The ArcGIS REST API provides access to ArcGIS Server and ArcGIS Online services to any language that can make requests and handles the returned responses. Python is one such language along with many others. To make use of this API, you must understand what requests can be made, how to structure those requests, and how to process the responses.
The operations provided through the API include the following:
Consume ArcGIS Server and ArcGIS Online services
Publish and manage services
Create and share ArcGIS Online or portal services
ArcGIS Server and ArcGIS Online administration
The REST API can be categorized into sections including using Esri-provided services, using your own services and services published by others, managing services, and administering services and portals.
All resources and operations exposed by the REST API are accessible through a hierarchy of endpoints or Uniform Resource Locators (URLs) for each GIS service published with the ArcGIS Server. When using the ArcGIS services portion of the REST API, you typically start from a well-known endpoint, which represents the server catalog.
You need to understand some basic concepts of the ArcGIS REST API before putting it to use through Python. Specifically, you need to know how to construct a URL and how to interpret the response that is returned. All resources and operations in the ArcGIS REST API are exposed through a hierarchy of endpoints. For now, let's examine the specific steps you need to understand to submit requests to the API through Python. The services directory can be used to generate a URL that can be used in your requests.
The first step is to determine the well-known endpoint. This represents a server catalog that is a set of operations that ArcGIS Server can perform along with specific services. The default endpoint for ArcGIS Server takes the form: http://<server>/arcgis/res
t/serviceshttp://<server>/arcgis/rest/services
.
The next step is to go to the /rest/services endpoint to see the content of the ArcGIS Server instance. For example, open a browser and navigate to http://sampleserver1.arcgisonline.com/arcgis/rest/services and you will be presented with a list of folders displayed as links, as seen in the screenshot:
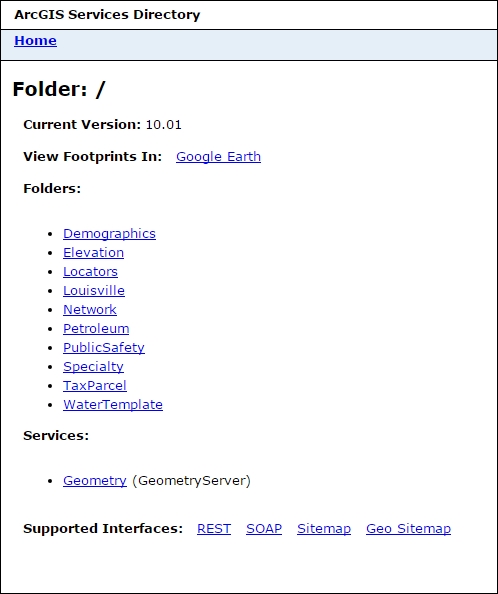
An ArcGIS Server instance does not have to have folders, but it is a good way of grouping services. You can click on a specific folder to see the services that are contained within.
Each service will have a name such as ESRI_Census_USA
along with a type such as MapServer
. The service type is listed in parentheses to the right hand side of the service name. In your browser with the sampleserver1
instance up, click through the various folders and services and note how the URL changes.
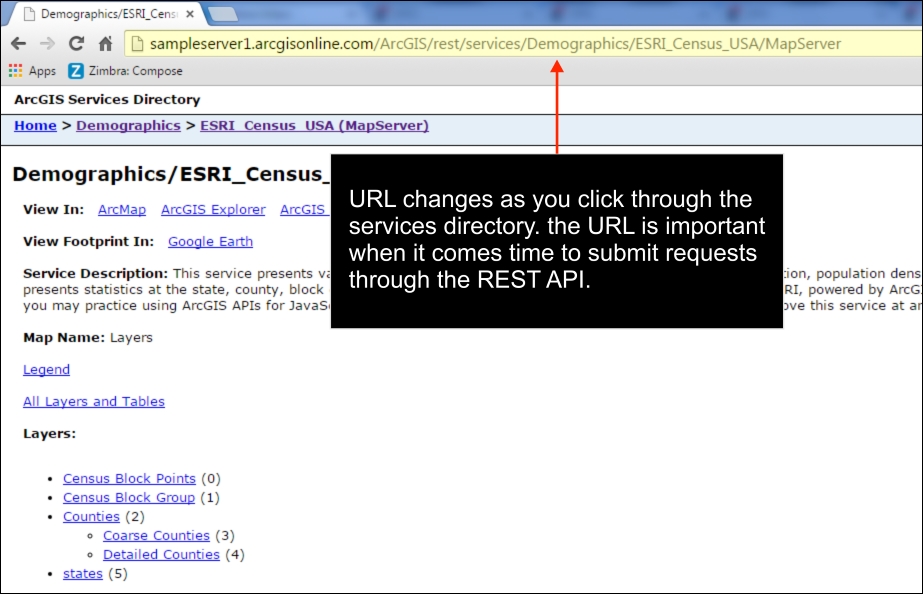
As you click on through the various links for a services directory, note how the URL in the address bar changes. This URL is very important because it provides you with the content that will be submitted through a Python request.
Now it's time to understand the documentation for the ArcGIS REST API. However, don't spend a lot of time on the documentation right now because we'll be going through many of the capabilities of the API as we move through the book.
Now properly construct the URL for the request. This is a very important step. The syntax for the request includes the path to the resource along with an operation name followed by a list of parameters. The operation name is what operation will be performed against the resource. For example, you might want to export a map to an image file. The question mark begins the list of parameters. Each parameter is then provided as a set of key or value pairs separated by an ampersand. All of this information is combined into a single URL string. A syntax example is provided as follows:
http://<resource-url>/<operation>?<parameter1=value1>&<parameter2=value2>
.
As we'll see later in the book, you can use the Python requests module to simplify this. The requests module allows you to define the list of parameters as a Python dictionary and then it handles the creation of the URL query string including URL encoding.
The response that is returned can be in various formats including .html
, .json
, .amf
, an image, and many others. To define how the response should be structured, you'll need to use the f
parameter. JSON is a very popular output format and can easily be handled in your Python code.
The Services Directory contains dialog boxes that you can use to generate parameter values. You can find links to these dialog boxes at the bottom of the services page. Click on one of the links to see the dialog box. This is illustrated in the following screenshot:
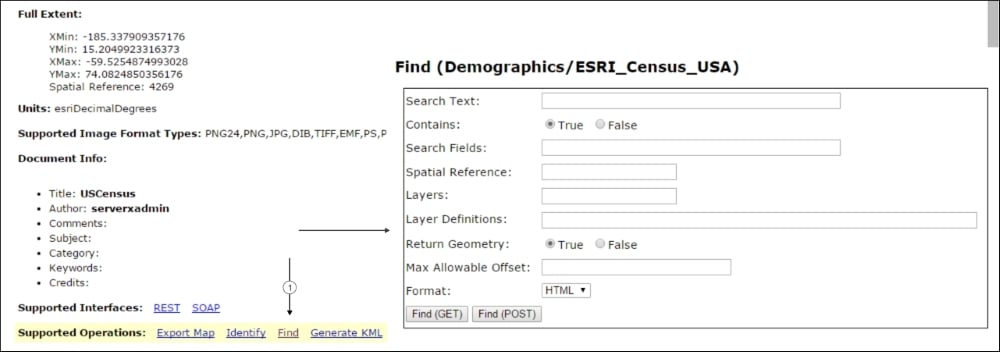
This is the preferred and most common way of making a URL request to ArcGIS Server. In this case the entire request is encoded in the URL. However, it does have a limitation of 1024 characters. Therefore, if you have a request that will exceed this number of characters you'll need to use the Post
method.
Now that you have at least a basic idea of how to construct a REST API query, let's discuss the capabilities provided by various sections of the API.
The REST API provides access to Esri provided services including those provided by ArcGIS Online. Service types include ready-made maps and basemaps
, geocoding, directions and routing, demographic and lifestyle attributes, and spatial analysis. Some of these services do require credits. When using services that require the use of pre-purchased credits you'll need to pass a token as part of the request for information from a service.
Mapping services include basemaps
of different varieties and sources including street maps, topographic maps, and hybrid maps. Esri also provides a World Geocoding service that can be used for address matching and reverse geocoding. A number of network analysis services, including routing, closest facility, service area, and others, can be used to accomplish network tasks. The GeoEnrichment
services provides access to demographic and lifestyle attributes. Other services include spatial analysis and elevation analysis.
Services that you have published as part of your own ArcGIS Server instance can be accessed through the REST API, as can services that others have provided and made available. There are many capabilities exposed by the REST API, so we'll just cover them at a high level for now. Generally, we can divide the capabilities into service-related functionality and functions that are more utilitarian in nature.
The REST API enables you to work with features, maps, geocode, geodata, geometry, geoprocessing, globe, image, network, schematic, and stream services. A wide array of operations is possible with each of these services. We'll discuss some of the capabilities provided.
For map and feature services, you can add attachments, export maps and tiles, retrieve features, find features, generate KML, render symbology, define HTML popups, identify features, retrieve a legend, perform queries, and more. You will find an example of a URL string used to perform a query against a layer as follows:
Feature services have the added capability of being able to perform edits, including adding and removing features, updating features, and deleting features. Here, you will find an example of a URL string used to delete a feature in a feature service:
http://services.myserver.com/ERmEceOGq5cHrItq/ArcGIS/rest/services/SanFrancisco/311Incidents/FeatureServer/0/deleteFeatures
.
http://services.myserver.com/ERmEceOGq5cHrItq/ArcGIS/rest/services/SanFrancisco/311Incidents/FeatureServer/0/deleteFeatures
.
Geocoding services provide the ability to geocode and reverse geocode addresses. Geocoding functionality provides the ability to map a single address or batch geocode a set of addresses. Reverse geocoding accepts a point and returns a set of address candidates. There is also a suggest operation that will provide a list of suggested addresses based on typed input from the user.
A geometry service is included with every ArcGIS Server instance and provides operations for many geometric operations including buffering, calculation of areas and lengths, generalization, intersection, projection, union, and many others. These operations work with individual geometry objects typically defined in a JSON format. An example of using the buffer operation is provided here:
Geoprocessing services represent geoprocessing tasks that have been created in ArcGIS Server. Operations provided through the API related to geoprocessing services include the ability to execute a task, cancel a job, retrieve the result of the task, and others.
Globe services published with ArcGIS Server provide information about the service including the service description as well as the layers published with the service. This includes individual layers as well as tiles.
Raster data can be accessed through an image service. This can include a single raster or multiple raster served as a single image through mosaicking. An image service supports accessing the mosaicked image, its catalog, and also the individual rasters in the catalog. Operations provided include export image, query, identify, download, measure, computer histograms, add, update, delete, upload, get samples, computer class statistics, and compute tie points.
Network service operations include solving closest facility tasks, routes, and a service area problem. You can also access network service information including the service description and the network layers associated with the service.
Schematic services support working with diagrams. Using this service, you can create, edit, delete, and save diagrams. Additional operations include loading, locking, querying, exporting, and updating diagrams, among others.
Stream services enable real-time applications where the datasets are frequently changing. This does require the ArcGIS GeoEvent
extension for ArcGIS Server that must be licensed and installed. The stream service resource provides basic information about the service, including event attribute fields, geometry, type, and WebSocket
resources. Operations include broadcast and subscribe. The broadcast operation serves as an endpoint for a stream service, and the subscribe operation serves as a connection point to a stream service.
The REST API includes a small number of utility functions to manage ArcGIS Server. The Catalog resource is the root note of an ArcGIS Server instance and can be used to retrieve the folders and services published. ServerInfo
is a resource that provides information about the server including version information, whether the server is using token-based authentication and the token services URL. The generateToken
resource generates an access token to access services that are token secured. The info resource provides information, metadata, and a thumbnail about services. Other operations include the export web map task, a refresh service, and a set of upload operations to upload data.
Using the REST API, you can manage your organization's ArcGIS Online account as well as the Portal for ArcGIS. Operations enable you to work with users, groups, and content. User operations include basic user information gathering, adding and removing users, sending a user invitation, searching for users, updating user information, getting and setting user tags, and enabling and disabling login access. Group operations including creating and deleting a group, joining a group, reassigning a group, updating a group, adding and removing users from a group, leaving a group, obtaining group information, and more. There are many content-related operations including creating services and folders, adding and deleting items, sharing and unsharing items, analysis of files before publication, the generation of output files, and much more.
You can programmatically administer your ArcGIS Server instance or Portal using the REST API. Using operations provided by the API, you can work with the site, clusters, services, security, system, data, uploads, logs, KML, info, and reports.
Site operations allow you to create, join, export, import, and delete a site. In addition, you can generate tokens, register, unregister, rename machines, work with SSL
, start and stop machines, and edit machines.
Cluster operations include starting and stopping a cluster, editing the protocol for a cluster, deleting a cluster, retrieving the services in a cluster, and adding and removing machines from a cluster.
There are many service operations including starting, stopping, editing, and deleting services, retrieving service statistics and service types, and adding and cleaning permissions. The items associated with a service also have operations including editing item information, uploading item information, deleting item information, and working with the service manifest. You can also federate and un-federate a service.
Security operations associated with the API including working with users, roles, security configuration, tokens, and working with the primary site administrator. You can add and remove users, update users, get a list of users, assign and remove roles, and get privileges. Role operations include adding, removing, and updating roles, searching for roles, getting roles for specific users, getting a list of users within a role, adding and removing users in a role, and assigning privileges to a role.
System operations allow you to update server properties, register, edit, clean, and unregister directories, edit configuration stores, work with web adaptors, retrieve job information, clear the cache, and edit the services directory.
Data operations include registering and unregistering a data item, finding data items, validating data items, starting, stopping, removing, and validating a data store, and updating the datastore
configuration.
Upload operations including uploading an item, registering an item, and working with individual items. For individual items you can upload a part, commit an item, delete an item, or retrieve item parts.
Log operations including editing log settings, querying logs, counting error reports, and cleaning logs.
There is a single operation related to KML
files. This is the Create KMZ
operation which will create a KMZ
file on the server from an input KML
file.
Finally, there is a set of operations related to usage reports. These include editing usage report settings, creating a usage report, editing or deleting a usage report, and querying report data.
The REST API includes operations that can be performed programmatically and that can't be performed using the Portal for ArcGIS website. Operations for Portal for ArcGIS include system and security operations.
System operations for Portal for ArcGIS include creating a site, working with licenses, working with web adaptors, directory operations, database operations, and system properties. License operations include updating the license manager, releasing a license, and working with entitlements including getting entitlements, importing entitlements, and removing entitlements. Web adaptor operations include unregistering a web adaptor and updating a web adaptor's configuration.
Security operations include working with users and groups, updating token configuration, setting up OAuth
, configuration operations, and working with SSL
certifications. User operations include creating users, searching for users, and refreshing user membership. Group operations include searching groups, refreshing group membership, getting users within a group, and getting a list of groups for a particular user. You can also update the token configuration. OAuth
operations provided by the REST API include changing the application id, getting the application information, and updating the app information. Configuration operations include updating the security configuration, updating the identity store, and testing the identity store. Using SSL
operations, you can update the web server certificate, generate a certificate, import an existing certificate, and export or delete a certificate.
The administration of ArcGIS Online hosted services using the REST API falls into two administrative categories: map services and feature services. Map services can be administered through operations including editing a service, checking the status of a service, refreshing a service, updating tiles, and getting tile creation information. For feature services, you can check the status of the service, refresh a service, add, update, or delete the definition, and work with the individual feature layers in the service.