In this recipe, we will take a quick look at the CoffeeScript language and command line.
CoffeeScript is a highly expressive programming language that does away with much of the ceremony required by JavaScript. It uses whitespace to define blocks of code and provides shortcuts for many of the programming constructs found in JavaScript.
For example, we can declare variables and functions without the var
keyword:
firstName = 'Mike'
We can define functions using the following syntax:
multiply = (a, b) -> a * b
Here, we defined a function named multiply
. It takes two arguments, a
and b
. Inside the function, we multiplied the two values. Note that there is no return
statement. CoffeeScript will always return the value of the last expression that is evaluated inside a function.
The preceding function is equivalent to the following JavaScript snippet:
var multiply = function(a, b) { return a * b; };
It's worth noting that the CoffeeScript code is only 28 characters long, whereas the JavaScript code is 50 characters long; that's 44 percent less code.
We can call our multiply
function in the following way:
result = multiply 4, 7
In CoffeeScript, using parenthesis is optional when calling a function with parameters, as you can see in our function call. However, note that parenthesis are required when executing a function without parameters, as shown in the following example:
displayGreeting = -> console.log 'Hello, world!' displayGreeting()
In this example, we must call the displayGreeting()
function with parenthesis.
Tip
You might also wish to use parenthesis to make your code more readable. Just because they are optional, it doesn't mean you should sacrifice the readability of your code to save a couple of keystrokes. For example, in the following code, we used parenthesis even though they are not required:
$('div.menu-item').removeClass 'selected'
Like functions, we can define JavaScript literal objects without the need for curly braces, as seen in the following employee
object:
employee = firstName: 'Mike' lastName: 'Hatfield' salesYtd: 13204.65
Notice that in our object definition, we also did not need to use a comma to separate our properties.
CoffeeScript supports the common if
conditional as well as an unless
conditional inspired by the Ruby language. Like Ruby, CoffeeScript also provides English keywords for logical operations such as is
, isnt
, or
, and and
. The following example demonstrates the use of these keywords:
isEven = (value) -> if value % 2 is 0 'is' else 'is not' console.log '3 ' + isEven(3) + ' even'
In the preceding code, we have an if
statement to determine whether a value is even or not. If the value is even, the remainder of value % 2
will be 0
. We used the is
keyword to make this determination.
Tip
JavaScript has a nasty behavior when determining equality between two values. In other languages, the double equal sign is used, such as value == 0
. In JavaScript, the double equal operator will use type coercion when making this determination. This means that 0 == '0'
; in fact, 0 == ''
is also true.
CoffeeScript avoids this using JavaScript's triple equals (===
) operator. This evaluation compares value and type such that 0 === '0'
will be false.
We can use if
and unless
as expression modifiers as well. They allow us to tack if
and unless
at the end of a statement to make simple one-liners.
For example, we can so something like the following:
console.log 'Value is even' if value % 2 is 0
Alternatively, we can have something like this:
console.log 'Value is odd' unless value % 2 is 0
We can also use the if...then
combination for a one-liner if
statement, as shown in the following code:
if value % 2 is 0 then console.log 'Value is even'
CoffeeScript has a switch
control statement that performs certain actions based on a list of possible values. The following lines of code show a simple switch
statement with four branching conditions:
switch task when 1 console.log 'Case 1' when 2 console.log 'Case 2' when 3, 4, 5 console.log 'Case 3, 4, 5' else console.log 'Default case'
In this sample, if the value of a task is 1
, case 1
will be displayed. If the value of a task is 3
, 4
, or 5
, then case 3
, 4
, or 5 is displayed, respectively. If there are no matching values, we can use an optional else
condition to handle any exceptions.
If your switch
statements have short operations, you can turn them into one-liners, as shown in the following code:
switch value when 1 then console.log 'Case 1' when 2 then console.log 'Case 2' when 3, 4, 5 then console.log 'Case 3, 4, 5' else console.log 'Default case'
CoffeeScript has a number of other productive shortcuts that we will cover in depth in Chapter 2, Starting with the Basics.
Tip
CoffeeScript provides a number of syntactic shortcuts to help us be more productive while writing more expressive code. Some people have claimed that this can sometimes make our applications more difficult to read, which will, in turn, make our code less maintainable. The key to highly readable and maintainable code is to use a consistent style when coding. I recommend that you follow the guidance provided by Polar in their CoffeeScript style guide at http://github.com/polarmobile/coffeescript-style-guide.
With CoffeeScript installed, you can use the coffee
command-line utility to execute CoffeeScript files, compile CoffeeScript files into JavaScript, or run an interactive CoffeeScript command shell.
In this section, we will look at the various options available when using the CoffeeScript command-line utility.
We can see a list of available commands by executing the following command in a command or terminal window:
coffee --help
This will produce the following output:
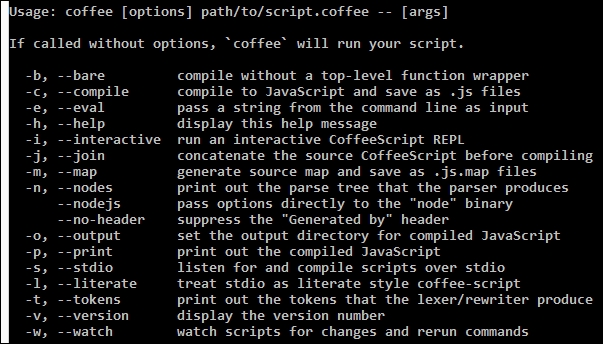
As you can see, the coffee
command-line utility provides a number of options. Of these, the most common ones include the following:
As we have been, CoffeeScript has an interactive shell that allows us to execute CoffeeScript commands. In this section, we will learn how to use the REPL shell. The REPL shell can be an excellent way to get familiar with CoffeeScript.
To launch the CoffeeScript REPL, open a command window and execute the coffee
command.
This will start the interactive shell and display the following prompt:
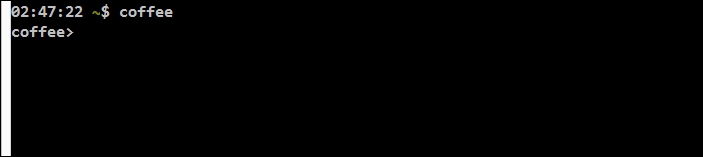
In the coffee>
prompt, we can assign values to variables, create functions, and evaluate results.
When we enter an expression and press the return key, it is immediately evaluated and the value is displayed.
For example, if we enter the expression x = 4
and press return, we would see what is shown in the following screenshot:

This did two things. First, it created a new variable named x
and assigned the value of 4
to it. Second, it displayed the result of the command.
Next, enter timesSeven = (value) -> value * 7
and press return:

You can see that the result of this line was the creation of a new function named timesSeven()
.
We can call our new function now:

By default, the REPL shell will evaluate each expression when you press the return key. What if we want to create a function or expression that spans multiple lines? We can enter the REPL multiline mode by pressing Ctrl + V. This will change our coffee>
prompt to a ------>
prompt. This allows us to enter an expression that spans multiple lines, such as the following function:

When we are finished with our multiline expression, press Ctrl + V again to have the expression evaluated. We can then call our new function:

The CoffeeScript REPL offers some handy helpers such as expression history and tab completion.
Pressing the up arrow key on your keyboard will circulate through the expressions we previously entered.
Using the Tab key will autocomplete our function or variable name. For example, with the isEvenOrOdd()
function, we can enter isEven
and press Tab to have the REPL complete the function name for us.