MultiPolyline and MultiPolygon layers allow you to combine multiple polylines and polygons. If you want to create group layers of different types, such as a marker layer with a circle, you can use a layer group or a feature group.
A layer group allows you to add multiple layers of different types to the map and manage them as a single layer. To use a layer group, you will need to define several layers:
var marker=L.marker([35.10418, -106.62987]).bindPopup("I am a Marker"); var marker2=L.marker([35.02381, -106.63811]).bindPopup("I am Marker 2"); var polyline=L.polyline([[35.10418, -106.62987],[35.19738, -106.875],[35.07946, -106.80634]], {color: 'red',weight:8}).bindPopup("I am a Polyline");
The preceding code creates two markers and a polyline. Note that you will not use the addTo(map)
function after creating the layers, like you did in the previous examples. You will let the layer group handle adding the layer to the map. A layer group requires a set of layers as a parameter:
var myLayerGroup=L.layerGroup([marker, polyline]).addTo(map);
In the previous code, an instance of L.layerGroup()
was created as myLayerGroup
. The layers passed as a parameter were marker
and polyline
. Finally, the layer group was added to the map. The earlier code shows three layers, but only two were added to the layer group. To add layers to a layer group without passing them as a parameter during creation, you can use the layer group addLayer()
method. This method takes a layer as a parameter, as shown in the following code:
myLayerGroup.addLayer(marker2);
Now, all three layers have been added to the layer group and are displayed on the map. The following screenshot shows the layer group added to the map:
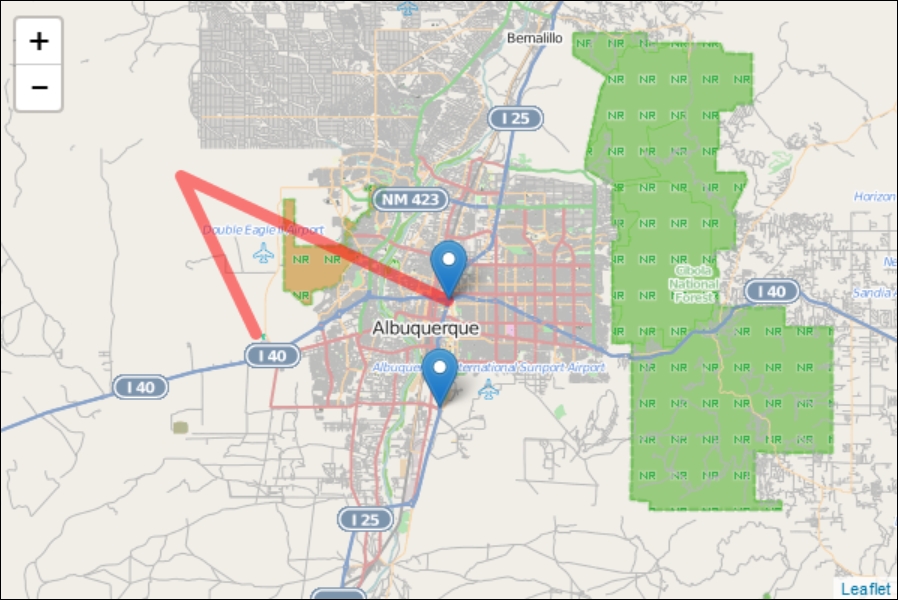
If you want to remove a layer from the layer group, you can use the removeLayer()
method and pass the layer name as a parameter:
myLayerGroup.removeLayer(marker);
If you remove a layer from the group, it will no longer be displayed on the map because the addTo()
function was called for the layer group and not the individual layer. If you want to display the layer but no longer want it to be part of the layer group, use the removeLayer()
function, as shown in the preceding code, and then add the layer to the map as shown in the earlier examples. This is shown in the following code:
marker.addTo(map);
All style options and pop ups need to be assigned to the layer when it is created. You cannot assign a style or pop ups to a layer group as a whole. This is where feature groups can be used.
A feature group is similar to a layer group, but extends it to allow mouse events and includes the bindPopup()
method. The constructor for a feature group is the same as the layer group: just pass a set of layers as a parameter. The following code displays an example of a feature group:
VarmyfeatureGroup=L.featureGroup([marker, marker2, polyline]) .addTo(map).setStyle({color:'purple',opacity:.5}) .bindPopup("We have the same popup because we are a group");
In the preceding code, the layers added are the same three that you added in the layer group. Since the feature group extends the layer group, you can assign a style and pop up to all of the layers at once. The following screenshot shows the feature group added to the map:
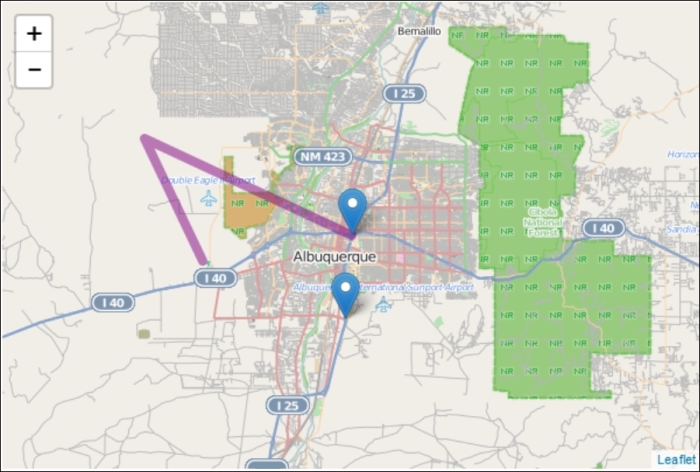
When you created the polyline in the previous example, you set the color to red
. Note now that since you passed style information to the feature group by setting the color to purple
, the polyline took the information from the feature group and discarded its original settings. If you removed the polyline from the feature group, it will be removed from the map as well. If you try to add the polyline to the map using addTo()
, as in the previous examples, it will still be purple and have the new pop up. The markers are still blue even though you passed style information to the feature group. The
setStyle()
method only applies to layers in the feature group that have a setStyle()
method. Since a polyline extends the path
class, it has a setStyle()
method. The markers do not have a setStyle()
method, so their color did not change.