We already know that we will write Java code that will itself use other people's Java code and will be compiled into DEX code that runs on the DVM. In addition to this, we will also be adding and editing other files as well. These files are known as Android resources.
Our app will include resources such as images, sounds, and user interface layouts that are kept in separate files from the Java code. We will slowly introduce ourselves to them over the course of this book.
They will also include files that contain the textual content of our app. It is a convention to refer to the text in our app through separate files because it makes them easy to change, and this makes it easy to create apps that work for multiple different languages.
Furthermore, the actual UI layouts of our apps, despite the option to implement them with a visual designer, are actually read from text-based files by Android.
Android (or any computer), of course, cannot read and recognize text in the same way that a human can. Therefore, we must present our resources in a highly organized and predefined manner. To do so, we will use Extensible Markup Language (XML). XML is a huge topic but, fortunately, its whole purpose is to be both human and machine readable. We do not need to learn this language, we just need to observe (and then conform to) a few rules. Furthermore, most of the time when we interact with XML, we will do so through a neat visual editor provided by Android Studio. We can tell when we are dealing with an XML resource because the filename will end with the .xml
extension.
You do not need to memorize this, as we will constantly be returning to this concept throughout the book.
In addition to these resources, it is worth noting that Java, as used in Android, has a structure to its code. There are many millions of lines of code that we can take advantage of. This code will obviously need to be organized in a way that makes it easy to find and refer to. It is organized under predefined packages that are specific to Android.
Whenever we create a new Android app, we will choose a unique name known as a package. We will see how to do this in the Our first Android app section. Packages are often separated into subpackages, so they can be grouped together with other similar packages. We can simply think of these as folders and subfolders.
We can also think of all the packages that the Android API makes available to us as books that contain code, from a library. Some common Android packages we will use include the following:
android.graphics
android.database
android.view.animation
As you can see, they are arranged and named to make what is contained in them as obvious as possible.
Tip
If you want to get an idea for the sheer depth and breadth of the Android API, then take a look at the Android package index at http://developer.android.com/reference/packages.html.
Earlier, we learned that reusable code blueprints that we can transform into objects are called classes. Classes are contained in these packages. We will see in our very first app how to easily import other people's packages along with specific classes from those packages for use in our projects. A class will almost always be contained in its own file, with the same name as the class, and have the .java
file extension.
In Java, we further break up our classes into sections that perform the different actions for our class. We call these sections methods. These are, most often, the methods of the class that we will use to access the functionality provided within all those millions of lines of code. We do not need to read the code. We just need to know which class does what we need, which package it is in, and which methods from within the class give us precisely the results we are after.
The next diagram shows a representation of the Android API. We can think about the structure of the code that we will write in exactly the same way, although we will most likely have just one package per app. Of course, because of the object-oriented nature of Java, we will only be using selective parts from this API. Also note that each class has its own distinct data. Typically, if you want access to the data in a class, you need to have an object of that class.
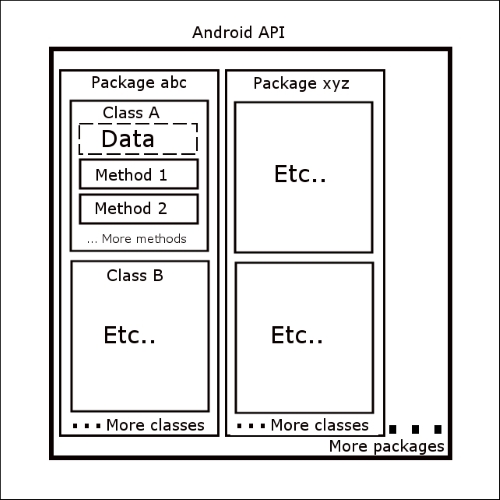
You do not need to memorize this, as we will constantly be returning to this concept throughout the book.
By the end of this chapter, we will have imported multiple packages and some classes from them and we will have used other people's methods. By the end of Chapter 2, Java – First Contact, we will have even written our very own methods as well.