The use of HTTP verbs allows a clear understanding of what an operation is going to do. In general, the primary or most commonly used HTTP verbs are POST, GET, PUT, PATCH, and DELETE, which stand for create, read, update (PATCH and PUT), and delete, respectively. Of course, there are also a lot of other verbs, but they are not used as frequently:
Method | HTTP method description |
GET | GET is the most common HTTP verb. Its function is to retrieve data from a server at the specified resource.
For example, a request made to the GET https://<HOST>/customers endpoint will retrieve all customers in a list format (if there is no pagination). There is also the possibility of retrieving a specific customer such as GET https://<HOST>/customers/1234; in this instance, only the customer with the 1234 ID will be retrieved. It is important to add that the GET request only retrieves data and does not modify any resources; it's considered a safe and idempotent method. |
HEAD | The HEAD method does exactly what GET does, except that the server replies without the body. |
POST |
The most common usage of POST methods is to create a new resource. |
PATCH | The PATCH method is used to apply partial modifications to a resource, such as updating a name or a date, but not the whole resource. |
PUT |
Different from the PATCH method, the PUT method replaces all of the resource. |
DELETE | The DELETE method removes a resource. |
CONNECT |
The CONNECT method converts the connection request to a transparent TCP/IP tunnel, generally to facilitate encrypted communication with SSL (HTTPS) through an unencrypted HTTP proxy. |
OPTIONS | The OPTIONS method returns the HTTP methods supported by the server for the specified URL. |
TRACE | The TRACE method returns the same request that is sent to see whether there were changes and/or additions made by intermediate servers. |
In order to explain these methods in detail, let's consider a simple entity called Customer. We will imagine that there is an API called Customers available through HTTP and its destination is a NoSQL database like in the following diagram:
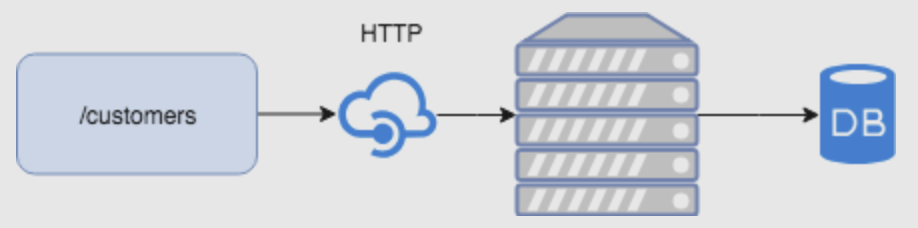
Considering that the database is empty, what should happen if we call the GET method by pointing to GET /CUSTOMERS? If you think that it should retrieve nothing, then you are absolutely right! Here is a request example:
GET https://<HOST>/customers
And here is the response:
[]
So, considering that there is no customer yet, let's create one using the POST method:
Request endpoint | Sample Request | Status code response |
POST /customers |
{ |
HTTP status code 201 |
Considering the POST method is successful, the HTTP status code should be 201 (we will talk more about status codes in Chapter 2, Principles of Designing RESTful APIs) and calling the GET method now; the response should be similar to the following code block (we're considering that there is just one single consumer of this endpoint and we are that consumer, so we can guarantee the data behavior into the server):
Request endpoint | Sample Request | Status code response |
GET /customers |
[ |
The HTTP status code is 200. |
What if we add two more customers and call the GET method again? Take a look at the following code block:
Request endpoint | Sample Response | Status code response |
GET /customers |
[ |
The HTTP status code is 200. |
Nice, so if we call the GET method for a specific ID, the response should be like the following:
Request endpoint | Sample Response | Status code response |
GET /customers/1 |
{ |
The HTTP status code is 200. |
We can also call POST methods for parent resources, such as /customers/1/orders, which will create new resources under the customer with ID 1. The same applies to the GET method, but only to retrieve the data as mentioned previously.
Okay, now that we know how to create and retrieve the resources, what if we want to change any information, such as the last name for John Doe? This one is easy—we just have to call the PATCH method in the same way that we called the POST method:
Request endpoint | Sample Request | Status code response |
PATCH /customers/1 |
{ |
The HTTP status code is 204. |
We can also change the information in a parent using a path as follows:
Request endpoint | Sample Request | Status code response |
PATCH /customers/1/orders |
{ |
The HTTP status code 204. |
And what if we need to change the whole resource? In that case, we should use the PUT method:
Request endpoint | Sample Request | Status code response |
PUT /customers/1 |
{ |
The HTTP status code is 201. |
Instead of PATCH, you can use the PUT method. To do so, all the parameters are required to be passed as the body into the request, even those parameters that haven't changed.
Finally, if you want to remove a resource, use the DELETE method:
Request endpoint | Status code response |
PUT /customers/1 |
The HTTP status code is 204. |