Let's start by creating a new .NET Core 3.0 WinForms application and later we'll also see how to upgrade an old .NET Core WinForms app to 3.0, so that we can show both ways of achieving this.
Using Visual Studio 2019, we will create a simple Windows Forms App template project. You can call the application anything you like, but I called mine eBookManager:
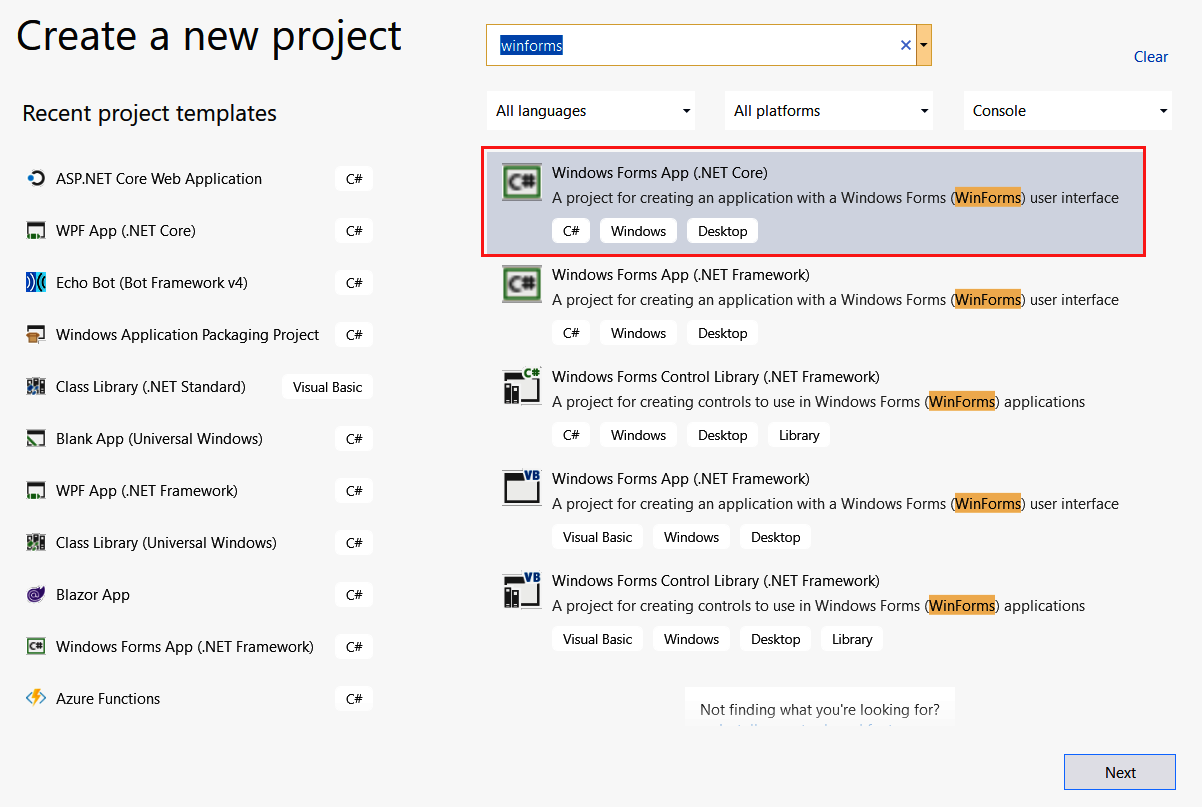
The process of creating a new project has changed slightly in Visual Studio 2019, and you are required to select the type of application, followed by where to create it:
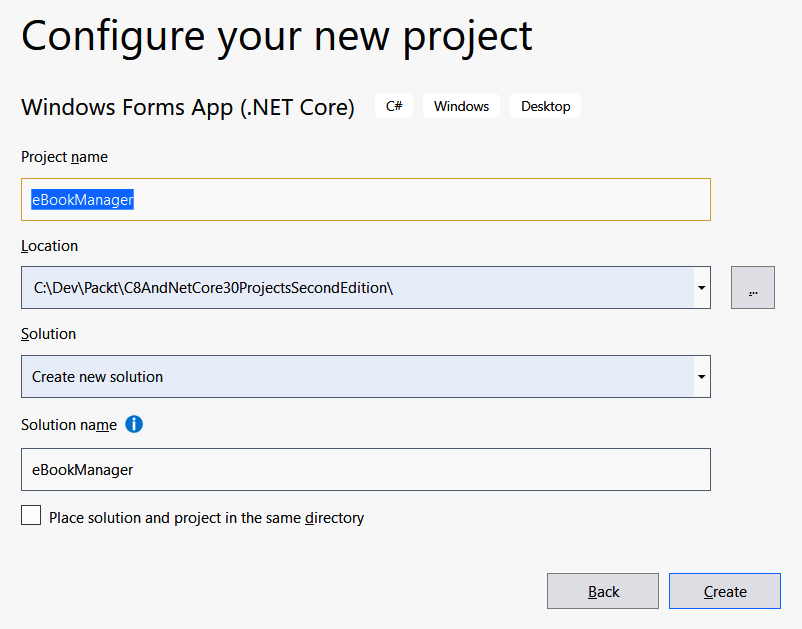
The project will be created and will look as follows:
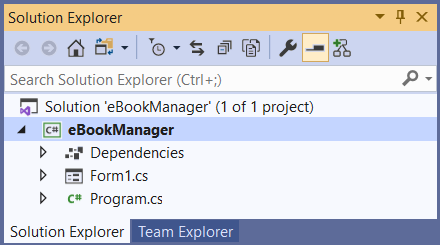
Our solution needs a class library project to contain the classes that drive the eBookManager application. In .NET Core 3.0, we have the option of creating either a .NET Core class library or a .NET Standard class library.
.NET Standard is a bit of a strange concept. In and of itself, it is not a technology, but a contract; creating a .NET Standard class library simply prevents you from using anything that is .NET Core—(or framework—) specific, and does not adhere to .NET Standard. The following document illustrates the .NET Standard versions, and what releases of Core and Framework support them: https://github.com/dotnet/standard/blob/master/docs/versions.md.
Add a new Class Library (.NET Standard) project to your solution and call it eBookManager.Engine:
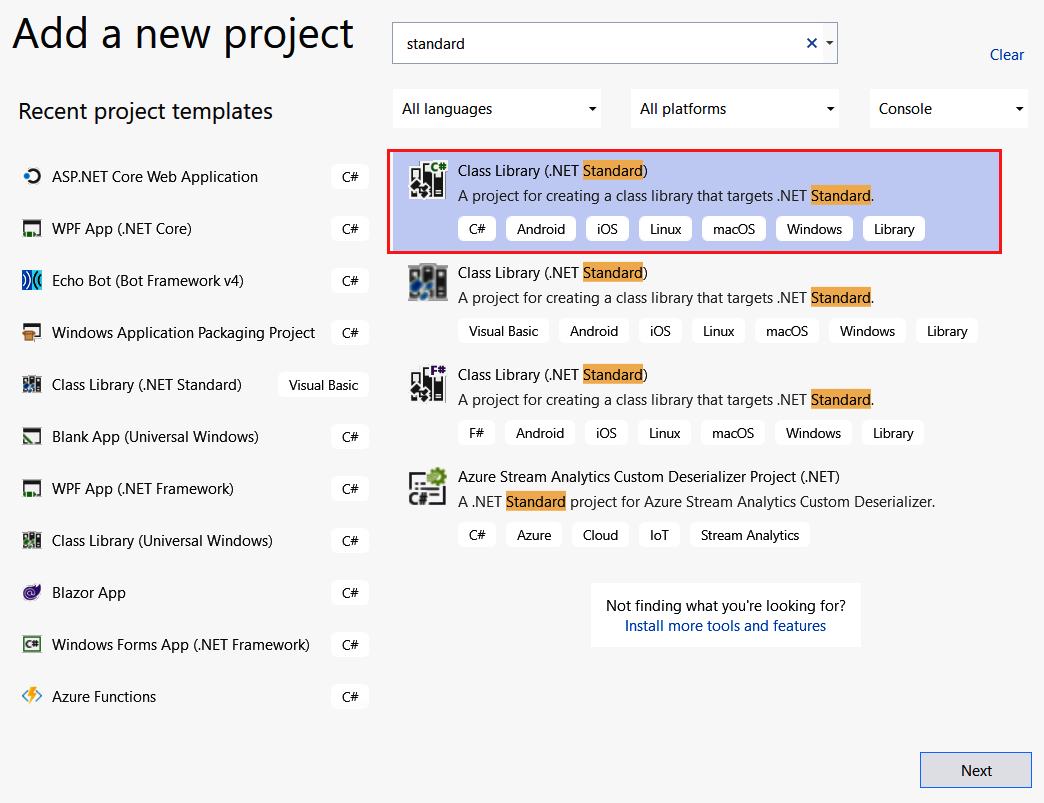
A class library project is added to the solution with the default class name. Change this class to Document:
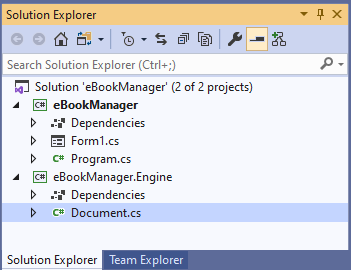
The Document class will represent a single eBook. When thinking of a book, we can have multiple properties representing a single book, but that would be representative of all books. An example of this would be the author. All books must have an author; otherwise, they would not exist.
The properties I have added to the class are merely my interpretation of what might represent a book. Feel free to add additional code to make this your own. Open the Document.cs file and add the following code to the class:
public class Document
{
public string Title { get; set; }
public string FileName { get; set; }
public string Extension { get; set; }
public DateTime LastAccessed { get; set; }
public DateTime Created { get; set; }
public string FilePath { get; set; }
public string FileSize { get; set; }
public string ISBN { get; set; }
public string Price { get; set; }
public string Publisher { get; set; }
public string Author { get; set; }
public DateTime PublishDate { get; set; }
public DeweyDecimal Classification { get; set; }
public string Category { get; set; }
}
You will notice that I have included a property called Classification of type DeweyDecimal. We have not added this class yet and will do so next. To the eBookManager.Engine project, add a class called DeweyDecimal. If you don't want to go to this level of classification for your eBooks, you can leave this class out. I have included it for the sake of completeness. We're going to introduce a neat little feature that's been in Visual Studio for some time: if you hover over the DeweyDecimal text, you'll see a lightbulb appear (you can bring this menu up manually by holding the Ctrl key and the dot key (Ctrl + .). I will be using this shortcut profusely throughout the rest of the book!):
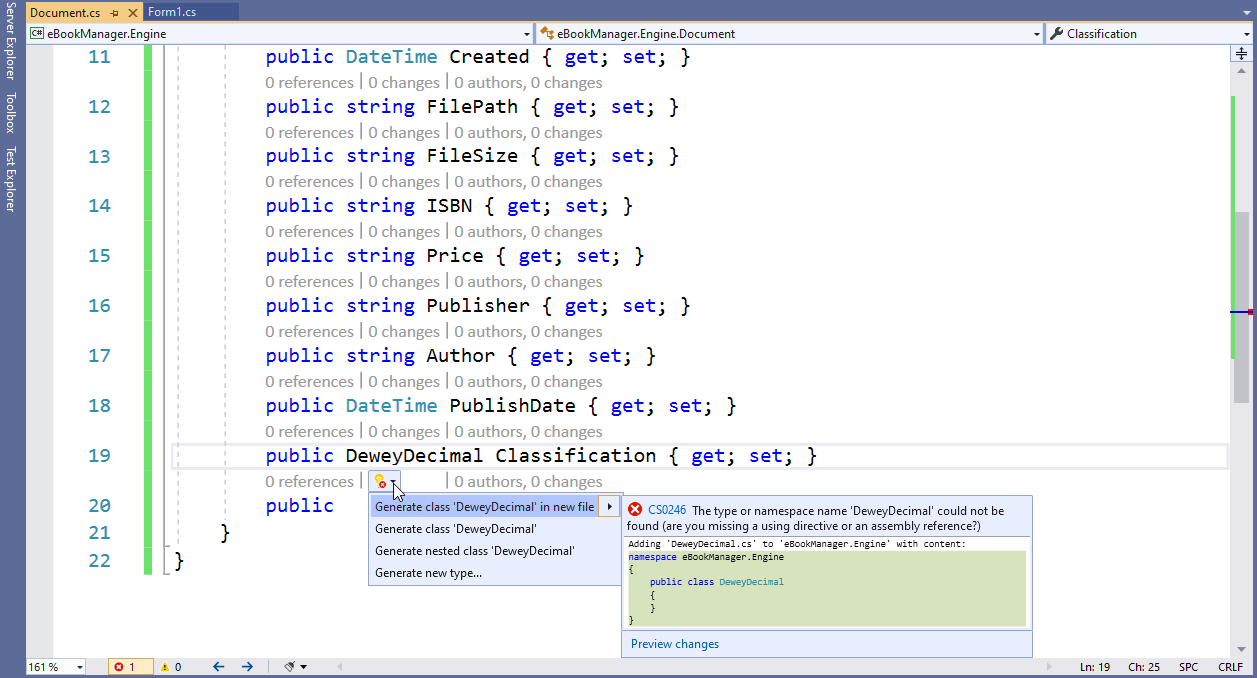
This allows us to create a new class with a couple of keystrokes. It also means that the name of the class will match the class name in the calling code.
The DeweyDecimal system is quite big. For this reason, I have not catered for every book classification available. I have also assumed that you only want to be working with programming eBooks. In reality, however, you may want to add other classifications, such as literature, the sciences, the arts, and so on. It is up to you:
- Open up the DeweyDecimal class and add the following code to the class:
public class DeweyDecimal
{
public string ComputerScience { get; set; } = "000";
public string DataProcessing { get; set; } = "004";
public string ComputerProgramming { get; set; } = "005";
}
Word nerds may disagree with me here, but I would like to remind them that I'm a code nerd. The classifications represented here are just so that I can catalog programming- and computer science-related eBooks. As mentioned earlier, you can change this to suit your needs.
- We now need to add in the heart of the eBookManager.Engine solution. This is a class called DocumentEngine and will contain the methods you need in order to work with the documents:
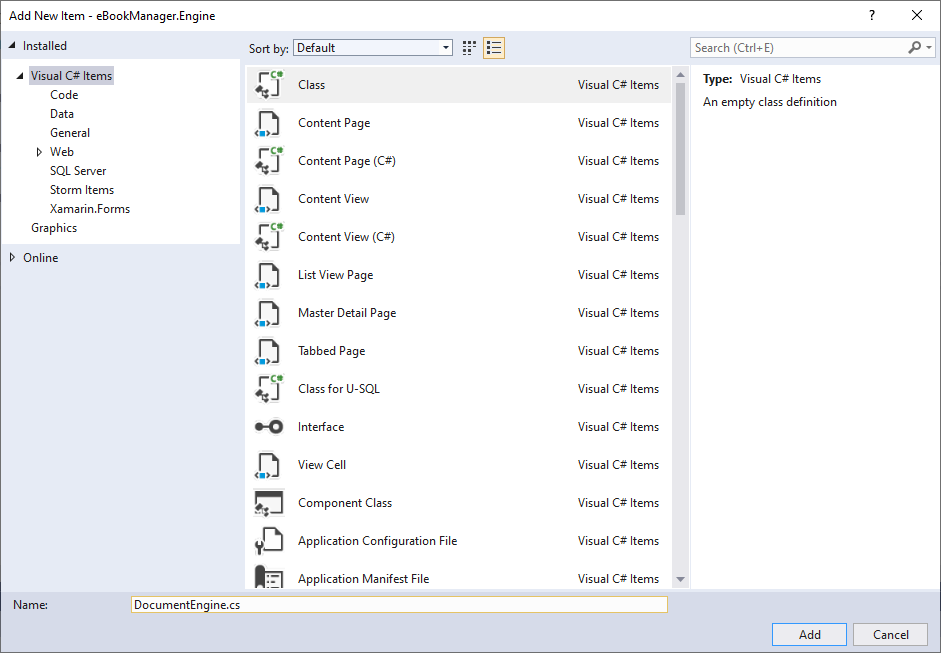
Your eBookManager.Engine solution will now contain the following classes:
- DeweyDecimal
- Document
- DocumentEngine
- We now need to add a reference to eBookManager.Engine from the eBookManager project:
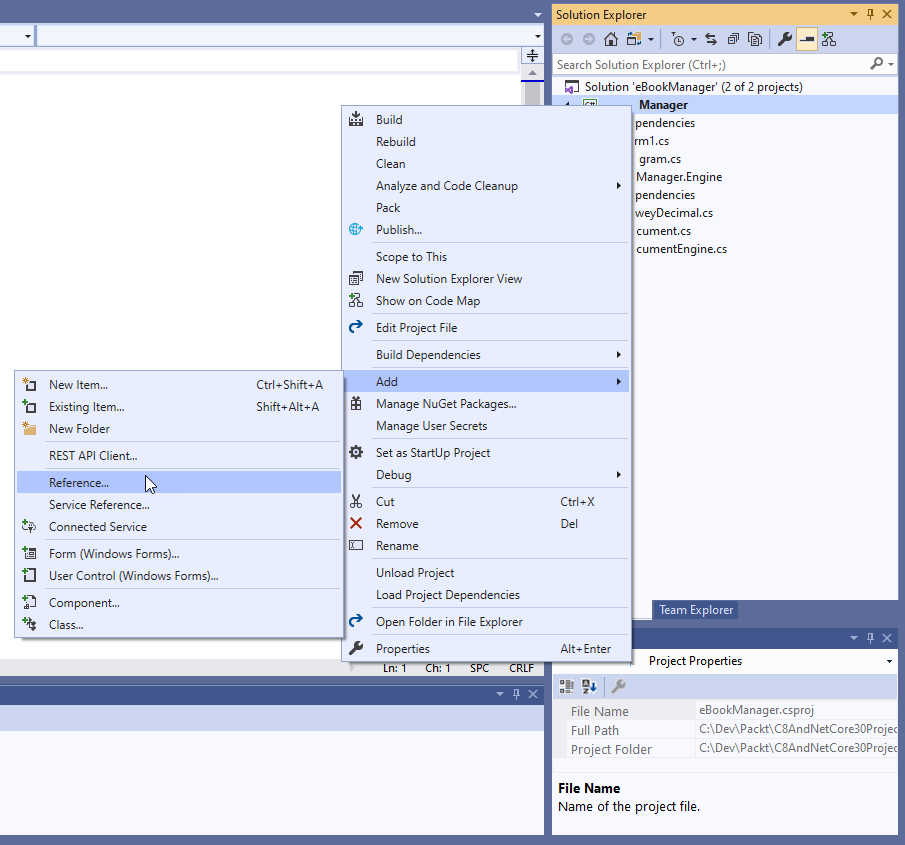
The eBookManager.Engine project will be available in the Projects section in the Reference Manager screen:
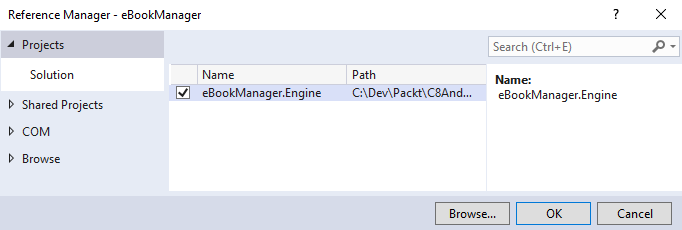
- Once we have added the reference, we need a Windows Form that will be responsible for importing new books. Add a new form called ImportBooks to the eBookManager solution:
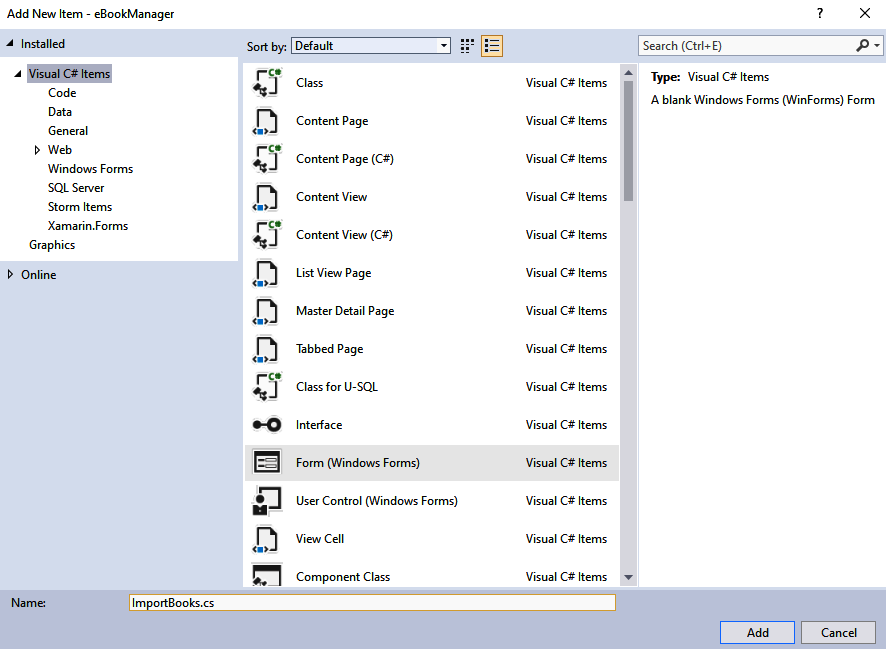
- We'll create a separate project for extension methods. Add the eBookManager.Helper class library project (again, as a .NET Standard class library project):
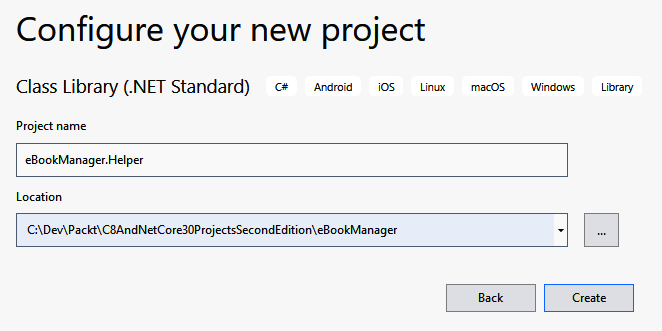
- We'll reference that from our main project (as before):
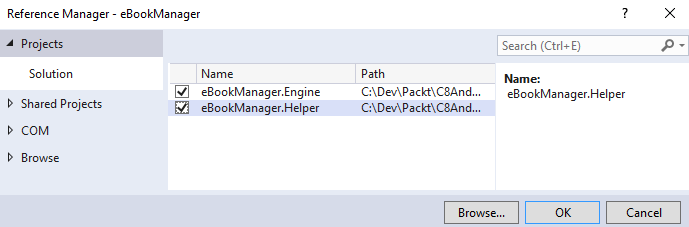
We've now set up the basics needed for our eBookManager application. Next, we will venture further into the guts of the application by writing some code.