In the previous recipe, you have learnt how to create an HTTP trigger and accept the input parameters. Let's now work on something interesting, that is, where you store the input data into a persistent medium. Azure Functions supports us to store data in many ways. For this example, we will store the data in Azure Table storage.
Persisting employee details using Azure Storage table output bindings
Getting ready
In this recipe, you will learn how easy it is to integrate an HTTP trigger and the Azure Table storage service using output bindings. The Azure HTTP trigger function receives the data from multiple sources and stores the user profile data in a storage table named tblUserProfile.
- For this recipe, we will use the same HTTP trigger that we have created in our previous recipe.
- We will be using Azure Storage Explorer which is a tool that helps us to work with the data stored in Azure Storage account. You can download it from http://storageexplorer.com/.
- You can learn more about Connect to the Storage Account using Azure Storage Explorer at https://docs.microsoft.com/en-us/azure/vs-azure-tools-storage-manage-with-storage-explorer
How to do it...
- Navigate to the Integrate tab of the RegisterUser HTTP trigger function.
- Click on the New Output button and select Azure Table Storage then click on the Select button:
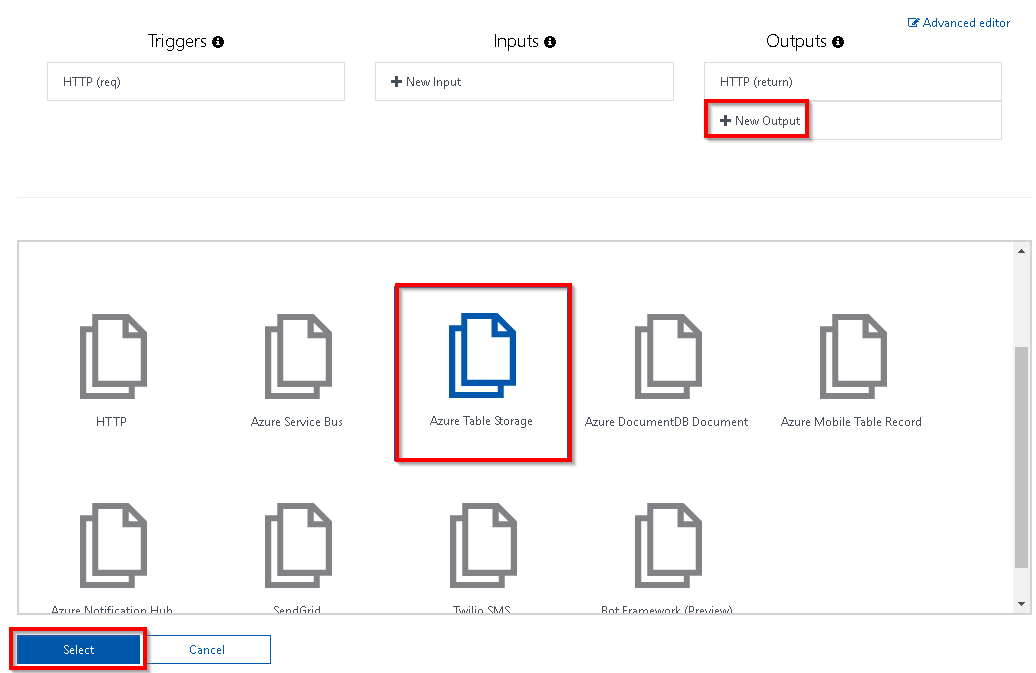
- Once you click on the Select button in the previous step, you will be prompted to choose the following settings of the Azure Table storage output bindings:
- Table parameter name: This is the name of the parameter that you will be using in the Run method of the Azure Function. For this example, please provide objUserProfileTable as the value.
- Table name: A new table in the Azure Table storage will be created to persist the data. If the table doesn't exist already, Azure will automatically create one for you! For this example, please provide tblUserProfile as the table name.
- Storage account connection: If you don't see the Storage account connection string, click on the new (shown in the following screenshot) to create a new one or to choose an existing storage account.
- The Azure Table storage output bindings should be as shown in the following screenshot:
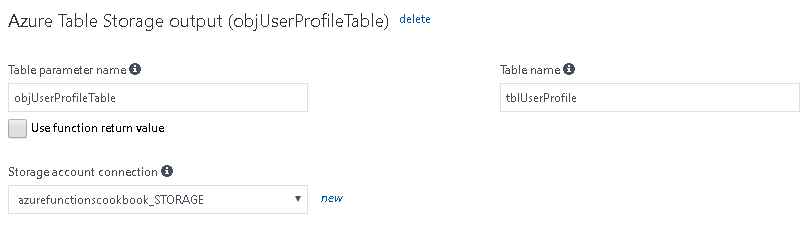
- Click on Save to save the changes.
- Navigate to the code editor by clicking on the function name and paste the following code:
#r "Microsoft.WindowsAzure.Storage"
using System.Net;
using Microsoft.WindowsAzure.Storage.Table;
public static async Task<HttpResponseMessage>
Run(HttpRequestMessage req,TraceWriter
log,CloudTable objUserProfileTable)
{
dynamic data = await
req.Content.ReadAsAsync<object>();
string firstname= data.firstname;
string lastname=data.lastname;
UserProfile objUserProfile = new UserProfile(firstname,
lastname);
TableOperation objTblOperationInsert =
TableOperation.Insert(objUserProfile);
objUserProfileTable.Execute(objTblOperationInsert);
return req.CreateResponse(HttpStatusCode.OK,
"Thank you for Registering..");
}
public class UserProfile : TableEntity
{
public UserProfile(string lastName, string firstName)
{
this.PartitionKey = "p1";
this.RowKey = Guid.NewGuid().ToString();;
this.FirstName = firstName;
this.LastName = lastName;
}
public UserProfile() { }
public string FirstName { get; set; }
public string LastName { get; set; }
}
- Let's execute the function by clicking on the Run button of the Test tab by passing firstname and lastname parameters in the Request body as shown in the following screenshot:
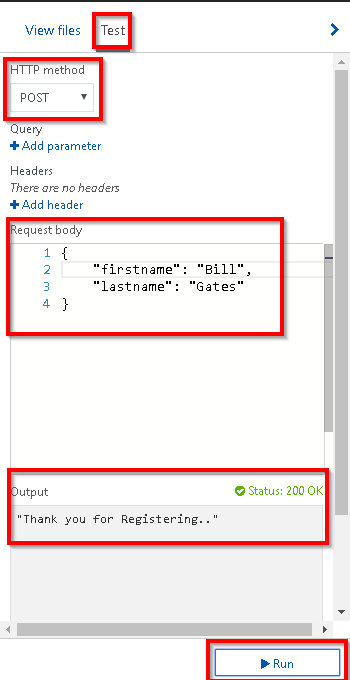
- If everything went well, you should get a Status 200 OK message in the Output box as shown in the preceding screenshot. Let's navigate to Azure Storage Explorer and view the table storage to see if the table named tblUserProfile was created successfully:
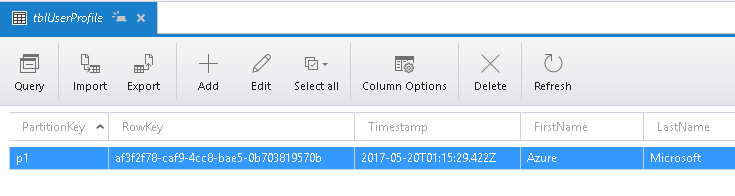
How it works...
Azure Functions allows us to easily integrate with other Azure services just by adding an output binding to the trigger. For this example, we have integrated the HTTP trigger with the Azure Storage table binding and also configured the Azure Storage account by providing the storage connection string and the Azure Storage table name in which we would like to create a record for each of the HTTP requests received by the HTTP trigger.
We have also added an additional parameter for handling the table storage named objUserProfileTable, of type CloudTable, to the Run method. We can perform all the operations on the Azure Table storage using objUserProfileTable.
We have also created an object of UserProfile, and filled it with the values received in the request object, and then passed it to a table operation. You can learn more about handling operations on Azure Table storage service from the URL https://docs.microsoft.com/en-us/azure/storage/storage-dotnet-how-to-use-tables.
Understanding more about Storage Connection
When you create a new storage connection (please refer to the third step of the How to do it... section of this recipe) a new App settings will be created as shown in the following screenshot:
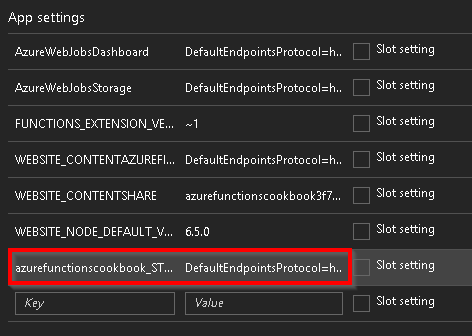
You can navigate to the App settings by clicking on Application settings of the Platform features tab as shown in the following screenshot:
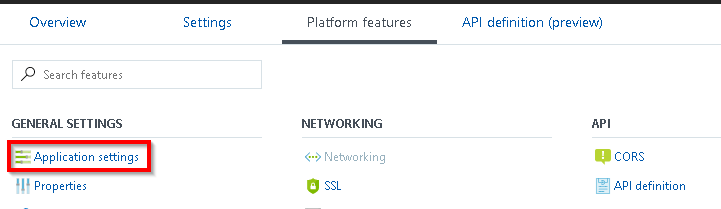
What is Azure Table storage service?
Azure Table storage service is a NoSQL key-value persistent medium for storing semi-structured data. You can learn more about the same at https://azure.microsoft.com/en-in/services/storage/tables/.
Partition key and row key
The primary key of Azure Table storage tables has two parts as follows:
- Partition key: Azure Table storage records are classified and organized into partitions. Each record located in a partition will have the same partition key (p1 in our example).
- Row key: A unique value should be assigned for each of the rows.
There's more...
Following is the very first line of the code in this recipe:
#r "Microsoft.WindowsAzure.Storage"
The preceding line of code instructs the function runtime to include a reference to the specified library to the current context.