', type: 'Mammal' }, { id: 2, name: 'Cat', type: 'Mammal' }, { id: 3, name: 'Goat', type: 'Mammal' }, { id: 4, name: 'Lizard', type: 'Reptile' }, { id: 5, name: 'Frog', type: 'Amphibian' }, { id: 6, name: 'Spider', type: 'Arachnid' }, { id: 7, name: 'Crocodile', type: 'Reptile' }, { id: 8, name: 'Tortoise', type: 'Reptile' }, { id: 9, name: 'Barracuda', type: 'Fish' }, { id: 10, name: 'Sheep', type: 'Mammal' }, { id: 11, name: 'Lion', type: 'Mammal' }, { id: 12, name: 'Seal', type: 'Mammal' } ]; var pageSize = 4; var currentPage = 1; var pagedResults = []; var totalResults = animals.length; $(function(){ }); </script> </head> <body> <ul id="list"></ul> <button class="previous"><< Previous</button> <button class="next">Next >></button> </body> </html>
Within the JavaScript in this page, we have declared a large array of objects named animals
, which represents a set of animals. Below this array, we have declared four more variables, which we will require in order to paginate the animals
array:
pageSize
: This indicates the amount of results we wish to be held on a single pagecurrentPage
: This indicates the current page that is being displayedpagedResults
: This indicates an array that contains a section of theanimals
array, which represents the pagetotalResults
: This indicates the number of objects within theanimals
array; in this case,12
To create a dynamic list with pages, perform each of the following steps:
Directly after
$(function(){});
but still within the<script></script>
tags, add the following JavaScript function:function updateList() { //Grab the required section of results from the animals list var end = (currentPage * pageSize); var start = (end - pageSize); pagedResults = animals.slice(start, end); //Empty the list element before repopulation $('#list').empty(); //Disable the previous button if we are on the first page if (currentPage <= 1) { $('.previous').prop("disabled", true); } //Enable the previous button if we are not on the first page else { $('.previous').prop("disabled", false); } //Disable the next button if there are no more pages if ((currentPage * pageSize) >= totalResults) { $('.next').prop("disabled", true); } //Enable the next button if there are results left to page else { $('.next').prop("disabled", false); } //Loop through the pages results and add them to the list $.each(pagedResults, function(index, obj){ $('#list').append("<li><strong>" + obj.name + "</strong> (" + obj.type + ")</li>"); }); }
Add the following JavaScript within
$(function(){});
in the preceding HTML page://Populate the list on load updateList(); $('.next').click(function(){ //Only increase the current page if there are enough results if ((currentPage * pageSize) <= totalResults) currentPage++; updateList(); }); $('.previous').click(function(){ //Only decrease the current page if it is currently greater than 1 if (currentPage > 1) currentPage--; updateList(); });
Although pagination can seem quite complicated, in principle, it is simple. We will need to use jQuery's click()
function to listen for click events on the next and previous buttons. When these buttons are pressed, the currentPage
variable is either incremented or decremented based on which button is clicked. After this, the updateList()
function takes the currentPage
value, works out which section of data it needs to use from the animals
array, populates the pagedResults
array with this data, and then loads these results into the HTML list element, #list
.
Additionally, we will need to disable the next or previous buttons depending on which page the user is currently viewing. If they are currently viewing the first page, the previous button can be disabled using jQuery's
prop()
function to set its disabled
property to true
. If the user is viewing the last page (which our function can work out using the totalResults
, currentPage
, and pageSize
variables), we need to disable the next button.
//Populate the list on load updateList(); $('.next').click(function(){ //Only increase the current page if there are enough results if ((currentPage * pageSize) <= totalResults) currentPage++; updateList(); }); $('.previous').click(function(){ //Only decrease the current page if it is currently greater than 1 if (currentPage > 1) currentPage--; updateList(); });
To expand on the well-commented code, the first thing we do is call a function named updateList()
, which we will look at a little later in this recipe.
Next, we attach a click event handler to the next button by passing a callback function as an argument. For this event function, we are able to specify some code to be executed every time the next button is clicked. The code we specify increments the currentPage
variable by 1
. If there is another page of data available, it works this out by forming the ((currentPage * pageSize) <= totalResults)
condition as part of the if
statement.
Finally, as a part of this click function, we call the previously mentioned updateList()
function.
We apply the same logic to the previous button also, except that we are decrementing the currentPage
value if the current page is greater than one; hence, there is a page to go back to.
Below $(function(){});
but still within the <script></script>
tags, add the following JavaScript function to your HTML page:
function updateList() { //Grab the required section of results from the animals list var end = (currentPage * pageSize); var start = (end - pageSize); pagedResults = animals.slice(start, end); //Empty the list element before repopulation $('#list').empty(); //Disable the previous button if we are on the first page if (currentPage <= 1) { $('.previous').prop("disabled", true); } //Enable the previous button if we are not on the first page else { $('.previous').prop("disabled", false); } //Disable the next button if there are no more pages if ((currentPage * pageSize) >= totalResults) { $('.next').prop("disabled", true); } //Enable the next button if there are results left to page else { $('.next').prop("disabled", false); } //Loop through the pages results and add them to the list $.each(pagedResults, function(index, obj){ $('#list').append("<li><strong>" + obj.name + "</strong> (" + obj.type + ")</li>"); }); }
To maintain good practices, the code is well-commented once again. The first action that this function performs is calculating which section of the animals
array it needs to use. Once it has calculated the start and end values, which are index values for the animals
array (for example, 0
to 4
for page one), it uses JavaScript's slice()
function to copy this data from the animals
array to the pagedResults
array.
Note
Be careful to not use the similar, JavaScript's
.splice()
function as this will actually remove the data from the animals
array as well as copy it to the pagedResults
array. Additionally, slice()
takes two arguments: the first is a zero-indexed number stating the start location of the array (that is, 0
is the beginning), and the second argument is not the location within the array but the number of elements from the starting point.
With the required results stored in the pagedResults
array, it uses jQuery's empty()
function to empty the unordered list, #list
, of any data. This is to prepare the list for repopulation. Otherwise, when the next or previous button is clicked and the updateList()
function is run, the results will just get appended to the end of the current list and not replaced.
The next section of code is to determine if the next and previous buttons need to be either disabled or enabled. We can work out whether the previous buttons need to be disabled by putting the condition (currentPage <= 1)
, which simply checks to see if the current page is less than or equal to one; if it is, we need to disable the previous button; otherwise, we need to enable it. This is done using jQuery's prop()
function, which allows us to manipulate the properties on selected elements; here, we change the disabled
property to either true
or false
. We can determine whether we need to disable the next button using ((currentPage * pageSize) >= totalResults)
, which calculates whether there are enough objects within the animals
array to create the next page; if there are not, we disable the button, but if there are, we enable it.
Finally, we use jQuery's $.each()
function to iterate through each of the objects within the pagedResults
array and append a list element with the data from each object to the unordered list on the page.
If you open the HTML page within the browser, you should see a similar page to the one illustrated as follows:
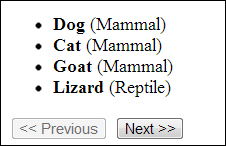
On page load, the list is populated with the first page of results, as currentPage
is set to 1
and the
updateList()
function is also set to run on page load, which disables the previous button.