In this section, we
shall compile, package, and deploy the EJB application to the WebLogic server using an Ant build file. First, add the web.xml
deployment descriptor. Create a WEB-INF
folder in the webModule
folder and add web.xml
to the WEB-INF
folder. The web.xml
is an optional deployment descriptor for a web application deployed to the WebLogic descriptor and is listed as follows:
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee/web- app_2_5.xsd" version="2.5"> </web-app>
If we were using servlets or other web application artifacts, we would have configured those in web.xml
. We need a build.xml
file for the tasks involved in assembling and deploying the entity bean application. Create a build.xml
file in the project EJB3JPA
by going to File | New | Other and in the New wizard, in XML, select XML file. Specify the following properties in the build.xml
file:
Property |
Value |
Description |
---|---|---|
|
|
Source directory |
|
|
Web module directory |
|
|
WebLogic home directory |
|
|
WebLogic Server directory |
|
|
Build directory |
|
|
Deploy directory |
Specify a path
element to include the WebLogic JAR files. Add the following tasks in the build file:
Task |
Description |
---|---|
|
Makes the required directories |
|
Compiles the Java classes |
|
Creates a EJB JAR |
|
Creates a web application WAR file |
|
Assembles the JAR and the WAR files into an EAR file |
|
Deploy the EAR file to WebLogic server |
|
Deletes directories when recompiling |
The build.xml
file is listed as follows:
<?xml version="1.0" encoding="UTF-8"?> <!-- WebLogic build file -->
First, declare the different properties used in the build script:
<project name="EJB3" default="deploy" basedir="."> <property environment="env" /> <property name="src.dir" value="${basedir}/ejbModule" /> <property name="web.module" value="${basedir}/webModule" /> <property name="weblogic.home" value="C:/Oracle/Middleware/" /> <property name="weblogic.server" value="${weblogic.home}/wlserver_12.1/server" /> <property name="build.dir" value="${basedir}/build" /> <property name="deploy.dir" value="${weblogic.home}/user_projects/domains/base_domain/autodeploy" />
Specify the class path for the classes to be compiled:
<path id="classpath"> <fileset dir="${weblogic.home}/modules"> <include name="*.jar" /> </fileset> <fileset dir="${weblogic.server}/lib"> <include name="*.jar" /> </fileset> <pathelement location="${build.dir}" /> </path> <property name="build.classpath" refid="classpath" /> <target name="prepare"> <mkdir dir="${build.dir}" /> </target>
Compile the Java classes, which include the entity beans and the session bean facade:
<target name="compile" depends="prepare"> <javac srcdir="${src.dir}" destdir="${build.dir}" debug="on" includes="**/*.java"> <classpath refid="classpath" /> </javac> </target>
Generate the EJB JAR file, which includes the compiled EJB classes and the deployment descriptors:
<target name="jar" depends="compile"> <jar destfile="${build.dir}/ejb3.jar"> <fileset dir="${build.dir}"> <include name="**/*.class" /> </fileset> <fileset dir="${src.dir}"> <include name="META-INF/*.xml" /> </fileset> </jar> </target>
Generate the web application WAR file, which includes the JSP client:
<target name="war" depends="jar"> <war warfile="${build.dir}/weblogic.war"> <fileset dir="webModule"> <include name="*.jsp" /> </fileset> <fileset dir="webModule"> <include name="WEB-INF/web.xml" /> </fileset> </war> </target>
Assemble the application into an EAR file, which includes the EJB JAR and the WAR files and the application.xml
deployment descriptor:
<target name="assemble-app" depends="war"> <jar jarfile="${build.dir}/ejb3.ear"> <metainf dir="META-INF"> <include name="application.xml" /> </metainf> <fileset dir="${build.dir}" includes="*.jar,*.war" /> </jar> </target>
Deploy the
application to the WebLogic Server deploy directory, the autodeployed
directory:
<target name="deploy" depends="assemble-app"> <copy file="${build.dir}/ejb3.ear" todir="${deploy.dir}" /> </target> <target name="clean"> <delete file="${build.dir}/ejb3.ear" /> <delete file="${build.dir}/ejb3.jar" /> <delete file="${build.dir}/weblogic.war" /> </target> </project>
Having created a build script, now we shall run it. Right-click on build.xml and select Run As | Ant Build as shown in the following screenshot:
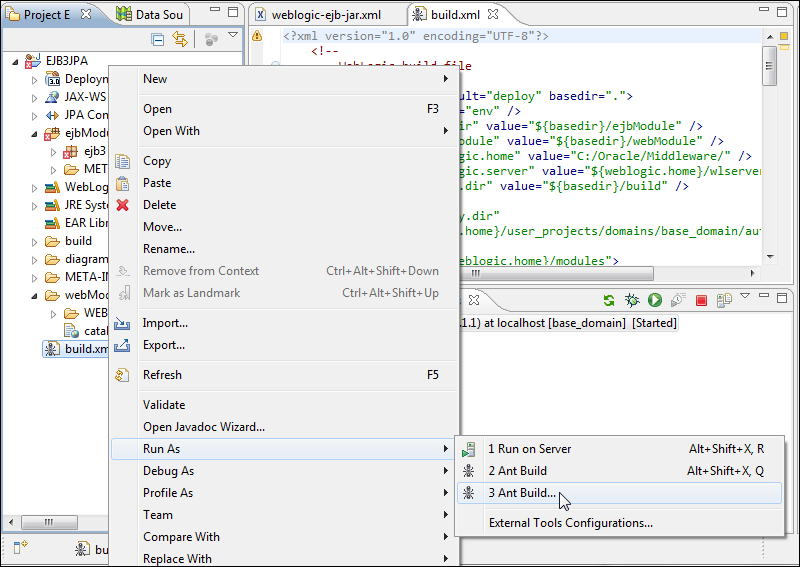
Select the deploy task and click on Run as shown in the following screenshot. As dependence is declared between tasks all the preceding tasks will also get run.
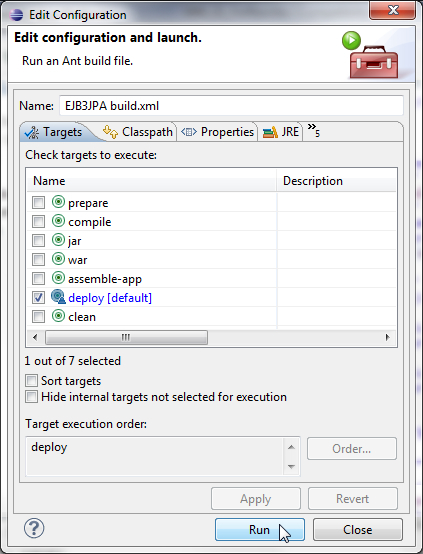
The EJB
application gets compiled, built, assembled, and deployed to WebLogic Server's autodeployed directory as an EAR file. The BUILD SUCCESSFUL message indicates that the build and deployment was without error. If the build needs to be re-run first run the clean
task, which deletes any previously generated EAR, WAR, and JAR files.
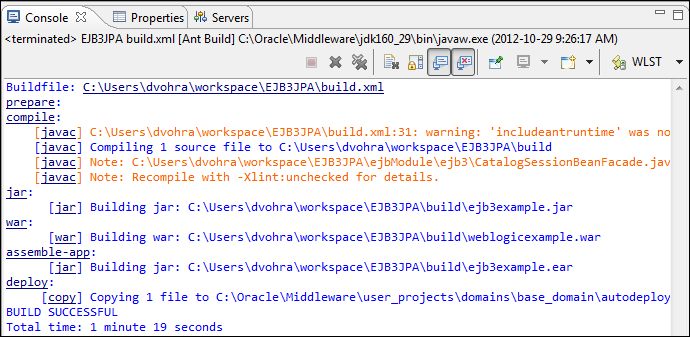
Start the WebLogic Server if not already started. In the WebLogic Server's Admin Server Console, the EAR file is shown deployed in Deployments. On expanding the EAR file the EJB JAR and web module WAR get listed as shown in the following screenshot:
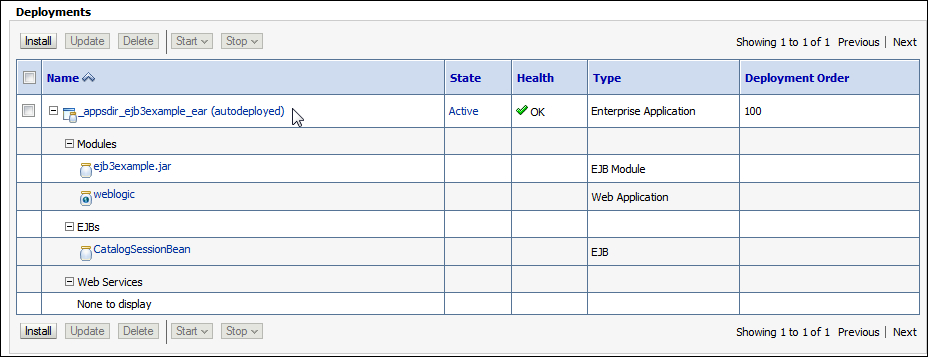