Installing Rust on any supported platform is simple. All we need to do is navigate to https://rustup.rs/. That page will give us a single-step procedure to install the command-line Rust compiler. The procedure differs slightly depending on the platform, but it's never difficult. Here we see the rustup.rs page for Linux:
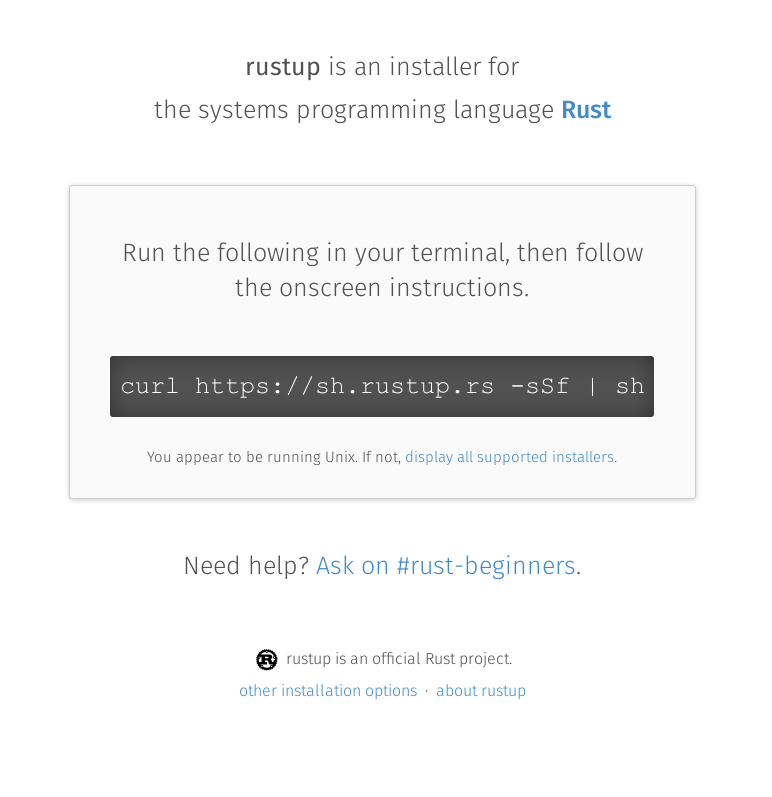
The installer doesn't just install the Rust compiler, it also installs a tool called rustup that can be used at any time to upgrade our compiler to the latest version. To do this, all we have to do is open up a command-line (or Terminal) window, and type: rustup update.
Upgrading the compiler needs to be simple because the Rust project uses a six-week rapid release schedule, meaning there's a new version of the compiler every six weeks, like clockwork. Each release contains whatever new features have been deemed to be stable in the six weeks since the previous release, in addition to the features of previous releases.
If we aren't willing to wait for a feature to be vetted and stabilized, for whatever reason, we can also use rustup to download, install, and update the beta or nightly releases of the compiler.
To download and install the beta compiler, we just need to type this: rustup toolchain install beta.
From that point on, when we use rustup to update our compiler, it will make sure that we have the newest versions of both the stable and beta compilers. We can then make the beta compiler active with rustup default beta.
Please note that the beta compiler is not the same thing as the next release of the stable compiler. The beta version is where features live before they graduate to stable, and features can and do remain in beta for years.
The nightly version is at most 24 hours behind the development code repository, which means that it might be broken in any number of ways. It's not particularly useful unless you're actually participating in the development of Rust itself. However, should you want to try it out, rustup can install and update it as well. You might also find yourself depending on a library that someone else has written that depends on features that only exist in the nightly build, in which case you'll need to tell rustup that you need the nightly build, too.
One of the things rustup will install is a tool called cargo, which we'll be seeing a lot of in this chapter, and using behind the scenes for the rest of this book. The cargo tool is the frontend to the whole Rust compiler system: it is used for creating new Rust project directories containing the initial boilerplate code for a program or library, for installing and managing dependencies, and for compiling Rust programs, among other things.