As a relatively new programming language, Go doesn't come pre-installed on many operating systems. This section steps through setting it up for any readers who haven't already done so.
Configuring a development environment for Windows can be complicated as there aren't many tools installed by default. Due to this, there are many options for setting up using external tools and packages (such as MSYS, MinGW, and Ubuntu Subsystem) but exploring these is out of the scope of this book. Thankfully, it's possible to start developing Go applications without the need for many additional development tools.
Firstly, if you haven't already done so, you need to download and install Git. The download is available at https://git-scm.com/download/win, and it should start automatically when you visit that page. Run the file that's downloaded, and the setup will start (if a notice says this isn't verified then tap the Install Anyway
button). The default options should work for most users—make sure that Use Git from the Windows Command Prompt
is selected to avoid more work later on.
Once this is completed, open a Command Prompt window (search for cmd
from the Start
menu if you don't have a shortcut) and type git --version
—you should see something like the following output:
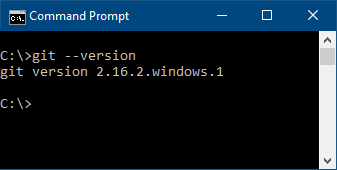
Testing Git is installed by checking the version
Next, you should install Go—that can be found at https://golang.org/dl/. On this page, choose the featured Microsoft Windows download (the name will end in .msi
). As with the Git installation, you'll need to run the downloaded file and possibly confirm that you would like to continue installing an unverified program. Once again, the default values should be suitable—if you change any of the configuration, make sure to update the following lines appropriately.
Once that installer has finished, return to your Command Prompt and type go version
, which should output the version number and quit:
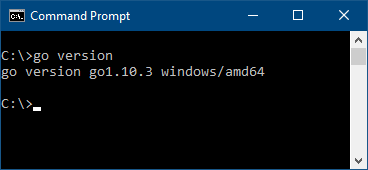
Testing Go is installed by checking the version
If the preceding installations succeeded, then your environment should be correctly configured. If you made some changes during the installation, you may need to make some adjustments to your environment configuration:
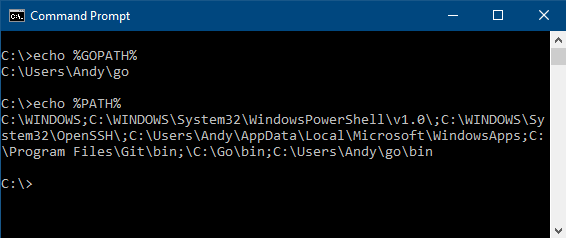
Checking that our %GOPATH%/bin appears in %PATH%
In the preceding output, you can see that Git\bin
, Go\bin
, and %GOPATH%
are included in your %PATH%
environment for finding executable files. If this isn't the case, you may need to log out or reboot for the settings to take effect.
Many developer tools (including Git) are installed as part of the XCode
package. If you haven't already installed Xcode for other development work, you can download it for free from the Mac App Store. Once installed, you should also set up the command-line tools—to do this go to the Xcode menu and select Preferences
, then Downloads
and Install Command Line Tools
.
If you're unsure about whether or not you've installed these already, then open the Terminal application and execute xcode-select
—if installed, that will execute normally, and if not, you'll be prompted to run the installation:
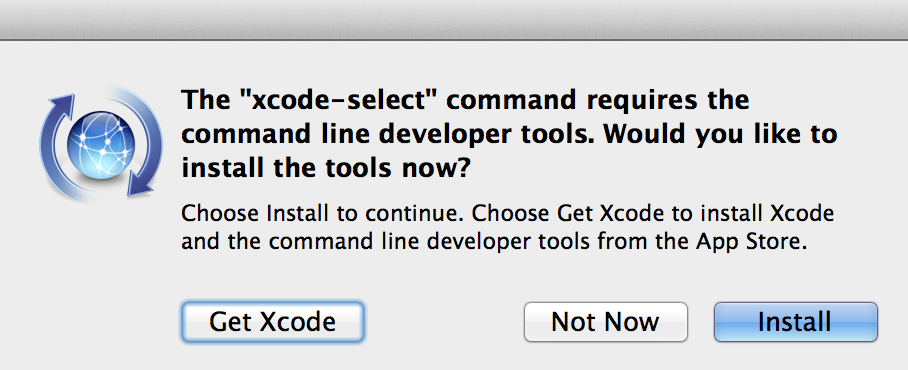
The installation dialog window if developer tools are not installed
In addition to these tools, you'll need to install Go. You can get the download package from https://golang.org/dl/—tap the featured download link for Apple macOS and run the installer package that downloads. You may need to close any open Terminal windows to update your environment variables.
Setting up the prerequisite software on Linux should only require installing the correct packages for your distribution. The git
package will provide the source control tools and the Go language should be in the go
or golang
package. Installing these will provide the necessary commands to run the examples in this book. You may need to add ~/go/bin
to your PATH
environment variable to be able to run tools that Go installs later.