All controls, and many other user-interface elements, such as menus, take an ID as an argument in their constructor that can be used to identify the control or object inside event handlers. Typically, the value of wx.ID_ANY
is used to let the system automatically generate an ID for the item, or the wx.NewId
function is used to create a new ID. However, there are also a number of predefined IDs available in the wx
module that have special meaning for certain common items that many applications tend to have, such as Copy/Paste menu items or Ok/Cancel buttons. The expected behavior and appearance of some of these items can vary from platform to platform. By using the stock ID, wxPython will take care of the differences for you. This recipe will show a few of the places in which these IDs can come in handy.
This code snippet shows how to make use of some of the predefined IDs to simplify the creation of some common UI elements:
class MyFrame(wx.Frame): def __init__(self, parent, id=wx.ID_ANY, title="", pos=wx.DefaultPosition, size=wx.DefaultSize, style=wx.DEFAULT_FRAME_STYLE, name="MyFrame"): super(MyFrame, self).__init__(parent, id, title, pos, size, style, name) # Attributes self.panel = wx.Panel(self) # Setup ok_btn = wx.Button(self.panel, wx.ID_OK) cancel_btn = wx.Button(self.panel, wx.ID_CANCEL, pos=(100, 0)) menu_bar = wx.MenuBar() edit_menu = wx.Menu() edit_menu.Append(wx.NewId(), "Test") edit_menu.Append(wx.ID_PREFERENCES) menu_bar.Append(edit_menu, "Edit") self.SetMenuBr(menu_bar)
The previous class will create the following window:
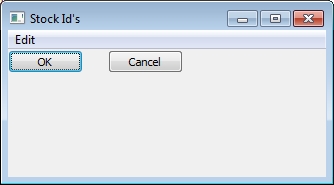
The first thing to notice in this recipe is that no labels were specified for the two buttons that we created. By using the Stock IDs for OK and Cancel as their IDs, the framework will automatically put the proper label o the control.
This also applies to menu items, as can be seen in our Edit menu for the Preferences item. Another important thing to note is that if this sample is run on Macintosh OS X, the framework will also automatically move the Preferences menu item to its expected location in the Application menu.
Using buttons with Stock IDs in a Modal Dialog will also allow the dialog to be dismissed, and return the appropriate value, such as wx.OK
or wx.CANCEL
, without the need to connect event handlers to the buttons for performing this standard action. Automatically getting the correct button layout for a dialog can also be achieved by using Stock IDs with StdDialogButtonSizer
.
The Creating Stock Buttons recipe in Chapter 3, Basic Building Blocks of a User Interface shows how Stock IDs can be used to construct standard buttons.
The Standard dialog button layout recipe in Chapter 7, Window Layout and Design shows how to easily add common buttons to dialogs by using Stock IDs.
The Optimizing for OS X recipe in Chapter 12, Building and Managing Applications for Distribution shows more uses for Stock IDs.