A vector is an n-tuple of real numbers. A tuple is a finite ordered list of elements. An n-tuple is an ordered list of elements which has n dimensions. In the context of games n is usually 2, 3, or 4. An n-dimensional vector is represented as follows:
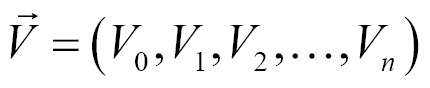
The subscript numbers are called the components of the vector. Components are expressed as a number or as a letter corresponding to the axis that component represents. Subscripts are indexed starting with 0. For example,
is the same as
. Axis x, y, z, and w correspond to the numbers 0, 1, 2, and 3, respectively.
Vectors are written as a capital bold letter with or without an arrow above it. and V are both valid symbols for vector V. Throughout this book we are going to be using the arrow notation.
A vector does not have a position; it has a magnitude and a direction. The components of a vector measure signed displacement. In a two-dimensional vector for example, the first component represents displacement on the X axis, while the second number represents displacement on the Y axis.
Visually, a vector is drawn as a displacement arrow. The two dimensional vector would be drawn as an arrow pointing to 3 units on the X axis and 2 units on the Y axis.
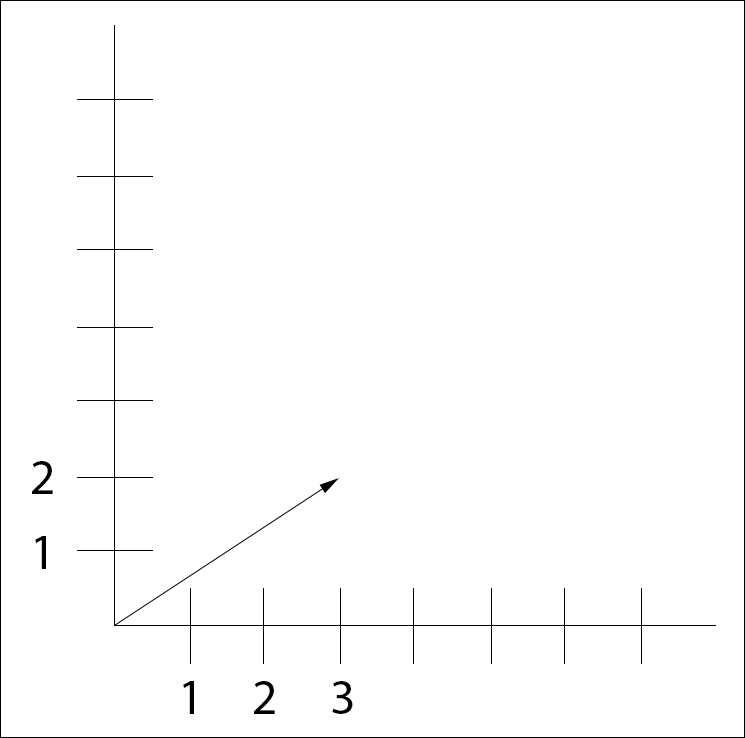
A vector consists of a direction and a magnitude. The direction is where the vector points and the magnitude is how far along that direction the vector is pointing. You can think of a vector as a series of instructions. For example, take three steps right and two steps up. Because a vector does not have a set position, where it is drawn does not matter as shown in the following diagram:
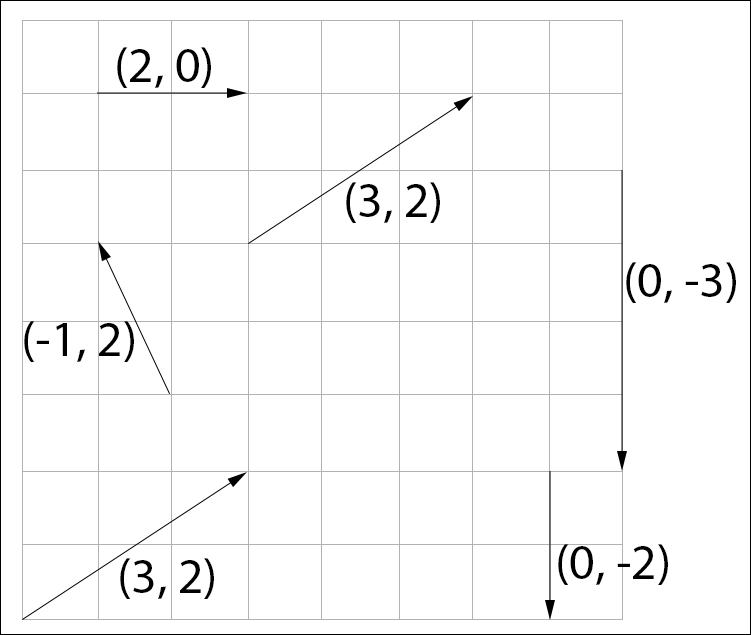
The preceding figure shows several vectors, with vector (3,2) appearing multiple times. The origin of a vector could be anywhere; the coordinate system of the preceding figure was omitted to emphasize this.
Video games commonly use two, three, and four-dimensional vectors. In this recipe, we are going to define C++ structures for two and three-dimensional vectors. These structures will expose each component of the vector by the name of an axis, as well as a numeric index.
Follow these steps to start implementing a math library with vector support:
Create a new C++ header file; call this file
vectors.h
; add standard C-style header guards to the file:#ifndef _H_MATH_VECTORS_ #define _H_MATH_VECTORS_ // Structure definitions // Method declarations #endif
Replace the
// Structure definitions
comment with the definition of a two-dimensional vector:typedef struct vec2 { union { struct { float x; float y; }; float asArray[2]; }; float& operator[](int i) { return asArray[i]; } } vec2;
After the definition of
vec2
, add the definition for a three-dimensional vector:typedef struct vec3 { union { struct { float x; float y; float z; }; float asArray[3]; }; float& operator[](int i) { return asArray[i]; } } vec3;
We have created two new structures, vec2
and vec3
. These structures represent two and three-dimensional vectors, respectively. The structures are similar because with every new dimension the vector just adds a new component.
Inside the vector structures we declare an anonymous union. This anonymous union allows us to access the components of the vector by name or as an index into an array of floats. Additionally, we overloaded the indexing operator for each structure. This will allow us to index the vectors directly.
With the access patterns we implemented, the components of a vector can be accessed in the following manner:
vec3 right = {1.0f, 0.0f, 0.0f}; std::cout<< "Component 0: " <<right.x<< "\n"; std::cout<< "Component 0: " <<right.asArray[0] << "\n"; std::cout<< "Component 0: " <<right[0] << "\n";
Games often use a four-dimensional vector, which adds a W component. However, this W component is not always treated as an axis. The W component is often used simply to store the result of a perspective divide, or to differentiate a vector from a point.
A vector can represent a point in space or a direction and a magnitude. A three-dimensional vector has no context; there is no way to tell from the x, y, and z components if the vector is supposed to be a point in space or a direction and a magnitude. In the context of games, this is what the W component of a four-dimensional vector is used for.
If the W component is 0, the vector is a direction and a magnitude. If the W component is anything else, usually 1, the vector is a point in space. This distinction seems arbitrary right now; it has to do with matrix transformations, which will be covered in Chapter 3, Matrix Transformations.
We did not implement a four-dimensional vector because we will not need it. Our matrix class will implement explicit functions for multiplying points and vectors. We will revisit this topic in Chapter 3, Matrix Transformations.