For most applications, you will want to display a window for its users to interact with. In wxPython, the most typical window object is known as a Frame
. This recipe will show you how to subass a Frame
and display it in an application.
This example extends upon the previous recipe to add a minimal empty application window:
import wx class MyApp(wx.App): def OnInit(self): self.frame = MyFrame(None, title="The Main Frame") self.SetTopWindow(self.frame) self.frame.Show() return True class MyFrame(wx.Frame): def __init__(self, parent, id=wx.ID_ANY, title="", pos=wx.DefaultPosition, size=wx.DefaultSize, style=wx.DEFAULT_FRAME_STYLE, name="MyFrame"): super(MyFrame, self).__init__(parent, id, title, pos, size, style, name) # Attributes self.panel = wx.Panel(self) if __name__ == "__main__": app = MyApp(False) app.MainLoop()
Running the previous code will result in a window like the following being shown:
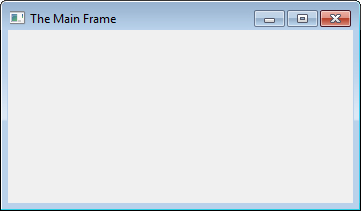
The Frame
is the main top-level window and container for most applications. Let's start by examining our MyFrame
class. In this class there is one important thing to note. We created a Panel
object as a child window of the Frame
. You can think of a Panel
as a box for containing other controls. Also, in order for a Frame
to operate and look correct on all platforms, it is important that it has a Panel
as its main child.
Firstly, in the OnInit
method of our App
, we create an instance of MyFrame
, passing None
as its first parameter. This parameter is used to specify the parent window of the Frame
. Because this is our main window, we pass in None
to indicate that it has no parent. Secondly, we call the SetTopWindow
method of our App
in order to set our newly-created MyFrame
instance as the application's top window. Thirdly and finally, we call Show
on our Frame
; this simply does what its name suggests, and shows the Frame
so that a user can see it, though the Frame
will not actually be visible on the screen until the MainLoop
is started.
The Frame
class has a number of style flags that can be set in its constructor to modify the behavior and appearance of the window. These style flags can be combined as a bitmask and are supplied as the value to the constructors' style parameter. The following table outlines some of the common ones. A full list of all available styles can be found in the wxPython online documentation, at http://wxpython.org/onlinedocs.php.
Style flags |
Description |
---|---|
This is a bitwise OR of the following flags:
| |
Display a title bar button that minimizes the Frame | |
Display a title bar button that maximizes the Frame | |
Display a title bar button that allows the Frame to be closed. (the "X" button) | |
Allow the Frame to be resized by the user when they drag the border | |
Displays a caption on the Frame | |
Display a system menu (that is, the menu that is shown when clicking in the frames icon on Windows) | |
Eliminates flicker caused by the background being repainted (Windows only) |