In order to follow this section, you won't need the WinForms app from the first edition—any WinForms app will do; however, it is recommended that you use that application, especially for the later section where we will discuss C# 8 features.
You can download the original project from the following location:
https://github.com/PacktPublishing/CSharp7-and-.NET-Core-2.0-Blueprints
If you download and run the application, you should see that it still works fine:
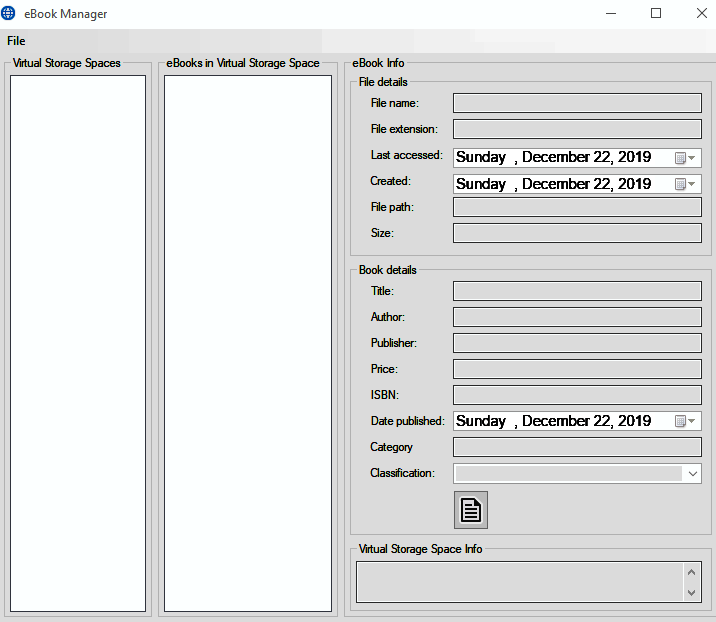
Let's now investigate how we can run this exact same code base under .NET Core 3. We'll start with the project file. Basically, we need to tell Visual Studio that we now have a .NET Core 3 project, and not a Framework one.
If you have PowerTools installed (https://marketplace.visualstudio.com/items?itemName=VisualStudioProductTeam.ProductivityPowerPack2017), you can do this from within Visual Studio; if not, then simply open the .csproj file using your favorite text editor:
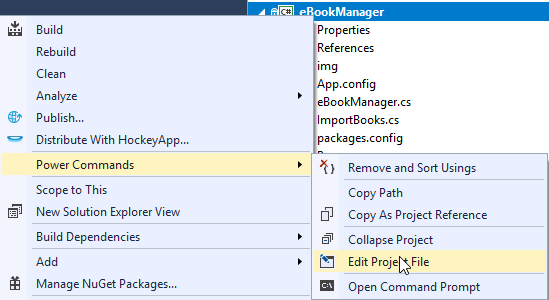
Change the contents of the .csproj file to the following:
<Project Sdk="Microsoft.NET.Sdk.WindowsDesktop"> <PropertyGroup> <OutputType>WinExe</OutputType> <TargetFramework>netcoreapp3.0</TargetFramework> <LangVersion>8.0</LangVersion> <AssetTargetFallback>uap10.0.18362</AssetTargetFallback> <UseWindowsForms>true</UseWindowsForms> </PropertyGroup> <ItemGroup> <PackageReference Include="Newtonsoft.Json" Version="12.0.2" /> </ItemGroup> <ItemGroup> <ProjectReference Include="..\eBookManager.Controls\eBookManager.Controls.csproj" /> <ProjectReference Include="..\eBookManager.Engine\eBookManager.Engine.csproj" /> <ProjectReference Include="..\eBookManager.Helper\eBookManager.Helper.csproj" /> </ItemGroup> <ItemGroup> <Reference Include="System"> <HintPath>System</HintPath> </Reference> </ItemGroup> </Project>
This is, essentially, all that's needed; however, you will need to decide what to do about the project's resources. You can manually just check that they are all set to copy to the output directory; alternatively, we can add an ItemGroup to the project file, such as the following:
<ItemGroup> <None Update="ico\importBooks.ico"> <CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory> </None> <None Update="ico\mainForm.ico"> <CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory> </None> <None Update="img\add_ebook_to_storage_space.png"> <CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory> </None> <None Update="img\add_new_storage_space.png"> <CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory> </None> <None Update="img\docx16.png"> <CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory> </None> <None Update="img\docxx16.png"> <CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory> </None> <None Update="img\eBook.png"> <CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory> </None> <None Update="img\epubx16.png"> <CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory> </None> <None Update="img\folder-close-x16.png"> <CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory> </None> <None Update="img\folder_exp_x16.png"> <CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory> </None> <None Update="img\image sources.txt"> <CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory> </None> <None Update="img\no_eBook.png"> <CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory> </None> <None Update="img\pdfx16.png"> <CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory> </None> <None Update="img\ReadEbook.png"> <CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory> </None> <None Update="img\storage_space_cloud.png"> <CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory> </None> </ItemGroup>
As you can see, the whole thing is a great deal simpler than the previous version of the file.
Unfortunately, Preview 1 was not stable enough to make the changes necessary for this chapter, and so we are bypassing the designer.
The next step is to delete the following files, found under Properties:
- AssemblyInfo.cs
- Settings.Designer.cs
- Settings.settings
In fact, by the end of this chapter, the entire Properties folder will be gone.
Actually, that's it. Simply reload the project and hit F5. The app is now running under .NET Core. However, it's very likely you'll get an error at this point. The reason is that we have two other projects that are still running under .NET Framework:
- eBookManager.Engine
- eBookManager.Helper
We'll need to migrate each project in a similar way; let's start with eBookManager.Engine. As before, edit the project file and replace what you find there with the following:
<Project Sdk="Microsoft.NET.Sdk"> <PropertyGroup> <TargetFramework>netcoreapp3.0</TargetFramework> </PropertyGroup> </Project>
As you can see, this is even simpler than before. In fact, it is not necessary to have this target 3.0; we could target 2.2, or even 2.1 if we so chose. Again, we'll delete AssemblyInfo.cs.
Finally, we come to eBookManager.Helper. Edit the project file again to match the following:
<Project Sdk="Microsoft.NET.Sdk"> <PropertyGroup> <TargetFramework>netcoreapp3.0</TargetFramework> </PropertyGroup> <ItemGroup> <PackageReference Include="Newtonsoft.Json" Version="11.0.2" /> </ItemGroup> <ItemGroup> <ProjectReference Include="..\eBookManager.Engine\eBookManager.Engine.csproj" /> </ItemGroup> </Project>
Again, we'll delete AssemblyInfo.cs. We'll also need to remove a stray reference to System.Windows.Forms in ExtensionMethods.cs.