In this task, we are going to list the pattern in the deck. Later, we will let the player select patterns from this deck.
We are going to need a new module to handle the display of the composition. Let's create a new empty JavaScript file named composition-view.js
.
We need to import the file into the index.html
file, as follows:
<script src='js/composition-view.js'></script>
Let's work on the pattern with the following steps:
In the game scene of the
index.html
file, we add two DOM elements, namely,#your-composition
and#deck
:<div id="game-scene" class="scene out"> ... <div id="your-composition"></div> <div id="deck" class="deck"></div> </div>
In the template element, we add the template for the pattern's slot:
<div id="element-template"> <!-- for deck view --> <div class="pattern-slot"> <div class="pattern" data-pattern="1"></div> </div> ... </div>
The following is our
composition-view.js
file:(function(){ var game = this.colorQuestGame = this.colorQuestGame || {}; // composition module game.compositionView = { node: document.getElementById('your-composition'), }; })();
Before the end of the
gameScene.visualize
method, we add the visualization logic for the player's composition:// randomize the patterns array patternsToShow.sort(function(a, b){ return Math.random() - 0.5; }); // empty the current deck view var deckNode = document.getElementById('deck'); deckNode.removeAllChildren(); // add the pattern to the deck view for (var i in patternsToShow) { var patternSlotNode = document.querySelector('#element-template .pattern-slot').cloneNode(/*clone children=*/true); patternSlotNode.querySelector('.pattern').setAttribute('data-pattern', patternsToShow[i]); deckNode.appendChild(patternSlotNode); }
From the
game.js
file, we remove all the selected patterns before starting a new level:nextLevel: function() { ... game.compositionView.node.removeAllChildren(); this.startLevel(); },
We need the following CSS style for the composition and patterns:
/* player's composition and pattern */ #your-composition { position: absolute; width: 100px; height: 100px; right: 65px; top: 120px; border: 3px solid #999; } #your-composition > .pattern { width: 100px; height: 100px; position: absolute; } /* deck and pattern */ .deck { position: absolute; top: 360px; left: 20px; } .pattern-slot { width: 100px; height: 100px; outline: 4px solid #BC7702; float: left; border-radius: 3px; margin: 10px 0 0 10px; } .deck .pattern { width: 100%; height: 100%; }
We should have the following screenshot once this task is completed. The deck shows the patterns that a player needs to compose the quest:
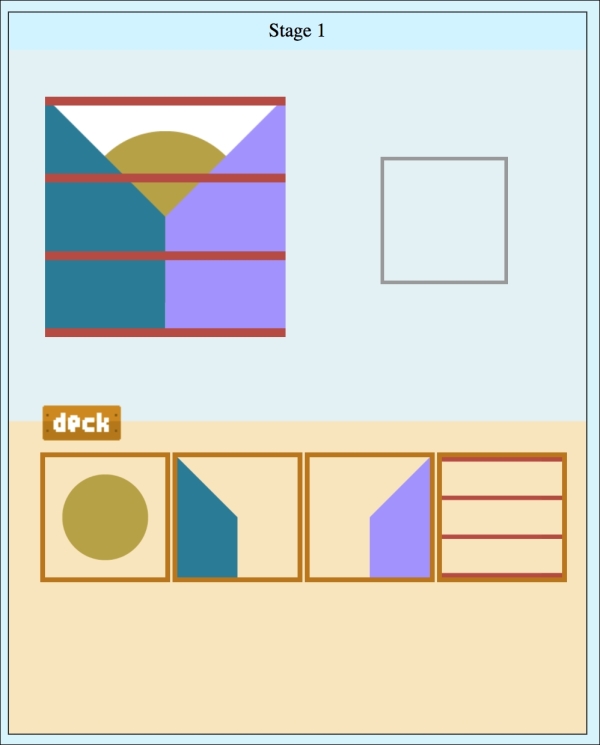
JavaScript comes with a sort
method for the array. Normally, we compare the given two array elements and return +1
or -1
to control elements' swapping.
We can randomize an array by randomly returning either +1
or -1
:
patternsToShow.sort(function(a, b){ return Math.random() - 0.5; });
After we randomize the patterns, we clone each pattern from the template and append them to the deck element.