Source maps and Chrome's developer tools can help troubleshoot our CoffeeScript that is destined for the Web. In this recipe, we will demonstrate how to debug CoffeeScript that is designed to run on the server.
Begin by installing the Node Inspector NPM module with the following command:
npm install -g node-inspector
To use Node Inspector, we will use the coffee
command to compile the CoffeeScript code we wish to debug and generate the source map.
In our example, we will use the following simple source code in a file named counting.coffee
:
for i in [1..10] if i % 2 is 0 console.log "#{i} is even!" else console.log "#{i} is odd!"
To use Node Inspector, we will compile our file and use the source map parameter with the following command:
coffee -c -m counting.coffee
Next, we will launch Node Inspector with the following command:
node-debug counting.js
When we run Node Inspector, it does two things.
First, it launches the Node debugger. This is a debugging service that allows us to step through code, hit line breaks, and evaluate variables. This is a built-in service that comes with Node. Second, it launches an HTTP handler and opens a browser that allows us to use Chrome's built-in debugging tools to use break points, step over and into code, and evaluate variables.
Node Inspector works well using source maps. This allows us to see our native CoffeeScript code and is an effective tool to debug server-side code.
The following screenshot displays our Chrome window with an active break point. In the local variables tool window on the right-hand side, you can see that the current value of i
is 2
:
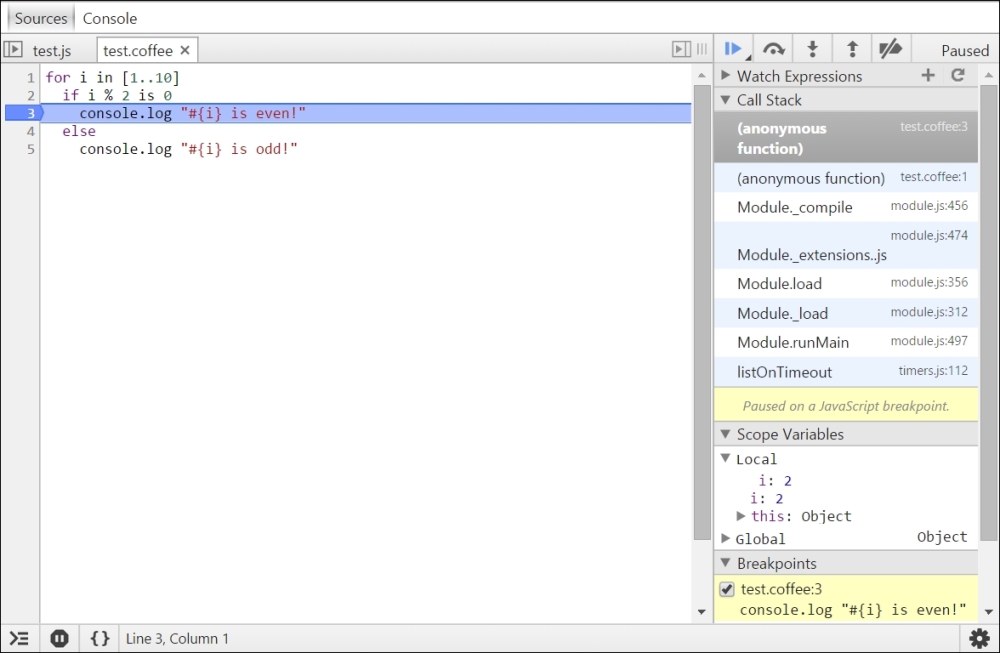
The highlighted line in the preceding screenshot depicts the log message.