Booleans and Conditionals
Booleans, named after George Boole, take the values of True or False. Although the idea behind Booleans is rather simple, they make programming immensely more powerful.
When writing programs, for instance, it's useful to consider multiple cases. If you prompt the user for information, you may want to respond differently depending upon the user's answer.
For instance, if the user gives a rating of 0 or 1, you may give a different response than a rating of 9 or 10. The keyword here is if
.
Programming based upon multiple cases is referred to as branching. Each branch is represented by a different conditional. Conditionals often start with an 'if
' clause, followed by 'else
' clauses. The choice of a branch is determined by Booleans, depending on whether the given conditions are True
or False
.
Booleans
In Python, a Boolean class object is represented by the bool
keyword and has a value of True
or False
.
Note
Boolean values must be capitalized in Python.
Exercise 13: Boolean Variables
In this short exercise, you will use, assign, and check the type of Boolean variables:
- Open a new Jupyter Notebook.
- Now, use a Boolean to classify someone as being over
18
using the following code snippet:over_18 = True type(over_18)
You should get the following output:
bool
The output is satisfied, and the type is mentioned as a Boolean, that is,
bool
. - Use a Boolean to classify someone as not being over
21
:over_21 = False type(over_21)
You should get the following output:
bool
In this short, quick exercise, you have learned about the bool
type, one of Python's most important types.
Logical Operators
Booleans may be combined with the and, or, and not logical operators.
For instance, consider the following propositions:
A = True
B = True
Y = False
Z = False
Not simply negates the value, as follows:
not A = False
not Z = True.
And is only true if both propositions are true. Otherwise, it is false:
A and B = True
A and Y = False
Y and Z = False
Or is true if either proposition is true. Otherwise, it is false:
A or B = True
A or Y = True
Y or Z = False
Now let's use them in the following practice example.
Determine whether the following conditions are True
or False
given that over_18 = True
and over_21 = False
:
over_18
andover_21
over_18
orover_21
- not
over_18
- not
over_21
or (over_21 or over_18
)
- You have to put this into code and first assign
True
andFalse
toover_18
andover_21
:over_18, over_21 = True, False
- Next you can assume the individual is
over_18
andover_21
:over_18 and over_21
You should get the following output:
False
- You now assume the individual is
over_18
orover_21
:over_18 or over_21
You should get the following output:
True
- You now assume the individual is not
over_18
:not over_18
You should get the following output:
False
- You assume the individual is not
over_21
or (over_21 or over_18
):not over_21 or (over_21 or over_18)
You should get the following output:
True
In the next section, we will learn about the comparison operators that go along with Booleans.
Comparison Operators
Python objects may be compared using a variety of symbols that evaluate to Booleans.
Figure 1.17 shows the comparison table with their corresponding operators:
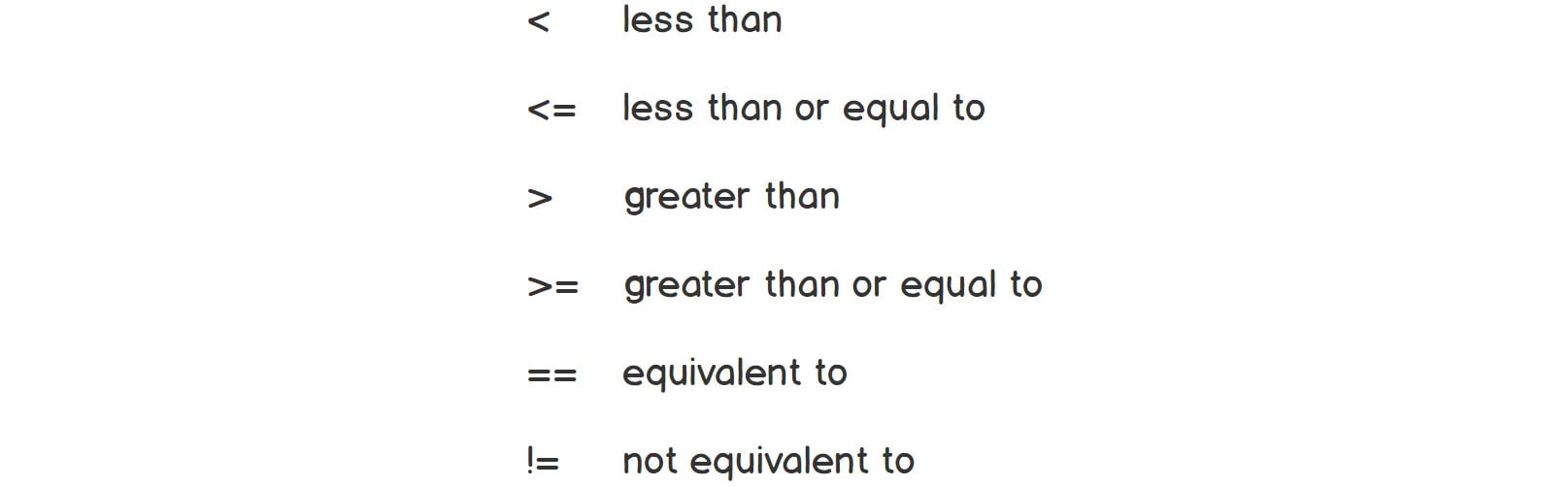
Figure 1.17: Comparison table with its corresponding symbols
Note
The =
and ==
symbols are often confused. The =
symbol is an assignment symbol. So, x = 3
assigns the integer 3
to the x
variable. The ==
symbol makes a comparison. Thus x == 3
checks to see whether x
is equivalent to 3
. The result of x == 3
will be True
or False
.
Exercise 14: Comparison Operators
In this exercise, you will practice using comparison operators. You will start with some basic mathematical examples:
- Open a new Jupyter Notebook.
- Now, set
age
as equal to20
and include a comparison operator to check whetherage
is less than13
:age = 20 age < 13
You should get the following output:
False
- Using the following code snippet, you can check whether
age
is greater than or equal to20
and less than or equal to21
:age >= 20 and age <= 21
You should get the following output:
True
- Now check whether
age
is equivalent to21
:age != 21
You should get the following output:
True
- Now, check whether
age
is equivalent to19
:age == 19
You should get the following output:
False
The double equals sign, or the equivalent operator,
==
, is very important in Python. It allows us to determine whether two objects are equal. You can now address the question of whether6
and6.0
are the same in Python. - Is
6
equivalent to6.0
in Python? Let's find out:6 == 6.0
You should get the following output:
True
This may come as a bit of a surprise.
6
and6.0
are different types, but they are equivalent. Why would that be?Since
6
and6.0
are equivalent mathematically, it makes sense that they would be equivalent in Python, even though the types are different. Consider whether 6 should be equivalent to 42/7. The mathematical answer is yes. Python often conforms to mathematical truths, even with integer division. You can conclude that it's possible for different types to have equivalent objects. - Now find out whether
6
is equivalent to the'6'
string:6 == '6'
You should get the following output:
False
Here, you emphasize that different types usually do not have equivalent objects. In general, it's a good idea to cast objects as the same type before testing for equivalence.
Next, let's find out whether someone is in their 20's or 30's:
(age >= 20 and age < 30) or (age >= 30 and age < 40)
You should get the following output:
True
Parentheses are not necessary when there is only one possible interpretation. When using more than two conditions, parentheses are generally a good idea. Note that parentheses are always permitted. The following is another approach:
(20 <= age < 30) or (30 <= age < 40)
You should get the following output:
True
Although the parentheses in the preceding code line are not strictly required, they make the code more readable. A good rule of thumb is to use parentheses for clarity.
By completing this exercise, you have practiced using different comparison operators.
Comparing Strings
Does 'a' < 'c'
make sense? What about 'New York' > 'San Francisco'
?
Python uses the convention of alphabetical order to make sense of these comparisons. Think of a dictionary: when comparing two words, the word that comes later in the dictionary is considered greater than the word that comes before.
Exercise 15: Comparing Strings
In this exercise, you will be comparing strings using Python:
- Open a new Jupyter Notebook.
- Let's compare single letters:
'a' < 'c'
You should get the following output:
True
- Now, let's compare 'New York' and 'San Francisco':
'New York' > 'San Francisco'
You should get the following output:
False
This is False
because 'New York' < 'San Francisco'
. 'New York' does not come later in the dictionary than 'San Francisco'.
In this exercise, you have learned how to compare strings using comparison operators.
Conditionals
Conditionals are used when we want to express code based upon a set of circumstances or values. Conditionals evaluate Boolean values or Boolean expressions, and they are usually preceded by 'if'
.
Let's say we are writing a voting program, and we want to print something only if the user is under 18.
The if Syntax
if age < 18: print('You aren\'t old enough to vote.')
There are several key components to a condition. Let's break them down.
The first is the 'if'
keyword. Most conditionals start with an if
clause. Everything between 'if'
and the colon is the condition that we are checking.
The next important piece is the colon :
. The colon indicates that the if
clause has completed. At this point, the compiler decides whether the preceding condition is True
or False
.
Syntactically, everything that follows the colon is indented.
Python uses indentation instead of brackets. Indentation can be advantageous when dealing with nested conditionals because it avoids cumbersome notation. Python indentation is expected to be four spaces and may usually be achieved by pressing Tab
on your keyboard.
Indented lines will only run if the condition evaluates to True
. If the condition evaluates to False
, the indented lines will be skipped over entirely.
Indentation
Indentation is one of Python's singular features. Indentation is used everywhere in Python. Indentation can be liberating. One advantage is the number of keystrokes. It takes one keystroke to tab, and two keystrokes to insert brackets. Another advantage is readability. It's clearer and easier to read code when it all shares the same indentation, meaning the block of code belongs to the same branch.
One potential drawback is that dozens of tabs may draw text offscreen, but this is rare in practice, and can usually be avoided with elegant code. Other concerns, such as indenting or unindenting multiple lines, may be handled via shortcuts. Select all of the text and press Tab
to indent. Select all of the text and press Shift + Tab
to unindent.
Note
Indentation is unique to Python. This may result in strong opinions on both sides. In practice, indentation has been shown to be very effective, and developers used to other languages will appreciate its advantages in time.
Exercise 16: Using the if Syntax
In this exercise, you will be using conditionals using the if
clause:
- Open a new Jupyter Notebook.
- Now, run multiple lines of code where you set the
age
variable to20
and add anif
clause, as mentioned in the following code snippet:age = 20 if age >= 18 and age < 21: print('At least you can vote.') print('Poker will have to wait.')
You should get the following output:
At least you can vote. Poker will have to wait.
There is no limit to the number of indented statements. Each statement will run in order, provided that the preceding condition is
True
. - Now, use nested conditionals:
if age >= 18: print('You can vote.') if age >= 21: print('You can play poker.')
You should get the following output:
You can vote.
In this case, it's true that
age >= 18
, so the first statement printsYou can vote
. The second condition, age>= 21
, however, is false, so the second statement does not get printed.
In this exercise, you have learned how to use conditionals using the if
clause. Conditionals will always start with if
.
if else
if
conditionals are commonly joined with else
clauses. The idea is as follows. Say you want to print something to all users unless the user is under 18. You can address this with an if-else
conditional. If the user is less than 18, you print one statement. Otherwise, you print another. The otherwise clause is preceded with else
.
Exercise 17: Using the if-else Syntax
In this exercise, you will learn how to use conditionals that have two options, one following if
, and one following else
:
- Open a new Jupyter Notebook.
- Introduce a voting program only to users over 18 by using the following code snippet:
age = 20 if age < 18: print('You aren\'t old enough to vote.') else: print('Welcome to our voting program.')
You should get the following output:
Welcome to our voting program.
Note
Everything after
else
is indented, just like everything after theif
loop. - Now run the following code snippet, which is an alternative to the code mentioned in step 2 of this exercise:
if age >= 18: print('Welcome to our voting program.') else: print('You aren\'t old enough to vote.')
You should get the following output:
Welcome to our voting program.
In this exercise, you have learned how to use if-else
in conjunction with loops.
There are many ways to write a program in Python. One is not necessarily better than another. It may be advantageous to write faster programs or more readable programs.
A program is a set of instructions run by a computer to complete a certain task. Programs may be one line of code, or tens of thousands. You will learn important skills and techniques for writing Python programs in various chapters throughout this book.
The elif Statement
elif
is short for else if. elif
does not have meaning in isolation. elif appears in between an if
and else
clause. An example should make things clearer. Have a look at the following code snippet and copy it into your Jupyter notebook. The explanation for this code is mentioned right after the output:
if age <= 10: print('Listen, learn, and have fun.') elif age<= 19: print('Go fearlessly forward.') elif age <= 29: print('Seize the day.') elif age <= 39: print('Go for what you want.') elif age <= 59: print('Stay physically fit and healthy.') else: print('Each day is magical.')
You should get the following output:
Seize the day.
Now, let's break down the code for a better explanation:
- The first line checks
if
age is less than or equal to10
. Since this condition is false, the next branch is checked. - The next branch is
elif
age<= 19
. This line checks if age is less than or equal to 19. This is also not true, so we move to the next branch. - The next branch is
elif
age<= 29
. This is true sinceage = 20
. The indented statement that follows will be printed. - Once any branch has been executed, the entire sequence is aborted, none of the subsequent
elif
or else branches are checked. - If none of the
if
orelif
branches were true, the finalelse
branch will automatically be executed.
In the next topic, you will be learning about loops.