You don't have to always load the entire D3 library. D3 is a modular library, so if you are only using some D3 features, you can include only the parts that you are using. The minified default bundle is about 240 KB in size, but you may be able to create your chart using as little as 13 KB if you don't need many modules. An animated interactive bar chart, complete with tooltips, transitions, colors, and SVG graphics can be created with less than 24 KB loading just the following two modules:
<script src="https://d3js.org/d3-selection.v1.min.js"></script>
<script src="https://d3js.org/d3-transition.v1.min.js"></script>
But if you need axes, maps, and other features, you will require more modules and dependencies. In this case, either use the default bundle, or set up a development environment where you can install each module using npm, since it automatically includes any dependencies. For production, you can generate and export a custom bundle using a packing tool such as Rollup. To install any module using npm, use the following:
npm install module-name
For the examples in this chapter (and most of the book) we will import D3 using the <script> tag with the CDN URL to the default bundle.
Even if you always use the default d3.js bundle, you should be aware of the modules it contains, and of the modules that are not part of the default bundle, because they need to be imported separately in case you require their functions. All the official documentation is also organized per module, and knowledge of the modular structure will allow you to tune the performance of your D3 application, in case you decide to create a custom bundle. Modules have their own versioning systems. You might need to load a module separately if you wish to use a feature included in a new major version, not yet included in the default bundle.
The following diagram shows the modules available in D3.js version 5, indicating direct dependencies (transitive dependencies are not shown). For example, if you need to use a function from the d3-scale module, you should import all the direct dependencies: (d3-array, d3-time-format, d3-collection, d3-format, d3-interpolate) and the transitive ones:( d3-time and d3-color). In this case, you should either use npm or import the default bundle.
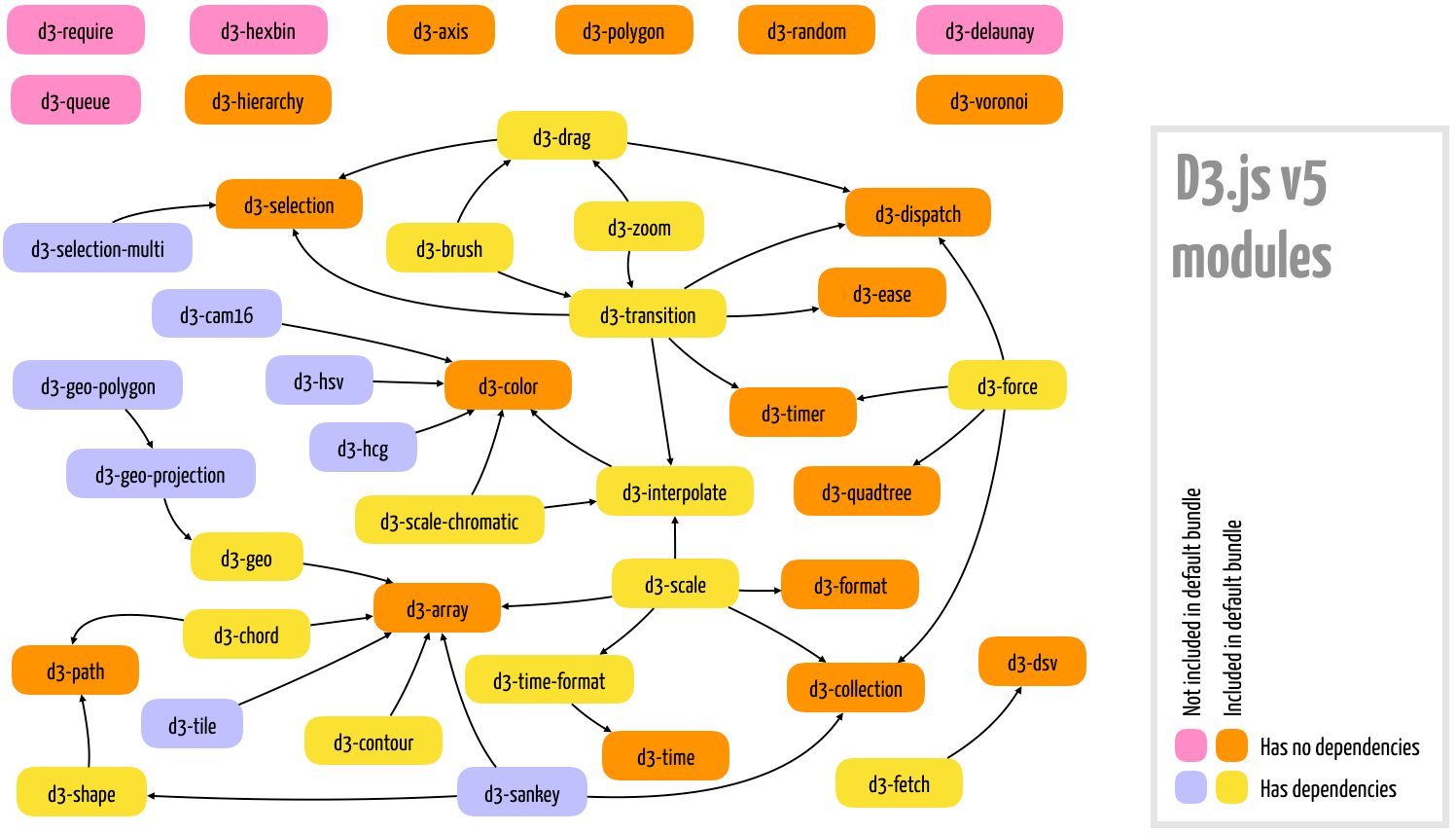
The following tables contain a quick reference of all modules available in the current version, classified according to their purpose. In this book, we will be using functions from almost all of them.